The Importance of Practicing Under Simulated Conditions in Coding Education
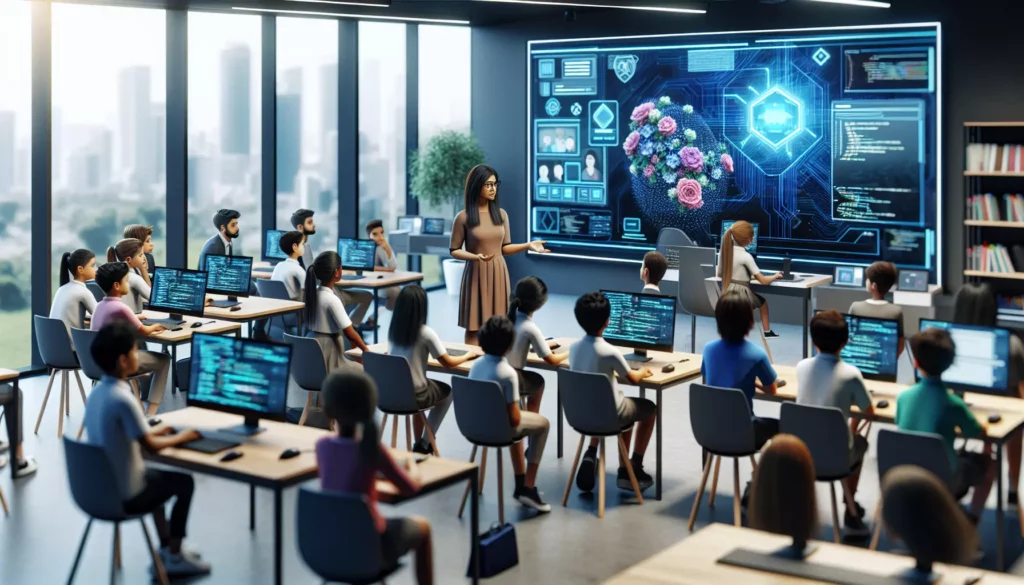
In the ever-evolving landscape of technology and software development, the ability to write efficient, clean, and bug-free code is a highly sought-after skill. As aspiring programmers and seasoned developers alike strive to improve their craft, one crucial aspect of learning often gets overlooked: practicing under simulated conditions. This approach to coding education can make a significant difference in how well-prepared individuals are for real-world challenges, particularly when it comes to technical interviews and on-the-job performance.
In this comprehensive guide, we’ll explore the importance of simulated practice in coding education, its benefits, and how platforms like AlgoCademy are revolutionizing the way programmers learn and prepare for their careers. We’ll delve into various aspects of this learning methodology and provide insights on how to make the most of simulated coding environments.
Understanding Simulated Conditions in Coding Education
Simulated conditions in coding education refer to learning environments that closely mimic real-world scenarios programmers might encounter in their professional lives. These can include:
- Time-constrained coding challenges
- Mock technical interviews
- Collaborative coding exercises
- Debugging sessions with intentionally introduced errors
- Code reviews and feedback sessions
The goal of these simulated conditions is to prepare learners for the pressures, expectations, and complexities they’ll face in actual coding interviews and professional development environments. By exposing students to these scenarios in a controlled, educational setting, they can develop not only technical skills but also the mental fortitude and problem-solving abilities required to excel in the field.
The Benefits of Practicing Under Simulated Conditions
1. Enhanced Problem-Solving Skills
When faced with simulated coding challenges, learners are forced to think on their feet and apply their knowledge in practical ways. This helps develop critical problem-solving skills that are essential in the world of programming. By working through complex algorithms and data structures under time constraints, students learn to break down problems, identify patterns, and implement efficient solutions more quickly.
2. Improved Time Management
Many coding interviews and real-world projects come with strict deadlines. Practicing under simulated conditions helps programmers become more adept at managing their time effectively. They learn to prioritize tasks, estimate the time required for different parts of a problem, and work efficiently under pressure.
3. Increased Confidence
Familiarity breeds confidence. By repeatedly exposing themselves to simulated coding scenarios, learners become more comfortable with the types of challenges they’ll face in actual interviews or on the job. This increased confidence can lead to better performance when it really counts.
4. Better Interview Preparation
Technical interviews, especially those at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), can be notoriously challenging. Simulated practice that mimics these interview conditions can give candidates a significant advantage. They’ll be familiar with the format, types of questions asked, and the pressure of coding on the spot.
5. Exposure to Different Coding Styles and Approaches
In simulated collaborative exercises or code review sessions, learners are exposed to different coding styles and problem-solving approaches. This broadens their perspective and helps them become more versatile programmers.
6. Identification of Weaknesses and Areas for Improvement
Simulated practice often reveals areas where a programmer needs to improve. Whether it’s a particular algorithm they struggle with or a tendency to overlook edge cases, identifying these weaknesses in a low-stakes environment allows learners to focus their efforts on improvement.
How AlgoCademy Incorporates Simulated Conditions
AlgoCademy, as a platform dedicated to coding education and programming skills development, recognizes the importance of simulated practice. Here’s how it integrates this approach into its learning ecosystem:
1. Timed Coding Challenges
AlgoCademy offers a variety of timed coding challenges that simulate the pressure of technical interviews. These challenges cover a range of difficulty levels and topics, allowing learners to gradually build their skills and confidence.
2. AI-Powered Mock Interviews
Leveraging artificial intelligence, AlgoCademy provides mock interview experiences that closely resemble those conducted by major tech companies. The AI adapts to the user’s skill level, providing appropriate follow-up questions and hints when needed.
3. Interactive Coding Tutorials
The platform’s interactive tutorials guide learners through complex coding concepts, allowing them to write and test code in real-time. This hands-on approach simulates the experience of working on actual projects.
4. Collaborative Coding Exercises
AlgoCademy facilitates collaborative coding sessions where learners can work together on projects, simulating the teamwork required in professional settings.
5. Code Review and Feedback System
After completing challenges or projects, users can receive detailed feedback on their code. This simulates the code review process common in professional development environments.
Implementing Simulated Practice in Your Coding Journey
Whether you’re using AlgoCademy or other resources, here are some tips for incorporating simulated practice into your coding education:
1. Set Realistic Time Constraints
When working on coding problems, set a timer to simulate the time pressure of an interview or project deadline. Start with generous time limits and gradually reduce them as you improve.
2. Practice Whiteboarding
Many technical interviews involve coding on a whiteboard. Practice solving problems on a physical whiteboard or using a digital equivalent to get comfortable with this format.
3. Explain Your Thought Process
Get in the habit of explaining your approach out loud as you code. This simulates the expectation in many interviews where candidates are asked to verbalize their problem-solving process.
4. Seek Out Code Reviews
Regularly have your code reviewed by peers or mentors. This will help you get used to receiving feedback and improve the quality of your code.
5. Participate in Coding Competitions
Platforms like LeetCode, HackerRank, and Codeforces offer coding competitions that simulate high-pressure coding scenarios.
6. Create Mock Interview Groups
Form study groups with fellow learners where you can take turns interviewing each other. This provides valuable practice in both solving problems and asking technical questions.
Common Algorithms and Data Structures to Focus On
When practicing under simulated conditions, it’s important to focus on the types of problems and concepts that frequently appear in technical interviews and real-world programming scenarios. Here are some key areas to concentrate on:
1. Array Manipulation
Arrays are fundamental data structures, and many interview questions involve manipulating them efficiently. Practice problems like two-pointer techniques, sliding window algorithms, and in-place operations.
2. String Processing
String manipulation is a common task in programming. Focus on problems involving string searching, pattern matching, and efficient string operations.
3. Linked Lists
Understanding linked list operations and being able to implement them quickly is crucial. Practice problems like reversing a linked list, detecting cycles, and merging sorted lists.
4. Trees and Graphs
Tree and graph traversal algorithms are essential. Focus on depth-first search (DFS), breadth-first search (BFS), and common tree operations like balancing and path finding.
5. Dynamic Programming
Dynamic programming is a powerful technique for solving optimization problems. Practice identifying and solving problems that can be optimized using memoization or tabulation.
6. Sorting and Searching
Understanding various sorting algorithms (e.g., quicksort, mergesort) and searching techniques (e.g., binary search) is crucial. Practice implementing these from scratch and analyzing their time and space complexities.
7. Hash Tables
Hash tables are used in many efficient algorithms. Practice problems that involve using hash tables for quick lookups and solving problems with O(1) time complexity.
Example: Simulated Coding Challenge
Let’s look at an example of a simulated coding challenge that you might encounter on a platform like AlgoCademy or in a technical interview. This problem tests your ability to work with arrays and implement an efficient solution under time constraints.
Problem: Two Sum
Given an array of integers nums
and an integer target
, return indices of the two numbers in the array such that they add up to the target
. You may assume that each input would have exactly one solution, and you may not use the same element twice.
Here’s a Python implementation of the solution:
def two_sum(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return [] # No solution found
# Test the function
nums = [2, 7, 11, 15]
target = 9
result = two_sum(nums, target)
print(f"Indices: {result}")
This solution uses a hash table (dictionary in Python) to achieve O(n) time complexity. It demonstrates efficient problem-solving and the use of appropriate data structures, which are key skills in simulated coding practice.
The Role of AI in Simulated Coding Practice
Artificial Intelligence is playing an increasingly important role in coding education, particularly in creating more realistic and adaptive simulated practice environments. Here’s how AI is enhancing the learning experience:
1. Personalized Problem Selection
AI algorithms can analyze a learner’s performance and select problems that are most beneficial for their skill level and learning goals. This ensures that practice sessions are always challenging but not overwhelming.
2. Real-time Feedback and Hints
During simulated coding sessions, AI can provide immediate feedback on code quality, efficiency, and correctness. It can also offer tailored hints when a learner is stuck, simulating the guidance a human interviewer might provide.
3. Natural Language Processing for Mock Interviews
Advanced NLP techniques allow AI to conduct mock interviews that feel more natural and responsive. The AI can ask follow-up questions, request clarifications, and even engage in discussions about design decisions.
4. Code Analysis and Optimization Suggestions
AI-powered tools can analyze submitted code and suggest optimizations or alternative approaches, helping learners understand different ways to solve problems efficiently.
5. Simulating Diverse Interview Styles
AI can be programmed to simulate different interviewer personalities and interview styles, preparing candidates for a wide range of potential interview scenarios.
Overcoming Challenges in Simulated Practice
While simulated practice offers numerous benefits, it also comes with its own set of challenges. Here are some common obstacles learners face and strategies to overcome them:
1. Performance Anxiety
Challenge: The pressure of timed challenges or mock interviews can cause anxiety, leading to underperformance.
Solution: Start with untimed practice to build confidence. Gradually introduce time limits, and practice relaxation techniques to manage stress during simulated sessions.
2. Plateau in Progress
Challenge: Learners may hit a plateau where they feel they’re not improving despite regular practice.
Solution: Vary the types of problems and difficulty levels. Seek out new resources or perspectives, and consider working with a mentor to identify areas for improvement.
3. Discouragement from Difficult Problems
Challenge: Consistently facing difficult problems can be discouraging, especially for beginners.
Solution: Mix easier problems with challenging ones to maintain motivation. Celebrate small victories and focus on the learning process rather than just the outcome.
4. Lack of Real-world Context
Challenge: Some simulated problems may feel disconnected from real-world programming tasks.
Solution: Seek out project-based learning opportunities that simulate actual development scenarios. Platforms like AlgoCademy often provide context for how abstract problems relate to real-world applications.
5. Overemphasis on Speed
Challenge: The focus on solving problems quickly in simulated environments may lead to neglecting code quality and best practices.
Solution: Balance timed challenges with untimed practice sessions focused on writing clean, maintainable code. Regularly review and refactor your solutions.
Measuring Progress in Simulated Practice
To ensure that your simulated practice is effective, it’s important to track your progress over time. Here are some metrics and methods to consider:
1. Problem-Solving Speed
Track how quickly you can solve problems of similar difficulty levels. Look for improvements in your average solving time.
2. Success Rate
Monitor the percentage of problems you can solve correctly within the given time constraints. Aim for a gradually improving success rate.
3. Complexity of Problems Solved
Keep track of the difficulty level of problems you can consistently solve. Progressively tackling more complex problems is a clear sign of improvement.
4. Code Quality Metrics
Use tools that analyze code quality, such as linters or static analysis tools. Track improvements in metrics like code readability, adherence to style guides, and reduction of code smells.
5. Mock Interview Performance
If using platforms with mock interview features, track your performance scores and feedback over time. Look for improvements in both technical skills and communication abilities.
6. Peer Comparisons
Many coding platforms offer leaderboards or percentile rankings. While not the only measure of success, improving your standing among peers can be motivating and indicative of progress.
Conclusion: Embracing Simulated Practice for Coding Success
In the dynamic field of software development, the ability to perform under pressure and adapt to various coding scenarios is invaluable. Practicing under simulated conditions offers a powerful way to develop these skills, preparing aspiring and experienced programmers alike for the challenges of technical interviews and professional coding environments.
Platforms like AlgoCademy are at the forefront of this educational approach, leveraging technology and AI to create immersive, realistic practice environments. By incorporating simulated practice into your learning routine, you can:
- Enhance your problem-solving skills
- Improve your ability to work under time constraints
- Gain confidence in your coding abilities
- Better prepare for technical interviews
- Develop a more well-rounded skill set
Remember that consistent, deliberate practice is key to improvement. Embrace the challenges of simulated coding environments, learn from your mistakes, and celebrate your progress. Whether you’re aiming for a position at a major tech company or looking to excel in your current role, the skills you develop through simulated practice will serve you well throughout your programming career.
As you continue your coding journey, leverage the resources available to you, seek out diverse practice opportunities, and don’t hesitate to push your boundaries. With dedication and the right approach to learning, you’ll be well-equipped to tackle any coding challenge that comes your way.