The Power of Mentorship in Accelerating Your Coding Journey
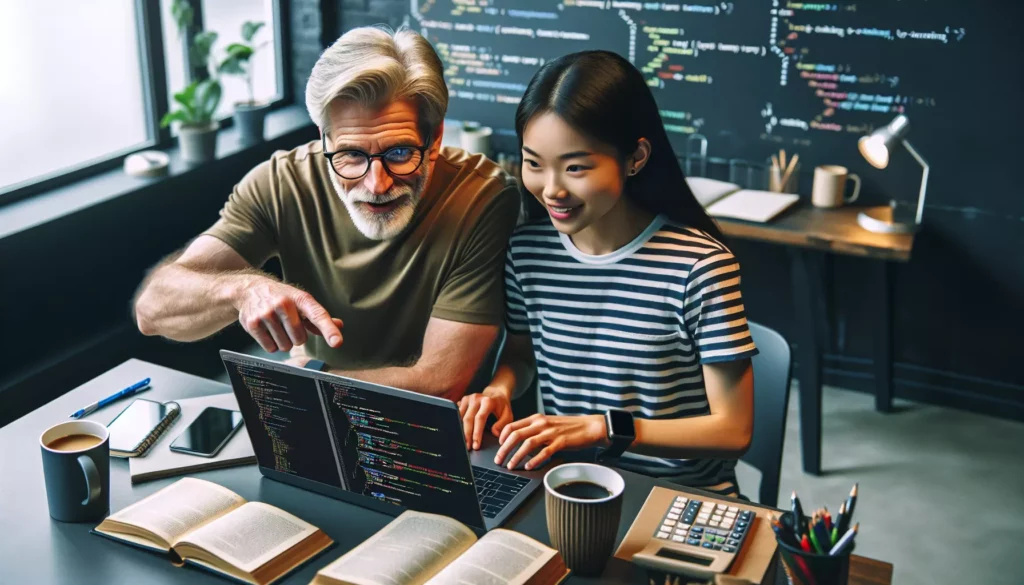
In the fast-paced world of technology and software development, the journey from novice coder to proficient programmer can be both exciting and challenging. While there are numerous resources available for self-study, including online courses, tutorials, and coding bootcamps, one often overlooked yet incredibly powerful tool for accelerating your coding journey is mentorship. In this comprehensive guide, we’ll explore the immense benefits of having a mentor, how to find the right mentor, and how to make the most of this invaluable relationship as you progress in your coding career.
The Importance of Mentorship in Coding
Mentorship has long been recognized as a crucial element in personal and professional development across various fields, and coding is no exception. Here are some key reasons why mentorship is particularly important in the world of programming:
1. Accelerated Learning
One of the primary benefits of having a mentor is the potential for accelerated learning. A mentor can help you navigate the vast landscape of programming languages, frameworks, and technologies, guiding you towards the most relevant and in-demand skills. They can share their experiences, point out common pitfalls, and provide shortcuts that might take years to discover on your own.
2. Real-world Insights
While coding tutorials and courses provide a solid foundation, they often lack the real-world context that comes from years of industry experience. A mentor can bridge this gap by sharing practical insights, best practices, and industry trends that aren’t typically covered in formal education.
3. Personalized Guidance
Every aspiring programmer has unique strengths, weaknesses, and career goals. A mentor can provide personalized guidance tailored to your specific needs, helping you focus on areas that require improvement and leveraging your strengths to accelerate your progress.
4. Networking Opportunities
Mentors often have extensive professional networks within the tech industry. By fostering a relationship with a mentor, you gain access to these networks, potentially opening doors to job opportunities, collaborative projects, and valuable connections with other professionals in the field.
5. Motivation and Accountability
The journey to becoming a proficient coder can be challenging, and it’s easy to lose motivation or become overwhelmed. A mentor can provide encouragement, set realistic goals, and hold you accountable for your progress, helping you stay on track and maintain momentum.
Finding the Right Mentor
Now that we’ve established the importance of mentorship in coding, let’s explore how to find the right mentor to guide you on your journey:
1. Identify Your Goals
Before seeking a mentor, it’s crucial to have a clear understanding of your goals. Are you looking to break into the tech industry? Do you want to specialize in a particular area of programming? Knowing your objectives will help you find a mentor whose expertise aligns with your aspirations.
2. Leverage Online Platforms
There are several online platforms dedicated to connecting mentors and mentees in the tech industry. Some popular options include:
- Codementor
- MentorCruise
- ADPList
These platforms allow you to browse profiles of potential mentors, read reviews, and connect with professionals who match your interests and goals.
3. Attend Tech Meetups and Conferences
Local tech meetups and industry conferences are excellent opportunities to network with experienced developers who might be interested in mentoring. Engaging in conversations and showing genuine interest in their work can lead to valuable mentorship relationships.
4. Reach Out to Your Network
Don’t underestimate the power of your existing network. Reach out to colleagues, friends, or acquaintances who work in the tech industry. They might be willing to mentor you directly or introduce you to potential mentors in their network.
5. Consider Formal Mentorship Programs
Many tech companies and professional organizations offer formal mentorship programs. These structured programs can provide a more systematic approach to mentorship, often with defined goals and timelines.
Making the Most of Your Mentorship
Once you’ve found a mentor, it’s essential to make the most of this valuable relationship. Here are some tips to ensure a productive mentorship experience:
1. Set Clear Expectations
From the outset, establish clear expectations with your mentor regarding the frequency of meetings, communication methods, and goals for the mentorship. This clarity will help both you and your mentor stay aligned and focused.
2. Come Prepared
Make the most of your mentor’s time by coming to each meeting prepared with specific questions, challenges you’re facing, or topics you’d like to discuss. This preparation demonstrates your commitment and helps keep the mentorship focused and productive.
3. Be Open to Feedback
One of the most valuable aspects of mentorship is receiving honest feedback. Be open to constructive criticism and view it as an opportunity for growth. Remember that your mentor’s insights come from years of experience and can significantly accelerate your learning.
4. Take Action on Advice
When your mentor provides advice or suggests resources, make a concerted effort to act on these recommendations. Demonstrating that you value and implement their guidance will strengthen the mentorship relationship and lead to more meaningful progress.
5. Share Your Progress
Keep your mentor updated on your progress, achievements, and any challenges you’re facing. This ongoing communication helps your mentor provide more targeted guidance and allows them to celebrate your successes along the way.
Leveraging Mentorship in Your Coding Projects
One of the most effective ways to apply mentorship to your coding journey is through hands-on projects. Here’s how you can leverage your mentor’s expertise in your coding projects:
1. Code Reviews
Ask your mentor to review your code for projects you’re working on. Their feedback can help you improve your coding style, identify potential bugs, and learn best practices. Here’s an example of how a mentor might provide feedback on a simple Python function:
# Original code
def calculate_average(numbers):
sum = 0
for num in numbers:
sum += num
return sum / len(numbers)
# Mentor's feedback:
# 1. Consider using a more descriptive variable name than 'sum'
# 2. You can use the built-in sum() function for better readability
# 3. Add error handling for empty lists
# Improved code
def calculate_average(numbers):
if not numbers:
raise ValueError("Cannot calculate average of an empty list")
total = sum(numbers)
return total / len(numbers)
2. Architecture Discussions
When starting a new project, discuss your planned architecture with your mentor. They can provide insights on scalability, maintainability, and potential pitfalls you might not have considered. For example, they might suggest using a design pattern that’s particularly well-suited for your project:
# Mentor's suggestion: Using the Observer pattern for a logging system
class Subject:
def __init__(self):
self._observers = []
def attach(self, observer):
self._observers.append(observer)
def detach(self, observer):
self._observers.remove(observer)
def notify(self, message):
for observer in self._observers:
observer.update(message)
class Logger(Subject):
def log(self, message):
print(f"Logging: {message}")
self.notify(message)
class FileObserver:
def update(self, message):
with open("log.txt", "a") as f:
f.write(f"{message}\n")
class EmailObserver:
def update(self, message):
print(f"Sending email: {message}")
# Usage
logger = Logger()
logger.attach(FileObserver())
logger.attach(EmailObserver())
logger.log("Important event occurred")
3. Problem-Solving Sessions
When facing a particularly challenging problem, schedule a problem-solving session with your mentor. They can guide you through their thought process, teaching you valuable problem-solving strategies. For instance, they might introduce you to the concept of dynamic programming for optimizing recursive solutions:
# Original recursive solution for Fibonacci sequence
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n-1) + fibonacci(n-2)
# Mentor's suggestion: Using dynamic programming
def fibonacci_dp(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
# Usage
print(fibonacci_dp(100)) # Much faster than the recursive version
4. Technology Selection
When choosing technologies for your projects, consult with your mentor. They can provide insights into the pros and cons of different technologies based on your project requirements and career goals. For example, they might advise on choosing between different web frameworks:
# Mentor's advice on choosing a web framework
"""
For a small, fast API project:
- Flask: Lightweight, easy to get started, great for microservices
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/api/data')
def get_data():
return jsonify({'message': 'Hello, World!'})
For a large, feature-rich web application:
- Django: Full-featured, includes ORM, admin interface, and more
from django.http import JsonResponse
from django.views import View
class DataView(View):
def get(self, request):
return JsonResponse({'message': 'Hello, World!'})
Consider your project's scale, team's expertise, and long-term maintenance when choosing.
"""
The Long-Term Impact of Mentorship
As you progress in your coding journey, the impact of mentorship extends far beyond just learning to code. Here are some long-term benefits you can expect:
1. Career Advancement
A mentor can provide valuable advice on career progression, helping you navigate job changes, salary negotiations, and professional development opportunities. Their industry insights can be invaluable when making important career decisions.
2. Leadership Skills
As you grow in your career, you may find yourself in leadership positions. The lessons learned from your mentor can help you become an effective leader and potentially a mentor to others in the future.
3. Continuous Learning
A good mentor instills a passion for continuous learning. They can introduce you to new technologies, methodologies, and industry trends, ensuring that you stay current in the ever-evolving world of technology.
4. Professional Network
Over time, the professional network you build through your mentor can lead to collaborations, job opportunities, and even potential business partnerships.
5. Confidence and Self-Efficacy
Regular guidance and support from a mentor can significantly boost your confidence in your coding abilities. This increased self-efficacy can lead to taking on more challenging projects and roles in your career.
Overcoming Common Mentorship Challenges
While mentorship can be incredibly beneficial, it’s not without its challenges. Here are some common issues you might face and how to overcome them:
1. Time Constraints
Both you and your mentor likely have busy schedules. To address this:
- Set a regular meeting schedule that works for both of you
- Use asynchronous communication methods like email or messaging apps for quick questions
- Be respectful of your mentor’s time by coming prepared to each meeting
2. Mismatched Expectations
If you find that your expectations don’t align with your mentor’s:
- Have an open discussion about goals and expectations
- Be willing to adjust your expectations or seek additional resources if needed
- Consider finding a new mentor if the mismatch is significant
3. Dependency
Avoid becoming overly dependent on your mentor:
- Use your mentor as a guide, but take responsibility for your own learning
- Practice problem-solving on your own before seeking help
- Gradually increase the time between mentorship sessions as you become more proficient
4. Keeping the Relationship Fresh
To maintain a dynamic and engaging mentorship:
- Regularly reassess and update your goals
- Bring new challenges or technologies to discuss
- Share your own insights and discoveries with your mentor
Conclusion: Embracing Mentorship for Coding Success
The journey from beginner to proficient coder is filled with challenges, opportunities, and constant learning. While self-study and formal education play crucial roles in this journey, the power of mentorship should not be underestimated. A good mentor can provide personalized guidance, industry insights, and accelerated learning opportunities that are difficult to obtain through other means.
By finding the right mentor, establishing clear goals, and actively engaging in the mentorship process, you can significantly accelerate your coding journey. Remember that mentorship is a two-way relationship that requires commitment, open communication, and a willingness to learn and grow.
As you progress in your coding career, consider not only the benefits you receive from mentorship but also how you can pay it forward. As you gain experience and expertise, you may find yourself in a position to mentor others, continuing the cycle of knowledge sharing and professional development in the tech community.
Embrace the power of mentorship, and watch as it transforms not only your coding skills but also your overall career trajectory in the exciting world of technology and software development.