The Importance of Unit Testing in Development: A Comprehensive Guide
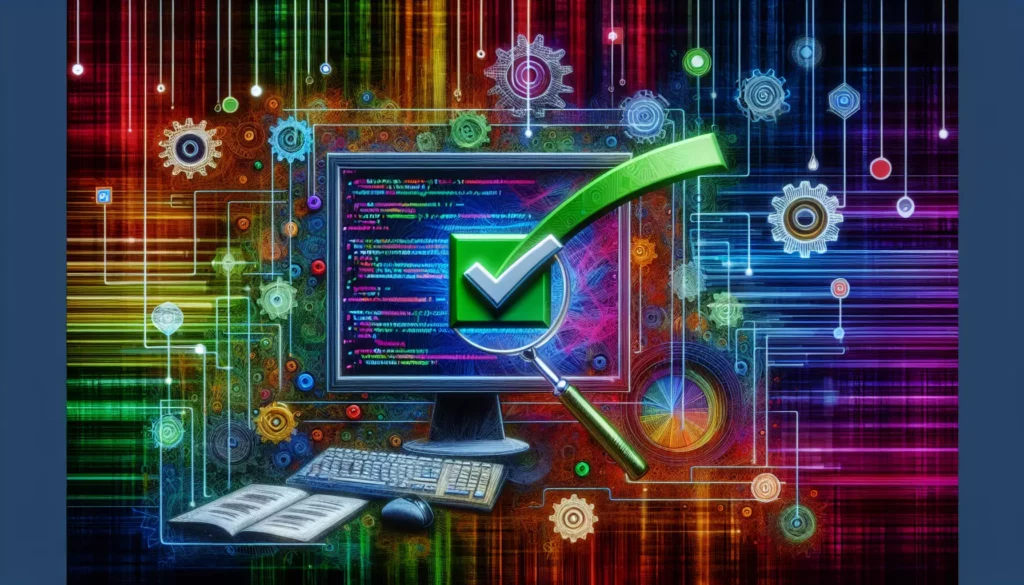
In the world of software development, quality assurance is paramount. One of the most effective ways to ensure the reliability and functionality of your code is through unit testing. This practice has become an essential part of the development process, especially for those aiming to work in major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google). In this comprehensive guide, we’ll explore the importance of unit testing in development, its benefits, best practices, and how it fits into the broader context of software engineering.
What is Unit Testing?
Before diving into the importance of unit testing, let’s first define what it is. Unit testing is a software testing method where individual units or components of a software are tested in isolation. The primary purpose is to validate that each unit of the software performs as designed.
A unit is the smallest testable part of any software. It usually has one or a few inputs and usually a single output. In procedural programming, a unit may be an individual program, function, procedure, etc. In object-oriented programming, the smallest unit is a method, which may belong to a base/super class, abstract class or derived/child class.
The Importance of Unit Testing
Now that we understand what unit testing is, let’s explore why it’s so crucial in the development process:
1. Early Bug Detection
One of the primary benefits of unit testing is the ability to catch bugs early in the development cycle. By testing individual components in isolation, developers can identify and fix issues before they become more complex and costly to resolve. This early detection can save significant time and resources in the long run.
2. Improved Code Quality
Unit testing encourages developers to write cleaner, more modular code. When you know your code will be tested, you’re more likely to structure it in a way that’s easily testable. This often results in more maintainable and readable code, which is crucial for large-scale projects and team collaborations.
3. Facilitates Changes and Refactoring
With a comprehensive suite of unit tests, developers can make changes to the codebase with confidence. If a change breaks existing functionality, the unit tests will catch it immediately. This safety net allows for more aggressive refactoring and optimization without the fear of introducing unintended side effects.
4. Serves as Documentation
Well-written unit tests can serve as a form of documentation for your code. They demonstrate how the code is supposed to work and provide examples of how to use various components. This can be invaluable for onboarding new team members or returning to a project after a long period.
5. Debugging Simplification
When a test fails, it points directly to the specific unit of code that’s not working as expected. This precision makes debugging much more straightforward compared to trying to track down issues in a fully integrated system.
6. Supports Agile and TDD Practices
Unit testing is a cornerstone of Test-Driven Development (TDD) and fits well with Agile methodologies. It allows for continuous integration and delivery, ensuring that new code additions don’t break existing functionality.
Best Practices for Unit Testing
To get the most out of unit testing, consider the following best practices:
1. Write Tests First
Following the TDD approach, write your tests before writing the actual code. This ensures that you’re designing your code to be testable from the start.
2. Keep Tests Simple and Focused
Each unit test should focus on a single functionality. Avoid complex tests that try to cover multiple scenarios at once.
3. Use Descriptive Test Names
Your test names should clearly describe what is being tested and under what conditions. This makes it easier to understand what went wrong when a test fails.
4. Aim for Independence
Tests should be independent of each other. The order in which tests are run should not matter, and one test should not rely on the state created by another test.
5. Mock External Dependencies
Use mocking frameworks to isolate the unit being tested from its dependencies. This ensures you’re truly testing the unit in isolation.
6. Maintain Your Tests
As your codebase evolves, so should your tests. Regularly review and update your test suite to ensure it remains relevant and effective.
Unit Testing Frameworks
There are numerous frameworks available for unit testing, depending on your programming language and specific needs. Here are some popular ones:
- JUnit (Java)
- pytest (Python)
- Mocha (JavaScript)
- NUnit (.NET)
- RSpec (Ruby)
Let’s look at a simple example of a unit test using pytest for Python:
def add(a, b):
return a + b
def test_add():
assert add(2, 3) == 5
assert add(-1, 1) == 0
assert add(-1, -1) == -2
In this example, we’re testing a simple addition function. The test checks if the function correctly handles positive numbers, negative numbers, and zero.
Unit Testing in the Context of FAANG Companies
For those aspiring to work at major tech companies like FAANG, understanding and implementing unit testing is crucial. These companies have large, complex codebases that require robust testing practices to maintain reliability and scalability.
Facebook uses a custom testing framework called Jest, which was later open-sourced. They emphasize the importance of fast, reliable tests to support their continuous deployment model.
Amazon
Amazon places a strong emphasis on automated testing, including unit tests, to ensure the reliability of their vast e-commerce platform and AWS services.
Apple
Apple provides XCTest, a testing framework integrated with Xcode, encouraging developers to write and run unit tests for iOS and macOS applications.
Netflix
Netflix has developed several testing tools, including Hystrix for fault tolerance testing. They rely heavily on automated testing to ensure the quality of their streaming service across multiple devices and platforms.
Google is known for its extensive use of automated testing, including unit tests. They’ve developed tools like Googletest for C++ testing and have contributed significantly to testing methodologies in the industry.
Challenges in Unit Testing
While unit testing offers numerous benefits, it’s not without its challenges:
1. Time Investment
Writing and maintaining unit tests takes time, which can be seen as a drawback in fast-paced development environments. However, this initial investment often pays off in the long run through reduced debugging time and fewer production issues.
2. Test Coverage
Determining what to test and how much test coverage is sufficient can be challenging. While 100% coverage might seem ideal, it’s often impractical and may not necessarily guarantee bug-free code.
3. Maintaining Test Suites
As the codebase evolves, test suites need to be updated accordingly. This ongoing maintenance can be time-consuming but is crucial for the continued effectiveness of your testing strategy.
4. Testing Complex Scenarios
Some functionalities, particularly those involving complex integrations or user interfaces, can be challenging to unit test effectively. In these cases, other testing methods like integration or end-to-end testing may be more appropriate.
Unit Testing and Algorithmic Thinking
Unit testing is not just about verifying code functionality; it also promotes algorithmic thinking. When writing tests, developers need to consider various scenarios, edge cases, and potential inputs. This process enhances problem-solving skills and encourages a more thorough understanding of the code’s behavior.
For example, when unit testing a sorting algorithm, you might consider the following test cases:
def test_sorting_algorithm():
assert sort([3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5]) == [1, 1, 2, 3, 3, 4, 5, 5, 5, 6, 9]
assert sort([]) == [] # Empty list
assert sort([1]) == [1] # Single element
assert sort([2, 2, 2, 2]) == [2, 2, 2, 2] # All identical elements
assert sort([-1, -5, 0, 3, -2]) == [-5, -2, -1, 0, 3] # Negative numbers
This example demonstrates how unit testing encourages thinking about various scenarios, including edge cases like empty lists or lists with a single element.
Unit Testing and Continuous Integration/Continuous Deployment (CI/CD)
Unit testing plays a crucial role in CI/CD pipelines, which are widely used in modern software development, especially in FAANG companies. In a CI/CD environment:
- Tests are automatically run whenever code is pushed to the repository.
- Failed tests prevent code from being merged or deployed, ensuring that only working code makes it to production.
- This automation allows for faster development cycles and more frequent, reliable releases.
Here’s a simple example of how unit tests might be integrated into a CI/CD pipeline using GitHub Actions:
name: Python application
on: [push]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up Python
uses: actions/setup-python@v2
with:
python-version: '3.x'
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install pytest
- name: Run tests
run: |
pytest
This workflow automatically runs pytest on every push to the repository, ensuring that all tests pass before the code can be merged or deployed.
Unit Testing and Code Reviews
Unit tests also play a significant role in the code review process. When reviewing code, the presence of comprehensive unit tests:
- Demonstrates that the developer has considered various scenarios and edge cases.
- Provides reviewers with clear examples of how the code is expected to behave.
- Increases confidence in the code’s correctness and robustness.
- Allows reviewers to focus on higher-level concerns like architecture and design, rather than hunting for basic bugs.
The Future of Unit Testing
As software development continues to evolve, so does the practice of unit testing. Some trends to watch include:
1. AI-Assisted Testing
Machine learning algorithms are being developed to help generate test cases, predict areas of code that are likely to contain bugs, and even write basic unit tests automatically.
2. Property-Based Testing
This approach involves defining general properties that your code should satisfy, rather than specific test cases. The testing framework then generates random inputs to verify these properties.
3. Snapshot Testing
Particularly useful for user interfaces, snapshot testing involves capturing a “snapshot” of your UI and comparing it to a reference snapshot to detect unintended changes.
4. Mutation Testing
This technique involves making small changes (mutations) to your code and running your tests. If your tests still pass after a mutation, it might indicate that your tests aren’t thorough enough.
Conclusion
Unit testing is a fundamental practice in modern software development, particularly for those aspiring to work in major tech companies like FAANG. It offers numerous benefits, including early bug detection, improved code quality, and easier maintenance and refactoring.
While it does come with challenges, the long-term benefits of unit testing far outweigh the initial time investment. By catching bugs early, facilitating code changes, and serving as living documentation, unit tests contribute significantly to the overall quality and reliability of software projects.
As you continue your journey in software development, remember that proficiency in unit testing is not just a valuable skill—it’s an essential practice for writing robust, maintainable code. Whether you’re working on personal projects, contributing to open source, or preparing for technical interviews at top tech companies, mastering unit testing will serve you well throughout your career.
Keep practicing, stay curious, and happy coding!