The Importance of Understanding Greedy Algorithms: A Comprehensive Guide
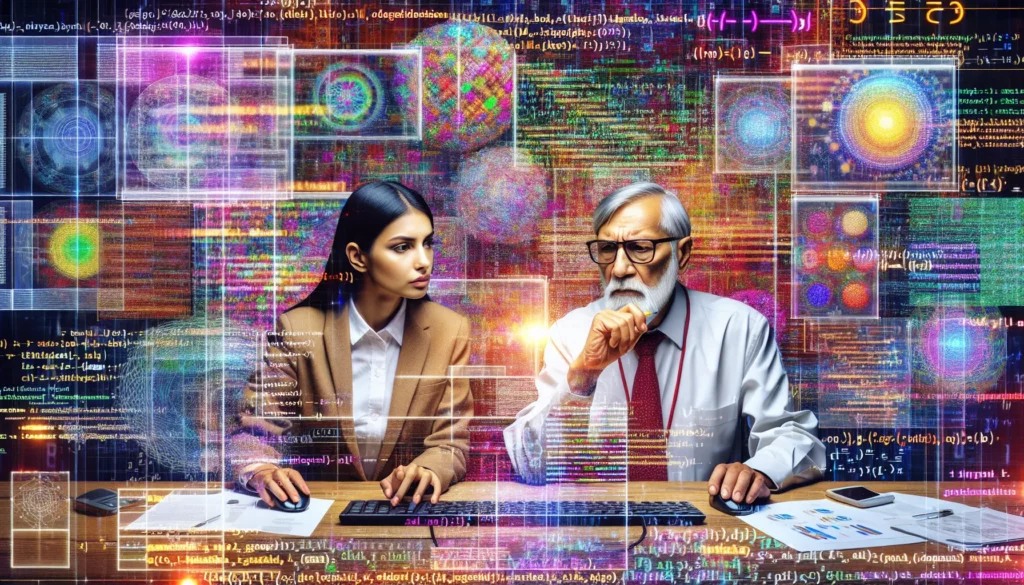
In the vast landscape of computer science and programming, algorithms serve as the backbone of efficient problem-solving. Among the various algorithmic paradigms, greedy algorithms stand out for their simplicity and effectiveness in certain scenarios. This comprehensive guide will delve into the world of greedy algorithms, exploring their significance, applications, and why understanding them is crucial for aspiring programmers and seasoned developers alike.
What Are Greedy Algorithms?
Greedy algorithms are a class of algorithms that make locally optimal choices at each step with the hope of finding a global optimum. In other words, they always make the choice that seems best at the moment without considering the bigger picture. This “greedy” approach can lead to efficient solutions for many problems, but it’s important to note that it doesn’t always guarantee the best overall solution.
The key characteristics of greedy algorithms include:
- Making the locally optimal choice at each step
- Never reconsidering previous choices
- Aiming to find an approximate global optimum
- Often simple and intuitive to implement
The Importance of Greedy Algorithms in Programming
Understanding greedy algorithms is crucial for several reasons:
1. Efficiency in Problem-Solving
Greedy algorithms are often the most efficient solution for certain types of problems. They can provide quick and straightforward solutions to complex issues, making them valuable in time-sensitive scenarios or when dealing with large datasets.
2. Foundational Knowledge
Greedy algorithms form a fundamental part of algorithmic thinking. They serve as a stepping stone to understanding more complex algorithmic paradigms and help develop problem-solving skills that are applicable across various domains in computer science.
3. Real-World Applications
Many real-world problems can be effectively solved using greedy algorithms. From scheduling tasks to optimizing network routing, greedy algorithms find applications in diverse fields such as:
- Data compression (e.g., Huffman coding)
- Graph algorithms (e.g., Dijkstra’s algorithm for shortest paths)
- Resource allocation in operating systems
- Optimization problems in various industries
4. Interview Preparation
Greedy algorithms are a popular topic in technical interviews, especially for positions at major tech companies. Understanding and being able to implement greedy solutions can give candidates a significant advantage in the competitive job market.
Common Greedy Algorithm Problems and Solutions
To better understand how greedy algorithms work, let’s explore some classic problems and their greedy solutions:
1. The Coin Change Problem
Problem: Given a set of coin denominations and a target amount, find the minimum number of coins needed to make up that amount.
Greedy Approach: Start with the largest denomination and use as many of those coins as possible, then move to the next largest denomination, and so on.
def coin_change(coins, amount):
coins.sort(reverse=True) # Sort coins in descending order
count = 0
for coin in coins:
while amount >= coin:
amount -= coin
count += 1
return count if amount == 0 else -1
# Example usage
coins = [25, 10, 5, 1]
amount = 63
result = coin_change(coins, amount)
print(f"Minimum number of coins: {result}")
Note: This greedy approach works for the US coin system but may not work for all coin systems.
2. Activity Selection Problem
Problem: Given a set of activities with start and finish times, select the maximum number of non-overlapping activities.
Greedy Approach: Sort activities by finish time and select activities that don’t overlap with the previously selected activity.
def activity_selection(activities):
# Sort activities by finish time
activities.sort(key=lambda x: x[1])
selected = [activities[0]]
last_finish = activities[0][1]
for activity in activities[1:]:
if activity[0] >= last_finish:
selected.append(activity)
last_finish = activity[1]
return selected
# Example usage
activities = [(1, 4), (3, 5), (0, 6), (5, 7), (3, 9), (5, 9), (6, 10), (8, 11), (8, 12), (2, 14), (12, 16)]
result = activity_selection(activities)
print(f"Selected activities: {result}")
3. Fractional Knapsack Problem
Problem: Given a set of items with weights and values, and a knapsack with a maximum weight capacity, maximize the value of items in the knapsack.
Greedy Approach: Sort items by value-to-weight ratio and add items to the knapsack in descending order of this ratio, taking fractions of items if necessary.
def fractional_knapsack(items, capacity):
# Sort items by value-to-weight ratio in descending order
items.sort(key=lambda x: x[1] / x[0], reverse=True)
total_value = 0
for weight, value in items:
if capacity >= weight:
# Take the whole item
capacity -= weight
total_value += value
else:
# Take a fraction of the item
fraction = capacity / weight
total_value += value * fraction
break
return total_value
# Example usage
items = [(10, 60), (20, 100), (30, 120)] # (weight, value)
capacity = 50
result = fractional_knapsack(items, capacity)
print(f"Maximum value: {result}")
When to Use Greedy Algorithms
Greedy algorithms are particularly useful in scenarios where:
- The problem has optimal substructure (optimal solution to the problem contains optimal solutions to sub-problems)
- The problem has the greedy choice property (locally optimal choices lead to a globally optimal solution)
- There’s a need for quick, approximate solutions
- The problem involves optimization over a set of choices
However, it’s crucial to recognize that greedy algorithms don’t always yield the optimal solution for every problem. In some cases, they may produce sub-optimal results or fail to find a solution at all.
Limitations of Greedy Algorithms
While greedy algorithms offer simplicity and efficiency, they come with certain limitations:
1. Not Always Optimal
Greedy algorithms make locally optimal choices, which don’t always lead to the globally optimal solution. For example, in the classic “Traveling Salesman Problem,” a greedy approach of always choosing the nearest unvisited city doesn’t guarantee the shortest overall route.
2. Lack of Foresight
By focusing on immediate gains, greedy algorithms may miss better long-term solutions. They don’t reconsider past decisions, which can sometimes lead to suboptimal results.
3. Problem-Specific
The effectiveness of a greedy algorithm often depends on the specific problem and its constraints. A greedy approach that works well for one problem may fail for a similar but slightly different problem.
4. Proving Correctness
It can be challenging to prove that a greedy algorithm always produces the optimal solution for a given problem. This often requires mathematical proofs or counterexamples.
Comparing Greedy Algorithms with Other Approaches
To better understand the place of greedy algorithms in the broader context of problem-solving techniques, let’s compare them with other common approaches:
Greedy vs. Dynamic Programming
Dynamic Programming (DP) is another powerful problem-solving technique that, like greedy algorithms, builds a solution incrementally. However, there are key differences:
- Greedy algorithms make the locally optimal choice at each step, while DP considers all possible choices and their consequences.
- DP is generally more powerful and can solve a wider range of problems optimally, but it often requires more time and space.
- Greedy algorithms are typically faster and use less memory, but may not always find the optimal solution.
Example: The Knapsack Problem
For the 0/1 Knapsack problem (where items cannot be divided), a greedy approach might not yield the optimal solution, whereas dynamic programming will always find the best solution.
Greedy vs. Brute Force
Brute force approaches involve exhaustively checking all possible solutions to find the best one. Compared to greedy algorithms:
- Brute force guarantees finding the optimal solution but can be extremely time-consuming for large inputs.
- Greedy algorithms are much faster but may not always find the best solution.
- Brute force is often used as a baseline or for small inputs, while greedy algorithms can handle larger scales more efficiently.
Greedy vs. Divide and Conquer
Divide and conquer algorithms break down a problem into smaller subproblems, solve them independently, and then combine the results. In contrast:
- Greedy algorithms make a single choice at each step without dividing the problem.
- Divide and conquer can be more versatile but may require more complex implementation.
- Some problems can be solved efficiently using either approach, depending on the specific requirements.
Implementing Greedy Algorithms: Best Practices
When implementing greedy algorithms, consider the following best practices:
1. Prove Correctness
Before implementing a greedy solution, try to prove (or at least convince yourself) that the greedy approach will lead to the optimal solution for the given problem.
2. Consider Edge Cases
Test your algorithm with various input sizes and edge cases to ensure it handles all scenarios correctly.
3. Optimize for Efficiency
Since one of the main advantages of greedy algorithms is their efficiency, focus on optimizing your implementation for speed and memory usage.
4. Use Appropriate Data Structures
Choose data structures that support efficient operations required by your greedy algorithm. For example, priority queues are often useful in greedy algorithms.
5. Document Your Approach
Clearly document the greedy strategy you’re using and why it’s appropriate for the problem at hand. This helps in understanding and maintaining the code.
Advanced Greedy Algorithm Concepts
As you delve deeper into greedy algorithms, you’ll encounter more advanced concepts and applications:
1. Matroids and Greedy Algorithms
Matroids are mathematical structures that generalize the notion of linear independence in vector spaces. Many optimization problems that can be solved optimally with greedy algorithms are instances of matroid optimization.
2. Approximation Algorithms
For some NP-hard problems, greedy algorithms can serve as approximation algorithms, providing solutions that are guaranteed to be within a certain factor of the optimal solution.
3. Online Algorithms
Greedy strategies are often employed in online algorithms, where decisions must be made without knowledge of future inputs.
4. Randomized Greedy Algorithms
Introducing randomness into greedy algorithms can sometimes improve their performance or provide probabilistic guarantees on the quality of the solution.
Greedy Algorithms in Technical Interviews
Understanding greedy algorithms is crucial for technical interviews, especially when applying to major tech companies. Here are some tips for tackling greedy algorithm problems in interviews:
1. Identify Greedy Choice Property
Look for problems where making the locally optimal choice at each step leads to a globally optimal solution.
2. Prove Correctness
Be prepared to explain why your greedy approach works. Practice articulating your reasoning clearly.
3. Consider Time and Space Complexity
Analyze and discuss the efficiency of your greedy solution in terms of both time and space complexity.
4. Implement Efficiently
Practice implementing greedy algorithms efficiently, using appropriate data structures and optimizations.
5. Know Classic Problems
Familiarize yourself with classic greedy problems like activity selection, Huffman coding, and minimum spanning trees.
Conclusion
Greedy algorithms represent a powerful and efficient approach to solving a wide range of problems in computer science and beyond. Their simplicity, combined with their effectiveness in certain scenarios, makes them an essential tool in any programmer’s toolkit.
Understanding greedy algorithms not only enhances problem-solving skills but also provides insights into algorithmic thinking and optimization techniques. While they may not always yield the optimal solution, their efficiency and straightforward nature make them invaluable in many real-world applications.
As you continue your journey in programming and computer science, remember that mastering greedy algorithms is just one step towards becoming a well-rounded problem solver. Combine this knowledge with other algorithmic paradigms, data structures, and problem-solving techniques to tackle complex challenges effectively.
Keep practicing, exploring new problems, and refining your skills. With dedication and continuous learning, you’ll be well-equipped to handle a wide array of algorithmic challenges, whether in technical interviews, competitive programming, or real-world software development.