The LeetCode Trap: Why Copying Solutions Won’t Make You a Better Problem Solver
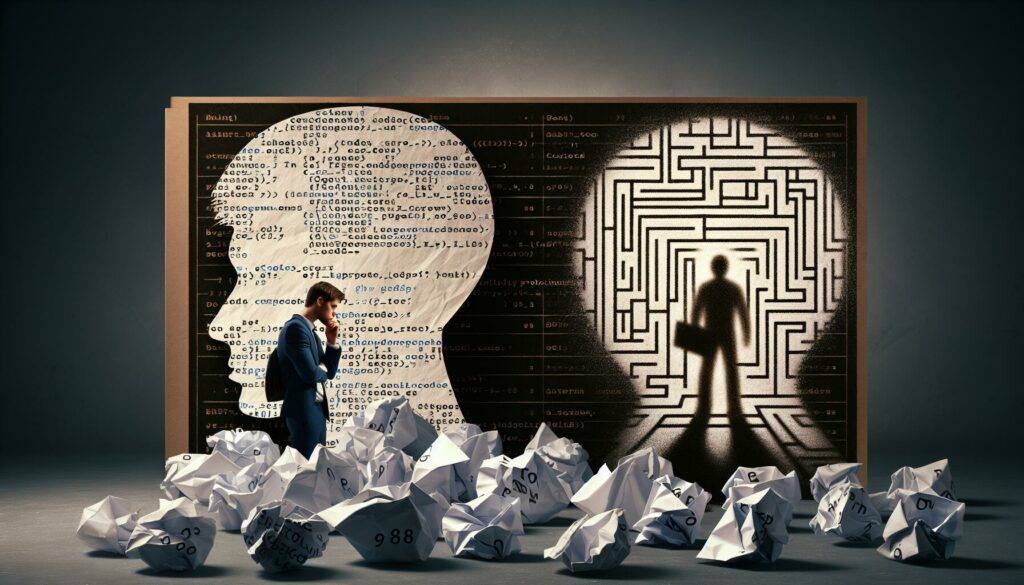
In the world of coding education and technical interview preparation, LeetCode has become a household name. Aspiring software engineers and seasoned developers alike flock to this platform to hone their algorithmic skills and prepare for the grueling technical interviews at top tech companies. However, there’s a dangerous trap that many fall into when using LeetCode: the temptation to simply copy and paste solutions without truly understanding the underlying concepts. In this comprehensive guide, we’ll explore why this approach is detrimental to your growth as a problem solver and offer strategies to avoid the LeetCode trap.
The Allure of Quick Fixes
It’s easy to see why copying solutions from LeetCode is so tempting. When faced with a difficult problem, the path of least resistance is to look up the answer and move on to the next challenge. This approach offers several perceived benefits:
- Instant gratification of seeing a “Solved” status
- Rapid progress through problem sets
- Exposure to optimal solutions
- Time-saving in a busy schedule
However, these short-term gains come at a significant cost to your long-term development as a programmer and problem solver.
The Hidden Costs of Copying Solutions
1. Stunted Problem-Solving Skills
The most significant drawback of copying solutions is that it prevents you from developing crucial problem-solving skills. When you encounter a challenging problem in a real-world scenario or during an actual interview, you won’t have the luxury of looking up the answer. By relying on pre-written solutions, you’re robbing yourself of the opportunity to:
- Analyze the problem thoroughly
- Break down complex issues into manageable components
- Develop your own algorithmic thinking
- Practice implementing solutions from scratch
2. Shallow Understanding
Copying a solution may give you the illusion of understanding, but it often leads to a superficial grasp of the concepts involved. You might be able to recite the steps of a particular algorithm, but without the struggle of deriving it yourself, you’re less likely to:
- Understand why certain approaches work better than others
- Recognize patterns that can be applied to similar problems
- Adapt solutions to slightly different scenarios
- Explain your reasoning clearly to others (a crucial skill in technical interviews)
3. Missed Learning Opportunities
The process of struggling with a problem, making mistakes, and iteratively improving your solution is where deep learning occurs. By skipping this process, you miss out on:
- Discovering alternative approaches you might not have considered
- Learning from your mistakes and misconceptions
- Developing debugging skills as you work through errors
- Building confidence in your ability to solve problems independently
4. False Sense of Progress
Rapidly completing LeetCode problems by copying solutions can give you a false sense of progress. You might feel like you’re advancing quickly, but in reality, you’re building a house of cards that’s likely to collapse under the pressure of a real interview or complex project.
The Right Way to Use LeetCode
Now that we’ve established why copying solutions is counterproductive, let’s explore how to use LeetCode effectively to become a better problem solver:
1. Attempt Problems Independently
Always start by trying to solve the problem on your own. Set a reasonable time limit (e.g., 30-45 minutes for medium difficulty problems) and give it your best shot. This approach allows you to:
- Practice problem analysis and solution design
- Identify gaps in your knowledge or skills
- Build confidence in your ability to tackle unknown problems
2. Use Hints Judiciously
If you’re stuck, it’s okay to look at hints or discuss the problem with others. However, try to limit yourself to small nudges rather than full solutions. Good resources for hints include:
- LeetCode’s built-in hint system
- Discussion forums (but avoid looking at complete code)
- General algorithm and data structure resources
3. Implement Your Own Solution
Even if you needed hints, always try to implement the solution yourself. This process helps solidify your understanding and improves your coding skills. Remember:
- Start with a brute force approach if necessary
- Gradually optimize your solution
- Focus on writing clean, readable code
4. Study Multiple Solutions
After successfully solving a problem (or giving it your best attempt), study various solutions to gain deeper insights:
- Compare your solution to others in terms of time and space complexity
- Analyze different approaches and understand their trade-offs
- Look for clever tricks or optimizations you might have missed
5. Revisit Problems
Spaced repetition is a powerful learning technique. Revisit problems you’ve solved after a few days or weeks to:
- Reinforce your understanding
- Identify areas where your recall or implementation skills have improved
- Challenge yourself to come up with alternative solutions
Building a Solid Foundation
To truly benefit from LeetCode and avoid the temptation to copy solutions, it’s crucial to build a strong foundation in computer science fundamentals. This includes:
1. Data Structures
A deep understanding of data structures is essential for efficient problem-solving. Focus on mastering:
- Arrays and strings
- Linked lists
- Stacks and queues
- Trees and graphs
- Hash tables
- Heaps
For each data structure, make sure you understand:
- Basic operations and their time complexities
- Common use cases and applications
- Implementation details in your preferred programming language
2. Algorithms
Familiarize yourself with fundamental algorithms and problem-solving techniques:
- Sorting and searching algorithms
- Dynamic programming
- Greedy algorithms
- Divide and conquer
- Backtracking
- Graph traversal (BFS, DFS)
For each algorithm, practice:
- Implementing it from scratch
- Analyzing its time and space complexity
- Identifying problems where it can be applied
3. Time and Space Complexity Analysis
Developing a strong intuition for algorithmic complexity is crucial for optimizing your solutions:
- Learn Big O notation and how to calculate it
- Practice identifying the time and space complexity of your code
- Understand the trade-offs between time and space efficiency
4. Problem-Solving Strategies
Develop a systematic approach to tackling coding problems:
- Read and understand the problem statement thoroughly
- Identify the inputs, outputs, and constraints
- Break down the problem into smaller subproblems
- Consider multiple approaches before coding
- Start with a simple solution and optimize incrementally
Leveraging Additional Resources
While LeetCode is an excellent platform for practice, it shouldn’t be your only resource. To become a well-rounded problem solver, consider incorporating the following into your learning journey:
1. Coding Books and Courses
Supplement your practice with structured learning materials:
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein
- Online courses from platforms like Coursera, edX, or Udacity
2. Interactive Coding Platforms
Explore other platforms that offer interactive coding experiences:
- HackerRank for a wide range of programming challenges
- CodeSignal for gamified coding practice
- Project Euler for mathematics-oriented programming problems
3. Open Source Projects
Contributing to open source projects can provide real-world problem-solving experience:
- Find beginner-friendly projects on GitHub
- Participate in coding challenges like Hacktoberfest
- Collaborate with other developers and learn from code reviews
4. Coding Competitions
Participate in coding competitions to challenge yourself and learn from others:
- Google Code Jam
- Facebook Hacker Cup
- TopCoder
- Codeforces
Developing a Growth Mindset
Avoiding the LeetCode trap and becoming a better problem solver requires more than just technical skills. It’s essential to cultivate a growth mindset:
1. Embrace Challenges
View difficult problems as opportunities for growth rather than obstacles:
- Celebrate the process of learning, not just the outcome
- Set realistic goals and track your progress over time
- Don’t be discouraged by initial failures or slow progress
2. Practice Regularly
Consistent practice is key to improvement:
- Set aside dedicated time for problem-solving practice
- Aim for a mix of easy, medium, and hard problems
- Focus on quality of learning rather than quantity of problems solved
3. Reflect on Your Learning
Take time to reflect on your problem-solving experiences:
- Keep a coding journal to document your thought processes
- Analyze your mistakes and learn from them
- Identify patterns in the types of problems you find challenging
4. Seek Feedback
Don’t hesitate to ask for help and feedback:
- Participate in coding communities and forums
- Find a coding buddy or mentor
- Share your solutions and ask for constructive criticism
Putting It All Together: A Sample Problem-Solving Approach
Let’s walk through an example of how to approach a LeetCode problem without falling into the trap of copying solutions. We’ll use the classic “Two Sum” problem:
Given an array of integers
nums
and an integertarget
, return indices of the two numbers such that they add up totarget
. You may assume that each input would have exactly one solution, and you may not use the same element twice.
Step 1: Understand the Problem
- Input: An array of integers and a target sum
- Output: Indices of two numbers that add up to the target
- Constraints: Each input has exactly one solution, can’t use the same element twice
Step 2: Consider Multiple Approaches
- Brute force: Check all pairs of numbers (O(n^2) time complexity)
- Sorting and two-pointer technique (O(n log n) time complexity)
- Hash table for complement lookup (O(n) time complexity)
Step 3: Implement a Solution
Let’s implement the hash table approach:
class Solution:
def twoSum(self, nums: List[int], target: int) -> List[int]:
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return [] # No solution found
Step 4: Analyze the Solution
- Time complexity: O(n) – we iterate through the array once
- Space complexity: O(n) – in the worst case, we store all numbers in the hash table
- This solution is optimal in terms of time complexity
Step 5: Consider Edge Cases and Optimizations
- What if the input array is empty?
- Can we handle duplicate numbers in the array?
- Is there a way to reduce space usage if memory is a constraint?
Step 6: Reflect and Learn
- What key insight made this solution efficient?
- How can we apply the hash table technique to similar problems?
- What other problems might benefit from this approach?
Conclusion: Embracing the Journey of Problem-Solving
The LeetCode trap of copying solutions is alluring, but it’s a shortcut that ultimately leads to a dead end in your development as a programmer and problem solver. By embracing the challenges, building a solid foundation, and approaching problems methodically, you’ll not only perform better in technical interviews but also become a more capable and confident software engineer.
Remember that becoming a great problem solver is a journey, not a destination. It requires patience, persistence, and a willingness to learn from both successes and failures. As you continue to practice and refine your skills, you’ll find that the satisfaction of solving a difficult problem on your own far outweighs the temporary relief of copying a solution.
So the next time you’re tempted to look up the answer to a LeetCode problem, pause and ask yourself: “What can I learn by struggling with this a bit longer?” Your future self will thank you for the investment in your problem-solving abilities, and you’ll be well-prepared to tackle whatever challenges come your way in your programming career.