The Mariner’s Guide to Coding: Navigating Software Seas with Nautical Techniques
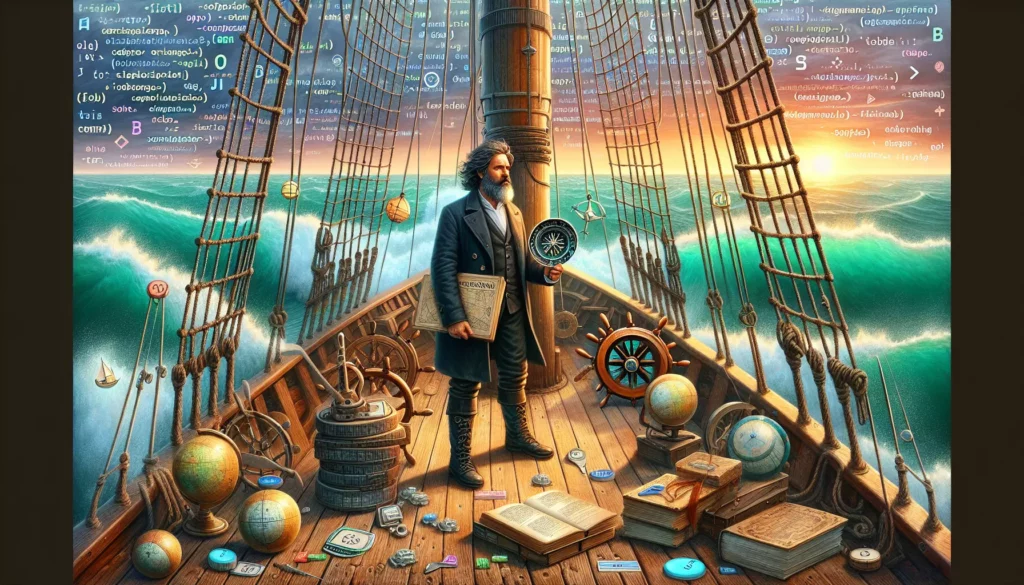
Ahoy, fellow code sailors! Welcome aboard the good ship AlgoCademy, where we’ll embark on a grand voyage through the vast oceans of software development. Just as ancient mariners used the stars to guide their way across uncharted waters, modern programmers can navigate the complex seas of coding using time-tested nautical techniques. In this comprehensive guide, we’ll explore how the principles of seamanship can be applied to the world of programming, helping you chart a course from novice coder to master developer.
1. Charting Your Course: Planning Your Coding Journey
Before any sailor sets out on a voyage, they must first chart their course. The same principle applies to your coding journey. Let’s explore how to map out your path to programming proficiency:
1.1. Determining Your Destination
Just as a ship needs a destination, you need a clear goal for your coding journey. Are you aiming to become a full-stack developer, a data scientist, or perhaps a mobile app creator? Knowing your end goal will help you plot the most efficient route to get there.
1.2. Identifying Waypoints
Sailors use waypoints to break down long journeys into manageable segments. In your coding voyage, these waypoints might include:
- Learning the basics of a programming language
- Mastering data structures and algorithms
- Building your first web application
- Contributing to an open-source project
- Preparing for technical interviews at top tech companies
1.3. Choosing Your Vessel
In nautical terms, your vessel is the programming language and tools you’ll use on your journey. Just as different ships are suited for different waters, various programming languages excel in different domains:
- Python: A versatile language, great for beginners and data science
- JavaScript: Essential for web development
- Java: Popular for enterprise applications and Android development
- C++: Powerful for system programming and game development
Choose your vessel wisely based on your destination and the waters you plan to navigate.
2. Learning the Ropes: Mastering Programming Fundamentals
Every sailor must learn the basics of seamanship before they can navigate treacherous waters. Similarly, mastering programming fundamentals is crucial for any aspiring developer.
2.1. Tying the Knots: Basic Syntax and Data Types
Just as sailors must know various knots for different situations, programmers must understand basic syntax and data types. Let’s look at some examples in Python:
# Variables and basic data types
name = "Captain Code" # String
age = 30 # Integer
height = 6.2 # Float
is_experienced = True # Boolean
# Basic operations
print(f"{name} is {age} years old and {height} feet tall.")
print(f"Experienced: {is_experienced}")
# Lists (similar to arrays in other languages)
crew_members = ["First Mate", "Navigator", "Engineer"]
print(f"Crew: {', '.join(crew_members)}")
# Dictionaries (key-value pairs)
ship_info = {"name": "SS Codebreaker", "type": "Frigate", "year": 2023}
print(f"Ship: {ship_info['name']}, Type: {ship_info['type']}")
2.2. Reading the Compass: Control Flow and Logic
Understanding control flow is like learning to read a compass. It helps you navigate through your code and make decisions based on different conditions:
# If-else statements
wind_speed = 25
if wind_speed < 10:
print("Calm seas ahead")
elif wind_speed < 20:
print("Moderate winds, proceed with caution")
else:
print("Strong winds, batten down the hatches!")
# Loops
for day in range(1, 8):
print(f"Day {day} of our journey")
crew_positions = ["Captain", "First Mate", "Navigator", "Engineer"]
i = 0
while i < len(crew_positions):
print(f"Crew member {i+1}: {crew_positions[i]}")
i += 1
2.3. Maintaining the Logbook: Functions and Modules
In seafaring, the ship’s logbook is crucial for recording important information. In programming, functions and modules serve a similar purpose by organizing and encapsulating code:
def calculate_nautical_miles(kilometers):
return kilometers / 1.852
def log_journey(start_port, end_port, distance_km):
nautical_miles = calculate_nautical_miles(distance_km)
return f"Journey from {start_port} to {end_port}: {nautical_miles:.2f} nautical miles"
# Using the functions
journey_log = log_journey("Port Python", "Harbor JavaScript", 500)
print(journey_log)
# Importing and using modules
import math
def calculate_wind_direction(x, y):
angle = math.atan2(y, x)
return math.degrees(angle) % 360
wind_direction = calculate_wind_direction(3, 4)
print(f"Wind direction: {wind_direction:.2f} degrees")
3. Navigating Rough Seas: Debugging and Problem-Solving
Even the most experienced sailors encounter storms and rough seas. In the world of coding, bugs and complex problems are our stormy waters. Let’s explore some techniques for navigating these challenges.
3.1. Using the Sextant: Debugging Techniques
Just as a sextant helps sailors determine their position, debugging tools and techniques help programmers locate and fix errors in their code.
- Print Debugging: The simplest form of debugging, similar to sending up flares to see where you are.
- Logging: More sophisticated than print debugging, logging helps you keep a record of your program’s behavior over time.
- Interactive Debuggers: These tools allow you to pause your program’s execution and examine its state, like dropping anchor to survey your surroundings.
Let’s look at an example of print debugging in Python:
def navigate_through_archipelago(islands):
route = []
for i, island in enumerate(islands):
print(f"Debugging: Currently at island {i}: {island}") # Debug print
if island['has_treasure']:
route.append(island['name'])
return route
archipelago = [
{'name': 'Python Isle', 'has_treasure': True},
{'name': 'Java Atoll', 'has_treasure': False},
{'name': 'Ruby Reef', 'has_treasure': True},
{'name': 'C++ Cay', 'has_treasure': False}
]
treasure_route = navigate_through_archipelago(archipelago)
print(f"Treasure found on: {treasure_route}")
3.2. Weathering the Storm: Error Handling
Error handling in programming is like preparing your ship for potential storms. It helps your program gracefully handle unexpected situations:
def divide_treasure(total_gold, crew_members):
try:
share = total_gold / crew_members
return f"Each crew member gets {share} gold coins"
except ZeroDivisionError:
return "Error: Cannot divide treasure among zero crew members"
except TypeError:
return "Error: Invalid input types for treasure division"
# Test the function
print(divide_treasure(1000, 5)) # Valid case
print(divide_treasure(1000, 0)) # Handling zero division
print(divide_treasure("lots", "many")) # Handling type error
3.3. Charting Unknown Waters: Problem-Solving Strategies
When faced with complex coding challenges, use these problem-solving strategies:
- Break it down: Divide the problem into smaller, manageable sub-problems.
- Research: Look for existing solutions or similar problems that have been solved.
- Pseudocode: Write out the logic in plain language before coding.
- Test and iterate: Implement a solution, test it, and refine as needed.
Here’s an example of breaking down a problem and using pseudocode:
# Problem: Find the most efficient route through a set of islands
# Pseudocode:
# 1. Create a function to calculate distance between two points
# 2. Generate all possible routes (permutations of islands)
# 3. Calculate the total distance for each route
# 4. Return the route with the shortest total distance
import itertools
import math
def calculate_distance(point1, point2):
return math.sqrt((point1[0] - point2[0])**2 + (point1[1] - point2[1])**2)
def find_shortest_route(islands):
shortest_distance = float('inf')
best_route = None
for route in itertools.permutations(islands):
total_distance = sum(calculate_distance(route[i], route[i+1]) for i in range(len(route)-1))
if total_distance < shortest_distance:
shortest_distance = total_distance
best_route = route
return best_route, shortest_distance
# Example usage
island_coordinates = [
(0, 0), # Starting point
(1, 5), # Island A
(3, 2), # Island B
(5, 3), # Island C
(4, 7) # Island D
]
best_route, distance = find_shortest_route(island_coordinates)
print(f"Best route: {best_route}")
print(f"Total distance: {distance:.2f} units")
4. Building Your Fleet: Object-Oriented Programming
As you progress in your coding journey, you’ll want to build more complex and organized systems. Object-Oriented Programming (OOP) is like building a fleet of specialized ships, each with its own purpose and capabilities.
4.1. Designing the Blueprint: Classes and Objects
In OOP, classes are like blueprints for ships, and objects are the actual ships built from those blueprints. Let’s create a simple ship class:
class Ship:
def __init__(self, name, ship_type, crew_capacity):
self.name = name
self.ship_type = ship_type
self.crew_capacity = crew_capacity
self.current_crew = 0
def add_crew(self, number):
if self.current_crew + number <= self.crew_capacity:
self.current_crew += number
return f"{number} crew members added. Total crew: {self.current_crew}"
else:
return f"Cannot add {number} crew members. Exceeds capacity."
def set_sail(self):
if self.current_crew > 0:
return f"{self.name} is setting sail with {self.current_crew} crew members!"
else:
return f"{self.name} cannot sail without a crew."
# Creating and using ship objects
galleon = Ship("Golden Hind", "Galleon", 100)
frigate = Ship("Sea Wolf", "Frigate", 50)
print(galleon.add_crew(80))
print(frigate.add_crew(40))
print(galleon.set_sail())
print(frigate.set_sail())
4.2. Inheritance: Building Specialized Vessels
Inheritance in OOP is like creating specialized ships based on a general design. Let’s create some specialized ship types:
class CargoShip(Ship):
def __init__(self, name, cargo_capacity):
super().__init__(name, "Cargo", 30)
self.cargo_capacity = cargo_capacity
self.current_cargo = 0
def load_cargo(self, amount):
if self.current_cargo + amount <= self.cargo_capacity:
self.current_cargo += amount
return f"{amount} tons of cargo loaded. Total cargo: {self.current_cargo} tons"
else:
return f"Cannot load {amount} tons. Exceeds capacity."
class Submarine(Ship):
def __init__(self, name):
super().__init__(name, "Submarine", 50)
self.submerged = False
def submerge(self):
self.submerged = True
return f"{self.name} is submerging"
def surface(self):
self.submerged = False
return f"{self.name} is surfacing"
# Using the specialized ships
cargo_ship = CargoShip("Heavy Lifter", 1000)
submarine = Submarine("Silent Hunter")
print(cargo_ship.add_crew(25))
print(cargo_ship.load_cargo(800))
print(submarine.add_crew(45))
print(submarine.submerge())
print(submarine.set_sail())
4.3. Polymorphism: Adapting to Different Seas
Polymorphism allows objects of different classes to be treated as objects of a common base class. This is like having different types of ships that can all navigate, but in their own specialized ways:
def embark_on_mission(ship):
print(f"Preparing {ship.name} for the mission:")
print(ship.add_crew(ship.crew_capacity))
print(ship.set_sail())
if isinstance(ship, CargoShip):
print(ship.load_cargo(ship.cargo_capacity))
elif isinstance(ship, Submarine):
print(ship.submerge())
# Embarking on missions with different ship types
embark_on_mission(galleon)
print("\n")
embark_on_mission(cargo_ship)
print("\n")
embark_on_mission(submarine)
5. Exploring New Horizons: Advanced Topics and Techniques
As you become more proficient in coding, you’ll want to explore advanced topics and techniques. These are like discovering new trade routes or advanced navigation methods in the world of sailing.
5.1. Asynchronous Programming: Multitasking at Sea
Asynchronous programming is like managing multiple tasks on a ship simultaneously. It allows your program to perform other operations while waiting for time-consuming tasks to complete. Here’s a simple example using Python’s asyncio:
import asyncio
async def scan_horizon(duration):
print(f"Scanning the horizon for {duration} seconds...")
await asyncio.sleep(duration)
print("Scan complete!")
async def check_supplies(duration):
print(f"Checking supplies, will take {duration} seconds...")
await asyncio.sleep(duration)
print("Supply check complete!")
async def main():
task1 = asyncio.create_task(scan_horizon(3))
task2 = asyncio.create_task(check_supplies(2))
print("Starting ship operations...")
await task1
await task2
print("All operations complete!")
asyncio.run(main())
5.2. Design Patterns: Tried and True Sailing Techniques
Design patterns in programming are like well-established sailing techniques. They provide tested solutions to common problems. Let’s look at the Observer pattern, which can be used to notify multiple objects about changes in another object:
class WeatherStation:
def __init__(self):
self._observers = []
self._temperature = 0
def add_observer(self, observer):
self._observers.append(observer)
def remove_observer(self, observer):
self._observers.remove(observer)
def notify_observers(self):
for observer in self._observers:
observer.update(self._temperature)
def set_temperature(self, temp):
self._temperature = temp
self.notify_observers()
class Ship:
def __init__(self, name):
self.name = name
def update(self, temperature):
if temperature > 30:
print(f"{self.name}: It's getting hot! Temperature is {temperature}°C")
elif temperature < 10:
print(f"{self.name}: It's getting cold! Temperature is {temperature}°C")
else:
print(f"{self.name}: Weather is pleasant. Temperature is {temperature}°C")
# Using the Observer pattern
weather_station = WeatherStation()
ship1 = Ship("Voyager")
ship2 = Ship("Explorer")
weather_station.add_observer(ship1)
weather_station.add_observer(ship2)
weather_station.set_temperature(25)
weather_station.set_temperature(32)
weather_station.set_temperature(8)
5.3. Data Structures and Algorithms: Navigating Efficiently
Understanding data structures and algorithms is crucial for efficient programming, just as knowing various navigation techniques is essential for efficient sailing. Let’s implement a simple pathfinding algorithm to find the shortest route between islands:
from collections import deque
def shortest_path(graph, start, end):
queue = deque([[start]])
visited = set([start])
while queue:
path = queue.popleft()
island = path[-1]
if island == end:
return path
for neighbor in graph[island]:
if neighbor not in visited:
visited.add(neighbor)
new_path = list(path)
new_path.append(neighbor)
queue.append(new_path)
return None
# Example island network
island_network = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
start_island = 'A'
end_island = 'F'
path = shortest_path(island_network, start_island, end_island)
if path:
print(f"Shortest route from {start_island} to {end_island}: {' -> '.join(path)}")
else:
print(f"No route found from {start_island} to {end_island}")
6. Docking at Port FAANG: Preparing for Tech Interviews
As you near the end of your coding journey, you might set your sights on the prestigious ports of FAANG (Facebook, Amazon, Apple, Netflix, Google) or other major tech companies. Preparing for these technical interviews is like preparing for a challenging sea trial.
6.1. Strengthening Your Ship: Mastering Core CS Concepts
To succeed in tech interviews, you need to have a strong grasp of core computer science concepts. This includes:
- Data Structures (Arrays, Linked Lists, Trees, Graphs, Hash Tables)
- Algorithms (Sorting, Searching, Dynamic Programming)
- Time and Space Complexity Analysis
- Object-Oriented Programming Principles
- System Design Basics
6.2. Mock Sea Trials: Practice Interviews
Practice makes perfect. Engage in mock interviews and coding challenges to prepare yourself. Platforms like AlgoCademy provide excellent resources for this. Here’s an example of a common interview question:
def is_balanced(s):
stack = []
opening = set('({[')
closing = set(')}]')
pair = {')': '(', '}': '{', ']': '['}
for char in s:
if char in opening:
stack.append(char)
elif char in closing:
if not stack or stack[-1] != pair[char]:
return False
stack.pop()
return len(stack) == 0
# Test cases
print(is_balanced("({[]})")) # True
print(is_balanced("([)]")) # False
print(is_balanced("((")) # False
6.3. Navigating the Interview: Problem-Solving Strategies
During the interview, use these strategies to navigate through tough questions:
- Clarify the problem and requirements
- Think out loud and communicate your thought process
- Start with a brute force solution, then optimize
- Consider edge cases and test your solution
- Analyze the time and space complexity of your solution
Conclusion: Your Ongoing Voyage
Remember, your coding journey doesn’t end when you reach a certain destination. Like a true sailor, a programmer’s voyage is lifelong, with always more to learn and explore. The seas of technology are ever-changing, with new languages, frameworks, and paradigms emerging regularly.
As you continue your journey, keep these nautical coding principles in mind:
- Always be learning: The best sailors and coders never stop improving their skills.
- Collaborate with your crew: Programming, like sailing, is often a team effort. Learn to work effectively with others.
- Navigate carefully: Plan your projects and career moves thoughtfully, just as you would plan a sea voyage.
- Be prepared for storms: Challenges and setbacks are part of the journey. Resilience and problem-solving skills will see you through.
- Enjoy the journey: Remember to take joy in the process of coding and creating, not just in reaching your destination.
With AlgoCademy as your trusted navigation tool, you’re well-equipped to sail the software seas. Whether you’re just starting out or preparing to dock at the ports of major tech companies, remember that every great programmer started as a novice coder. Your skills will grow with each line of code you write, each problem you solve, and each project you complete.
So hoist the mainsail, plot your course, and set forth on your coding adventure. The vast oceans of software development await, filled with challenges, discoveries, and endless possibilities. Fair winds and following seas, fellow code sailor!