The OSI Model: A Comprehensive Guide for Aspiring Programmers
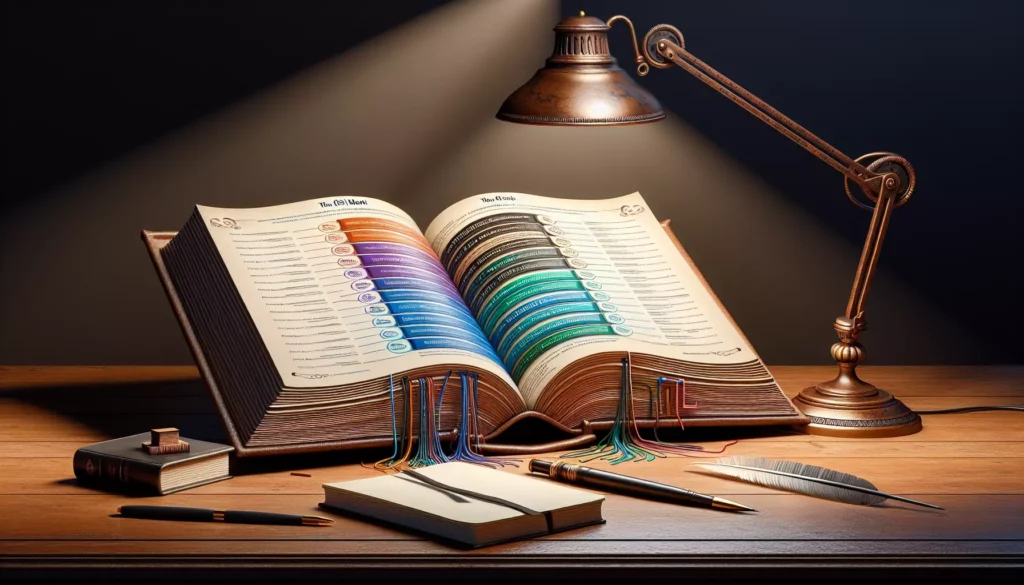
As an aspiring programmer or software developer, understanding network communication is crucial. One fundamental concept that forms the backbone of modern networking is the OSI (Open Systems Interconnection) model. This comprehensive guide will delve deep into the OSI model, its layers, and its relevance to coding and software development.
What is the OSI Model?
The OSI model is a conceptual framework that describes how data communication occurs between devices in a network. Developed by the International Organization for Standardization (ISO) in 1984, it provides a standardized way to understand network architecture and protocols.
The model consists of seven layers, each responsible for specific functions in the data communication process. These layers work together to ensure seamless communication between different devices and systems, regardless of their underlying hardware or software.
The Seven Layers of the OSI Model
Let’s explore each layer of the OSI model in detail, starting from the bottom:
1. Physical Layer
The Physical Layer is the lowest layer of the OSI model and deals with the physical transmission of data over a network medium. It defines the electrical, mechanical, and functional specifications for activating, maintaining, and deactivating physical connections.
Key aspects of the Physical Layer include:
- Bit-level transmission
- Physical network topologies
- Cable types and connectors
- Signal encoding and modulation
- Data transmission rates
For programmers, understanding the Physical Layer is crucial when working with low-level network programming or embedded systems. It provides insights into how data is physically transmitted and received, which can be valuable when optimizing network performance or troubleshooting connectivity issues.
2. Data Link Layer
The Data Link Layer is responsible for the reliable transfer of data between two directly connected nodes over a physical layer. It ensures that data is formatted correctly and transmitted with the necessary synchronization, error control, and flow control.
Key functions of the Data Link Layer include:
- Framing: Organizing data into frames
- Physical addressing (MAC addresses)
- Error detection and correction
- Flow control
- Access control for shared media
For programmers, the Data Link Layer is relevant when working with network drivers, implementing low-level protocols, or developing applications that require direct access to network interfaces. Understanding this layer can help in optimizing data transfer and implementing efficient error-handling mechanisms.
3. Network Layer
The Network Layer is responsible for routing data packets between different networks. It provides logical addressing and determines the best path for data to travel from the source to the destination.
Key functions of the Network Layer include:
- Logical addressing (IP addresses)
- Routing
- Packet forwarding
- Fragmentation and reassembly of packets
- Traffic control
For programmers, understanding the Network Layer is essential when developing applications that involve network communication, especially those that require knowledge of IP addressing and routing. This layer is crucial for implementing features like network discovery, load balancing, and creating custom routing protocols.
4. Transport Layer
The Transport Layer ensures reliable data transfer between end systems. It provides mechanisms for segmentation, flow control, and error control to guarantee that data is delivered accurately and in the correct order.
Key functions of the Transport Layer include:
- Segmentation and reassembly of data
- Connection-oriented and connectionless communication
- End-to-end error recovery
- Flow control
- Multiplexing and demultiplexing
For programmers, the Transport Layer is crucial when developing applications that require reliable data transfer, such as file transfer protocols or real-time communication systems. Understanding protocols like TCP (Transmission Control Protocol) and UDP (User Datagram Protocol) is essential for implementing efficient and robust network applications.
5. Session Layer
The Session Layer establishes, manages, and terminates sessions between applications. It provides mechanisms for organizing and synchronizing data exchange between applications running on different devices.
Key functions of the Session Layer include:
- Session establishment, maintenance, and termination
- Synchronization of data exchange
- Checkpointing and recovery
- Dialog control (half-duplex or full-duplex)
For programmers, understanding the Session Layer is important when developing applications that require long-lived connections or need to maintain state across multiple requests. This knowledge is particularly useful when implementing features like authentication, authorization, and session management in web applications.
6. Presentation Layer
The Presentation Layer is responsible for data formatting, encryption, and compression. It ensures that data exchanged between applications is in a format that can be understood by both the sender and receiver.
Key functions of the Presentation Layer include:
- Data formatting and conversion
- Encryption and decryption
- Data compression and decompression
- Character encoding and conversion
For programmers, the Presentation Layer is crucial when working with data serialization, encryption algorithms, or implementing data compression techniques. Understanding this layer is essential for developing secure applications and ensuring interoperability between different systems.
7. Application Layer
The Application Layer is the topmost layer of the OSI model and provides network services directly to end-users or applications. It defines protocols and interfaces used by applications for network communication.
Key functions of the Application Layer include:
- Providing network services to applications
- Defining application-level protocols
- Supporting client-server models
- Implementing user authentication and privacy mechanisms
For programmers, the Application Layer is where most high-level network programming occurs. Understanding this layer is crucial for developing network applications, implementing APIs, and working with protocols like HTTP, FTP, SMTP, and DNS.
The OSI Model in Practice: A Simple Example
To better understand how the OSI model works in practice, let’s consider a simple example of sending an email:
- Application Layer: The user composes an email using an email client (e.g., Outlook, Gmail).
- Presentation Layer: The email content is formatted, and any attachments are compressed and encrypted if necessary.
- Session Layer: A session is established with the email server.
- Transport Layer: The email data is segmented into smaller units (packets) for transmission.
- Network Layer: The packets are assigned IP addresses and routed through the network.
- Data Link Layer: The packets are framed and prepared for transmission over the physical medium.
- Physical Layer: The framed data is converted into electrical, optical, or radio signals and transmitted over the network.
At the receiving end, the process is reversed, with each layer performing its respective functions to reconstruct the original email message.
Relevance of the OSI Model in Modern Programming
While the OSI model is primarily a conceptual framework, understanding its principles is invaluable for modern programmers and software developers. Here’s why:
1. Network Programming
When developing network applications, knowledge of the OSI model helps in:
- Choosing appropriate protocols for different communication requirements
- Implementing efficient data transfer mechanisms
- Troubleshooting network-related issues
- Optimizing application performance across different network layers
2. API Design and Implementation
Understanding the OSI model aids in:
- Designing robust and scalable APIs
- Implementing secure communication channels
- Ensuring interoperability between different systems
- Defining clear boundaries between different layers of an application
3. Security Implementation
Knowledge of the OSI model is crucial for:
- Implementing encryption and authentication mechanisms
- Developing secure network protocols
- Identifying potential security vulnerabilities at different layers
- Designing comprehensive security solutions for networked applications
4. Performance Optimization
Understanding the OSI model helps in:
- Identifying bottlenecks in network communication
- Optimizing data transfer rates and reducing latency
- Implementing efficient caching mechanisms
- Designing scalable and high-performance network architectures
Practical Applications of the OSI Model in Coding
Let’s explore some practical applications of the OSI model in coding, with examples:
1. Socket Programming
Socket programming is a fundamental technique for network communication that primarily operates at the Transport Layer (Layer 4) of the OSI model. Here’s a simple example of a TCP server in Python:
import socket
def start_server():
host = 'localhost'
port = 12345
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_socket.bind((host, port))
server_socket.listen(1)
print(f"Server listening on {host}:{port}")
while True:
client_socket, address = server_socket.accept()
print(f"Connection from {address}")
data = client_socket.recv(1024).decode()
print(f"Received: {data}")
response = f"Server received: {data}"
client_socket.send(response.encode())
client_socket.close()
if __name__ == '__main__':
start_server()
This example demonstrates how to create a simple TCP server that listens for incoming connections, receives data from clients, and sends a response. Understanding the Transport Layer helps in implementing reliable data transfer and handling connection-oriented communication.
2. HTTP Client Implementation
Implementing an HTTP client involves working with the Application Layer (Layer 7) of the OSI model. Here’s a simple example using Python’s requests library:
import requests
def fetch_webpage(url):
try:
response = requests.get(url)
response.raise_for_status()
return response.text
except requests.exceptions.RequestException as e:
print(f"An error occurred: {e}")
return None
if __name__ == '__main__':
url = "https://www.example.com"
content = fetch_webpage(url)
if content:
print(f"Successfully fetched content from {url}")
print(content[:200]) # Print first 200 characters
else:
print(f"Failed to fetch content from {url}")
This example shows how to implement a simple HTTP client that fetches the content of a webpage. Understanding the Application Layer protocols like HTTP is crucial for developing web applications and APIs.
3. Implementing Encryption
Encryption is typically handled at the Presentation Layer (Layer 6) of the OSI model. Here’s an example of implementing simple encryption using Python’s cryptography library:
from cryptography.fernet import Fernet
def generate_key():
return Fernet.generate_key()
def encrypt_message(message, key):
f = Fernet(key)
encrypted_message = f.encrypt(message.encode())
return encrypted_message
def decrypt_message(encrypted_message, key):
f = Fernet(key)
decrypted_message = f.decrypt(encrypted_message).decode()
return decrypted_message
if __name__ == '__main__':
key = generate_key()
message = "Hello, World! This is a secret message."
encrypted = encrypt_message(message, key)
print(f"Encrypted message: {encrypted}")
decrypted = decrypt_message(encrypted, key)
print(f"Decrypted message: {decrypted}")
This example demonstrates how to implement basic encryption and decryption using the Fernet symmetric encryption algorithm. Understanding the Presentation Layer helps in implementing secure data transmission and storage in applications.
4. Network Packet Analysis
Analyzing network packets involves understanding multiple layers of the OSI model. Here’s an example using the scapy library in Python to capture and analyze network packets:
from scapy.all import *
def packet_callback(packet):
if packet.haslayer(IP):
ip_src = packet[IP].src
ip_dst = packet[IP].dst
print(f"IP: {ip_src} -> {ip_dst}")
if packet.haslayer(TCP):
sport = packet[TCP].sport
dport = packet[TCP].dport
print(f"TCP: {sport} -> {dport}")
if packet.haslayer(Raw):
print(f"Payload: {packet[Raw].load}")
print("---")
def capture_packets(interface, count):
sniff(iface=interface, prn=packet_callback, count=count)
if __name__ == '__main__':
interface = "eth0" # Change this to your network interface
packet_count = 10
print(f"Capturing {packet_count} packets on interface {interface}...")
capture_packets(interface, packet_count)
This example shows how to capture and analyze network packets, demonstrating the interplay between different OSI layers (Network, Transport, and Application). Understanding packet structure and analysis is crucial for network programming, security auditing, and troubleshooting.
Conclusion
The OSI model provides a comprehensive framework for understanding network communication and is an essential concept for aspiring programmers and software developers. By grasping the principles of each layer, you can develop more efficient, secure, and robust network applications.
As you progress in your coding journey, keep the OSI model in mind when working on network-related projects. It will help you make informed decisions about protocol selection, security implementation, and performance optimization. Remember that while real-world networking doesn’t always strictly adhere to the OSI model, its concepts remain invaluable for conceptualizing and solving network-related challenges.
Continue to explore and practice implementing features at different layers of the OSI model. This knowledge will prove invaluable as you tackle more complex networking projects and prepare for technical interviews at top tech companies. Happy coding!