The Minimalist’s Guide to Coding Interviews: Solving Problems with the Least Amount of Code
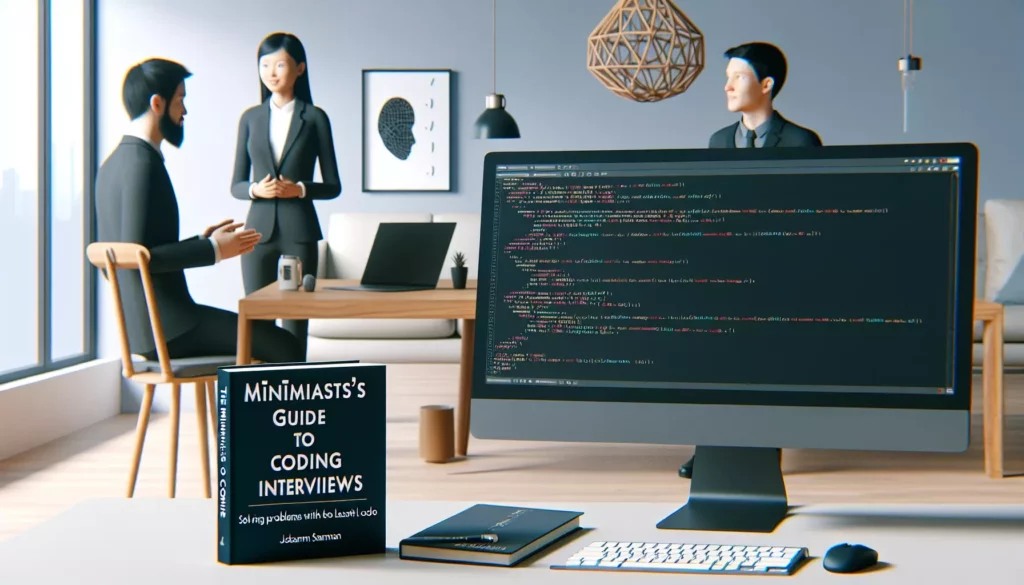
In the world of software development, coding interviews have become a rite of passage for aspiring programmers and seasoned professionals alike. As candidates vie for positions at top tech companies, the ability to solve complex problems efficiently and elegantly has never been more crucial. Enter the minimalist approach to coding interviews – a strategy that emphasizes solving problems with the least amount of code possible. This guide will explore how embracing minimalism can not only improve your performance in coding interviews but also make you a better programmer overall.
Why Minimalism Matters in Coding Interviews
Before we dive into the techniques and strategies, let’s understand why a minimalist approach is valuable in coding interviews:
- Clarity and Readability: Concise code is often easier to read and understand, both for you and your interviewer.
- Efficiency: Minimalist solutions are frequently more efficient in terms of time and space complexity.
- Demonstrating Mastery: Writing minimal code shows a deep understanding of the language and problem-solving techniques.
- Time Management: In time-constrained interviews, writing less code means more time for testing and optimization.
- Reduced Bug Potential: Fewer lines of code generally mean fewer opportunities for bugs to creep in.
Fundamental Principles of Minimalist Coding
To excel in coding interviews with a minimalist approach, internalize these key principles:
1. Understand the Problem Thoroughly
Before writing a single line of code, ensure you fully grasp the problem. Ask clarifying questions and consider edge cases. A deep understanding often leads to simpler solutions.
2. Plan Before You Code
Spend time planning your approach. Sketch out your algorithm or data structure on paper or a whiteboard. This pre-coding phase can significantly reduce the amount of code you’ll need to write.
3. Leverage Built-in Functions and Libraries
Most programming languages come with powerful built-in functions and standard libraries. Familiarize yourself with these tools to avoid reinventing the wheel.
4. Choose the Right Data Structures
Selecting the appropriate data structure can dramatically simplify your code. For example, using a set instead of a list for quick lookups can eliminate the need for nested loops.
5. Embrace Functional Programming Concepts
Functional programming techniques like map, filter, and reduce can often replace multiple lines of imperative code with a single, expressive line.
Minimalist Coding Techniques
Now that we’ve covered the principles, let’s explore some specific techniques to write minimal code during interviews:
1. Use List Comprehensions (Python)
List comprehensions in Python allow you to create lists in a single line of code. They’re concise and often more readable than traditional loops.
# Instead of:
squared_numbers = []
for i in range(10):
squared_numbers.append(i ** 2)
# Use:
squared_numbers = [i ** 2 for i in range(10)]
2. Leverage the Ternary Operator
The ternary operator can replace simple if-else statements, reducing your code to a single line.
# Instead of:
if x > 0:
result = "Positive"
else:
result = "Non-positive"
# Use:
result = "Positive" if x > 0 else "Non-positive"
3. Utilize Short-Circuit Evaluation
Take advantage of short-circuit evaluation in logical operations to write more concise conditional statements.
# Instead of:
if x is not None and len(x) > 0:
do_something(x)
# Use:
x and do_something(x)
4. Master String Formatting
Use f-strings (in Python) or template literals (in JavaScript) for cleaner string formatting.
# Python
name = "Alice"
age = 30
message = f"{name} is {age} years old."
// JavaScript
const name = "Alice";
const age = 30;
const message = `${name} is ${age} years old.`;
5. Employ Destructuring Assignments
Destructuring assignments can make your code more readable and concise, especially when working with arrays or objects.
# Python
a, b = [1, 2]
// JavaScript
const [a, b] = [1, 2];
const { name, age } = person;
Minimalist Approaches to Common Interview Problems
Let’s apply these minimalist techniques to some common coding interview problems:
1. Reversing a String
# Python
def reverse_string(s):
return s[::-1]
// JavaScript
const reverseString = s => s.split('').reverse().join('');
2. Finding the Maximum Element in an Array
# Python
def find_max(arr):
return max(arr)
// JavaScript
const findMax = arr => Math.max(...arr);
3. Checking if a String is a Palindrome
# Python
def is_palindrome(s):
return s == s[::-1]
// JavaScript
const isPalindrome = s => s === s.split('').reverse().join('');
4. Removing Duplicates from an Array
# Python
def remove_duplicates(arr):
return list(set(arr))
// JavaScript
const removeDuplicates = arr => [...new Set(arr)];
5. Counting Word Frequency
# Python
from collections import Counter
def word_frequency(text):
return Counter(text.split())
// JavaScript
const wordFrequency = text =>
text.split(' ').reduce((acc, word) => ({...acc, [word]: (acc[word] || 0) + 1}), {});
Advanced Minimalist Techniques
As you progress in your coding journey, consider these advanced minimalist techniques:
1. Recursion for Elegant Solutions
Recursive solutions can often be more concise than their iterative counterparts. However, use recursion judiciously, as it can impact performance for large inputs.
# Python: Factorial calculation
def factorial(n):
return 1 if n <= 1 else n * factorial(n - 1)
2. Generator Expressions for Memory Efficiency
In Python, generator expressions can be more memory-efficient than list comprehensions for large datasets.
# Instead of:
sum([x**2 for x in range(1000000)])
# Use:
sum(x**2 for x in range(1000000))
3. Lambda Functions for Quick One-liners
Lambda functions can be useful for simple operations, especially when used with higher-order functions like map, filter, and reduce.
# Python
squares = list(map(lambda x: x**2, range(10)))
// JavaScript
const squares = Array.from({length: 10}, (_, i) => i ** 2);
4. Method Chaining for Fluent Interfaces
Method chaining can lead to more readable and concise code, especially when working with objects or data processing pipelines.
# Python (using pandas)
result = (df
.groupby('category')
.agg({'sales': 'sum'})
.sort_values('sales', ascending=False)
.head(5)
)
// JavaScript (using lodash)
const result = _
.chain(data)
.groupBy('category')
.mapValues(group => _.sumBy(group, 'sales'))
.toPairs()
.sortBy(1)
.reverse()
.take(5)
.fromPairs()
.value();
5. Bitwise Operations for Optimization
In certain scenarios, bitwise operations can lead to highly optimized and concise solutions, especially for problems involving powers of 2 or binary representations.
# Python: Check if a number is a power of 2
def is_power_of_two(n):
return n > 0 and (n & (n - 1)) == 0
// JavaScript: Swap two numbers without a temporary variable
let a = 5, b = 10;
a ^= b;
b ^= a;
a ^= b;
Balancing Minimalism and Readability
While striving for minimalism in your code is admirable, it’s crucial to maintain a balance between conciseness and readability. Here are some tips to ensure your minimalist code remains clear and maintainable:
1. Use Descriptive Variable Names
Even in short code snippets, use meaningful variable names. This helps convey the purpose of each element in your solution.
# Less clear
def f(l):
return sum(x for x in l if x % 2 == 0)
# More clear
def sum_even_numbers(numbers):
return sum(num for num in numbers if num % 2 == 0)
2. Add Comments for Complex Logic
If your minimalist solution involves complex logic or non-obvious techniques, add brief comments to explain your approach.
# Python: Find the single number in an array where every other number appears twice
def find_single(nums):
# Use XOR to cancel out pairs, leaving the single number
return reduce(lambda x, y: x ^ y, nums)
3. Break Down Complex One-liners
If a one-liner becomes too complex or hard to read, consider breaking it down into multiple lines or separate functions.
# Instead of:
result = [func1(func2(x)) for x in data if condition(x)]
# Consider:
def process_item(x):
if condition(x):
return func1(func2(x))
return None
result = [processed for processed in map(process_item, data) if processed is not None]
4. Use Type Hints (in Python) or JSDoc (in JavaScript)
Adding type information can make your code more self-documenting and catch potential errors early.
# Python with type hints
def binary_search(arr: List[int], target: int) -> int:
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
// JavaScript with JSDoc
/**
* Performs binary search on a sorted array.
* @param {number[]} arr - The sorted array to search.
* @param {number} target - The target value to find.
* @returns {number} The index of the target if found, or -1 if not found.
*/
function binarySearch(arr, target) {
let left = 0, right = arr.length - 1;
while (left <= right) {
const mid = Math.floor((left + right) / 2);
if (arr[mid] === target) return mid;
if (arr[mid] < target) left = mid + 1;
else right = mid - 1;
}
return -1;
}
Common Pitfalls to Avoid
While pursuing minimalism in your coding interview solutions, be wary of these common pitfalls:
1. Sacrificing Readability for Brevity
Don’t make your code so terse that it becomes difficult to understand. Remember, your interviewer needs to follow your thought process.
2. Overusing Advanced Language Features
While using advanced language features can lead to concise code, make sure you can explain how they work. Don’t use them just to show off.
3. Ignoring Edge Cases
In the pursuit of minimal code, don’t forget to handle edge cases and potential errors. A concise solution that fails on edge cases is worse than a slightly longer, robust solution.
4. Premature Optimization
Focus on getting a correct solution first, then optimize if time allows. Don’t sacrifice correctness or clarity for minor performance gains.
5. Neglecting Time and Space Complexity
A minimal solution isn’t always the most efficient. Be prepared to discuss the time and space complexity of your approach.
Practicing Minimalist Coding
To improve your minimalist coding skills for interviews, consider these practice strategies:
1. Solve Problems Multiple Times
After solving a problem, try to solve it again with a focus on minimizing your code. Compare your solutions and analyze the trade-offs.
2. Study Others’ Solutions
Platforms like LeetCode and HackerRank often showcase highly optimized and concise solutions. Study these to learn new techniques.
3. Participate in Coding Challenges
Many online platforms host coding challenges that emphasize solving problems with minimal code. These can be great practice for interviews.
4. Refactor Existing Code
Take existing, verbose solutions and challenge yourself to refactor them into more concise versions without losing functionality.
5. Pair Programming
Practice with a friend or mentor. Explain your minimalist solutions and get feedback on clarity and effectiveness.
Conclusion
Mastering the art of minimalist coding can significantly enhance your performance in technical interviews. By writing concise, efficient, and readable code, you demonstrate not only your problem-solving skills but also your deep understanding of the programming language and best practices.
Remember, the goal is not just to write the least amount of code possible, but to find the sweet spot between brevity and clarity. As you practice and refine your skills, you’ll develop an intuition for when to apply minimalist techniques and when a more detailed approach is necessary.
Ultimately, the minimalist approach to coding interviews is about more than just impressing your interviewer. It’s about developing a mindset that values efficiency, clarity, and elegance in your code – qualities that will serve you well throughout your career as a software developer.
As you prepare for your next coding interview, challenge yourself to think minimally. Embrace the techniques and principles outlined in this guide, but always prioritize correctness and readability. With practice and dedication, you’ll find yourself solving complex problems with elegant, minimal solutions, setting yourself apart as a skilled and efficient programmer.