The Power of Recursion: When and How to Use It
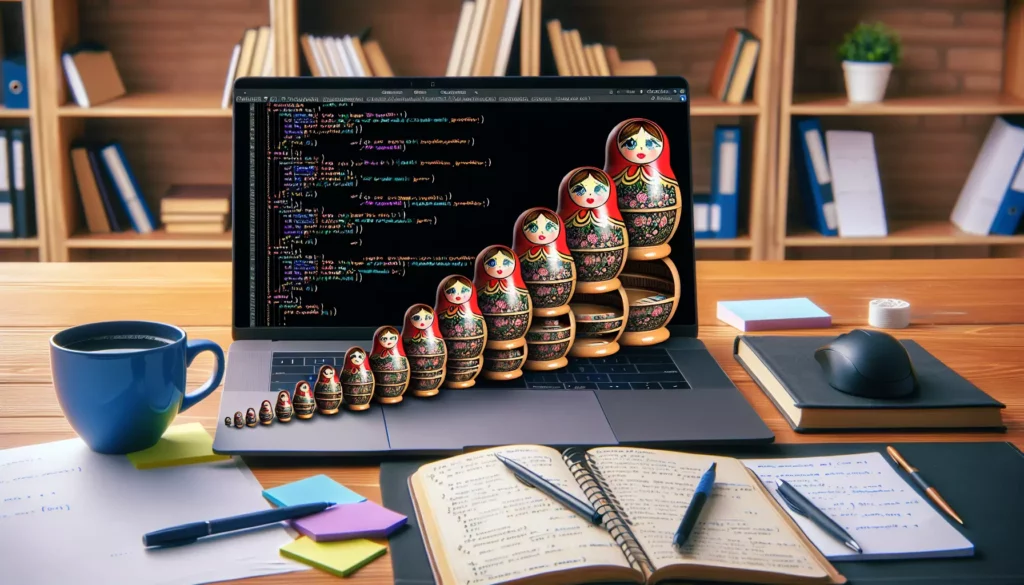
In the world of programming, there are numerous techniques and concepts that developers must master to become proficient. One such powerful concept is recursion. Whether you’re a beginner just starting your coding journey or an experienced programmer preparing for technical interviews at major tech companies, understanding recursion is crucial. In this comprehensive guide, we’ll explore the power of recursion, when to use it, and how to implement it effectively in your code.
What is Recursion?
Recursion is a programming technique where a function calls itself to solve a problem. It’s a way of breaking down a complex problem into smaller, more manageable subproblems. The function continues to call itself with modified inputs until it reaches a base case, which is a condition that stops the recursive calls.
The basic structure of a recursive function typically looks like this:
def recursive_function(parameters):
if base_case_condition:
return base_case_result
else:
return recursive_function(modified_parameters)
This structure includes two essential components:
- Base case: The condition that stops the recursion
- Recursive case: The part where the function calls itself with modified parameters
When to Use Recursion
Recursion can be a powerful tool in your programming arsenal, but it’s not always the best solution for every problem. Here are some scenarios where recursion shines:
1. Problems with a Recursive Nature
Some problems are inherently recursive in nature. These are problems that can be naturally broken down into smaller, similar subproblems. Examples include:
- Traversing tree-like data structures
- Calculating factorials
- Generating Fibonacci sequences
- Solving the Towers of Hanoi puzzle
2. Divide and Conquer Algorithms
Recursion is often used in divide and conquer algorithms, where a problem is divided into smaller subproblems, solved recursively, and then combined to solve the original problem. Examples include:
- Merge Sort
- Quick Sort
- Binary Search
3. Backtracking Algorithms
Recursion is a natural fit for backtracking algorithms, which explore all possible solutions by incrementally building candidates and abandoning those that fail to satisfy the problem’s constraints. Examples include:
- Solving Sudoku puzzles
- Finding paths in a maze
- N-Queens problem
4. Problems with Recursive Mathematical Definitions
Some mathematical concepts are defined recursively, making recursion an intuitive choice for implementing them. Examples include:
- Calculating exponents
- Computing greatest common divisor (GCD)
- Evaluating polynomial expressions
How to Use Recursion Effectively
Now that we understand when to use recursion, let’s dive into how to implement it effectively in your code.
1. Identify the Base Case
The most critical step in writing a recursive function is identifying the base case. This is the condition that will stop the recursion and prevent infinite loops. Without a proper base case, your function will continue calling itself indefinitely, leading to a stack overflow error.
Let’s look at a simple example of calculating factorial:
def factorial(n):
if n == 0 or n == 1: # Base case
return 1
else:
return n * factorial(n - 1) # Recursive case
In this example, the base case is when n is 0 or 1, as the factorial of 0 and 1 is defined as 1.
2. Ensure Progress Towards the Base Case
Each recursive call should move the problem closer to the base case. This typically involves modifying the input parameters in some way. In the factorial example above, we subtract 1 from n in each recursive call, ensuring that we eventually reach the base case.
3. Think Recursively
When designing a recursive solution, it’s helpful to think in terms of how the current problem relates to a smaller subproblem. Ask yourself: “How can I express this problem in terms of a smaller version of itself?”
For example, when implementing a recursive solution for the Fibonacci sequence, we can think of it this way:
def fibonacci(n):
if n <= 1: # Base case
return n
else:
return fibonacci(n - 1) + fibonacci(n - 2) # Recursive case
Here, we express the nth Fibonacci number as the sum of the (n-1)th and (n-2)th Fibonacci numbers.
4. Use Helper Functions When Necessary
Sometimes, you may need additional parameters to keep track of information during recursive calls. In such cases, it’s often helpful to use a helper function that contains these additional parameters.
For example, if we want to find the kth smallest element in a binary search tree, we might use a helper function like this:
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def kth_smallest(root, k):
def inorder(node):
nonlocal k
if not node:
return None
left = inorder(node.left)
if left is not None:
return left
k -= 1
if k == 0:
return node.val
return inorder(node.right)
return inorder(root)
In this example, the helper function `inorder` keeps track of the count `k` as it traverses the tree.
5. Be Mindful of Space Complexity
While recursion can lead to elegant and concise code, it’s important to be aware of its space complexity. Each recursive call adds a new frame to the call stack, which can lead to stack overflow errors for very deep recursions.
In some cases, you may need to consider tail recursion optimization or iterative solutions for better space efficiency. Tail recursion is a special form of recursion where the recursive call is the last operation in the function, allowing some compilers to optimize the stack usage.
Common Recursive Patterns
As you work with recursion more, you’ll start to recognize common patterns. Here are a few you’re likely to encounter:
1. Linear Recursion
In linear recursion, each function call makes at most one recursive call. The factorial function we saw earlier is an example of linear recursion.
2. Binary Recursion
Binary recursion involves making two recursive calls in each function call. The Fibonacci function is a classic example of binary recursion.
3. Mutual Recursion
Mutual recursion occurs when two or more functions call each other recursively. This can be useful for problems that have a natural back-and-forth structure.
Here’s an example of mutual recursion to determine if a number is even or odd:
def is_even(n):
if n == 0:
return True
else:
return is_odd(n - 1)
def is_odd(n):
if n == 0:
return False
else:
return is_even(n - 1)
4. Nested Recursion
In nested recursion, the recursive call is used as an argument to another recursive call. This is a more complex form of recursion and is less common, but can be powerful for certain problems.
An example of nested recursion is the Ackermann function:
def ackermann(m, n):
if m == 0:
return n + 1
elif n == 0:
return ackermann(m - 1, 1)
else:
return ackermann(m - 1, ackermann(m, n - 1))
Recursion vs. Iteration
While recursion can lead to elegant solutions for many problems, it’s not always the best choice. Here’s a comparison of recursion and iteration:
Advantages of Recursion:
- Can lead to cleaner, more readable code for problems with a recursive nature
- Often mirrors the mathematical definition of the problem
- Well-suited for problems involving tree-like structures
Disadvantages of Recursion:
- Can be less efficient in terms of space complexity due to the call stack
- May lead to stack overflow errors for very deep recursions
- Can be slower than iterative solutions due to function call overhead
When to Choose Recursion:
- When the problem has a natural recursive structure
- When the depth of recursion is manageable
- When code readability is a priority
When to Choose Iteration:
- When dealing with large datasets or deep recursions
- When optimizing for performance
- When the problem can be easily expressed iteratively
Common Pitfalls and How to Avoid Them
While recursion is a powerful tool, it comes with its own set of challenges. Here are some common pitfalls and how to avoid them:
1. Infinite Recursion
Infinite recursion occurs when the base case is never reached, causing the function to call itself indefinitely. To avoid this:
- Always ensure you have a well-defined base case
- Make sure each recursive call moves towards the base case
- Double-check your logic to ensure all paths eventually lead to the base case
2. Stack Overflow
Stack overflow can occur when the recursion depth is too great for the available stack space. To mitigate this:
- Consider using tail recursion when possible
- Use iterative solutions for problems with potentially deep recursion
- Implement a hybrid approach, using recursion for smaller inputs and iteration for larger ones
3. Redundant Calculations
Some recursive algorithms, like the naive implementation of Fibonacci, can lead to many redundant calculations. To optimize this:
- Use memoization to store and reuse previously calculated results
- Consider dynamic programming approaches for problems with overlapping subproblems
4. Difficulty in Debugging
Recursive functions can be more challenging to debug due to their nature. To make debugging easier:
- Use print statements or logging to track the progress of recursive calls
- Break down complex recursive functions into smaller, more manageable parts
- Use debugger tools that allow you to step through recursive calls
Real-World Applications of Recursion
Recursion isn’t just a theoretical concept; it has many practical applications in real-world programming scenarios. Here are some examples:
1. File System Operations
Recursion is often used for operations that involve traversing directory structures, such as searching for files, copying directories, or calculating disk usage.
2. Parsing and Compiling
Many parsing algorithms, such as those used in compilers and interpreters, use recursion to process nested structures in programming languages.
3. Graph Algorithms
Recursion is commonly used in graph traversal algorithms like Depth-First Search (DFS) and in solving graph-related problems.
4. Fractal Generation
Fractals, which are infinitely complex patterns that are self-similar across different scales, are often generated using recursive algorithms.
5. Game AI
Recursive algorithms like Minimax are used in game AI to determine the best move in turn-based games like chess or tic-tac-toe.
Conclusion
Recursion is a powerful and elegant technique that can lead to concise and intuitive solutions for many programming problems. By understanding when and how to use recursion effectively, you can write more efficient and readable code, especially when dealing with problems that have a naturally recursive structure.
As you continue your coding journey, whether you’re just starting out or preparing for technical interviews at major tech companies, mastering recursion will be a valuable skill in your programming toolkit. Remember to practice implementing recursive solutions, but also to critically evaluate when recursion is the best approach for a given problem.
Keep exploring, keep coding, and embrace the power of recursive thinking in your problem-solving approach!