The Importance of Practice Tests for Algorithm Mastery
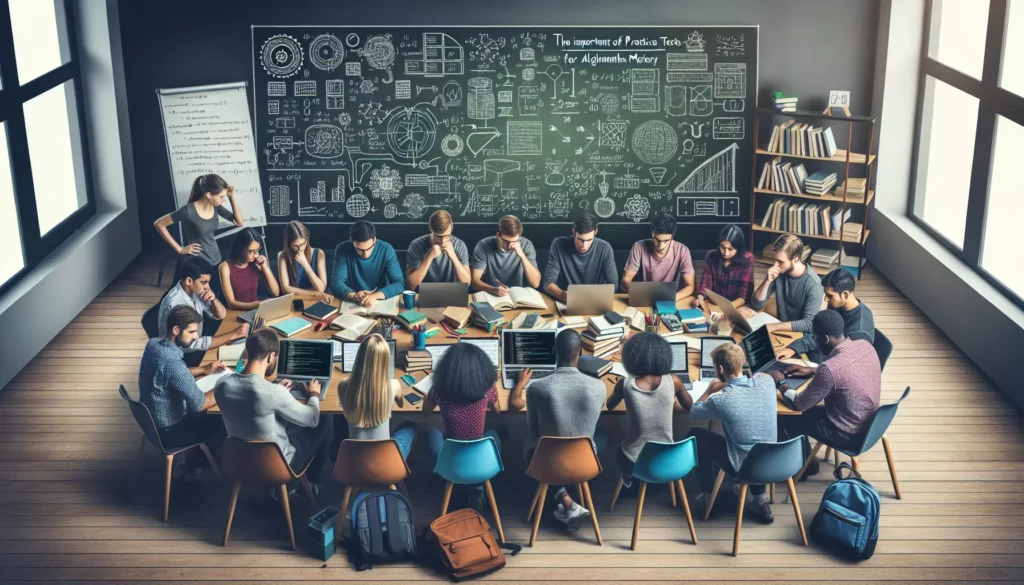
In the ever-evolving world of technology and software development, mastering algorithms is a crucial skill for any aspiring programmer. Whether you’re a beginner just starting your coding journey or an experienced developer aiming to land a job at a top tech company, the importance of practice tests in honing your algorithmic skills cannot be overstated. This article will explore why practice tests are essential for algorithm mastery and how platforms like AlgoCademy are revolutionizing the way programmers prepare for technical interviews and enhance their coding abilities.
Why Algorithms Matter in Programming
Before diving into the importance of practice tests, let’s briefly discuss why algorithms are so crucial in programming:
- Efficiency: Well-designed algorithms optimize resource usage, making programs faster and more efficient.
- Problem-solving: Algorithms provide structured approaches to solving complex problems.
- Scalability: Good algorithmic knowledge helps in creating solutions that can handle large-scale data and operations.
- Interview preparation: Many tech companies, especially FAANG (Facebook, Amazon, Apple, Netflix, Google), heavily emphasize algorithmic skills in their hiring process.
The Role of Practice Tests in Algorithm Mastery
Now that we understand the significance of algorithms, let’s explore why practice tests are indispensable for mastering them:
1. Reinforcing Theoretical Knowledge
While theoretical understanding is important, practice tests bridge the gap between theory and application. They allow you to:
- Apply concepts learned in a practical setting
- Identify areas where your theoretical understanding may be lacking
- Reinforce your knowledge through hands-on problem-solving
2. Improving Problem-Solving Skills
Regular practice with algorithmic problems enhances your ability to:
- Break down complex problems into manageable components
- Recognize patterns and apply appropriate algorithmic techniques
- Develop a systematic approach to problem-solving
3. Enhancing Code Writing Speed and Accuracy
Practice tests help you:
- Write code more quickly and efficiently
- Reduce errors and bugs in your implementations
- Improve your ability to translate ideas into working code
4. Familiarizing with Different Problem Types
Exposure to a variety of practice tests allows you to:
- Encounter diverse problem scenarios
- Learn to adapt your knowledge to different contexts
- Broaden your understanding of algorithmic applications
5. Building Confidence
Regular practice leads to:
- Increased confidence in your problem-solving abilities
- Reduced anxiety when facing new or challenging problems
- Better performance under pressure, such as in technical interviews
6. Identifying Weaknesses and Tracking Progress
Practice tests serve as a valuable tool for:
- Pinpointing areas that need improvement
- Tracking your progress over time
- Setting realistic goals for skill development
How AlgoCademy Enhances Algorithm Practice
Platforms like AlgoCademy have revolutionized the way programmers approach algorithm practice. Here’s how AlgoCademy contributes to effective algorithm mastery:
1. Interactive Coding Tutorials
AlgoCademy offers interactive tutorials that guide you through various algorithmic concepts. These tutorials:
- Provide step-by-step explanations of complex algorithms
- Allow you to write and execute code in real-time
- Offer immediate feedback on your solutions
2. Diverse Problem Sets
The platform provides a wide range of practice problems, including:
- Beginner-friendly challenges to build foundational skills
- Advanced problems that mimic technical interview questions
- Real-world scenarios to apply algorithmic thinking
3. AI-Powered Assistance
AlgoCademy leverages artificial intelligence to enhance your learning experience:
- Personalized problem recommendations based on your skill level
- Intelligent hints and suggestions when you’re stuck
- Adaptive learning paths that evolve with your progress
4. Performance Analytics
The platform offers detailed analytics to help you track your progress:
- Visualizations of your solving patterns and efficiency
- Identification of areas that need more practice
- Comparison of your performance with peers or industry standards
5. FAANG-Focused Preparation
AlgoCademy specifically caters to those aiming for positions at top tech companies:
- Practice tests modeled after actual FAANG interview questions
- Industry insights on what these companies look for in candidates
- Strategies for tackling high-pressure interview scenarios
Best Practices for Using Practice Tests
To maximize the benefits of practice tests for algorithm mastery, consider the following tips:
1. Consistent Practice
Regularity is key to improvement. Aim to:
- Set aside dedicated time for algorithm practice daily or weekly
- Maintain a consistent schedule to build a habit
- Gradually increase the difficulty of problems as you progress
2. Time Management
Simulate real-world conditions by:
- Setting time limits for solving problems
- Practicing under timed conditions to improve efficiency
- Learning to allocate time wisely between understanding, coding, and optimizing
3. Thorough Analysis
After completing a practice test:
- Review your solution, even if it’s correct
- Analyze alternative approaches and their trade-offs
- Study optimal solutions provided by the platform or community
4. Focus on Understanding
Prioritize comprehension over memorization:
- Strive to understand the underlying principles of each algorithm
- Practice explaining your approach, as you would in an interview
- Relate new problems to previously solved ones to reinforce patterns
5. Leverage Community Resources
Engage with the coding community:
- Participate in forums and discussion boards
- Collaborate with peers on problem-solving
- Share your solutions and learn from others’ approaches
Common Algorithmic Concepts to Master
While practicing, focus on mastering these fundamental algorithmic concepts:
1. Array Manipulation
Arrays are fundamental data structures. Practice problems involving:
- Searching and sorting arrays
- Two-pointer techniques
- Sliding window algorithms
2. String Processing
String manipulation is crucial in many applications. Focus on:
- Pattern matching algorithms
- String parsing and validation
- Encoding and compression techniques
3. Linked Lists
Understanding linked lists is essential. Practice:
- Traversal and manipulation of linked lists
- Detecting cycles and intersections
- Implementing operations like reversal and merging
4. Trees and Graphs
These structures are prevalent in many problems. Focus on:
- Tree traversal algorithms (in-order, pre-order, post-order)
- Graph search algorithms (BFS, DFS)
- Shortest path algorithms (Dijkstra’s, A*)
5. Dynamic Programming
This powerful technique is often used in optimization problems. Practice:
- Identifying overlapping subproblems
- Memoization and tabulation techniques
- Solving classic DP problems (e.g., knapsack, longest common subsequence)
6. Greedy Algorithms
Greedy approaches can solve many optimization problems efficiently. Focus on:
- Understanding when greedy solutions are applicable
- Implementing common greedy algorithms (e.g., Huffman coding, Dijkstra’s)
- Proving the correctness of greedy solutions
Implementing a Sample Algorithm
To illustrate the importance of practice, let’s implement a simple yet fundamental algorithm: binary search. This algorithm efficiently finds an element in a sorted array.
Here’s a Python implementation of binary search:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
# Example usage
sorted_array = [1, 3, 5, 7, 9, 11, 13, 15, 17]
target = 7
result = binary_search(sorted_array, target)
if result != -1:
print(f"Element {target} found at index {result}")
else:
print(f"Element {target} not found in the array")
This implementation demonstrates several key concepts:
- Efficient searching in a sorted array
- Use of pointers (left and right) to narrow down the search space
- Logarithmic time complexity, making it much faster than linear search for large datasets
By practicing implementations like this and variations of search algorithms, you’ll develop a deeper understanding of algorithmic principles and improve your coding skills.
The Road to Algorithm Mastery
Mastering algorithms is a journey that requires dedication, consistent practice, and a structured approach. Here’s a roadmap to guide your path to algorithm mastery:
1. Build a Strong Foundation
- Start with basic data structures (arrays, linked lists, stacks, queues)
- Learn fundamental algorithms (sorting, searching, basic graph algorithms)
- Understand time and space complexity analysis
2. Practice Regularly
- Solve a mix of easy, medium, and hard problems
- Use platforms like AlgoCademy for structured practice
- Participate in coding contests to challenge yourself
3. Study Advanced Topics
- Dive deeper into advanced data structures (trees, heaps, hash tables)
- Master complex algorithmic paradigms (dynamic programming, divide and conquer)
- Explore specialized algorithms (e.g., for string processing, computational geometry)
4. Apply Your Knowledge
- Work on personal projects that involve algorithmic challenges
- Contribute to open-source projects to gain real-world experience
- Mentor others to reinforce your understanding
5. Stay Updated
- Follow developments in algorithm research
- Learn about industry-specific algorithmic applications
- Adapt to new programming languages and paradigms
Conclusion
The importance of practice tests in achieving algorithm mastery cannot be overstated. They provide a structured, hands-on approach to learning that bridges the gap between theoretical knowledge and practical application. Platforms like AlgoCademy offer invaluable resources for aspiring programmers and seasoned developers alike, providing a comprehensive ecosystem for skill development and interview preparation.
Remember that mastering algorithms is not just about acing technical interviews or landing a job at a top tech company. It’s about developing a problem-solving mindset, improving your coding efficiency, and expanding your capabilities as a programmer. The skills you gain through consistent practice will serve you well throughout your career, enabling you to tackle complex challenges and create innovative solutions.
As you embark on or continue your journey towards algorithm mastery, embrace the power of practice tests. Set goals, track your progress, and celebrate your achievements along the way. With dedication and the right resources, you’ll find yourself not just solving algorithmic problems, but truly understanding and appreciating the elegance and efficiency of well-designed algorithms.
Happy coding, and may your algorithm practice lead you to new heights in your programming career!