The Power of Clean Code: Best Practices for Effective Programming
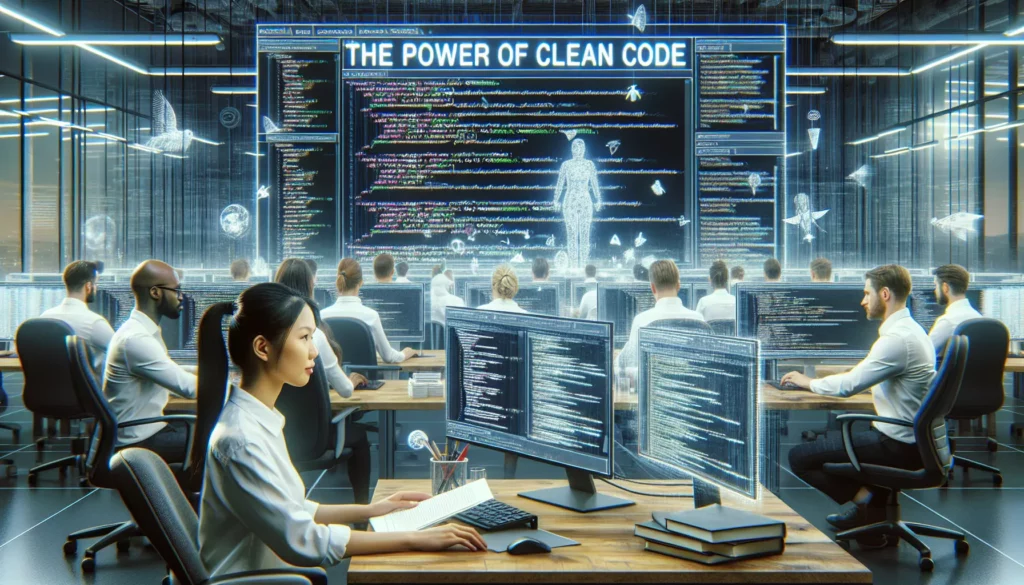
In the world of software development, writing code that works is just the beginning. To truly excel as a programmer and create maintainable, efficient, and collaborative projects, you need to master the art of writing clean code. Clean code is not just about aesthetics; it’s about creating software that is easy to understand, modify, and scale. In this comprehensive guide, we’ll explore the power of clean code and delve into best practices that will elevate your programming skills to new heights.
What is Clean Code?
Clean code refers to code that is easy to read, understand, and maintain. It follows a set of principles and best practices that make it more efficient, less prone to bugs, and simpler to modify or extend. Robert C. Martin, also known as Uncle Bob, popularized the concept in his book “Clean Code: A Handbook of Agile Software Craftsmanship.” According to Martin, clean code is code that is focused, simple, and direct.
The benefits of writing clean code include:
- Improved readability and understandability
- Easier maintenance and debugging
- Enhanced collaboration among team members
- Reduced technical debt
- Increased productivity in the long run
- Better scalability of projects
Best Practices for Writing Clean Code
1. Use Meaningful and Pronounceable Variable Names
One of the fundamental aspects of clean code is using clear and descriptive variable names. Avoid single-letter variables or cryptic abbreviations. Instead, opt for names that explain the purpose or content of the variable.
Bad example:
int d; // elapsed time in days
Good example:
int elapsedTimeInDays;
By using descriptive names, you make your code self-documenting and easier for others (including your future self) to understand without the need for extensive comments.
2. Write Short, Focused Functions
Functions should be small and focused on doing one thing well. This principle, known as the Single Responsibility Principle (SRP), is crucial for creating maintainable code. Aim to keep functions under 20-30 lines of code.
Bad example:
function processUserData(user) {
// Validate user input
if (!user.name || !user.email) {
throw new Error('Invalid user data');
}
// Save user to database
database.save(user);
// Send welcome email
emailService.sendWelcomeEmail(user.email);
// Log user activity
logger.log('New user registered:', user.name);
}
Good example:
function processUserData(user) {
validateUserData(user);
saveUserToDatabase(user);
sendWelcomeEmail(user);
logUserActivity(user);
}
function validateUserData(user) {
if (!user.name || !user.email) {
throw new Error('Invalid user data');
}
}
function saveUserToDatabase(user) {
database.save(user);
}
function sendWelcomeEmail(user) {
emailService.sendWelcomeEmail(user.email);
}
function logUserActivity(user) {
logger.log('New user registered:', user.name);
}
By breaking down the function into smaller, focused functions, the code becomes more modular and easier to test and maintain.
3. Use Consistent Formatting and Indentation
Consistent formatting and proper indentation are crucial for code readability. Many programming languages have style guides that you can follow. For example, in Python, the PEP 8 style guide is widely used. Consistent formatting makes it easier for developers to scan and understand the code structure quickly.
Bad example:
function calculateTotal(items){
for(let i=0;i<items.length;i++){
total+=items[i].price;
}
return total;
}
Good example:
function calculateTotal(items) {
let total = 0;
for (let i = 0; i < items.length; i++) {
total += items[i].price;
}
return total;
}
4. Write Self-Documenting Code
While comments can be helpful, it’s even better to write code that is self-explanatory. Use clear function and variable names, and structure your code in a way that its purpose is evident without the need for extensive comments.
Bad example:
// Check if n is even
function f(n) {
return n % 2 == 0;
}
Good example:
function isEven(number) {
return number % 2 === 0;
}
5. Follow the DRY Principle (Don’t Repeat Yourself)
Avoid duplicating code by extracting common functionality into reusable functions or classes. This principle, known as DRY (Don’t Repeat Yourself), helps reduce redundancy and makes your codebase easier to maintain.
Bad example:
function calculateAreaOfSquare(side) {
return side * side;
}
function calculateAreaOfRectangle(length, width) {
return length * width;
}
function calculateAreaOfCircle(radius) {
return Math.PI * radius * radius;
}
Good example:
function calculateArea(shape, ...dimensions) {
switch(shape) {
case 'square':
return dimensions[0] * dimensions[0];
case 'rectangle':
return dimensions[0] * dimensions[1];
case 'circle':
return Math.PI * dimensions[0] * dimensions[0];
default:
throw new Error('Unknown shape');
}
}
6. Use Meaningful Constants
Instead of using magic numbers or strings directly in your code, define constants with descriptive names. This makes your code more readable and easier to maintain.
Bad example:
if (user.age > 18) {
// Allow access
}
Good example:
const MINIMUM_AGE_FOR_ACCESS = 18;
if (user.age > MINIMUM_AGE_FOR_ACCESS) {
// Allow access
}
7. Handle Errors Gracefully
Proper error handling is crucial for writing robust and reliable code. Use try-catch blocks to handle exceptions, and provide meaningful error messages to help with debugging.
Bad example:
function divideNumbers(a, b) {
return a / b;
}
Good example:
function divideNumbers(a, b) {
if (b === 0) {
throw new Error('Division by zero is not allowed');
}
return a / b;
}
8. Write Clean and Concise Comments
While self-documenting code is ideal, there are times when comments are necessary. When you do use comments, make sure they are clear, concise, and add value. Avoid obvious comments that simply restate what the code is doing.
Bad example:
// This function adds two numbers
function add(a, b) {
return a + b;
}
Good example:
/**
* Calculates the sum of two numbers.
* @param {number} a - The first number.
* @param {number} b - The second number.
* @returns {number} The sum of a and b.
*/
function add(a, b) {
return a + b;
}
9. Use Consistent Naming Conventions
Adopt and stick to a consistent naming convention throughout your project. This includes conventions for variables, functions, classes, and file names. Common conventions include camelCase for variables and functions, PascalCase for classes, and kebab-case for file names.
Bad example (mixing conventions):
function Calculate_area(radius) {
return Math.PI * radius * radius;
}
const User_age = 25;
class userProfile {
// ...
}
Good example (consistent conventions):
function calculateArea(radius) {
return Math.PI * radius * radius;
}
const userAge = 25;
class UserProfile {
// ...
}
10. Avoid Deep Nesting
Deeply nested code is hard to read and understand. Try to keep your code’s nesting level to a minimum. Use early returns, guard clauses, or extract complex conditions into separate functions to reduce nesting.
Bad example:
function processOrder(order) {
if (order.isValid) {
if (order.hasStock) {
if (order.paymentAuthorized) {
// Process the order
} else {
throw new Error('Payment not authorized');
}
} else {
throw new Error('Out of stock');
}
} else {
throw new Error('Invalid order');
}
}
Good example:
function processOrder(order) {
if (!order.isValid) {
throw new Error('Invalid order');
}
if (!order.hasStock) {
throw new Error('Out of stock');
}
if (!order.paymentAuthorized) {
throw new Error('Payment not authorized');
}
// Process the order
}
Tools and Techniques for Maintaining Clean Code
To help you maintain clean code, consider using the following tools and techniques:
1. Code Linters
Linters are tools that analyze your code for potential errors, style violations, and suspicious constructs. They help enforce coding standards and catch common mistakes. Popular linters include:
- ESLint for JavaScript
- Pylint for Python
- RuboCop for Ruby
- Checkstyle for Java
2. Code Formatters
Code formatters automatically format your code according to predefined rules, ensuring consistent style across your project. Some popular formatters include:
- Prettier for JavaScript, CSS, and more
- Black for Python
- gofmt for Go
- Clang-Format for C/C++
3. Version Control Systems
Using version control systems like Git allows you to track changes, collaborate with others, and maintain a clean and organized codebase. It also enables you to revert changes if needed and manage different versions of your code.
4. Code Reviews
Regular code reviews are an excellent way to maintain code quality and share knowledge within a team. They help catch issues early, ensure adherence to coding standards, and provide opportunities for learning and improvement.
5. Continuous Integration (CI)
Implementing CI practices helps maintain code quality by automatically running tests, linters, and other checks whenever changes are pushed to the repository. This ensures that code issues are caught early in the development process.
6. Refactoring
Regularly refactoring your code helps maintain its cleanliness and improve its structure over time. Set aside time for refactoring sessions to address technical debt and improve code quality.
The Impact of Clean Code on Software Development
Writing clean code has a significant impact on various aspects of software development:
1. Maintainability
Clean code is easier to maintain and update. When you or other developers need to make changes or fix bugs, clean code allows for quicker understanding and modification of the existing codebase.
2. Scalability
As projects grow in size and complexity, clean code becomes even more crucial. Well-structured, modular code is easier to scale and extend with new features or functionality.
3. Collaboration
Clean code facilitates better collaboration among team members. When code is easy to read and understand, it’s easier for developers to work together, review each other’s work, and contribute to shared projects.
4. Reduced Bugs
Clean code is less prone to bugs and errors. By following best practices and writing clear, focused code, you reduce the chances of introducing unintended behavior or side effects.
5. Improved Performance
While clean code doesn’t automatically lead to better performance, it often results in more efficient algorithms and data structures. Clean code is easier to optimize and refactor for performance improvements when needed.
6. Faster Onboarding
For new team members or developers taking over a project, clean code significantly reduces the time needed to understand and start contributing to the codebase.
Conclusion
The power of clean code lies in its ability to transform software development from a solitary act of writing functional code into a collaborative, efficient, and sustainable process. By following best practices for clean code, you not only improve the quality of your work but also contribute to a more productive and enjoyable development experience for yourself and your team.
Remember that writing clean code is a skill that develops over time. It requires practice, attention to detail, and a commitment to continuous improvement. As you apply these principles in your daily coding activities, you’ll find that your code becomes more readable, maintainable, and robust.
Embrace the power of clean code, and watch as it elevates your programming skills, enhances your projects, and sets you apart as a professional developer. Clean code is not just a set of rules; it’s a mindset that will serve you well throughout your programming career.