The Power of Constraints: How Limiting Your Options Can Help You Solve Problems Faster
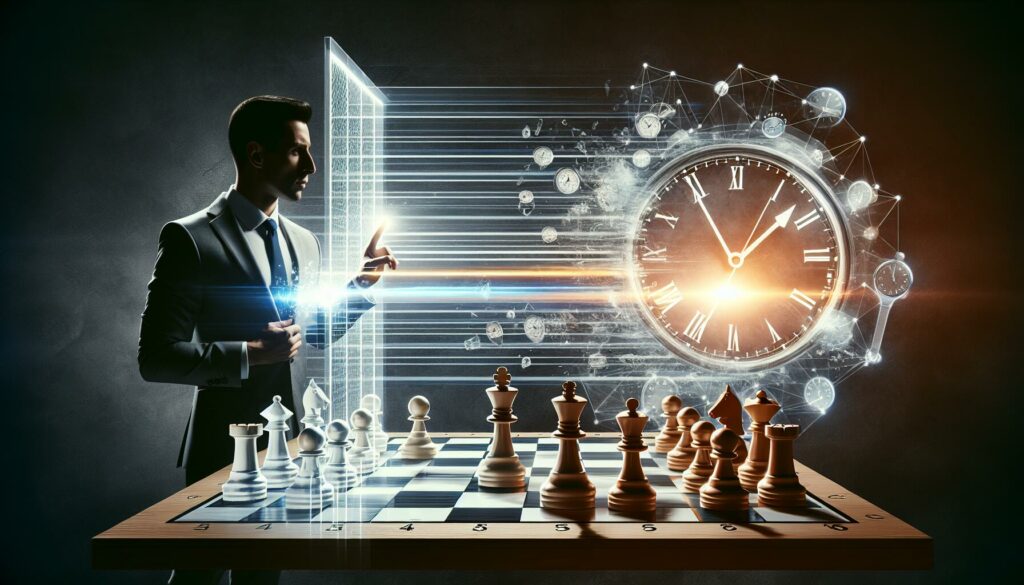
In the world of programming and problem-solving, we often think that having more options and resources at our disposal leads to better solutions. However, counterintuitively, imposing constraints on ourselves can actually enhance our problem-solving abilities and lead to more efficient, creative, and elegant solutions. This concept, known as “the power of constraints,” is a valuable tool in the arsenal of any programmer or problem solver.
In this article, we’ll explore how setting constraints, such as time or memory limits, can simplify problem-solving by eliminating unnecessary complexities. We’ll also delve into examples where constraints guide you toward the optimal solution faster, and discuss how this principle applies to coding education and skills development.
Understanding the Power of Constraints
The power of constraints is rooted in the idea that limitations can foster creativity and efficiency. When we’re faced with boundaries, our minds are forced to think more critically and innovatively to find solutions within those limits. This concept is applicable across various fields, from art and design to engineering and computer science.
In programming, constraints can take many forms:
- Time complexity limits
- Memory usage restrictions
- Input/output constraints
- Programming language or paradigm restrictions
- Coding style guidelines
By imposing these constraints, we challenge ourselves to find more efficient and elegant solutions, often leading to improved algorithms and code quality.
How Constraints Simplify Problem-Solving
Constraints help simplify problem-solving in several ways:
1. Narrowing the Solution Space
When faced with a problem, the number of potential solutions can be overwhelming. Constraints help narrow down the solution space, making it easier to focus on viable options. For example, if you’re given a time complexity constraint of O(n log n) for a sorting algorithm, you can immediately rule out slower algorithms like bubble sort or insertion sort, and focus on more efficient options like merge sort or quicksort.
2. Encouraging Creativity
Constraints force us to think outside the box and come up with creative solutions. When we can’t rely on brute-force approaches or excessive resources, we’re compelled to find more innovative ways to solve problems.
3. Improving Efficiency
By setting limits on time or memory usage, we’re pushed to develop more efficient algorithms and data structures. This not only solves the immediate problem but also improves our overall coding skills and understanding of algorithmic efficiency.
4. Enhancing Focus
Constraints help us focus on the core problem by eliminating distractions and unnecessary complexities. When we have limited resources, we’re forced to prioritize what’s truly important in solving the problem at hand.
Examples of Constraints in Action
Let’s explore some examples where constraints can guide us toward optimal solutions faster:
Example 1: Two Sum Problem with Memory Constraint
Consider the classic “Two Sum” problem: given an array of integers and a target sum, find two numbers in the array that add up to the target.
Without constraints, a simple brute-force solution might look like this:
def two_sum(nums, target):
for i in range(len(nums)):
for j in range(i + 1, len(nums)):
if nums[i] + nums[j] == target:
return [i, j]
return []
This solution has a time complexity of O(n^2) and doesn’t use any extra space. However, if we impose a time complexity constraint of O(n) and allow for additional memory usage, we can come up with a more efficient solution:
def two_sum(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return []
By allowing extra memory usage (the dictionary), we’ve significantly improved the time complexity from O(n^2) to O(n). This constraint-driven approach leads us to a more optimal solution.
Example 2: String Compression with Space Constraint
Let’s consider a problem where we need to compress a string by replacing consecutive repeated characters with the character followed by the count of repetitions. However, we have a space constraint that requires us to do this in-place, without using extra space.
A naive approach might create a new string, which violates our space constraint:
def compress_string(s):
result = []
count = 1
for i in range(1, len(s)):
if s[i] == s[i-1]:
count += 1
else:
result.append(s[i-1] + str(count))
count = 1
result.append(s[-1] + str(count))
return ''.join(result)
To adhere to the space constraint, we need to think more creatively. Here’s an in-place solution:
def compress_string(chars):
write = 0
anchor = 0
for read in range(len(chars)):
if read + 1 == len(chars) or chars[read + 1] != chars[read]:
chars[write] = chars[anchor]
write += 1
if read > anchor:
for digit in str(read - anchor + 1):
chars[write] = digit
write += 1
anchor = read + 1
return write
This solution compresses the string in-place, satisfying our space constraint while still efficiently solving the problem.
Example 3: Fibonacci with Time and Space Constraints
The Fibonacci sequence is a classic problem in computer science. Let’s say we need to find the nth Fibonacci number, but we have both time and space constraints: we need to do it in O(n) time and O(1) space.
A simple recursive solution would be:
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n-1) + fibonacci(n-2)
However, this solution has a time complexity of O(2^n) and doesn’t meet our constraints. A dynamic programming approach with memoization would solve the time complexity issue but would still use O(n) space.
To meet both our time and space constraints, we can use an iterative solution:
def fibonacci(n):
if n <= 1:
return n
a, b = 0, 1
for _ in range(2, n + 1):
a, b = b, a + b
return b
This solution has a time complexity of O(n) and uses only O(1) extra space, meeting both our constraints and leading us to the optimal solution.
Applying Constraints in Coding Education
The concept of constraints is particularly valuable in coding education and skills development. Platforms like AlgoCademy, which focus on interactive coding tutorials and preparing learners for technical interviews, can leverage the power of constraints to enhance learning experiences:
1. Gradual Introduction of Constraints
In a learning environment, constraints can be introduced gradually. For beginners, the focus might be on solving problems correctly without any time or space constraints. As learners progress, time and space complexity constraints can be introduced, challenging them to optimize their solutions.
2. Language-Specific Constraints
To help learners master specific programming languages, exercises can impose constraints that force the use of language-specific features. For example, in Python, a constraint might require the use of list comprehensions or generator expressions to solve a problem efficiently.
3. Interview Preparation
For those preparing for technical interviews, especially for major tech companies (often referred to as FAANG), practicing with constraints is crucial. These companies often present problems with specific time and space complexity requirements, mirroring real-world scenarios where efficient resource usage is critical.
4. Algorithmic Thinking Development
Constraints encourage algorithmic thinking by pushing learners to consider the efficiency of their solutions. This helps in developing a deeper understanding of data structures and algorithms, which is essential for solving complex problems.
5. Real-World Problem Simulation
In real-world software development, constraints are omnipresent. By incorporating constraints into coding exercises, educational platforms can better prepare learners for the challenges they’ll face in their careers.
Strategies for Leveraging Constraints in Problem-Solving
To effectively use constraints in problem-solving, consider the following strategies:
1. Analyze the Problem and Constraints
Before diving into coding, thoroughly analyze the problem and the given constraints. Understanding the limitations will help you approach the problem more effectively.
2. Start with a Naive Solution
Begin by implementing a simple solution without worrying about constraints. This gives you a baseline to work from and helps you understand the problem better.
3. Iteratively Optimize
Once you have a working solution, iteratively optimize it to meet the constraints. This might involve choosing different data structures, altering your algorithm, or refactoring your code.
4. Consider Trade-offs
Often, optimizing for one constraint (e.g., time complexity) might come at the cost of another (e.g., space complexity). Consider these trade-offs and choose the best balance for your specific problem.
5. Learn from Existing Algorithms
Study well-known algorithms and data structures. Understanding how these solutions work within certain constraints can inspire your own problem-solving approaches.
6. Practice Regularly
The more you practice solving problems with constraints, the better you’ll become at recognizing patterns and applying efficient solutions.
The Role of AI in Constraint-Based Problem Solving
Artificial Intelligence can play a significant role in helping learners navigate constraint-based problem-solving:
1. Personalized Constraint Introduction
AI can analyze a learner’s skill level and progressively introduce constraints that challenge them appropriately, ensuring a personalized learning experience.
2. Solution Analysis
AI-powered tools can analyze submitted solutions, providing feedback on whether they meet the given constraints and suggesting optimizations if they don’t.
3. Hint Generation
When learners struggle with a constrained problem, AI can generate hints that guide them towards efficient solutions without giving away the answer entirely.
4. Pattern Recognition
AI can help identify patterns in how different constraints affect solution approaches, helping learners develop a more intuitive understanding of algorithmic efficiency.
Conclusion
The power of constraints in problem-solving and coding education cannot be overstated. By limiting our options, we often find ourselves on a clearer path to optimal solutions. Constraints push us to think more creatively, work more efficiently, and develop a deeper understanding of algorithmic principles.
For platforms like AlgoCademy and other coding education resources, incorporating constraint-based learning can significantly enhance the learning experience. It prepares learners not just to solve problems, but to solve them efficiently and elegantly – a skill that’s invaluable in both technical interviews and real-world software development.
As you continue your journey in programming and problem-solving, embrace constraints as tools for growth rather than obstacles. Challenge yourself with time and space complexity limits, explore language-specific constraints, and continually push the boundaries of your problem-solving abilities. Remember, it’s often within the confines of constraints that true innovation and mastery emerge.
By harnessing the power of constraints, you’ll not only become a more efficient problem solver but also develop the kind of algorithmic thinking and coding skills that are highly valued in the tech industry. So the next time you face a coding challenge, don’t just ask yourself how to solve it – ask yourself how to solve it within specific constraints. You might be surprised at the creative and efficient solutions you discover.