The Power of First Principles Thinking in Coding: Solve Any Problem from Scratch
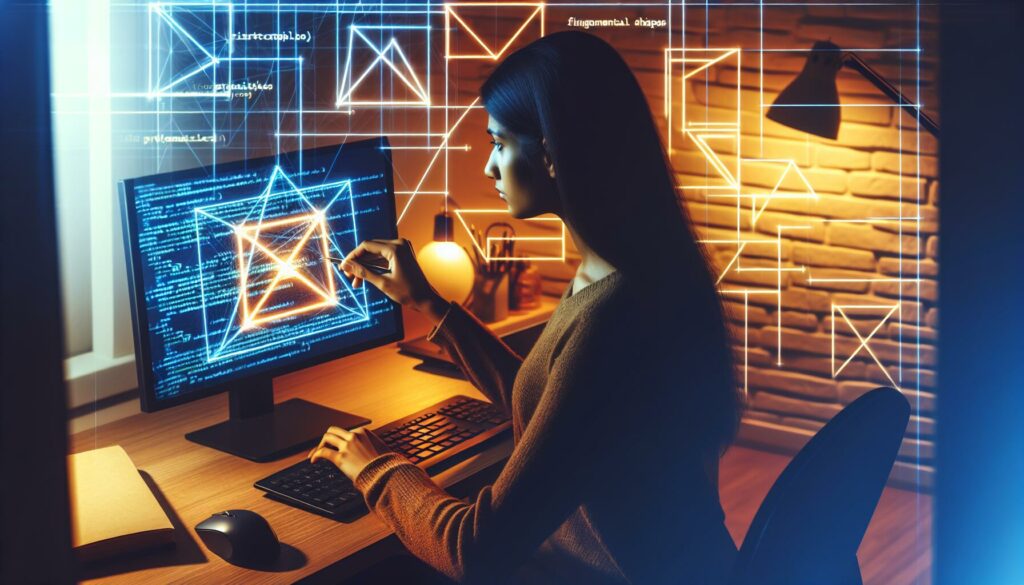
In the ever-evolving world of programming, developers are constantly faced with new challenges and complex problems. Whether you’re a beginner just starting your coding journey or an experienced programmer preparing for technical interviews at top tech companies, having a powerful problem-solving approach can make all the difference. Enter first principles thinking – a method championed by innovators like Elon Musk that can revolutionize the way you approach coding challenges.
What is First Principles Thinking?
First principles thinking is a problem-solving approach that involves breaking down complex problems into their most basic, fundamental truths and then reassembling them from the ground up. Instead of relying on analogies or previous solutions, this method encourages you to question assumptions and build solutions based on core facts.
Elon Musk, the entrepreneur behind companies like SpaceX and Tesla, has famously used this approach to tackle seemingly impossible challenges. He explains it as “boiling things down to their fundamental truths and then reasoning up from there.”
How First Principles Thinking Applies to Coding
In the context of programming, first principles thinking can be an incredibly powerful tool. It allows developers to approach problems without being constrained by existing solutions or conventional wisdom. Here’s how you can apply this method to your coding practice:
- Identify the problem: Clearly define what you’re trying to solve.
- Break it down: Deconstruct the problem into its most basic components.
- Question assumptions: Challenge any preconceived notions about the problem or potential solutions.
- Identify fundamental truths: Determine the core facts or principles related to the problem.
- Build from the ground up: Use these fundamental truths to construct a solution.
The Benefits of First Principles Thinking in Coding
Applying first principles thinking to your coding practice can yield numerous benefits:
- Enhanced problem-solving skills: By breaking problems down to their core components, you’ll develop a deeper understanding of the challenges you face.
- Increased creativity: Freeing yourself from established solutions allows for more innovative approaches.
- Better adaptability: You’ll be better equipped to tackle unfamiliar problems or rapidly changing technologies.
- Improved code quality: Solutions built from first principles are often more elegant and efficient.
- Stronger foundational knowledge: Regularly applying this method will strengthen your understanding of fundamental programming concepts.
Practical Examples of First Principles Thinking in Coding
Let’s explore some concrete examples of how first principles thinking can be applied to common coding challenges:
1. Sorting Algorithms
Instead of immediately jumping to implement a well-known sorting algorithm like quicksort or mergesort, start by considering the fundamental problem: arranging elements in a specific order.
Breaking it down to first principles:
- We have a collection of elements.
- Each element can be compared to others.
- We need to arrange these elements based on their relative order.
From these basic truths, you might derive a simple sorting algorithm like bubble sort:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
return arr
While bubble sort may not be the most efficient algorithm, deriving it from first principles helps build a strong foundation for understanding more complex sorting methods.
2. String Reversal
When faced with the task of reversing a string, instead of immediately using a built-in function or a common solution, break down the problem:
- A string is a sequence of characters.
- Each character has a position in the sequence.
- Reversing means the last character becomes first, the second-to-last becomes second, and so on.
From these principles, you might develop a solution like this:
def reverse_string(s):
reversed_chars = []
for i in range(len(s) - 1, -1, -1):
reversed_chars.append(s[i])
return ''.join(reversed_chars)
3. Fibonacci Sequence
When asked to generate the Fibonacci sequence, start with the basic definition:
- The sequence starts with 0 and 1.
- Each subsequent number is the sum of the two preceding ones.
From these principles, you can derive a simple recursive solution:
def fibonacci(n):
if n <= 1:
return n
else:
return fibonacci(n-1) + fibonacci(n-2)
While this solution may not be the most efficient for large values of n, it directly translates the fundamental principles of the Fibonacci sequence into code.
Applying First Principles Thinking to Advanced Coding Challenges
As you progress in your coding journey and tackle more complex problems, first principles thinking becomes even more valuable. Let’s look at how this approach can be applied to some advanced coding challenges:
1. Designing a Cache System
When tasked with designing a cache system, start by breaking down the fundamental requirements:
- A cache stores data for quick retrieval.
- It has a limited capacity.
- It needs to handle both reading and writing operations.
- There should be a strategy for removing items when the cache is full.
From these principles, you might start by implementing a basic Least Recently Used (LRU) cache:
from collections import OrderedDict
class LRUCache:
def __init__(self, capacity):
self.cache = OrderedDict()
self.capacity = capacity
def get(self, key):
if key not in self.cache:
return -1
else:
self.cache.move_to_end(key)
return self.cache[key]
def put(self, key, value):
if key in self.cache:
self.cache.move_to_end(key)
self.cache[key] = value
if len(self.cache) > self.capacity:
self.cache.popitem(last=False)
This implementation directly addresses each of the fundamental requirements we identified.
2. Implementing a Basic Database
If asked to implement a simple database system, consider the core principles:
- Data needs to be stored persistently.
- Data should be retrievable by some kind of identifier.
- The system should support basic operations like create, read, update, and delete (CRUD).
Based on these principles, you might start with a simple file-based system:
import json
import os
class SimpleDB:
def __init__(self, filename):
self.filename = filename
self.data = {}
if os.path.exists(filename):
with open(filename, 'r') as f:
self.data = json.load(f)
def create(self, key, value):
if key not in self.data:
self.data[key] = value
self._save()
return True
return False
def read(self, key):
return self.data.get(key)
def update(self, key, value):
if key in self.data:
self.data[key] = value
self._save()
return True
return False
def delete(self, key):
if key in self.data:
del self.data[key]
self._save()
return True
return False
def _save(self):
with open(self.filename, 'w') as f:
json.dump(self.data, f)
# Usage
db = SimpleDB('mydb.json')
db.create('user1', {'name': 'Alice', 'age': 30})
print(db.read('user1'))
db.update('user1', {'name': 'Alice', 'age': 31})
db.delete('user1')
This basic implementation satisfies the core requirements of data storage, retrieval, and CRUD operations.
Overcoming Challenges with First Principles Thinking
While first principles thinking is a powerful tool, it’s not without its challenges. Here are some common obstacles you might face when applying this method to coding, and strategies to overcome them:
1. Overcoming Ingrained Patterns
Challenge: As programmers, we often develop habits and go-to solutions. It can be difficult to step back and approach a problem from first principles.
Solution: Practice deliberately setting aside your initial impulses. Before diving into coding, take time to write down the fundamental truths of the problem. This can help reset your thinking and open up new possibilities.
2. Dealing with Time Constraints
Challenge: In time-pressured situations like coding interviews or tight project deadlines, it might seem faster to rely on familiar solutions.
Solution: Remember that investing time in first principles thinking often leads to more efficient and elegant solutions. Practice this method regularly so it becomes second nature, allowing you to apply it quickly even under pressure.
3. Balancing Depth with Practicality
Challenge: It’s possible to get caught up in breaking down problems to such a fundamental level that you lose sight of practical implementation.
Solution: Set clear boundaries for your analysis. Focus on breaking down the problem to a level that provides new insights without getting lost in unnecessary details.
4. Integrating with Existing Systems
Challenge: When working on large, established codebases, it can be difficult to apply first principles thinking without disrupting existing architecture.
Solution: Use first principles thinking to understand the core problems the existing system is solving. Then, look for opportunities to apply this understanding to improve or extend the system within its current constraints.
First Principles Thinking in Different Programming Paradigms
The beauty of first principles thinking is that it can be applied across various programming paradigms. Let’s explore how this approach can be used in different contexts:
Object-Oriented Programming (OOP)
In OOP, first principles thinking can guide you in designing classes and their relationships. Consider a task to model a library system:
- Identify the core entities: Books, Users, Loans
- Define their essential attributes and behaviors
- Establish the relationships between these entities
This might lead to a basic implementation like:
class Book:
def __init__(self, title, author, isbn):
self.title = title
self.author = author
self.isbn = isbn
self.is_available = True
class User:
def __init__(self, name, user_id):
self.name = name
self.user_id = user_id
self.borrowed_books = []
class Library:
def __init__(self):
self.books = []
self.users = []
def add_book(self, book):
self.books.append(book)
def register_user(self, user):
self.users.append(user)
def lend_book(self, user, book):
if book.is_available and book in self.books and user in self.users:
book.is_available = False
user.borrowed_books.append(book)
return True
return False
def return_book(self, user, book):
if book in user.borrowed_books:
book.is_available = True
user.borrowed_books.remove(book)
return True
return False
Functional Programming
In functional programming, first principles thinking can help in breaking down operations into pure functions. Let’s consider a task to process a list of numbers:
- Identify the core operations: Filtering, mapping, reducing
- Define these operations as pure functions
- Compose these functions to achieve the desired result
This might result in code like:
def is_even(n):
return n % 2 == 0
def square(n):
return n ** 2
def sum_list(numbers):
return sum(numbers)
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
result = sum_list(map(square, filter(is_even, numbers)))
print(result) # Sum of squares of even numbers
First Principles Thinking in Software Architecture
When designing software architecture, first principles thinking can guide you to create robust and scalable systems. Let’s apply this to designing a basic web application architecture:
- Identify core components: Client, Server, Database
- Define essential functionalities: User Interface, Business Logic, Data Storage
- Establish communication patterns: HTTP requests, Database queries
This might lead to a basic three-tier architecture:
from flask import Flask, request, jsonify
import sqlite3
app = Flask(__name__)
# Database layer
def get_db():
db = sqlite3.connect('example.db')
db.row_factory = sqlite3.Row
return db
# Business logic layer
def get_user(user_id):
db = get_db()
user = db.execute('SELECT * FROM users WHERE id = ?', (user_id,)).fetchone()
db.close()
return dict(user) if user else None
def create_user(name, email):
db = get_db()
cursor = db.cursor()
cursor.execute('INSERT INTO users (name, email) VALUES (?, ?)', (name, email))
db.commit()
user_id = cursor.lastrowid
db.close()
return user_id
# Presentation layer (API)
@app.route('/user/<int:user_id>', methods=['GET'])
def api_get_user(user_id):
user = get_user(user_id)
if user:
return jsonify(user)
return jsonify({'error': 'User not found'}), 404
@app.route('/user', methods=['POST'])
def api_create_user():
data = request.json
user_id = create_user(data['name'], data['email'])
return jsonify({'id': user_id}), 201
if __name__ == '__main__':
app.run(debug=True)
This basic structure separates concerns and provides a foundation that can be expanded upon as the application grows.
Cultivating First Principles Thinking in Your Coding Practice
Developing a habit of first principles thinking takes time and practice. Here are some strategies to incorporate this approach into your daily coding routine:
1. Question Everything
Whenever you encounter a new problem or are about to implement a solution, pause and ask yourself: “Why am I doing it this way?” Challenge your assumptions and try to identify the core problem you’re trying to solve.
2. Practice Decomposition
Regularly practice breaking down complex problems into their constituent parts. This skill is at the heart of first principles thinking and becomes more natural with practice.
3. Embrace the Beginner’s Mindset
Try to approach each problem as if you’re seeing it for the first time. This can help you avoid falling into habitual thinking patterns and open up new possibilities.
4. Experiment with Different Solutions
Once you’ve broken a problem down to its first principles, challenge yourself to come up with multiple solutions. This exercise can help you see the problem from different angles and potentially discover more efficient or elegant approaches.
5. Learn Fundamental Concepts Deeply
Invest time in deeply understanding fundamental programming concepts. The better you understand these building blocks, the more effectively you can apply first principles thinking to complex problems.
6. Reflect on Your Process
After solving a problem, take time to reflect on your approach. Could you have broken it down further? Were there assumptions you could have challenged? Use these reflections to refine your first principles thinking in future problems.
Conclusion: The Transformative Power of First Principles Thinking in Coding
First principles thinking is more than just a problem-solving technique; it’s a mindset that can transform your approach to coding and software development. By breaking problems down to their fundamental truths and building solutions from the ground up, you can:
- Develop more innovative and efficient solutions
- Gain a deeper understanding of complex systems
- Adapt more easily to new technologies and paradigms
- Approach unfamiliar problems with confidence
- Continuously improve your coding skills and knowledge
Whether you’re a beginner learning the basics of programming or an experienced developer preparing for technical interviews at top tech companies, cultivating first principles thinking can give you a significant edge. It aligns perfectly with the goals of platforms like AlgoCademy, which emphasize algorithmic thinking and problem-solving skills.
Remember, the journey to mastering first principles thinking is ongoing. Each problem you encounter is an opportunity to practice and refine this skill. Embrace the challenge, question your assumptions, and don’t be afraid to start from scratch. With time and practice, you’ll find yourself approaching coding challenges with newfound creativity and insight, able to solve any problem from first principles.
As you continue your coding journey, whether you’re working through interactive tutorials, preparing for technical interviews, or building complex systems, let first principles thinking be your guide. It’s not just about solving the problem at hand, but about developing a mindset that will serve you throughout your programming career.