The Importance of Version Control in Software Development
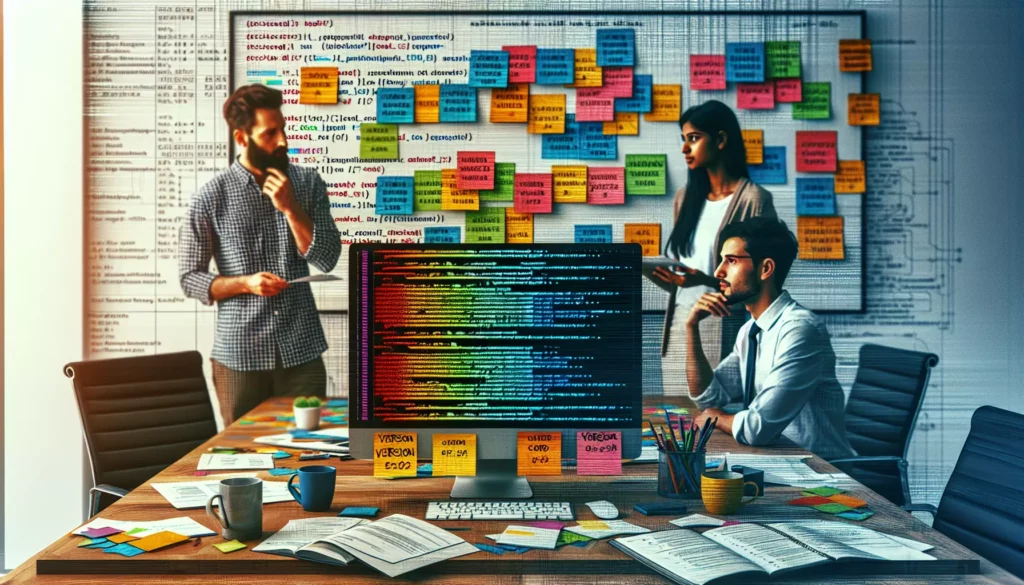
In the fast-paced world of software development, managing code changes, collaborating with team members, and maintaining a reliable history of project evolution are crucial aspects of successful development practices. This is where version control systems come into play, serving as an indispensable tool for developers of all levels. In this comprehensive guide, we’ll explore the importance of version control in software development, its benefits, popular tools, and best practices for implementation.
What is Version Control?
Version control, also known as source control or revision control, is a system that helps track and manage changes to files over time. It allows multiple developers to work on the same project simultaneously, keeping a record of modifications, and providing the ability to revert to previous versions if needed. Version control systems (VCS) are particularly vital in software development, where code bases can become complex and involve numerous contributors.
The Benefits of Version Control
1. Collaboration and Team Productivity
One of the primary advantages of version control is its ability to facilitate collaboration among team members. With a VCS in place, multiple developers can work on the same project concurrently, without fear of overwriting each other’s changes. This leads to increased productivity and smoother teamwork.
2. Code History and Traceability
Version control systems maintain a detailed history of all changes made to the codebase. This allows developers to track the evolution of the project, understand why certain decisions were made, and identify when and by whom specific changes were introduced. This traceability is invaluable for debugging, code reviews, and maintaining code quality.
3. Backup and Recovery
By using a VCS, developers can create backups of their code at various stages of development. In case of data loss or system failure, it’s possible to recover previous versions of the code quickly. This feature provides a safety net and peace of mind for development teams.
4. Branching and Experimentation
Version control systems allow developers to create branches, which are separate lines of development. This feature enables experimentation with new features or bug fixes without affecting the main codebase. Branches can be merged back into the main project once the changes are tested and approved.
5. Code Review and Quality Assurance
Many version control systems integrate with code review tools, making it easier for team members to review each other’s code before it’s merged into the main project. This process helps maintain code quality, catch bugs early, and ensure adherence to coding standards.
Popular Version Control Systems
1. Git
Git is arguably the most popular version control system in use today. Created by Linus Torvalds in 2005, Git is a distributed version control system that offers speed, data integrity, and support for distributed, non-linear workflows. Its popularity has led to the creation of hosting platforms like GitHub, GitLab, and Bitbucket.
Key Features of Git:
- Distributed architecture
- Branching and merging
- Small and fast
- Data integrity
- Staging area
2. Subversion (SVN)
Apache Subversion, often abbreviated as SVN, is a centralized version control system. While not as popular as Git, it still has a significant user base, especially in enterprise environments.
Key Features of SVN:
- Centralized architecture
- Directory versioning
- Atomic commits
- Efficient handling of binary files
3. Mercurial
Mercurial is another distributed version control system, similar to Git. It’s known for its simplicity and ease of use, making it a popular choice for some development teams.
Key Features of Mercurial:
- Distributed architecture
- Easy to learn and use
- Efficient handling of large repositories
- Built-in web interface
Implementing Version Control in Your Development Workflow
Now that we understand the importance and benefits of version control, let’s look at how to implement it effectively in your development workflow.
1. Choose the Right VCS
Select a version control system that best fits your team’s needs and workflow. Consider factors such as learning curve, integration with existing tools, and scalability. For most teams, Git is an excellent choice due to its widespread adoption and robust feature set.
2. Set Up a Repository
Create a central repository to store your project’s code. This can be hosted on platforms like GitHub, GitLab, or Bitbucket, or on your own servers. Here’s an example of how to initialize a Git repository:
$ mkdir my-project
$ cd my-project
$ git init
$ git add .
$ git commit -m "Initial commit"
3. Establish Branching Strategies
Develop a branching strategy that works for your team. A popular approach is the Git Flow workflow, which uses separate branches for features, releases, and hotfixes. Here’s a simple example of creating and switching to a new feature branch:
$ git checkout -b feature/new-login-page
# Make changes and commit
$ git push origin feature/new-login-page
4. Commit Regularly
Make small, frequent commits that represent logical units of work. This practice makes it easier to track changes, review code, and revert if necessary. Use clear and descriptive commit messages to explain the purpose of each change.
$ git add login.html
$ git commit -m "Add responsive design to login page"
5. Use Pull Requests
Implement a pull request (PR) process for code reviews. This allows team members to discuss changes, suggest improvements, and ensure code quality before merging into the main branch.
6. Integrate with CI/CD
Integrate your version control system with Continuous Integration and Continuous Deployment (CI/CD) tools. This automation can help catch bugs early and streamline the deployment process.
Best Practices for Version Control
1. Write Clear Commit Messages
Use descriptive, concise commit messages that explain the purpose of the change. A good commit message should complete the sentence “If applied, this commit will…”
2. Keep Repositories Clean
Use .gitignore files to exclude unnecessary files (like build artifacts or IDE-specific files) from version control. This keeps your repository clean and focused on source code.
3. Use Meaningful Branch Names
Choose clear, descriptive names for your branches that indicate their purpose. For example, “feature/user-authentication” or “bugfix/login-error”.
4. Regularly Pull and Push Changes
Frequently pull changes from the remote repository to stay up-to-date with your team’s work. Similarly, push your changes regularly to share your progress and back up your work.
5. Resolve Conflicts Promptly
When conflicts arise during merges or rebases, address them promptly. Communicate with team members involved to ensure the resolution is correct and doesn’t introduce new issues.
6. Use Tags for Releases
Use tags to mark important points in your project’s history, such as release versions. This makes it easy to reference and revert to specific states of your project.
$ git tag -a v1.0 -m "Version 1.0 release"
$ git push origin v1.0
Advanced Version Control Concepts
1. Rebasing
Rebasing is an alternative to merging that can create a cleaner project history. It involves moving or combining a sequence of commits to a new base commit. Here’s an example of rebasing a feature branch onto the main branch:
$ git checkout feature-branch
$ git rebase main
2. Cherry-picking
Cherry-picking allows you to apply specific commits from one branch to another. This can be useful when you want to incorporate a particular change without merging entire branches:
$ git cherry-pick <commit-hash>
3. Interactive Rebasing
Interactive rebasing gives you fine-grained control over your commit history, allowing you to reorder, edit, or squash commits:
$ git rebase -i HEAD~3
4. Git Hooks
Git hooks are scripts that Git executes before or after events such as commit, push, and receive. They can be used to enforce coding standards, run tests, or trigger notifications:
#!/bin/sh
# .git/hooks/pre-commit
npm run lint
if [ $? -ne 0 ]; then
echo "Linting failed. Please fix errors before committing."
exit 1
fi
Version Control in the Context of Coding Education
For platforms like AlgoCademy that focus on coding education and preparing for technical interviews, understanding version control is crucial. Here’s why:
1. Industry Standard Practice
Version control is ubiquitous in professional software development. Familiarity with VCS is often expected in technical interviews and is essential for working on real-world projects.
2. Portfolio Building
Using version control systems like Git allows learners to showcase their projects and contributions on platforms like GitHub, which can serve as a portfolio for potential employers.
3. Collaboration Skills
Learning to use version control effectively develops crucial collaboration skills that are highly valued in the tech industry. It prepares students for working in team environments.
4. Problem-Solving and Debugging
Version control systems provide powerful tools for tracking down bugs and understanding code evolution, which aligns with the problem-solving focus of platforms like AlgoCademy.
5. Code Review Practice
The pull request process in Git-based workflows provides excellent practice for code reviews, a common activity in technical interviews and professional development.
Integrating Version Control into Coding Education
For educational platforms like AlgoCademy, integrating version control into the curriculum can greatly enhance the learning experience:
1. Version Control Tutorials
Provide dedicated tutorials on version control basics, focusing on Git as the most widely used system. Cover topics like creating repositories, making commits, branching, and merging.
2. Interactive Exercises
Develop interactive exercises that simulate real-world version control scenarios. For example, resolving merge conflicts or reviewing pull requests.
3. Project-Based Learning
Incorporate version control into project-based assignments, requiring students to use Git for managing their code and collaborating with peers.
4. GitHub Integration
Integrate with GitHub or similar platforms to allow students to push their projects and assignments directly from the learning environment.
5. Code Review Modules
Create modules focused on code review best practices, utilizing pull requests and diff views to teach students how to give and receive constructive feedback on code.
Conclusion
Version control is an indispensable tool in modern software development. Its importance extends beyond mere code management; it fosters collaboration, ensures code quality, and provides a safety net for experimentation and growth. For aspiring developers and those preparing for technical interviews, mastering version control is a crucial step towards professional success.
By implementing version control systems like Git and following best practices, development teams can streamline their workflows, improve code quality, and build more robust software products. For educational platforms like AlgoCademy, integrating version control into the curriculum prepares students for real-world development scenarios and enhances their problem-solving and collaboration skills.
As the software development landscape continues to evolve, the principles of version control remain constant. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for a technical interview, a solid understanding of version control will serve you well throughout your career. Embrace these tools and practices, and you’ll be well-equipped to tackle the challenges of modern software development.