The Power of Modular Arithmetic in Problem Solving
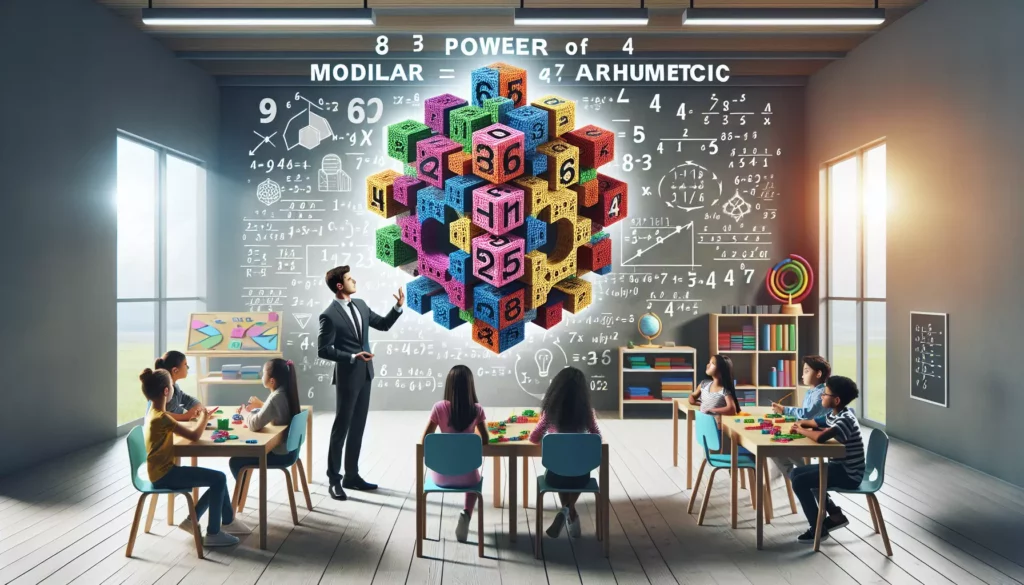
In the world of coding and algorithmic problem-solving, modular arithmetic stands out as a powerful tool that can simplify complex calculations and provide elegant solutions to a wide range of problems. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at top tech companies, understanding and mastering modular arithmetic can give you a significant edge. In this comprehensive guide, we’ll explore the concept of modular arithmetic, its applications in computer science, and how it can be leveraged to solve various coding challenges.
What is Modular Arithmetic?
Modular arithmetic, also known as clock arithmetic, is a system of arithmetic for integers where numbers “wrap around” upon reaching a certain value—the modulus. In simpler terms, it’s a way of performing arithmetic operations where the result is always expressed as the remainder when divided by a fixed number (the modulus).
The most common real-world example of modular arithmetic is the 12-hour clock. When it’s 10 o’clock and 5 hours pass, we don’t say it’s 15 o’clock; instead, we say it’s 3 o’clock. This is because in a 12-hour clock system, we perform arithmetic modulo 12.
In mathematical notation, we express modular arithmetic using the congruence symbol (≡). For example:
14 ≡ 2 (mod 12)
This reads as “14 is congruent to 2 modulo 12” or “14 and 2 have the same remainder when divided by 12”.
Basic Operations in Modular Arithmetic
Let’s look at how basic arithmetic operations work in modular arithmetic:
1. Addition
(a + b) mod m = ((a mod m) + (b mod m)) mod m
Example: (15 + 17) mod 7 = (1 + 3) mod 7 = 4 mod 7 = 4
2. Subtraction
(a – b) mod m = ((a mod m) – (b mod m) + m) mod m
Example: (15 – 17) mod 7 = ((1 – 3 + 7) mod 7 = 5 mod 7 = 5
3. Multiplication
(a * b) mod m = ((a mod m) * (b mod m)) mod m
Example: (15 * 17) mod 7 = (1 * 3) mod 7 = 3 mod 7 = 3
4. Exponentiation
a^b mod m = ((a mod m)^b) mod m
Example: 3^4 mod 5 = 81 mod 5 = 1
Applications of Modular Arithmetic in Computer Science
Modular arithmetic has numerous applications in computer science and programming. Here are some key areas where it proves invaluable:
1. Hashing
Hash functions, which are crucial in data structures like hash tables, often use modular arithmetic to map keys to array indices. The modulo operation helps in distributing keys uniformly across the array.
2. Cryptography
Many cryptographic algorithms rely heavily on modular arithmetic. For instance, the RSA algorithm, one of the first public-key cryptosystems, is based on modular exponentiation.
3. Random Number Generation
Linear congruential generators, a class of pseudorandom number generators, use modular arithmetic to produce sequences of numbers that appear random.
4. Cyclic Redundancy Check (CRC)
CRC, used for error detection in digital networks and storage devices, performs polynomial division using modular arithmetic.
5. Clock Arithmetic
When dealing with time-based calculations, such as adding hours to a given time, modular arithmetic (usually modulo 24 or 12) is often employed.
Problem-Solving with Modular Arithmetic
Now, let’s dive into some practical problem-solving scenarios where modular arithmetic can be applied effectively.
Problem 1: The Josephus Problem
The Josephus Problem is a theoretical problem related to a circular arrangement of n people, numbered from 1 to n. Starting from the first person, every k-th person is eliminated until only one person remains. The task is to find the position of the survivor.
This problem can be solved efficiently using modular arithmetic. Here’s a Python implementation:
def josephus(n, k):
survivor = 0
for i in range(1, n + 1):
survivor = (survivor + k) % i
return survivor + 1
# Example usage
n = 7
k = 3
result = josephus(n, k)
print(f"For n = {n} and k = {k}, the survivor is at position {result}")
In this solution, we use the modulo operation to simulate the circular nature of the problem, efficiently calculating the survivor’s position without actually simulating the entire process.
Problem 2: Fibonacci Numbers Modulo M
Calculating large Fibonacci numbers can quickly lead to integer overflow. However, if we’re only interested in the remainder when divided by a number M, we can use modular arithmetic to keep our calculations manageable.
Here’s a Python function to calculate the nth Fibonacci number modulo M:
def fibonacci_mod(n, m):
if n <= 1:
return n
a, b = 0, 1
for _ in range(2, n + 1):
a, b = b, (a + b) % m
return b
# Example usage
n = 1000
m = 10**9 + 7
result = fibonacci_mod(n, m)
print(f"The {n}th Fibonacci number modulo {m} is {result}")
This implementation uses modular arithmetic to keep the numbers small throughout the calculation, allowing us to compute very large Fibonacci numbers efficiently.
Problem 3: Modular Exponentiation
Calculating large powers can result in enormous numbers that are difficult to handle. Modular exponentiation allows us to compute (x^n) mod m efficiently, even for very large values of n.
Here’s an efficient implementation using the square-and-multiply algorithm:
def mod_pow(base, exponent, modulus):
result = 1
base = base % modulus
while exponent > 0:
if exponent % 2 == 1:
result = (result * base) % modulus
exponent = exponent >> 1
base = (base * base) % modulus
return result
# Example usage
x = 2
n = 1000000
m = 1000000007
result = mod_pow(x, n, m)
print(f"{x}^{n} mod {m} = {result}")
This algorithm has a time complexity of O(log n), making it much more efficient than naively computing x^n and then taking the modulus.
Advanced Concepts in Modular Arithmetic
As you delve deeper into modular arithmetic, you’ll encounter more advanced concepts that are particularly useful in competitive programming and cryptography:
1. Modular Multiplicative Inverse
The modular multiplicative inverse of an integer a modulo m is an integer x such that:
ax ≡ 1 (mod m)
This concept is crucial in various algorithms and cryptographic protocols. It can be computed efficiently using the extended Euclidean algorithm:
def extended_gcd(a, b):
if a == 0:
return b, 0, 1
else:
gcd, x, y = extended_gcd(b % a, a)
return gcd, y - (b // a) * x, x
def mod_inverse(a, m):
gcd, x, _ = extended_gcd(a, m)
if gcd != 1:
raise ValueError("Modular inverse does not exist")
else:
return x % m
# Example usage
a = 3
m = 11
inv = mod_inverse(a, m)
print(f"The modular multiplicative inverse of {a} modulo {m} is {inv}")
2. Chinese Remainder Theorem (CRT)
The Chinese Remainder Theorem is a powerful tool in number theory that allows us to solve systems of simultaneous congruences. It has applications in various areas, including cryptography and parallel computation.
Here’s a Python implementation of the Chinese Remainder Theorem:
def chinese_remainder_theorem(remainders, moduli):
total = 0
product = 1
for modulus in moduli:
product *= modulus
for remainder, modulus in zip(remainders, moduli):
p = product // modulus
total += remainder * mod_inverse(p, modulus) * p
return total % product
# Example usage
remainders = [2, 3, 2]
moduli = [3, 5, 7]
result = chinese_remainder_theorem(remainders, moduli)
print(f"The solution to the system of congruences is {result}")
3. Euler’s Totient Function
Euler’s totient function, denoted as φ(n), counts the number of integers up to n that are coprime to n. It’s an important concept in number theory and has applications in cryptography, particularly in the RSA algorithm.
Here’s a simple implementation to calculate Euler’s totient function:
def euler_totient(n):
result = n
for i in range(2, int(n**0.5) + 1):
if n % i == 0:
while n % i == 0:
n //= i
result *= (1 - 1/i)
if n > 1:
result *= (1 - 1/n)
return int(result)
# Example usage
n = 36
phi = euler_totient(n)
print(f"φ({n}) = {phi}")
Modular Arithmetic in Competitive Programming
Modular arithmetic is a staple in competitive programming, often used to handle large numbers and avoid overflow issues. Here are some tips for using modular arithmetic effectively in coding competitions:
1. Use a Large Prime Modulus
When the problem doesn’t specify a modulus, it’s common to use a large prime number like 10^9 + 7. This helps avoid collisions and maintains the distribution of results.
2. Precompute Modular Inverses
If you need to perform many divisions modulo m, precomputing modular inverses can save time during the actual computation.
3. Handle Negative Numbers
Remember that the result of a modulo operation can be negative in some programming languages. To ensure a positive result, you can use:
((a % m) + m) % m
4. Use Built-in Modulo Operators
Many programming languages have built-in modulo operators that are optimized for performance. In Python, for example, the % operator performs modular arithmetic.
Conclusion
Modular arithmetic is a powerful tool in the arsenal of any programmer or computer scientist. Its applications range from basic problem-solving to advanced cryptographic protocols. By understanding and mastering modular arithmetic, you can tackle a wide range of coding challenges more efficiently and elegantly.
As you continue your journey in coding education and skills development, remember that concepts like modular arithmetic are fundamental building blocks that can significantly enhance your problem-solving abilities. Whether you’re preparing for technical interviews at major tech companies or simply looking to improve your algorithmic thinking, investing time in understanding and practicing modular arithmetic will undoubtedly pay dividends.
Keep exploring, keep practicing, and don’t hesitate to dive into more advanced topics as you grow more comfortable with the basics. The world of algorithms and data structures is vast and fascinating, and modular arithmetic is just one of many powerful tools at your disposal. Happy coding!