The Planning Phase: Why Proper Planning Is Key to Successfully Coding a Project
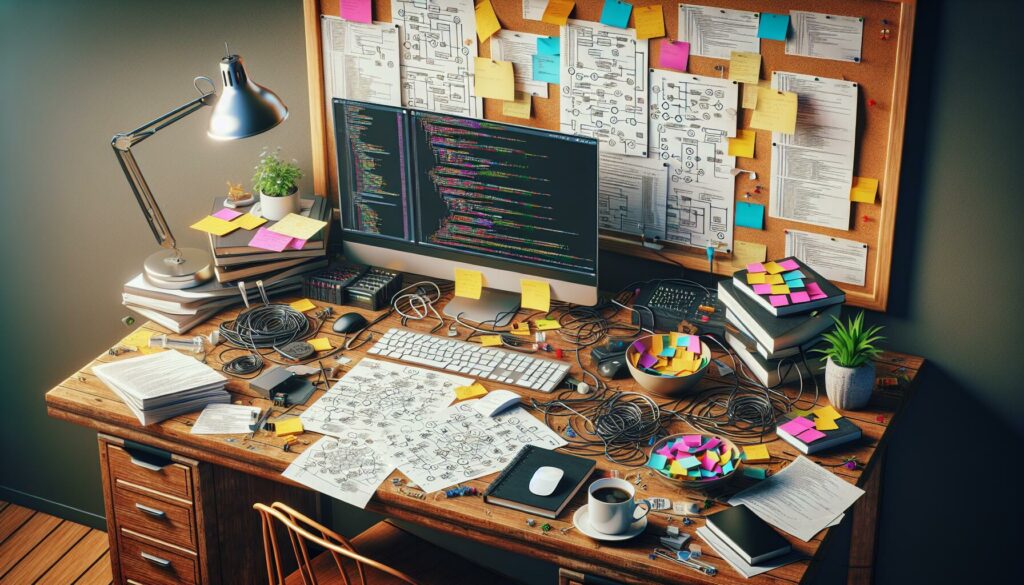
In the world of software development, the adage “measure twice, cut once” couldn’t be more applicable. The planning phase of a coding project is often overlooked or rushed, with eager developers jumping straight into writing code. However, this approach can lead to costly mistakes, wasted time, and subpar results. In this comprehensive guide, we’ll explore why proper planning is crucial for successfully coding a project and provide you with a template to ensure your next endeavor is set up for success from the start.
The Importance of Thorough Planning
Before we dive into the specifics of how to plan a coding project, let’s first understand why planning is so critical:
1. Clarity of Vision
A well-planned project provides a clear vision of what you’re trying to achieve. It helps you define the scope, objectives, and deliverables of your project, ensuring that everyone involved has a shared understanding of the end goal.
2. Resource Optimization
Proper planning allows you to allocate resources effectively. This includes time, manpower, and budget. By identifying potential challenges and requirements upfront, you can avoid overcommitting or underestimating the resources needed.
3. Risk Mitigation
Through thorough planning, you can identify potential risks and develop strategies to mitigate them. This proactive approach can save you from costly setbacks later in the development process.
4. Improved Collaboration
A well-documented plan serves as a reference point for all team members. It facilitates better communication and collaboration, as everyone can refer to the same set of guidelines and expectations.
5. Scalability and Maintainability
Planning ahead allows you to design your project with scalability and maintainability in mind. This foresight can save you from major refactoring efforts down the line as your project grows or evolves.
6. Cost-Effectiveness
While it might seem counterintuitive to spend time on planning rather than coding, a well-planned project often results in fewer errors, less rework, and a more efficient development process, ultimately saving time and money.
Key Components of Project Planning
Now that we understand the importance of planning, let’s break down the key components that should be included in your project planning phase:
1. Project Scope and Objectives
Clearly define what your project aims to achieve. This includes:
- Project goals and objectives
- Key features and functionalities
- Target audience or users
- Project constraints (time, budget, resources)
2. User Stories and Requirements
Break down your project into user stories or specific requirements. This helps in understanding the project from the user’s perspective and ensures that all necessary features are accounted for.
3. Architecture Design
Outline the high-level structure of your project. This includes:
- System architecture
- Technology stack selection
- Integration points with external systems
- Scalability considerations
4. Database Schema
If your project involves data storage, design your database schema. Consider:
- Entity-Relationship Diagrams (ERD)
- Data models
- Database type (relational, NoSQL, etc.)
5. API Design
If your project includes APIs, plan them out:
- Endpoint definitions
- Request/response formats
- Authentication and authorization mechanisms
6. User Interface (UI) Design
Create mockups or wireframes of your user interface. This helps in visualizing the end product and can reveal potential usability issues early on.
7. Project Timeline and Milestones
Break your project into phases or sprints, setting realistic timelines and milestones for each.
8. Risk Assessment
Identify potential risks and develop mitigation strategies for each.
A Template for Project Planning
To help you get started with your project planning, here’s a template you can follow:
1. Project Overview
<!-- Project Name -->
Project Name: [Your Project Name]
<!-- Project Description -->
Description: [A brief overview of what your project aims to achieve]
<!-- Project Objectives -->
Objectives:
1. [Objective 1]
2. [Objective 2]
3. [Objective 3]
<!-- Target Audience -->
Target Audience: [Describe your intended users]
<!-- Project Constraints -->
Constraints:
- Time: [e.g., 3 months]
- Budget: [e.g., $50,000]
- Resources: [e.g., 2 developers, 1 designer]
2. User Stories
User Story 1:
As a [type of user], I want [an action] so that [a benefit/a value].
User Story 2:
As a [type of user], I want [an action] so that [a benefit/a value].
[Continue with more user stories as needed]
3. Feature Set
Core Features:
1. [Feature 1]
2. [Feature 2]
3. [Feature 3]
Nice-to-Have Features:
1. [Feature 1]
2. [Feature 2]
3. [Feature 3]
4. System Architecture
Architecture Overview:
[Describe your high-level system architecture]
Technology Stack:
- Frontend: [e.g., React.js]
- Backend: [e.g., Node.js with Express]
- Database: [e.g., MongoDB]
- Other Technologies: [e.g., Redis for caching]
External Integrations:
1. [Integration 1]
2. [Integration 2]
Scalability Considerations:
[Describe how your architecture will handle growth]
5. Database Schema
Database Type: [e.g., Relational - PostgreSQL]
Entities:
1. User
- id (Primary Key)
- username
- email
- password_hash
- created_at
- updated_at
2. Product
- id (Primary Key)
- name
- description
- price
- category_id (Foreign Key)
- created_at
- updated_at
[Continue with more entities as needed]
Relationships:
- User to Product: One-to-Many
- Product to Category: Many-to-One
[Include an Entity-Relationship Diagram if possible]
6. API Design
Base URL: https://api.yourproject.com/v1
Endpoints:
1. User Registration
POST /users
Request Body: {
"username": "string",
"email": "string",
"password": "string"
}
Response: {
"id": "string",
"username": "string",
"email": "string",
"created_at": "timestamp"
}
2. Get Product
GET /products/{id}
Response: {
"id": "string",
"name": "string",
"description": "string",
"price": "number",
"category": {
"id": "string",
"name": "string"
}
}
[Continue with more endpoint definitions]
Authentication:
- Method: JWT (JSON Web Tokens)
- Token Lifespan: 24 hours
7. UI Design
For this section, include links to your wireframes or mockups. You can use tools like Figma, Sketch, or even hand-drawn sketches to visualize your user interface.
Wireframes:
- Homepage: [Link to wireframe]
- User Profile: [Link to wireframe]
- Product Listing: [Link to wireframe]
Color Scheme:
- Primary: #3498db
- Secondary: #2ecc71
- Accent: #e74c3c
Typography:
- Headings: Roboto, sans-serif
- Body: Open Sans, sans-serif
8. Project Timeline
Phase 1: Planning and Design (2 weeks)
- Week 1: Project scoping, user stories, and feature set definition
- Week 2: Architecture design, database schema, and API design
Phase 2: Development (8 weeks)
- Weeks 3-4: Set up development environment and implement core backend functionality
- Weeks 5-6: Develop frontend components and integrate with backend
- Weeks 7-8: Implement authentication and authorization
- Weeks 9-10: Develop additional features and refine UI/UX
Phase 3: Testing and Refinement (2 weeks)
- Week 11: Conduct thorough testing (unit tests, integration tests, user acceptance testing)
- Week 12: Bug fixes and performance optimization
Phase 4: Deployment and Launch (1 week)
- Week 13: Prepare production environment and deploy application
- Launch and post-launch monitoring
9. Risk Assessment
1. Risk: Scope creep
Mitigation: Clearly define project scope in the planning phase and implement a change control process.
2. Risk: Technical challenges with chosen technology stack
Mitigation: Conduct a proof of concept for critical components early in the development phase.
3. Risk: Data security vulnerabilities
Mitigation: Implement security best practices, conduct regular security audits, and use encryption for sensitive data.
4. Risk: Missed deadlines
Mitigation: Build in buffer time for each phase and have weekly progress check-ins.
[Continue with more risks and mitigation strategies as needed]
Implementing Your Plan
Once you have your project plan in place, it’s time to put it into action. Here are some tips for successfully implementing your plan:
1. Use Project Management Tools
Utilize tools like Jira, Trello, or Asana to track tasks, assign responsibilities, and monitor progress. These tools can help you visualize your project timeline and ensure everyone is on the same page.
2. Regular Check-ins
Schedule regular team meetings to discuss progress, challenges, and any necessary adjustments to the plan. This helps maintain alignment and allows for quick problem-solving.
3. Version Control
Use a version control system like Git to manage your codebase. This allows for better collaboration, easier tracking of changes, and the ability to revert if necessary.
4. Documentation
Maintain clear documentation throughout the development process. This includes code comments, API documentation, and user guides. Good documentation makes it easier for team members to understand and work with the codebase.
5. Continuous Integration and Deployment (CI/CD)
Implement CI/CD practices to automate testing and deployment processes. This helps catch issues early and ensures a smooth transition from development to production.
6. Flexibility
While it’s important to stick to your plan, be prepared to adapt if necessary. Unexpected challenges may arise, and being flexible allows you to adjust your approach without derailing the entire project.
The Role of Planning in Coding Education
As we consider the importance of planning in the context of AlgoCademy’s focus on coding education and programming skills development, it’s crucial to emphasize how proper planning fits into the broader picture of becoming a proficient developer.
1. Developing a Problem-Solving Mindset
The planning phase of a project is essentially an exercise in problem-solving. It requires you to break down a large, complex task into smaller, manageable pieces. This skill is invaluable not just in project planning, but in tackling coding challenges and technical interviews as well.
2. Understanding the Big Picture
While AlgoCademy focuses on helping learners master specific coding concepts and algorithms, the planning phase teaches developers to think about how these individual components fit into a larger system. This holistic understanding is crucial for progressing from writing isolated code snippets to building complete, functional applications.
3. Preparing for Technical Interviews
Many technical interviews, especially for positions at major tech companies, include system design questions. These questions assess a candidate’s ability to plan and architect large-scale systems. By practicing project planning, you’re developing skills that directly translate to success in these high-stakes interview scenarios.
4. Enhancing Communication Skills
Creating a project plan requires clear communication of ideas, both in writing and visually through diagrams and mockups. These communication skills are essential for working effectively in development teams and explaining complex technical concepts to non-technical stakeholders.
5. Cultivating Best Practices
The planning phase introduces developers to industry best practices such as writing user stories, designing scalable architectures, and conducting risk assessments. Familiarity with these practices sets a strong foundation for professional development work.
Conclusion
The planning phase is a critical component of any successful coding project. It provides clarity, mitigates risks, and sets the foundation for efficient development. By following the template and guidelines provided in this article, you’ll be well-equipped to plan your next project thoroughly.
Remember, the time invested in planning pays dividends throughout the development process and beyond. It not only leads to better-executed projects but also contributes to your growth as a developer, enhancing your problem-solving skills, system design abilities, and overall coding proficiency.
As you continue your journey in coding education and skills development, whether through platforms like AlgoCademy or personal projects, make project planning an integral part of your process. It’s a skill that will serve you well in your studies, in technical interviews, and throughout your career as a software developer.
Happy planning, and may your next coding project be a resounding success!