The Journey of Learning Algorithms: Tips and Resources
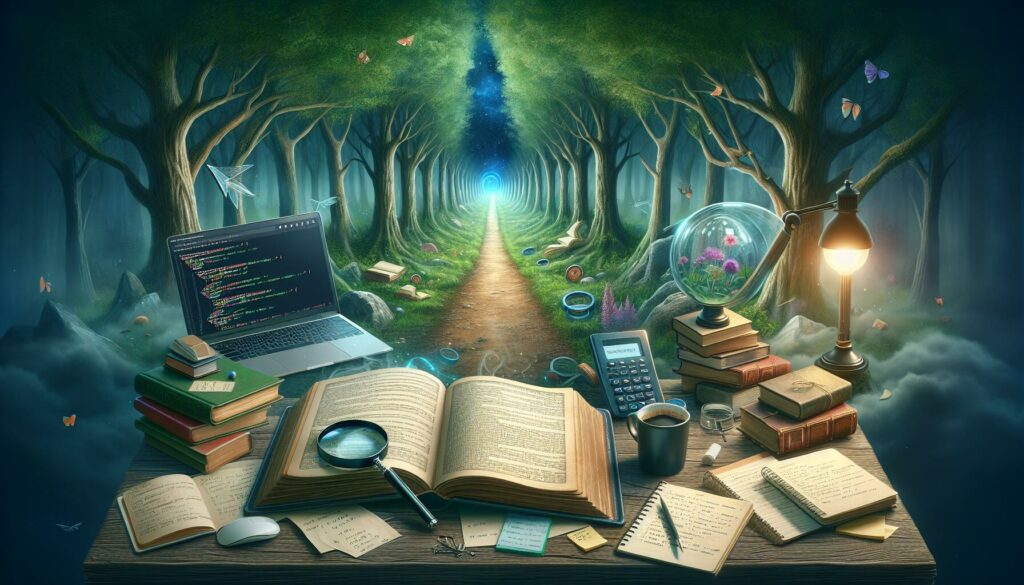
In the ever-evolving world of technology, mastering algorithms has become an essential skill for programmers and aspiring software engineers. Whether you’re a beginner taking your first steps into coding or an experienced developer aiming to crack those challenging technical interviews at top tech companies, understanding algorithms is crucial. This comprehensive guide will take you through the journey of learning algorithms, providing valuable tips, resources, and insights to help you excel in your coding education and career.
Why Learn Algorithms?
Before diving into the how-to’s, let’s address the why. Algorithms are the building blocks of efficient problem-solving in computer science. They provide structured approaches to tackle complex issues and optimize solutions. Here are some key reasons to invest time in learning algorithms:
- Problem-solving skills: Algorithms teach you how to break down complex problems into manageable steps.
- Efficiency: Understanding algorithms helps you write more efficient code, saving time and resources.
- Technical interviews: Many top tech companies, especially FAANG (Facebook, Amazon, Apple, Netflix, Google), heavily emphasize algorithmic knowledge in their interview process.
- Career advancement: Proficiency in algorithms can open doors to higher-level positions and more challenging projects.
- Foundational knowledge: Algorithms form the basis for understanding more advanced concepts in computer science.
Getting Started with Algorithms
Embarking on your algorithm learning journey can seem daunting, but with the right approach and resources, it can be an exciting and rewarding experience. Here’s how to get started:
1. Master the Basics of Programming
Before diving deep into algorithms, ensure you have a solid foundation in at least one programming language. Python is often recommended for beginners due to its readability and extensive libraries, but languages like Java, C++, or JavaScript are also excellent choices. Familiarize yourself with basic programming concepts such as variables, data types, control structures, and functions.
2. Understand Data Structures
Data structures are fundamental to algorithms. Start by learning about arrays, linked lists, stacks, queues, trees, and graphs. Understanding how these structures work and when to use them will significantly aid your algorithm learning process.
3. Start with Simple Algorithms
Begin with basic sorting and searching algorithms like bubble sort, selection sort, insertion sort, linear search, and binary search. Implement these algorithms yourself to get a hands-on understanding of how they work.
4. Gradually Increase Complexity
As you become comfortable with basic algorithms, move on to more complex ones. Explore divide-and-conquer algorithms, dynamic programming, greedy algorithms, and graph algorithms. Each category will introduce you to new problem-solving techniques.
5. Practice, Practice, Practice
Consistent practice is key to mastering algorithms. Solve coding challenges regularly on platforms like LeetCode, HackerRank, or CodeForces. Start with easier problems and gradually tackle more difficult ones as your skills improve.
Essential Algorithm Concepts to Learn
As you progress in your algorithm journey, focus on mastering these key concepts:
1. Time and Space Complexity
Understanding Big O notation is crucial for analyzing the efficiency of algorithms. Learn to evaluate and optimize the time and space complexity of your solutions.
2. Recursion
Many algorithms use recursive approaches. Practice solving problems using recursion to build your problem-solving skills.
3. Sorting Algorithms
Beyond basic sorting algorithms, learn more efficient ones like Merge Sort, Quick Sort, and Heap Sort. Understand their implementations and use cases.
4. Searching Algorithms
Master various searching techniques including binary search, depth-first search (DFS), and breadth-first search (BFS).
5. Dynamic Programming
This powerful technique is often used in optimization problems. Start with simple dynamic programming problems and gradually tackle more complex ones.
6. Graph Algorithms
Learn algorithms for graph traversal, shortest path problems (like Dijkstra’s algorithm), and minimum spanning trees (like Kruskal’s algorithm).
Tips for Effective Algorithm Learning
To make your learning journey more efficient and enjoyable, consider these tips:
1. Understand Before Memorizing
Focus on understanding the logic and intuition behind algorithms rather than memorizing their implementations. This approach will help you apply algorithmic thinking to new problems.
2. Visualize Algorithms
Use visualization tools or draw diagrams to understand how algorithms work. Websites like VisuAlgo offer interactive visualizations of various algorithms and data structures.
3. Implement from Scratch
Don’t just read about algorithms; implement them yourself. This hands-on approach will deepen your understanding and improve your coding skills.
4. Solve Real-World Problems
Apply the algorithms you learn to solve real-world problems. This practice will help you understand their practical applications and limitations.
5. Participate in Coding Competitions
Join online coding competitions or hackathons. These events can be great motivators and provide opportunities to apply your skills in time-constrained environments.
6. Collaborate and Discuss
Join coding communities, participate in forums, or find a study group. Discussing problems and solutions with others can provide new perspectives and deepen your understanding.
Resources for Learning Algorithms
There are numerous resources available for learning algorithms. Here are some top recommendations:
1. Online Courses
- Coursera: “Algorithms Specialization” by Stanford University
- edX: “Algorithms and Data Structures” by MIT
- Udacity: “Intro to Algorithms” course
2. Books
- “Introduction to Algorithms” by Thomas H. Cormen, Charles E. Leiserson, Ronald L. Rivest, and Clifford Stein
- “Algorithms” by Robert Sedgewick and Kevin Wayne
- “Grokking Algorithms” by Aditya Bhargava (great for beginners)
3. Websites and Platforms
- LeetCode: Offers a vast collection of coding challenges with a focus on algorithms
- HackerRank: Provides algorithm challenges and contests
- GeeksforGeeks: Comprehensive resource for algorithm tutorials and practice problems
- AlgoExpert: Platform designed specifically for algorithm preparation
4. YouTube Channels
- MIT OpenCourseWare
- mycodeschool
- Back To Back SWE
Preparing for Technical Interviews
As you progress in your algorithm learning journey, you might set your sights on technical interviews at top tech companies. Here are some tips to prepare:
1. Focus on Core Data Structures and Algorithms
Ensure you have a strong grasp of fundamental data structures (arrays, linked lists, trees, graphs) and algorithms (sorting, searching, dynamic programming). These form the basis of many interview questions.
2. Practice Coding on a Whiteboard
Many technical interviews involve coding on a whiteboard or in a simple text editor. Practice solving problems without the aid of an IDE to simulate interview conditions.
3. Learn to Communicate Your Thought Process
In interviews, the process of arriving at a solution is often as important as the solution itself. Practice explaining your thought process out loud as you solve problems.
4. Time Your Problem-Solving
Technical interviews often have time constraints. Practice solving problems within specific time limits to improve your speed and efficiency.
5. Mock Interviews
Conduct mock interviews with friends or use platforms like Pramp that offer peer-to-peer mock interviews. This will help you get comfortable with the interview format and receive feedback.
6. Review Company-Specific Information
Research the types of questions typically asked by the companies you’re interested in. Each company may have a slightly different focus in their technical interviews.
Advanced Topics in Algorithms
Once you’ve mastered the fundamentals, consider exploring these advanced topics:
1. Advanced Data Structures
Learn about more complex data structures like segment trees, Fenwick trees, and disjoint set unions. These can be crucial for solving advanced algorithmic problems.
2. String Algorithms
Explore algorithms specific to string manipulation, such as KMP algorithm, Rabin-Karp algorithm, and suffix arrays.
3. Network Flow Algorithms
Study algorithms like Ford-Fulkerson and Edmonds-Karp for solving network flow problems.
4. Computational Geometry
Learn algorithms for geometric problems, including convex hull algorithms and line intersection algorithms.
5. Approximation Algorithms
Understand how to approach NP-hard problems with approximation algorithms.
The Role of AI in Learning Algorithms
Artificial Intelligence is revolutionizing the way we learn and practice algorithms. AI-powered platforms can provide personalized learning experiences, offer intelligent hints, and even generate practice problems tailored to your skill level. Here’s how AI can enhance your algorithm learning journey:
1. Personalized Learning Paths
AI algorithms can analyze your performance and create customized learning paths, focusing on areas where you need improvement.
2. Intelligent Problem Recommendations
Based on your solving patterns and progress, AI can recommend problems that are most suitable for your current skill level and learning goals.
3. Real-time Feedback and Hints
AI-powered coding platforms can provide instant feedback on your code and offer intelligent hints when you’re stuck, mimicking a human tutor.
4. Code Analysis and Optimization Suggestions
Advanced AI tools can analyze your code for efficiency and suggest optimizations, helping you learn best practices and improve your algorithmic thinking.
5. Natural Language Processing for Problem Understanding
AI with natural language processing capabilities can help break down complex problem statements, making it easier to understand and approach challenging algorithms.
Maintaining Motivation and Overcoming Challenges
Learning algorithms can be a challenging journey with its ups and downs. Here are some strategies to stay motivated and overcome common hurdles:
1. Set Realistic Goals
Break your learning journey into smaller, achievable goals. Celebrate each milestone to maintain motivation.
2. Join a Community
Engage with like-minded learners through online forums, local meetups, or study groups. Sharing experiences and challenges can be incredibly motivating.
3. Track Your Progress
Keep a log of the problems you’ve solved and the concepts you’ve mastered. Reviewing your progress can be a great motivator during challenging times.
4. Take Breaks and Avoid Burnout
Learning algorithms can be intense. Remember to take regular breaks and engage in other activities to avoid burnout.
5. Apply What You Learn
Try to apply the algorithms you learn to real-world problems or personal projects. Seeing practical applications can reignite your interest and motivation.
6. Embrace the Challenge
Remember that struggling with difficult concepts is a natural part of the learning process. Embrace these challenges as opportunities for growth.
Conclusion
The journey of learning algorithms is a rewarding adventure that opens up a world of possibilities in programming and problem-solving. From mastering the basics to tackling advanced topics, each step builds your skills and prepares you for exciting opportunities in the tech industry.
Remember, the key to success lies in consistent practice, curiosity, and perseverance. Utilize the wealth of resources available, leverage AI-powered learning tools, and don’t hesitate to seek help when needed. Whether you’re aiming to ace technical interviews at top tech companies or simply want to become a better programmer, a strong foundation in algorithms will serve you well throughout your career.
As you embark on or continue this journey, keep in mind that every expert was once a beginner. Stay patient, stay curious, and most importantly, enjoy the process of learning and growing. The world of algorithms is vast and ever-evolving, offering endless opportunities for those willing to explore and master it.
Happy coding, and may your algorithm journey be filled with exciting discoveries and satisfying problem-solving adventures!