The Mathematics Behind Algorithm Design: Unlocking the Power of Computational Thinking
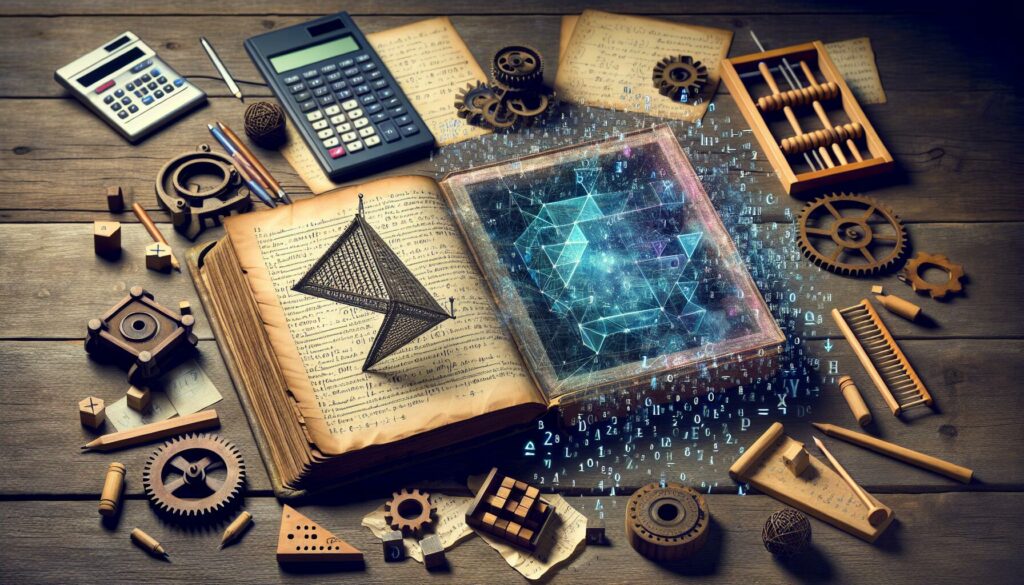
In the ever-evolving landscape of computer science and software engineering, algorithm design stands as a cornerstone of efficient problem-solving and optimized computational processes. At its core, algorithm design is deeply rooted in mathematical principles, drawing from various branches such as discrete mathematics, graph theory, and probability. This intricate relationship between mathematics and algorithm design not only shapes the way we approach complex problems but also forms the foundation of modern computing.
In this comprehensive exploration, we’ll delve into the mathematical concepts that underpin algorithm design, understand their practical applications, and see how they contribute to creating elegant and efficient solutions in the world of programming. Whether you’re a budding programmer looking to enhance your skills or an experienced developer aiming to refine your algorithmic thinking, this journey through the mathematics of algorithm design will provide valuable insights and deepen your understanding of computational problem-solving.
1. The Foundations: Discrete Mathematics in Algorithm Design
Discrete mathematics serves as the bedrock for many concepts in computer science, particularly in algorithm design. Unlike continuous mathematics, which deals with smooth and unbroken functions, discrete mathematics focuses on distinct, separate values. This makes it particularly well-suited for describing computational processes and data structures.
1.1 Set Theory and Its Applications
Set theory is a fundamental concept in discrete mathematics that finds extensive use in algorithm design. Sets are collections of distinct objects, and operations on sets such as union, intersection, and complement form the basis for many algorithmic operations.
For example, when designing algorithms for database operations, set theory plays a crucial role:
- Union operations are used in merging datasets
- Intersection helps in finding common elements between two sets
- Set difference is useful in filtering out unwanted data
Consider a simple example of using set operations in Python:
set_a = {1, 2, 3, 4, 5}
set_b = {4, 5, 6, 7, 8}
union_result = set_a.union(set_b)
intersection_result = set_a.intersection(set_b)
difference_result = set_a.difference(set_b)
print(f"Union: {union_result}")
print(f"Intersection: {intersection_result}")
print(f"Difference (A - B): {difference_result}")
This code demonstrates how set operations can be efficiently implemented and used in algorithms dealing with data manipulation and analysis.
1.2 Logic and Boolean Algebra
Logic and Boolean algebra form the foundation of conditional statements and decision-making in algorithms. Boolean algebra, with its operations like AND, OR, and NOT, is essential for creating complex conditional logic in algorithms.
For instance, in designing search algorithms or implementing decision trees, Boolean logic plays a pivotal role. Consider this pseudocode for a simple decision tree:
if (condition_A AND condition_B) OR (NOT condition_C):
execute_action_1()
else if condition_D XOR condition_E:
execute_action_2()
else:
execute_default_action()
This structure demonstrates how Boolean algebra can be used to create complex decision pathways in algorithms, enabling them to handle various scenarios efficiently.
1.3 Combinatorics and Its Role in Optimization
Combinatorics, the study of counting, arrangement, and combination of objects, is crucial in algorithm design, especially for optimization problems. It helps in understanding the number of possible solutions or arrangements, which is vital for designing efficient search and optimization algorithms.
For example, in solving the Traveling Salesman Problem (TSP), combinatorics helps us understand the complexity of the problem. For n cities, there are (n-1)! possible routes. This understanding guides the development of heuristic algorithms that can find near-optimal solutions without exploring all possibilities.
Here’s a simple Python function to calculate the number of possible routes for the TSP:
import math
def calculate_tsp_routes(num_cities):
return math.factorial(num_cities - 1)
print(f"Number of possible routes for 5 cities: {calculate_tsp_routes(5)}")
print(f"Number of possible routes for 10 cities: {calculate_tsp_routes(10)}")
This calculation demonstrates how quickly the problem space grows, emphasizing the need for efficient algorithmic approaches in combinatorial optimization problems.
2. Graph Theory: The Backbone of Network Algorithms
Graph theory is a branch of mathematics that studies the relationships between objects. In computer science, it forms the basis for numerous algorithms dealing with networks, paths, and connectivity.
2.1 Fundamentals of Graph Theory
A graph consists of vertices (nodes) and edges (connections between nodes). This simple structure can represent a wide variety of real-world scenarios, from social networks to computer networks, from transportation systems to data dependencies.
Key concepts in graph theory include:
- Directed vs Undirected Graphs
- Weighted vs Unweighted Graphs
- Connected Components
- Cycles and Paths
These concepts are fundamental to designing algorithms for problems like shortest path finding, network flow, and connectivity analysis.
2.2 Graph Traversal Algorithms
Graph traversal algorithms are essential for exploring and analyzing graph structures. The two primary traversal methods are:
- Depth-First Search (DFS)
- Breadth-First Search (BFS)
Here’s a simple implementation of DFS in Python:
def dfs(graph, start, visited=None):
if visited is None:
visited = set()
visited.add(start)
print(start, end=' ')
for next in graph[start] - visited:
dfs(graph, next, visited)
return visited
graph = {'A': set(['B', 'C']),
'B': set(['A', 'D', 'E']),
'C': set(['A', 'F']),
'D': set(['B']),
'E': set(['B', 'F']),
'F': set(['C', 'E'])}
dfs(graph, 'A')
This DFS implementation showcases how graph theory concepts translate into practical algorithms for exploring network structures.
2.3 Shortest Path Algorithms
Shortest path algorithms are crucial in various applications, from route planning to network optimization. Some key algorithms include:
- Dijkstra’s Algorithm: For finding the shortest path between nodes in a graph
- Bellman-Ford Algorithm: Useful for graphs with negative edge weights
- Floyd-Warshall Algorithm: For finding shortest paths between all pairs of vertices
These algorithms leverage graph theory to solve complex path-finding problems efficiently. For instance, Dijkstra’s algorithm uses a greedy approach to find the optimal path, demonstrating how mathematical principles guide algorithmic design.
3. Probability and Randomized Algorithms
Probability theory plays a significant role in the design of randomized algorithms, which use random numbers to guide their execution. These algorithms often provide efficient solutions to problems that are difficult to solve deterministically.
3.1 Basics of Probability in Algorithms
Understanding probability distributions, expected values, and variance is crucial for analyzing the performance of randomized algorithms. These concepts help in predicting the behavior and efficiency of algorithms that rely on random choices.
For example, in the analysis of quicksort with random pivot selection, probability theory helps us understand the expected running time and the likelihood of worst-case scenarios.
3.2 Monte Carlo Algorithms
Monte Carlo algorithms use repeated random sampling to obtain numerical results. They are particularly useful for problems where a deterministic approach is impractical or impossible.
A classic example is the estimation of π using the Monte Carlo method:
import random
def estimate_pi(num_points):
inside_circle = 0
total_points = num_points
for _ in range(total_points):
x = random.uniform(-1, 1)
y = random.uniform(-1, 1)
if x*x + y*y <= 1:
inside_circle += 1
pi_estimate = 4 * inside_circle / total_points
return pi_estimate
print(f"Estimated value of π: {estimate_pi(1000000)}")
This algorithm demonstrates how randomization can be used to approximate complex mathematical values, showcasing the power of probabilistic methods in algorithm design.
3.3 Las Vegas Algorithms
Unlike Monte Carlo algorithms, Las Vegas algorithms always produce the correct result but have a randomized running time. These algorithms are useful when correctness is crucial, but efficiency can vary.
An example of a Las Vegas algorithm is the randomized quicksort, where the pivot is chosen randomly. This approach helps avoid worst-case scenarios that can occur with deterministic pivot selection.
4. Numerical Methods and Approximation Algorithms
Numerical methods are mathematical tools used to find approximate solutions to problems that cannot be solved analytically. In algorithm design, these methods are crucial for dealing with continuous problems in a discrete computational environment.
4.1 Numerical Integration and Differentiation
Numerical integration and differentiation techniques are essential for solving problems in scientific computing, simulations, and data analysis. Algorithms like the trapezoidal rule or Simpson’s rule for integration, and finite difference methods for differentiation, are based on mathematical approximations.
Here’s a simple implementation of the trapezoidal rule for numerical integration in Python:
def trapezoidal_rule(f, a, b, n):
h = (b - a) / n
sum = 0.5 * (f(a) + f(b))
for i in range(1, n):
x = a + i * h
sum += f(x)
return h * sum
# Example usage
import math
def f(x):
return math.sin(x)
result = trapezoidal_rule(f, 0, math.pi, 1000)
print(f"Integral of sin(x) from 0 to π: {result}")
This algorithm demonstrates how mathematical concepts of calculus are translated into computational methods for solving complex problems.
4.2 Linear Programming and Optimization
Linear programming is a method for achieving the best outcome in a mathematical model whose requirements are represented by linear relationships. It’s widely used in optimization problems across various fields, including resource allocation, production planning, and network flow problems.
The simplex algorithm, a cornerstone of linear programming, is a prime example of how mathematical theory translates into practical algorithmic solutions for complex optimization problems.
4.3 Approximation Algorithms for NP-Hard Problems
For many NP-hard problems, finding exact solutions in polynomial time is believed to be impossible. Approximation algorithms provide near-optimal solutions to these problems in reasonable time frames.
For instance, the greedy approximation algorithm for the set cover problem demonstrates how mathematical analysis can guide the development of algorithms that provide guaranteed approximation ratios:
def greedy_set_cover(universe, subsets):
elements = set(e for s in subsets for e in s)
if elements != universe:
return None
covered = set()
cover = []
while covered != elements:
subset = max(subsets, key=lambda s: len(s - covered))
cover.append(subset)
covered |= subset
return cover
# Example usage
universe = set(range(1, 11))
subsets = [set([1, 2, 3, 4, 5]),
set([4, 5, 6, 7]),
set([6, 7, 8, 9, 10]),
set([1, 2, 3, 4]),
set([4, 5, 6]),
set([7, 8, 9, 10])]
result = greedy_set_cover(universe, subsets)
print("Greedy Set Cover Solution:", result)
This algorithm showcases how mathematical principles guide the development of approximation techniques for complex combinatorial problems.
5. Computational Complexity and Algorithm Analysis
Understanding the mathematical foundations of computational complexity is crucial for analyzing and comparing algorithms. This field provides the tools to measure and predict algorithm performance, guiding the design of efficient solutions.
5.1 Big O Notation and Asymptotic Analysis
Big O notation is a mathematical notation used to describe the limiting behavior of a function when the argument tends towards a particular value or infinity. In algorithm analysis, it’s used to classify algorithms according to how their running time or space requirements grow as the input size grows.
Key concepts include:
- O(1): Constant time complexity
- O(log n): Logarithmic time complexity
- O(n): Linear time complexity
- O(n log n): Linearithmic time complexity
- O(n²): Quadratic time complexity
- O(2â¿): Exponential time complexity
Understanding these concepts helps in predicting algorithm performance and making informed decisions about algorithm selection and optimization.
5.2 Recurrence Relations and Master Theorem
Recurrence relations are equations that define a sequence recursively. They are particularly useful in analyzing the time complexity of recursive algorithms. The Master Theorem provides a cookbook method for solving a broad class of recurrence relations.
For example, the recurrence relation for the Merge Sort algorithm is:
T(n) = 2T(n/2) + O(n)
Using the Master Theorem, we can determine that the time complexity of Merge Sort is O(n log n). This mathematical analysis guides our understanding of the algorithm’s efficiency and scalability.
5.3 Space-Time Tradeoffs
The study of space-time tradeoffs involves analyzing how adjusting the space usage of an algorithm affects its time complexity, and vice versa. This concept is crucial in designing algorithms that balance efficiency with resource constraints.
For instance, dynamic programming often trades increased space usage for improved time complexity. The Fibonacci sequence calculation demonstrates this tradeoff:
def fibonacci_recursive(n):
if n <= 1:
return n
return fibonacci_recursive(n-1) + fibonacci_recursive(n-2)
def fibonacci_dynamic(n):
fib = [0] * (n + 1)
fib[1] = 1
for i in range(2, n + 1):
fib[i] = fib[i-1] + fib[i-2]
return fib[n]
# Compare performance
import time
n = 30
start = time.time()
print(f"Recursive Fibonacci({n}): {fibonacci_recursive(n)}")
print(f"Time taken: {time.time() - start} seconds")
start = time.time()
print(f"Dynamic Fibonacci({n}): {fibonacci_dynamic(n)}")
print(f"Time taken: {time.time() - start} seconds")
This example illustrates how mathematical analysis of algorithms can guide practical implementation choices, balancing time efficiency with memory usage.
Conclusion: The Symbiosis of Mathematics and Algorithm Design
As we’ve explored throughout this article, the relationship between mathematics and algorithm design is deep and multifaceted. From the foundational concepts of discrete mathematics to the complexities of computational analysis, mathematical principles permeate every aspect of algorithm development and optimization.
Key takeaways include:
- Discrete mathematics provides the language and tools for describing and manipulating computational structures.
- Graph theory forms the backbone of network algorithms and data structure design.
- Probability theory enables the development of powerful randomized algorithms.
- Numerical methods bridge the gap between continuous mathematical problems and discrete computational solutions.
- Computational complexity analysis, rooted in mathematical principles, guides algorithm design and optimization.
For aspiring programmers and seasoned developers alike, a strong grasp of these mathematical concepts is invaluable. It not only enhances problem-solving skills but also provides a deeper understanding of why certain algorithmic approaches work and how they can be optimized.
As the field of computer science continues to evolve, the synergy between mathematics and algorithm design will undoubtedly lead to new breakthroughs and innovations. By embracing this mathematical foundation, developers can unlock new levels of creativity and efficiency in their algorithmic thinking, paving the way for more elegant, powerful, and optimized software solutions.
Remember, every line of code you write is an expression of mathematical logic. By honing your mathematical skills alongside your programming abilities, you’re not just becoming a better coder – you’re becoming a more complete and versatile problem solver in the digital age.