The Power of Pseudocode: How Writing Pseudocode First Can Lead to a Better Solution
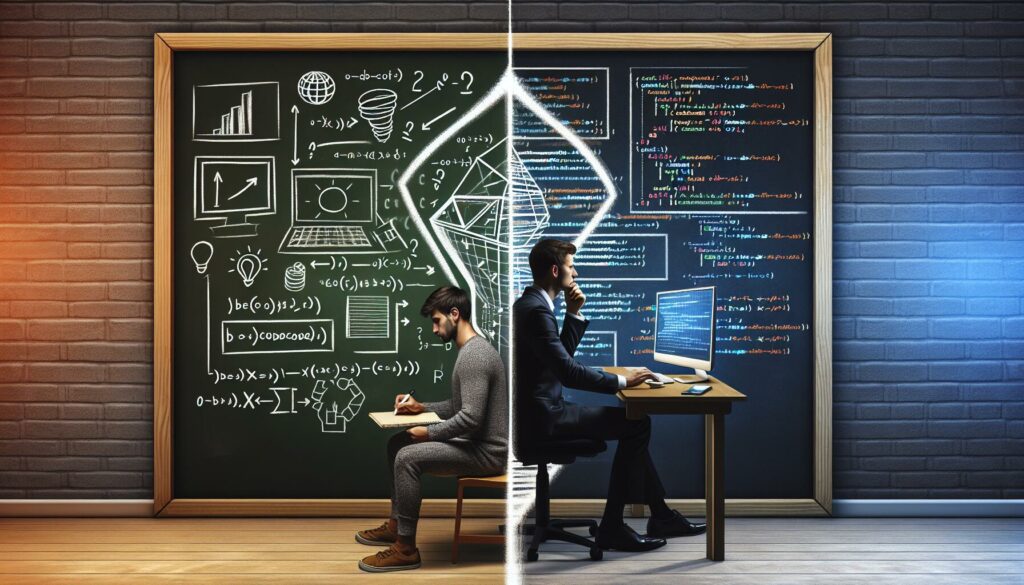
In the world of programming and software development, the journey from a problem statement to a fully functional solution can be complex and challenging. Whether you’re a seasoned developer or just starting your coding journey, one powerful technique that can significantly improve your problem-solving skills and code quality is the use of pseudocode. In this comprehensive guide, we’ll explore the importance of writing pseudocode before diving into actual coding, and how this practice can lead to better solutions, especially in high-pressure situations like technical interviews.
What is Pseudocode?
Before we delve into the benefits of pseudocode, let’s establish a clear understanding of what it is. Pseudocode is a informal, high-level description of a computer program or algorithm. It uses structured English-like statements to outline the logic and flow of a program without adhering to the strict syntax rules of any particular programming language.
The primary purpose of pseudocode is to create a blueprint or roadmap of your solution, focusing on the logic and structure rather than the specific implementation details. It serves as a bridge between human thinking and computer code, allowing developers to plan and organize their thoughts before translating them into a formal programming language.
The Benefits of Writing Pseudocode First
Now that we understand what pseudocode is, let’s explore why writing it before diving into actual coding can lead to better solutions:
1. Clarifies Thinking and Logic
Writing pseudocode forces you to think through the problem-solving process step by step. It helps you break down complex problems into smaller, more manageable chunks, making it easier to identify potential issues or gaps in your logic early on. By focusing on the high-level structure of your solution, you can avoid getting bogged down in language-specific syntax or implementation details prematurely.
2. Reduces Logical Errors
One of the most significant advantages of using pseudocode is its ability to help you catch logical errors before you start coding. By outlining your solution in a more abstract form, you can more easily spot flaws in your reasoning or identify edge cases that you might have overlooked. This early detection of logical errors can save you considerable time and frustration during the actual coding phase.
3. Improves Code Structure and Organization
Pseudocode allows you to plan the overall structure of your program or algorithm before writing any actual code. This pre-planning helps you create a more organized and modular codebase, making it easier to maintain and modify in the future. By thinking through the different components and their interactions in advance, you can design a more cohesive and efficient solution.
4. Enhances Communication
Pseudocode serves as an excellent communication tool, especially when working in teams or explaining your solution to others. Its language-agnostic nature makes it accessible to both technical and non-technical stakeholders, facilitating better collaboration and understanding among team members. In a technical interview setting, clearly articulating your thought process through pseudocode can demonstrate your problem-solving skills and ability to communicate complex ideas effectively.
5. Accelerates the Coding Process
While it might seem counterintuitive, spending time on pseudocode can actually speed up the overall development process. By having a clear roadmap of your solution, you can write code more efficiently, with fewer interruptions to rethink your approach or debug logical errors. This is particularly valuable in time-constrained situations like coding interviews or when working on tight project deadlines.
6. Facilitates Language-Independent Problem Solving
Pseudocode allows you to focus on the core logic and algorithm of your solution without being constrained by the syntax or features of a specific programming language. This language-independent approach helps develop your problem-solving skills in a more versatile way, making it easier to adapt your solutions to different programming languages or environments as needed.
How to Write Effective Pseudocode
Now that we’ve established the benefits of using pseudocode, let’s discuss some tips and best practices for writing clear and concise pseudocode, especially in high-pressure situations like technical interviews:
1. Use Clear and Consistent Language
While pseudocode doesn’t follow strict syntax rules, it’s important to use clear and consistent language throughout your pseudocode. Stick to common programming constructs and keywords that are easily understood, such as:
- IF, ELSE, ELSE IF for conditional statements
- FOR, WHILE for loops
- FUNCTION or PROCEDURE for defining functions
- INPUT and OUTPUT for handling data input and output
- RETURN for specifying function return values
Example:
FUNCTION findMaxElement(arr)
IF arr is empty
RETURN null
END IF
max = arr[0]
FOR each element in arr
IF element > max
max = element
END IF
END FOR
RETURN max
END FUNCTION
2. Keep It Concise but Informative
Aim for a balance between brevity and clarity in your pseudocode. Provide enough detail to convey the logic of your solution without getting bogged down in unnecessary specifics. Use indentation and line breaks to improve readability and structure.
3. Use Descriptive Variable and Function Names
Choose meaningful names for variables, functions, and procedures in your pseudocode. This practice not only makes your pseudocode more readable but also translates well when you start writing actual code. Avoid single-letter variable names unless they’re used in well-known contexts (e.g., ‘i’ for loop counters).
4. Include Comments and Explanations
While pseudocode itself is meant to be self-explanatory, don’t hesitate to include comments or brief explanations for complex logic or important decisions. This can be particularly helpful in interview settings where you want to demonstrate your thought process clearly.
5. Start with High-Level Steps
Begin your pseudocode with a high-level overview of your solution, then gradually add more detail. This top-down approach helps you maintain a clear structure and allows you to adjust your strategy easily if needed.
Example:
FUNCTION sortArray(arr)
// 1. Choose a pivot element
// 2. Partition the array around the pivot
// 3. Recursively sort the left and right subarrays
// 4. Combine the sorted subarrays
// Detailed implementation...
END FUNCTION
6. Use Abstraction When Appropriate
Don’t feel obligated to detail every single operation in your pseudocode. Use abstraction for well-known operations or sub-procedures to keep your pseudocode focused on the main logic of your solution.
7. Iterate and Refine
As you develop your pseudocode, don’t be afraid to iterate and refine your approach. The beauty of pseudocode is its flexibility, allowing you to quickly adjust your strategy without the overhead of rewriting actual code.
Pseudocode in Technical Interviews: A Practical Approach
Technical interviews can be high-pressure situations where time is limited, and the ability to think clearly and communicate effectively is crucial. Here’s a step-by-step approach to using pseudocode effectively in interview settings:
1. Clarify the Problem
Before you start writing any pseudocode, make sure you fully understand the problem. Ask clarifying questions about input formats, expected outputs, constraints, and edge cases. This information will help you craft a more accurate and efficient solution.
2. Outline the High-Level Approach
Start by writing a brief, high-level outline of your approach. This serves two purposes: it helps you organize your thoughts and demonstrates to the interviewer that you have a clear strategy in mind.
3. Write Pseudocode for Key Components
Develop pseudocode for the main components of your solution. Focus on the core logic and algorithms, using clear and concise language. Remember to think out loud as you write, explaining your reasoning to the interviewer.
4. Discuss Time and Space Complexity
As you develop your pseudocode, consider and discuss the time and space complexity of your solution. This shows the interviewer that you’re thinking about efficiency and scalability.
5. Refine and Optimize
If time allows, look for opportunities to refine and optimize your solution. Discuss potential improvements or alternative approaches with the interviewer, using your pseudocode as a reference point.
6. Transition to Actual Code
Once you and the interviewer are satisfied with your pseudocode solution, begin translating it into actual code. Your well-structured pseudocode will serve as an excellent guide, making this process smoother and more efficient.
Common Pitfalls to Avoid When Writing Pseudocode
While pseudocode is a powerful tool, there are some common mistakes to avoid:
1. Being Too Vague
While pseudocode should be high-level, it shouldn’t be so vague that it fails to communicate the essential logic of your solution. Strike a balance between abstraction and specificity.
2. Using Too Much Programming Language Syntax
Remember that pseudocode is meant to be language-agnostic. Avoid using too much syntax specific to a particular programming language, as this can defeat the purpose of writing pseudocode.
3. Neglecting Error Handling and Edge Cases
Don’t forget to include basic error handling and consider edge cases in your pseudocode. This demonstrates thoroughness and attention to detail.
4. Overcomplicating Simple Concepts
For simple operations or well-known algorithms, it’s okay to use a higher level of abstraction. Don’t get bogged down in detailing every step of a standard sorting algorithm, for example.
5. Not Iterating on Your Pseudocode
Treat your pseudocode as a living document. Be prepared to revise and refine it as you think through the problem more deeply or receive feedback from interviewers or colleagues.
Conclusion: Embracing the Power of Pseudocode
In the fast-paced world of software development, where efficiency and clarity are paramount, the practice of writing pseudocode before diving into actual coding stands out as a powerful technique for crafting better solutions. By clarifying your thinking, reducing logical errors, improving code structure, enhancing communication, and accelerating the overall development process, pseudocode serves as an invaluable tool in a programmer’s arsenal.
Whether you’re tackling a complex algorithm, preparing for a technical interview, or collaborating on a large-scale project, the ability to articulate your ideas clearly through pseudocode can set you apart as a thoughtful and effective problem solver. By following the tips and best practices outlined in this guide, you can harness the full potential of pseudocode to elevate your programming skills and produce more robust, efficient, and maintainable code.
Remember, the goal of pseudocode is not to replace actual coding but to complement and enhance the development process. By integrating pseudocode into your problem-solving toolkit, you’re investing in a skill that will serve you well throughout your programming career, helping you tackle challenges with greater confidence and clarity.
So the next time you’re faced with a coding challenge, take a step back, grab a pen and paper (or open a text editor), and start with pseudocode. You might be surprised at how this simple practice can transform your approach to problem-solving and lead you to better, more elegant solutions.