The Most Common Mistake in Coding Interview Answers: A Comprehensive Guide
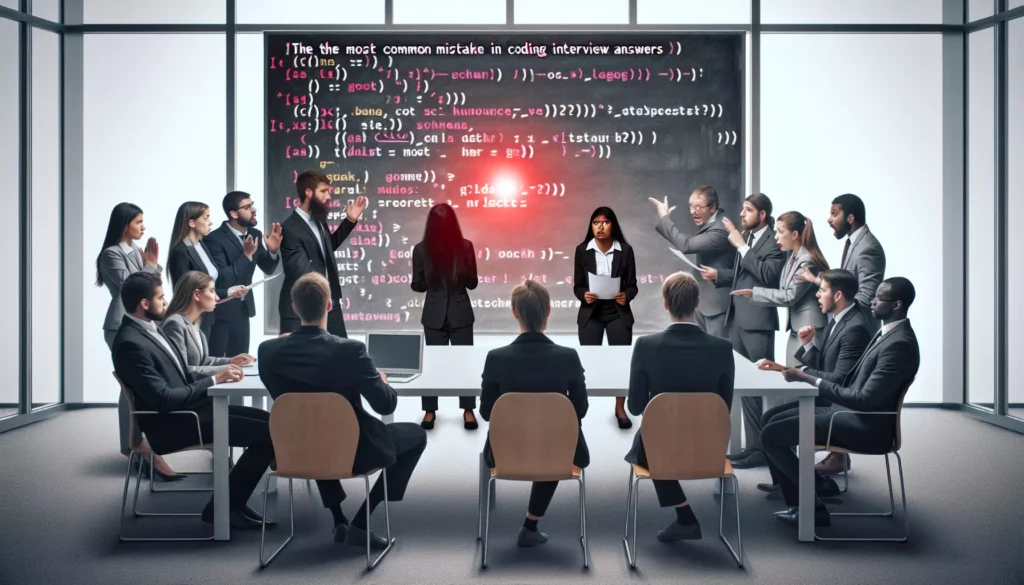
Coding interviews are a crucial step in landing your dream job in the tech industry. Whether you’re aiming for a position at a FAANG company (Facebook, Amazon, Apple, Netflix, Google) or any other tech giant, mastering the art of coding interviews is essential. However, even the most talented programmers can stumble during these high-pressure situations. In this comprehensive guide, we’ll explore the most common mistake in coding interview answers and provide you with strategies to avoid it, ultimately boosting your chances of success.
Understanding the Importance of Coding Interviews
Before we dive into the most common mistake, it’s crucial to understand why coding interviews are so important in the tech industry. These interviews serve several purposes:
- Assessing problem-solving skills
- Evaluating coding proficiency
- Gauging algorithmic thinking
- Testing communication abilities
- Determining cultural fit
Given the significance of these interviews, it’s no wonder that candidates often feel immense pressure to perform well. This pressure can lead to mistakes, but one stands out as the most common and potentially damaging.
The Most Common Mistake: Diving into Code Too Quickly
The single most common mistake that candidates make during coding interviews is diving into code too quickly. This error stems from a combination of factors, including nervousness, eagerness to impress, and a misunderstanding of what interviewers are looking for. Let’s break down why this is such a critical mistake and how it can impact your interview performance.
Why Rushing into Code is Problematic
- Lack of problem understanding: By jumping straight into coding, you may miss crucial details or misinterpret the problem statement.
- Inefficient solutions: Without proper planning, you’re more likely to implement a suboptimal solution that may not scale well.
- Difficulty in explaining thought process: Interviewers want to understand your thinking, which becomes challenging if you start coding immediately.
- Increased likelihood of errors: Rushing often leads to simple mistakes that could have been avoided with better preparation.
- Missed opportunities for optimization: You may overlook more efficient algorithms or data structures by not taking the time to analyze the problem thoroughly.
The Correct Approach: A Step-by-Step Guide
Now that we understand the most common mistake, let’s explore the correct approach to tackling coding interview questions. Following these steps will help you avoid the pitfall of diving into code too quickly and showcase your problem-solving skills effectively.
1. Clarify the Problem
Before writing a single line of code, make sure you fully understand the problem. Ask clarifying questions to eliminate any ambiguities. This step is crucial because it demonstrates your analytical skills and attention to detail.
Example questions to ask:
- What are the input constraints?
- How should I handle edge cases?
- Are there any performance requirements?
- Can you provide an example of expected input and output?
2. Discuss Potential Approaches
Once you have a clear understanding of the problem, brainstorm and discuss potential solutions with your interviewer. This step showcases your ability to think critically and consider multiple approaches.
Key points to cover:
- Different algorithms that could solve the problem
- Trade-offs between time and space complexity
- Potential edge cases and how to handle them
- Any assumptions you’re making about the problem
3. Outline Your Chosen Approach
After discussing potential solutions, choose the most appropriate approach and outline it before coding. This step helps organize your thoughts and provides a roadmap for implementation.
Elements to include in your outline:
- High-level algorithm description
- Key data structures you’ll use
- Main functions or methods you’ll implement
- Expected time and space complexity
4. Write Pseudocode
Before diving into actual code, write pseudocode to further refine your approach. This step helps catch logical errors early and makes the coding process smoother.
Benefits of writing pseudocode:
- Easier to spot logical flaws
- Helps break down complex problems into manageable steps
- Allows for quick iterations and improvements
- Demonstrates your ability to plan and structure code
5. Implement the Solution
Now that you have a solid plan, it’s time to write the actual code. As you implement your solution, explain your thought process and any decisions you’re making along the way.
Tips for effective implementation:
- Write clean, readable code
- Use meaningful variable and function names
- Comment on complex parts of your code
- Handle edge cases as discussed earlier
6. Test Your Solution
After implementing your solution, thoroughly test it with various inputs, including edge cases. This step demonstrates your attention to detail and commitment to producing reliable code.
Testing strategies:
- Use small, simple test cases first
- Gradually increase complexity of test cases
- Test edge cases and boundary conditions
- Consider using a test-driven development approach
7. Optimize and Refine
If time allows, look for opportunities to optimize your solution. This could involve improving the time or space complexity, enhancing readability, or adding additional features.
Areas to consider for optimization:
- Algorithmic improvements
- Code refactoring for better readability
- Memory usage optimization
- Error handling and robustness
Real-World Example: Solving the Two Sum Problem
To illustrate the correct approach, let’s walk through solving the classic “Two Sum” problem, a common coding interview question.
Problem Statement: Given an array of integers and a target sum, return the indices of two numbers in the array that add up to the target sum.
Step 1: Clarify the Problem
Questions to ask:
- Can the array contain negative numbers?
- Are there always exactly two numbers that sum to the target?
- What should we return if no solution is found?
- Can we assume the input array is sorted?
Step 2: Discuss Potential Approaches
Potential solutions:
- Brute force approach: Check all pairs of numbers (O(n^2) time complexity)
- Sorting and two-pointer approach: Sort the array and use two pointers (O(n log n) time complexity)
- Hash table approach: Use a hash table to store complements (O(n) time complexity)
Step 3: Outline Chosen Approach
Let’s choose the hash table approach for its optimal time complexity:
- Iterate through the array once
- For each number, calculate its complement (target – number)
- Check if the complement exists in the hash table
- If found, return the current index and the complement’s index
- If not found, add the current number and its index to the hash table
Step 4: Write Pseudocode
function twoSum(nums, target):
create empty hash table
for i from 0 to length of nums - 1:
complement = target - nums[i]
if complement exists in hash table:
return [hash table[complement], i]
else:
add nums[i] and i to hash table
return [] // No solution found
Step 5: Implement the Solution
Here’s the Python implementation of the Two Sum solution:
def two_sum(nums, target):
complement_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in complement_dict:
return [complement_dict[complement], i]
complement_dict[num] = i
return [] # No solution found
Step 6: Test Your Solution
Let’s test our implementation with various cases:
print(two_sum([2, 7, 11, 15], 9)) # Expected: [0, 1]
print(two_sum([3, 2, 4], 6)) # Expected: [1, 2]
print(two_sum([3, 3], 6)) # Expected: [0, 1]
print(two_sum([1, 2, 3, 4], 10)) # Expected: []
Step 7: Optimize and Refine
Our solution already has an optimal time complexity of O(n). However, we can improve its readability and add error handling:
def two_sum(nums, target):
if not isinstance(nums, list) or not all(isinstance(x, (int, float)) for x in nums):
raise ValueError("Input must be a list of numbers")
if not isinstance(target, (int, float)):
raise ValueError("Target must be a number")
complement_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in complement_dict:
return [complement_dict[complement], i]
complement_dict[num] = i
return None # Changed from [] to None for clarity
# Example usage
try:
result = two_sum([2, 7, 11, 15], 9)
print(f"Result: {result}")
except ValueError as e:
print(f"Error: {e}")
Common Variations of the Most Common Mistake
While diving into code too quickly is the most common mistake, there are several variations and related errors that candidates often make during coding interviews. Being aware of these can help you avoid them and improve your overall performance.
1. Neglecting to Consider Edge Cases
Even when candidates take the time to plan their approach, they sometimes forget to consider edge cases. This oversight can lead to incomplete or incorrect solutions.
How to avoid this:
- Always ask about potential edge cases during the problem clarification stage
- Include edge case handling in your pseudocode and implementation
- Test your solution with edge cases before declaring it complete
2. Overcomplicating the Solution
In an attempt to impress the interviewer, some candidates may choose unnecessarily complex solutions when a simpler approach would suffice.
How to avoid this:
- Start with the simplest solution that solves the problem correctly
- Discuss trade-offs between simplicity and efficiency with your interviewer
- Only introduce complexity if it significantly improves performance and is justified
3. Poor Time Management
Spending too much time on planning or getting stuck on a particular aspect of the problem can leave insufficient time for implementation and testing.
How to avoid this:
- Practice time management during mock interviews
- Set mental time limits for each step of the problem-solving process
- If stuck, communicate with the interviewer and ask for hints if necessary
4. Failing to Communicate Effectively
Some candidates focus so intently on solving the problem that they forget to communicate their thought process clearly to the interviewer.
How to avoid this:
- Practice explaining your thought process out loud while solving problems
- Engage with the interviewer throughout the problem-solving process
- Ask for feedback and clarification if you’re unsure about your approach
5. Ignoring Space Complexity
While time complexity is often the primary focus, neglecting space complexity can lead to suboptimal solutions, especially for large-scale problems.
How to avoid this:
- Always consider both time and space complexity when discussing potential approaches
- Be prepared to explain the space complexity of your chosen solution
- Look for opportunities to optimize space usage in your implementation
The Role of Practice in Avoiding Common Mistakes
Recognizing the most common mistake in coding interviews is just the first step. To truly excel in these high-pressure situations, consistent practice is essential. Here are some strategies to incorporate into your preparation:
1. Regular Problem Solving
Set aside time each day to solve coding problems. Platforms like LeetCode, HackerRank, and AlgoCademy offer a wide range of problems to practice with.
2. Mock Interviews
Participate in mock interviews with friends, mentors, or through online platforms. This helps simulate the pressure of real interviews and allows you to practice your communication skills.
3. Time-Boxed Problem Solving
Practice solving problems within a set time limit to improve your time management skills and ability to work under pressure.
4. Code Reviews
Have your solutions reviewed by peers or mentors. This can help you identify areas for improvement in your problem-solving approach and coding style.
5. Study Data Structures and Algorithms
Regularly review and study fundamental data structures and algorithms. A strong foundation in these areas will help you approach problems more effectively.
6. Analyze Official Solutions
After solving a problem, study official solutions or highly-rated community solutions. This can expose you to different approaches and optimization techniques.
7. Reflect on Your Performance
After each practice session or mock interview, take time to reflect on what went well and what could be improved. This self-awareness will help you grow as a problem solver.
Conclusion: Mastering the Art of Coding Interviews
The most common mistake in coding interview answers – diving into code too quickly – is a pitfall that many candidates fall into. By understanding this mistake and following the structured approach outlined in this guide, you can significantly improve your performance in coding interviews.
Remember, success in coding interviews is not just about solving the problem correctly. It’s about demonstrating your problem-solving process, communication skills, and ability to write clean, efficient code. By avoiding the common mistake of rushing into implementation and instead taking a methodical approach, you’ll be better equipped to showcase your true potential to interviewers.
As you continue your journey in preparing for coding interviews, keep in mind that practice and persistence are key. Utilize resources like AlgoCademy to hone your skills, and don’t be discouraged by setbacks. Each interview, whether successful or not, is an opportunity to learn and improve.
With dedication and the right approach, you’ll be well on your way to acing your coding interviews and landing your dream job in the tech industry. Good luck, and happy coding!