The Parallels Between Cooking and Coding: Recipes for Successful Algorithms
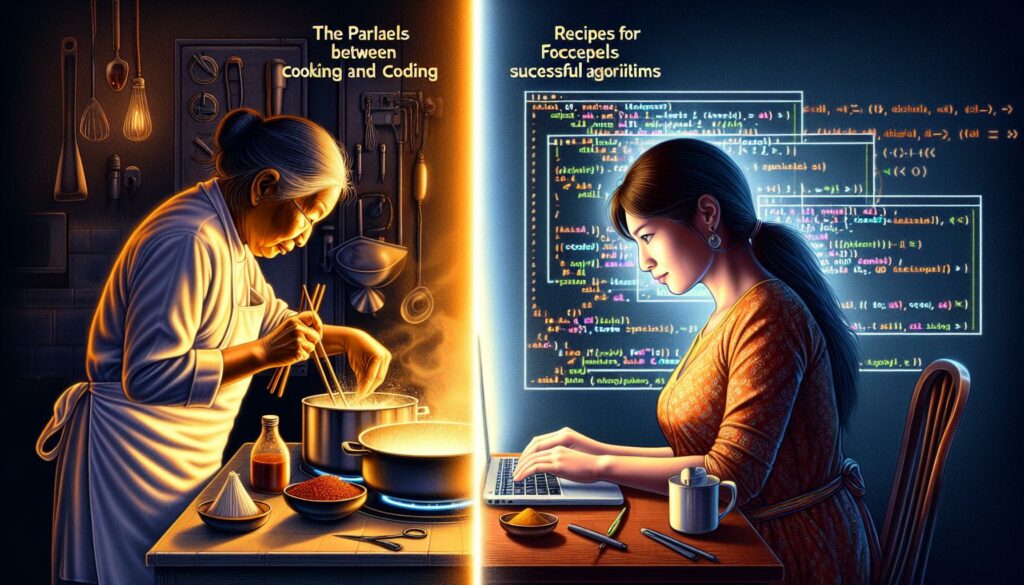
At first glance, the worlds of cooking and coding might seem worlds apart. One involves pots, pans, and a dash of creativity in the kitchen, while the other requires computers, keyboards, and logical thinking. However, upon closer inspection, these two disciplines share striking similarities. Both cooking and coding are forms of creation, following step-by-step processes to produce a desired outcome. In this article, we’ll explore the fascinating parallels between culinary arts and algorithm design, uncovering how the principles of cooking can inform and enhance our approach to coding.
1. Recipes and Algorithms: The Blueprint for Success
Just as a chef relies on a recipe to create a delicious dish, a programmer depends on algorithms to solve complex problems. Both recipes and algorithms serve as blueprints, providing a structured approach to achieving a specific goal.
The Recipe as an Algorithm
Consider a recipe for baking chocolate chip cookies:
1. Preheat oven to 375°F (190°C)
2. Cream together butter, sugar, and brown sugar
3. Beat in eggs and vanilla
4. Mix in flour, baking soda, and salt
5. Stir in chocolate chips
6. Drop spoonfuls of dough onto baking sheets
7. Bake for 9-11 minutes
8. Cool on wire racks
This recipe is essentially an algorithm for creating cookies. It provides a series of steps, each with a specific purpose, that when followed correctly, result in a batch of delicious cookies.
The Algorithm as a Recipe
Now, let’s look at a simple sorting algorithm, like Bubble Sort:
function bubbleSort(arr) {
let n = arr.length;
for (let i = 0; i < n-1; i++) {
for (let j = 0; j < n-i-1; j++) {
if (arr[j] > arr[j+1]) {
// swap elements
let temp = arr[j];
arr[j] = arr[j+1];
arr[j+1] = temp;
}
}
}
return arr;
}
This algorithm, like a recipe, provides a step-by-step process for sorting an array. Each step serves a specific purpose in achieving the final goal of a sorted array.
2. Ingredients and Variables: The Building Blocks
In cooking, ingredients are the fundamental components that, when combined correctly, create a dish. Similarly, in programming, variables are the basic elements that, when manipulated properly, form the basis of our algorithms.
Choosing the Right Ingredients
A chef carefully selects ingredients based on their properties and how they interact with other components of the dish. For instance, baking powder is used in cakes to create a light, airy texture, while yeast is preferred for bread to create a chewy consistency.
Selecting Appropriate Variables
In the same vein, a programmer chooses variables based on the specific requirements of the problem at hand. For example:
// For storing a person's age
let age: int = 25;
// For storing a person's name
let name: string = "John Doe";
// For storing a list of numbers
let numbers: array = [1, 2, 3, 4, 5];
// For storing a true/false condition
let isAdult: boolean = true;
Just as using salt instead of sugar would ruin a cake, using the wrong variable type can lead to errors or inefficiencies in your code.
3. Preparation and Initialization: Setting the Stage
Before diving into the main cooking process, chefs engage in mise en place – the preparation and organization of ingredients and tools. Similarly, programmers often need to initialize variables and set up their environment before executing the main algorithm.
Mise en Place in Cooking
A chef preparing to make a stir-fry might:
- Chop all vegetables
- Measure out sauces and spices
- Prepare the wok and cooking utensils
- Have all ingredients within reach
Initialization in Coding
A programmer preparing to implement a sorting algorithm might:
// Initialize the array to be sorted
let arr = [64, 34, 25, 12, 22, 11, 90];
// Set up variables for tracking the sorting process
let n = arr.length;
let swapped;
// Prepare any necessary helper functions
function swap(arr, i, j) {
let temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
Both processes involve setting up the necessary components to ensure smooth execution of the main task.
4. Techniques and Functions: The Tools of the Trade
Chefs employ various cooking techniques to transform raw ingredients into delicious dishes. Similarly, programmers use functions to manipulate data and perform specific tasks within their algorithms.
Cooking Techniques
Common cooking techniques include:
- Sautéing
- Roasting
- Braising
- Baking
- Grilling
Each technique is suited to different ingredients and desired outcomes. For example, sautéing is great for quickly cooking vegetables while maintaining their crispness, while braising is ideal for tenderizing tough cuts of meat.
Programming Functions
In programming, functions serve a similar purpose:
// Function to calculate the average of an array
function calculateAverage(arr) {
let sum = arr.reduce((a, b) => a + b, 0);
return sum / arr.length;
}
// Function to find the maximum value in an array
function findMax(arr) {
return Math.max(...arr);
}
// Function to check if a number is prime
function isPrime(num) {
if (num <= 1) return false;
for (let i = 2; i <= Math.sqrt(num); i++) {
if (num % i === 0) return false;
}
return true;
}
Like cooking techniques, these functions are tools that can be applied in various situations to achieve specific outcomes.
5. Timing and Complexity: The Art of Efficiency
In both cooking and coding, timing and efficiency are crucial. A chef must consider how long each component of a dish takes to cook and coordinate these timings to ensure everything is ready at the right moment. Similarly, a programmer must consider the time complexity of their algorithms to ensure efficient execution.
Timing in Cooking
When preparing a multi-course meal, a chef might plan like this:
- Start the slow-cooked main dish first (e.g., a roast)
- Prepare cold appetizers while the main dish cooks
- Begin cooking side dishes as the main dish nears completion
- Finish with quick-to-prepare desserts
Complexity in Coding
In programming, we use Big O notation to describe the time complexity of algorithms. For example:
// O(1) - Constant time
function getFirstElement(arr) {
return arr[0];
}
// O(n) - Linear time
function findElement(arr, target) {
for (let i = 0; i < arr.length; i++) {
if (arr[i] === target) return i;
}
return -1;
}
// O(n^2) - Quadratic time
function bubbleSort(arr) {
for (let i = 0; i < arr.length; i++) {
for (let j = 0; j < arr.length - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
[arr[j], arr[j + 1]] = [arr[j + 1], arr[j]];
}
}
}
return arr;
}
// O(log n) - Logarithmic time
function binarySearch(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left <= right) {
let mid = Math.floor((left + right) / 2);
if (arr[mid] === target) return mid;
if (arr[mid] < target) left = mid + 1;
else right = mid - 1;
}
return -1;
}
Just as a chef aims to minimize cooking time without sacrificing quality, a programmer strives to optimize algorithm efficiency while maintaining functionality.
6. Experimentation and Optimization: Refining the Process
Both cooking and coding involve a process of continuous improvement through experimentation and optimization. Chefs experiment with different ingredient combinations and cooking methods to enhance flavors and textures. Similarly, programmers refine their algorithms to improve performance and functionality.
Culinary Experimentation
A chef might experiment by:
- Adjusting ingredient ratios
- Trying different cooking temperatures
- Incorporating new ingredients
- Modifying cooking times
Algorithm Optimization
A programmer might optimize an algorithm by:
// Original function
function fibonacci(n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
// Optimized function using memoization
function fibonacciMemo(n, memo = {}) {
if (n in memo) return memo[n];
if (n <= 1) return n;
memo[n] = fibonacciMemo(n - 1, memo) + fibonacciMemo(n - 2, memo);
return memo[n];
}
In both cases, the goal is to improve the end result through iterative refinement and creative problem-solving.
7. Scalability: From Home Cooking to Industrial Kitchens
The principles of cooking apply whether you’re preparing a meal for two or catering for a thousand. Similarly, good coding practices should scale from small scripts to large, complex systems.
Scaling Up in Cooking
When scaling up a recipe, a chef must consider:
- Proportional increase of ingredients
- Adjustments to cooking times and temperatures
- Equipment capacity
- Workflow and team coordination
Scaling Up in Coding
When scaling up a program, a developer must consider:
// Small scale: Simple array manipulation
let numbers = [1, 2, 3, 4, 5];
let doubled = numbers.map(n => n * 2);
// Large scale: Distributed computing with Apache Spark
import org.apache.spark.sql.SparkSession
val spark = SparkSession.builder().appName("Large Scale Doubling").getOrCreate()
val numbers = spark.range(1, 1000000000) // 1 billion numbers
val doubled = numbers.map(n => n * 2)
doubled.show()
In both cases, the fundamental principles remain the same, but the implementation details change to accommodate the larger scale.
8. Documentation: Preserving Knowledge
Just as cherished family recipes are passed down through generations, well-documented code ensures that knowledge is preserved and can be understood by others.
Recipe Documentation
A well-documented recipe might include:
- List of ingredients with precise measurements
- Step-by-step instructions
- Cooking times and temperatures
- Special techniques or tips
- Variations or substitutions
Code Documentation
Well-documented code might look like this:
/**
* Calculates the Fibonacci number at a given position.
* Uses dynamic programming for optimized performance.
*
* @param {number} n - The position in the Fibonacci sequence
* @returns {number} The Fibonacci number at position n
*/
function fibonacci(n) {
if (n <= 1) return n;
let fib = [0, 1];
for (let i = 2; i <= n; i++) {
// Calculate next Fibonacci number
fib[i] = fib[i-1] + fib[i-2];
}
return fib[n];
}
Both forms of documentation serve to make the process reproducible and understandable to others.
9. Error Handling: Dealing with the Unexpected
In cooking, things don’t always go as planned. A sauce might separate, or a soufflé might fall. Similarly, in programming, unexpected inputs or system states can lead to errors. Both chefs and programmers need strategies to handle these situations gracefully.
Cooking Error Handling
A chef might handle errors by:
- Having backup ingredients on hand
- Knowing techniques to rescue dishes (e.g., how to fix a broken sauce)
- Having alternative recipes ready in case of a major failure
Programming Error Handling
A programmer might handle errors like this:
function divideNumbers(a, b) {
try {
if (b === 0) {
throw new Error("Cannot divide by zero");
}
return a / b;
} catch (error) {
console.error("An error occurred:", error.message);
return null;
} finally {
console.log("Division operation attempted");
}
}
console.log(divideNumbers(10, 2)); // Output: 5
console.log(divideNumbers(10, 0)); // Output: null (with error message)
In both cases, the goal is to gracefully handle unexpected situations and provide a way to recover or at least fail safely.
10. Creativity and Problem-Solving: The Heart of the Craft
At their core, both cooking and coding are creative endeavors that require problem-solving skills. A chef faced with dietary restrictions or limited ingredients must creatively adapt recipes. Similarly, a programmer faced with unique requirements or constraints must find innovative solutions.
Culinary Creativity
A chef might exercise creativity by:
- Fusion cuisine: combining elements from different culinary traditions
- Ingredient substitutions: finding alternatives for unavailable or restricted ingredients
- New cooking techniques: experimenting with molecular gastronomy or sous-vide
Coding Creativity
A programmer might demonstrate creativity through:
// Traditional approach to checking if a number is even
function isEven(num) {
return num % 2 === 0;
}
// Creative bitwise approach
function isEvenBitwise(num) {
return (num & 1) === 0;
}
// Creative approach to reversing a string
function reverseString(str) {
return str.split('').reduce((rev, char) => char + rev, '');
}
Both fields reward those who can think outside the box and come up with novel solutions to challenges.
Conclusion: The Recipe for Success
As we’ve explored, the parallels between cooking and coding are numerous and profound. Both disciplines require a mix of technical skill, creativity, and problem-solving ability. They both involve following structured processes while also knowing when and how to improvise. Whether you’re crafting a gourmet meal or designing a complex algorithm, the principles of preparation, execution, and refinement remain the same.
By recognizing these similarities, we can apply lessons from one field to the other. A programmer might approach a coding problem with the systematic mindset of a chef preparing a multi-course meal. A chef might debug a failed recipe with the analytical approach of a programmer troubleshooting code.
Ultimately, both cooking and coding are about creating something valuable from raw ingredients, whether those ingredients are food items or lines of code. They are both crafts that require practice, patience, and a willingness to learn from mistakes. And in both cases, the joy of creation and the satisfaction of a job well done are the ultimate rewards.
So the next time you’re stuck on a coding problem, why not take a break and cook a meal? You might find that the process of following a recipe inspires a new approach to your algorithm. Or when you’re experimenting in the kitchen, think about how your culinary creativity might translate to innovative coding solutions. By embracing the parallels between these two seemingly disparate fields, we can become more well-rounded and creative practitioners in both.