The Importance of Unit Testing in Software Development: A Comprehensive Guide
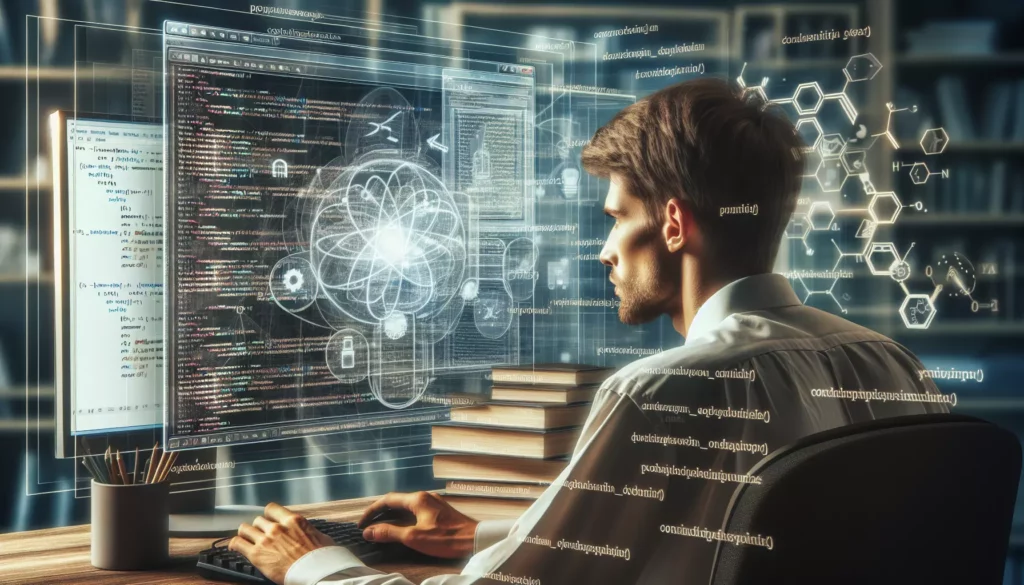
In the ever-evolving landscape of software development, ensuring code quality and reliability has become paramount. One of the most effective ways to achieve this is through unit testing. This comprehensive guide will delve into the importance of unit testing in development, exploring its benefits, best practices, and how it fits into the broader context of software quality assurance.
What is Unit Testing?
Before we dive into the importance of unit testing, let’s first define what it is. Unit testing is a software testing method where individual units or components of a software are tested in isolation. The primary purpose is to validate that each unit of the software performs as designed.
A unit is the smallest testable part of any software. It usually has one or a few inputs and usually a single output. In procedural programming, a unit may be an individual program, function, procedure, etc. In object-oriented programming, the smallest unit is a method, which may belong to a base/super class, abstract class or derived/child class.
The Importance of Unit Testing
Now that we understand what unit testing is, let’s explore why it’s so crucial in software development:
1. Early Bug Detection
One of the most significant advantages of unit testing is its ability to catch bugs early in the development process. By testing individual components in isolation, developers can identify and fix issues before they become more complex and costly to resolve later in the development cycle.
2. Facilitates Changes and Simplifies Integration
Unit tests make it easier to refactor code and make changes with confidence. When you modify your code and run the tests, you can immediately see if the changes have broken any existing functionality. This is particularly valuable when integrating different parts of an application, as it helps ensure that individual components work correctly before they’re combined.
3. Improves Code Quality
Writing unit tests often leads to better code design. When developers write tests first (a practice known as Test-Driven Development or TDD), they’re forced to think about the structure and interface of their code before implementing it. This often results in more modular, flexible, and maintainable code.
4. Serves as Documentation
Well-written unit tests can serve as documentation for your code. They provide examples of how the code is intended to be used and what output is expected for given inputs. This can be incredibly helpful for other developers (or yourself in the future) who need to understand or modify the code.
5. Reduces Debugging Time
When a test fails, it often points directly to where the code needs to be fixed. This significantly reduces the time spent on debugging, as developers can quickly isolate the issue to a specific unit of code.
6. Encourages Modular Design
To make code easily testable, developers often need to design it in a modular way. This modularity is a key principle of good software design, leading to more flexible and maintainable code.
7. Provides Confidence in Code Correctness
A comprehensive suite of unit tests provides confidence that the code is working as expected. This is especially important when making changes or adding new features, as you can quickly verify that existing functionality hasn’t been broken.
Best Practices for Unit Testing
To get the most out of unit testing, it’s important to follow some best practices:
1. Write Tests First
Following the Test-Driven Development (TDD) approach, write your tests before writing the actual code. This helps ensure that your code is testable from the start and meets the specified requirements.
2. Keep Tests Simple and Focused
Each unit test should focus on testing a single behavior or aspect of your code. Avoid complex tests that try to verify multiple things at once.
3. Use Descriptive Test Names
Your test names should clearly describe what is being tested and under what conditions. This makes it easier to understand what a failing test is actually testing.
4. Aim for High Code Coverage
While 100% code coverage isn’t always necessary or practical, aim to cover as much of your code as possible with unit tests. This includes both the “happy path” and edge cases.
5. Make Tests Independent
Each test should be able to run independently of others. Avoid dependencies between tests, as this can make your test suite brittle and hard to maintain.
6. Use Mocking and Stubbing
When testing units that depend on other components, use mocking or stubbing to isolate the unit under test. This allows you to test the unit’s behavior without being affected by its dependencies.
7. Keep Tests Fast
Unit tests should run quickly. If your tests are slow, developers will be less likely to run them frequently, which defeats much of the purpose of having tests.
Unit Testing Frameworks
There are numerous frameworks available for unit testing, depending on the programming language you’re using. Here are some popular ones:
- Java: JUnit, TestNG
- Python: unittest, pytest
- JavaScript: Jest, Mocha
- C#: NUnit, xUnit.net
- Ruby: RSpec, Minitest
- C++: Google Test, Catch2
These frameworks provide tools and utilities to make writing and running tests easier, as well as reporting mechanisms to help you understand your test results.
Example of a Unit Test
Let’s look at a simple example of a unit test in Python using the unittest framework:
import unittest
def add(a, b):
return a + b
class TestAddFunction(unittest.TestCase):
def test_add_positive_numbers(self):
self.assertEqual(add(2, 3), 5)
def test_add_negative_numbers(self):
self.assertEqual(add(-1, -1), -2)
def test_add_zero(self):
self.assertEqual(add(5, 0), 5)
if __name__ == '__main__':
unittest.main()
In this example, we’re testing a simple add function with three different test cases: adding positive numbers, negative numbers, and adding zero. Each test case is a method in our TestAddFunction class, which inherits from unittest.TestCase.
Unit Testing in the Context of AlgoCademy
At AlgoCademy, we emphasize the importance of unit testing as part of our comprehensive approach to coding education and programming skills development. Here’s how unit testing fits into our broader educational goals:
1. Reinforcing Best Practices
By introducing unit testing early in our curriculum, we help students develop good habits and understand the importance of writing testable code from the start.
2. Improving Problem-Solving Skills
Writing effective unit tests requires students to think critically about different scenarios and edge cases, enhancing their problem-solving abilities.
3. Preparing for Technical Interviews
Many technical interviews, especially at major tech companies, include questions about testing. Understanding unit testing gives our students an edge in these situations.
4. Enhancing Code Quality
As students progress through our platform, the practice of unit testing helps them write cleaner, more modular, and more reliable code.
5. Real-World Skill Development
Unit testing is a crucial skill in professional software development. By incorporating it into our curriculum, we’re preparing students for real-world development practices.
Challenges in Unit Testing
While unit testing offers numerous benefits, it’s not without its challenges:
1. Time Investment
Writing and maintaining unit tests takes time, which can be a challenge in projects with tight deadlines. However, this investment often pays off in the long run through reduced debugging time and improved code quality.
2. Learning Curve
For developers new to unit testing, there can be a significant learning curve in understanding how to write effective tests and use testing frameworks.
3. Keeping Tests Up to Date
As code changes, tests need to be updated as well. Failing to maintain tests can lead to false positives or negatives, reducing their effectiveness.
4. Testing Complex Scenarios
Some scenarios, particularly those involving complex dependencies or interactions between multiple components, can be challenging to unit test effectively.
5. Overreliance on Code Coverage
While code coverage is a useful metric, it’s possible to have high coverage without actually testing all important scenarios. It’s important to focus on the quality of tests, not just quantity.
Unit Testing vs. Other Types of Testing
While unit testing is crucial, it’s just one part of a comprehensive testing strategy. Other types of testing include:
Integration Testing
This tests how different components or services work together. While unit tests focus on isolated components, integration tests ensure that these components interact correctly when combined.
Functional Testing
This type of testing verifies that the software meets the specified functional requirements. It’s typically done from the user’s perspective and doesn’t concern itself with the underlying code structure.
End-to-End Testing
This involves testing the entire application from start to finish, simulating real-world scenarios. It ensures that the flow of an application is performing as designed from start to finish.
Performance Testing
This type of testing is done to determine how a system performs in terms of responsiveness and stability under a particular workload.
Each of these testing types serves a different purpose and complements unit testing in ensuring overall software quality.
The Future of Unit Testing
As software development continues to evolve, so too does the practice of unit testing. Here are some trends shaping the future of unit testing:
1. AI-Assisted Testing
Artificial Intelligence and Machine Learning are being increasingly used to generate test cases, predict areas of code that are likely to contain bugs, and even write basic unit tests.
2. Shift-Left Testing
This approach involves moving testing earlier in the development process, with unit testing playing a crucial role. This trend is likely to continue as organizations seek to catch and fix issues as early as possible.
3. Increased Automation
While unit tests are already typically automated, we’re likely to see even more automation in areas like test case generation, test execution, and results analysis.
4. Integration with CI/CD Pipelines
As Continuous Integration and Continuous Deployment (CI/CD) practices become more widespread, unit tests are being more tightly integrated into these pipelines, running automatically with each code change.
5. Focus on Non-Functional Requirements
While unit tests traditionally focus on functional correctness, there’s a growing trend towards using them to test non-functional requirements like performance and security at the unit level.
Conclusion
Unit testing is a fundamental practice in modern software development, offering numerous benefits from improved code quality to easier maintenance and refactoring. While it requires an investment of time and effort, the long-term benefits in terms of code reliability, reduced debugging time, and overall software quality make it an essential part of any development process.
At AlgoCademy, we recognize the critical importance of unit testing in developing well-rounded, job-ready programmers. By incorporating unit testing into our curriculum, we’re not just teaching coding skills, but instilling best practices that will serve our students throughout their careers in software development.
As the field of software development continues to evolve, the importance of unit testing is only likely to grow. By mastering this crucial skill, developers can ensure they’re well-prepared for the challenges and opportunities of modern software development.