The Importance of Mastering the Fundamentals as a Self-Taught Developer
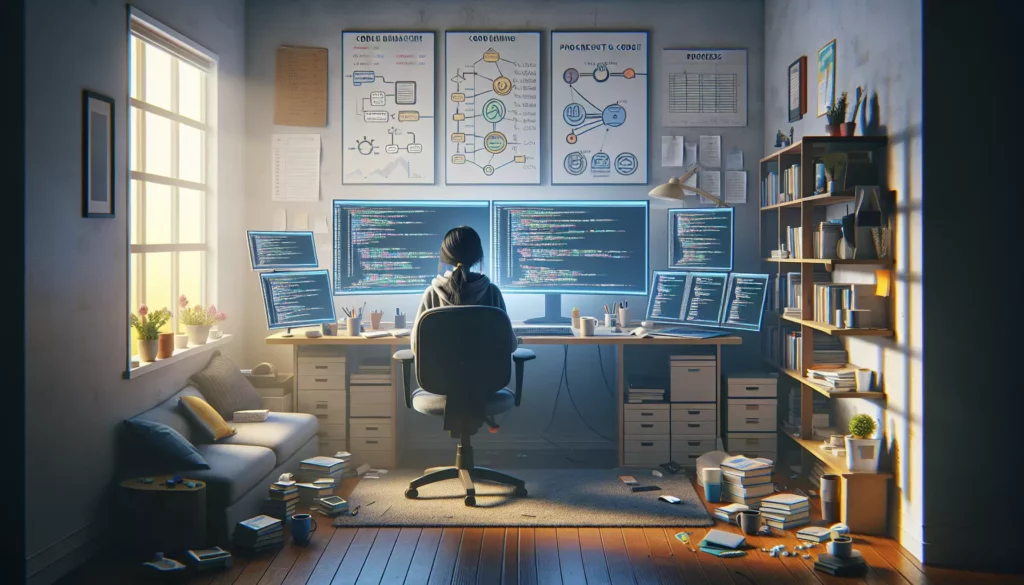
In the ever-evolving world of technology, becoming a self-taught developer has become an increasingly popular path for many aspiring programmers. With countless online resources, coding bootcamps, and platforms like AlgoCademy at your fingertips, it’s easier than ever to dive into the world of coding. However, amidst the excitement of learning new languages and frameworks, one crucial aspect often gets overlooked: mastering the fundamentals.
In this comprehensive guide, we’ll explore why mastering the fundamentals is essential for self-taught developers, how it can impact your career trajectory, and provide practical tips on how to build a solid foundation in programming.
Why Fundamentals Matter
Before we delve into the specifics, let’s understand why focusing on fundamentals is so crucial for self-taught developers:
- Long-term career success: A strong grasp of fundamentals allows you to adapt to new technologies and languages more easily.
- Problem-solving skills: Understanding core concepts helps you approach complex problems with confidence and creativity.
- Efficiency: Knowing the basics inside and out leads to writing cleaner, more efficient code.
- Interviews and job prospects: Many technical interviews focus on fundamental concepts, especially at major tech companies.
- Continuous learning: A solid foundation makes it easier to learn advanced topics and stay current in the field.
Key Fundamentals for Self-Taught Developers
Now that we understand the importance of fundamentals, let’s explore the key areas that self-taught developers should focus on:
1. Data Structures and Algorithms
Data structures and algorithms form the backbone of computer science and are crucial for writing efficient, scalable code. As a self-taught developer, it’s easy to overlook these concepts in favor of learning the latest framework or language. However, a deep understanding of data structures and algorithms can set you apart from other developers and is often a key focus in technical interviews.
Key concepts to master include:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Sorting and Searching Algorithms
- Dynamic Programming
- Big O Notation and Time Complexity Analysis
Platforms like AlgoCademy offer interactive tutorials and problem sets specifically designed to help you master these fundamental concepts. By working through algorithmic challenges and understanding the underlying principles, you’ll develop a problem-solving mindset that’s invaluable in real-world development scenarios.
2. Programming Paradigms
Understanding different programming paradigms is crucial for becoming a well-rounded developer. While you may start with one paradigm, being familiar with others will broaden your perspective and allow you to choose the best approach for any given problem.
Key paradigms to explore include:
- Procedural Programming
- Object-Oriented Programming (OOP)
- Functional Programming
- Declarative Programming
Let’s take a closer look at Object-Oriented Programming, as it’s widely used in many modern programming languages:
// Example of a simple class in Python
class Car:
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
self.speed = 0
def accelerate(self, increment):
self.speed += increment
def brake(self, decrement):
self.speed = max(0, self.speed - decrement)
def get_info(self):
return f"{self.year} {self.make} {self.model}, current speed: {self.speed} mph"
# Creating an instance of the Car class
my_car = Car("Toyota", "Corolla", 2022)
my_car.accelerate(30)
print(my_car.get_info()) # Output: 2022 Toyota Corolla, current speed: 30 mph
This example demonstrates key OOP concepts such as classes, objects, methods, and encapsulation. Understanding these paradigms will help you write more organized, maintainable code and choose the right approach for different projects.
3. Version Control
Version control is a fundamental skill that every developer, self-taught or otherwise, must master. Git is the most widely used version control system, and understanding its concepts and commands is crucial for collaborating with other developers and managing your own projects.
Key Git concepts to learn include:
- Basic commands (init, add, commit, push, pull)
- Branching and merging
- Resolving conflicts
- Working with remote repositories
- Git workflows (e.g., Git Flow, GitHub Flow)
Here’s a basic example of using Git commands:
# Initialize a new Git repository
git init
# Add files to the staging area
git add .
# Commit changes
git commit -m "Initial commit"
# Create and switch to a new branch
git checkout -b feature-branch
# Make changes and commit
git add .
git commit -m "Add new feature"
# Switch back to the main branch
git checkout main
# Merge the feature branch
git merge feature-branch
# Push changes to a remote repository
git push origin main
Mastering version control will not only help you manage your own projects more effectively but also prepare you for collaborative work environments.
4. Database Fundamentals
While not all developers work directly with databases, understanding database concepts is crucial for building robust applications. Whether you’re working with relational databases like MySQL or PostgreSQL, or NoSQL databases like MongoDB, grasping the fundamentals will serve you well.
Key database concepts to learn include:
- Relational vs. NoSQL databases
- Basic SQL queries (SELECT, INSERT, UPDATE, DELETE)
- Database design and normalization
- Indexing and query optimization
- Transactions and ACID properties
Here’s a simple example of a SQL query:
-- Create a table
CREATE TABLE users (
id INT PRIMARY KEY,
username VARCHAR(50) NOT NULL,
email VARCHAR(100) UNIQUE,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
-- Insert data
INSERT INTO users (id, username, email) VALUES (1, 'johndoe', 'john@example.com');
-- Query data
SELECT * FROM users WHERE username = 'johndoe';
Understanding database fundamentals will help you design more efficient data models and write optimized queries, skills that are valuable in many development roles.
5. Web Technologies and Protocols
For many self-taught developers, web development is often the entry point into programming. However, it’s crucial to understand the underlying technologies and protocols that power the web, rather than just focusing on specific frameworks or libraries.
Key web fundamentals to master include:
- HTML, CSS, and JavaScript
- HTTP/HTTPS protocols
- RESTful API design
- Web security basics (CORS, XSS, CSRF)
- Browser rendering and the Document Object Model (DOM)
- Responsive design principles
Here’s a simple example of a RESTful API endpoint using Express.js:
const express = require('express');
const app = express();
app.get('/api/users', (req, res) => {
// In a real application, this data would come from a database
const users = [
{ id: 1, name: 'John Doe' },
{ id: 2, name: 'Jane Smith' }
];
res.json(users);
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Understanding these fundamentals will give you a deeper appreciation of how web applications work and help you build more robust, scalable web solutions.
Strategies for Mastering Fundamentals
Now that we’ve covered the key areas to focus on, let’s explore some strategies for effectively mastering these fundamentals:
1. Consistent Practice
Consistency is key when it comes to learning programming fundamentals. Set aside dedicated time each day or week to work on fundamental concepts. Platforms like AlgoCademy offer structured learning paths and daily coding challenges that can help you maintain a consistent practice routine.
2. Build Projects
Apply your knowledge by building projects from scratch. This hands-on approach will help you understand how different concepts work together in real-world scenarios. Start with simple projects and gradually increase complexity as you grow more confident.
3. Participate in Coding Challenges
Websites like LeetCode, HackerRank, and CodeWars offer coding challenges that test your problem-solving skills and understanding of data structures and algorithms. Regularly participating in these challenges can sharpen your skills and prepare you for technical interviews.
4. Read and Analyze Code
Reading and understanding other people’s code is a valuable skill. Contribute to open-source projects or review code on platforms like GitHub. This practice will expose you to different coding styles and help you learn best practices.
5. Teach Others
Teaching is one of the best ways to solidify your understanding of a concept. Start a blog, create video tutorials, or mentor other beginners. Explaining concepts to others will highlight any gaps in your knowledge and deepen your understanding.
6. Leverage Interactive Learning Platforms
Utilize interactive learning platforms like AlgoCademy that offer hands-on coding experiences, immediate feedback, and personalized learning paths. These platforms can provide a structured approach to mastering fundamentals, often with features like AI-powered assistance to help you overcome challenges.
Overcoming Common Challenges
As a self-taught developer focusing on fundamentals, you may encounter several challenges. Here’s how to overcome them:
1. Information Overload
With the vast amount of resources available, it’s easy to feel overwhelmed. Combat this by creating a structured learning plan and focusing on one concept at a time. Use platforms like AlgoCademy that offer curated learning paths to guide your studies.
2. Lack of Formal Structure
Without the structure of a formal education program, it can be challenging to know what to learn next. Overcome this by following curriculum outlines from reputable computer science programs or using structured learning platforms designed for self-taught developers.
3. Imposter Syndrome
Many self-taught developers struggle with feelings of inadequacy. Remember that even experienced developers continually learn and improve. Focus on your progress and celebrate small victories along the way.
4. Balancing Theory and Practice
While understanding theory is important, it’s equally crucial to apply what you learn. Strike a balance by implementing theoretical concepts in practical projects or coding challenges immediately after learning them.
5. Staying Motivated
Maintaining motivation over the long term can be challenging. Set achievable goals, track your progress, and remind yourself of your long-term objectives. Joining coding communities or finding a mentor can also provide support and accountability.
The Road to Mastery: A Lifelong Journey
Mastering the fundamentals as a self-taught developer is not a one-time achievement but a continuous process. As you progress in your career, you’ll find that revisiting and deepening your understanding of these core concepts will consistently improve your skills and open new opportunities.
Remember that even experienced developers regularly review and refine their understanding of fundamentals. It’s this ongoing commitment to the basics that often separates good developers from great ones.
As you continue your journey, consider these final tips:
- Stay curious: Always be open to learning new concepts and questioning your assumptions.
- Embrace challenges: Don’t shy away from difficult problems; they’re opportunities for growth.
- Network: Connect with other developers, attend meetups, and participate in online communities to share knowledge and stay motivated.
- Keep up with industry trends: While focusing on fundamentals, stay aware of new technologies and how they relate to core concepts.
- Reflect on your learning: Regularly assess your progress and adjust your learning strategies as needed.
By dedicating yourself to mastering the fundamentals, you’re laying a solid foundation for a successful and fulfilling career in software development. Whether you’re aiming to land your first job, advance in your current role, or prepare for technical interviews at major tech companies, a strong grasp of these core concepts will serve you well.
Platforms like AlgoCademy can be invaluable resources on this journey, offering structured paths to master algorithms, data structures, and problem-solving skills. By combining these resources with consistent practice, project-based learning, and a commitment to continuous improvement, you’ll be well-equipped to tackle any challenge that comes your way in the dynamic world of software development.
Remember, every expert was once a beginner. With dedication, persistence, and a focus on the fundamentals, you have the power to transform yourself into the developer you aspire to be. Embrace the journey, celebrate your progress, and never stop learning. The world of technology is vast and ever-changing, but with a solid foundation in the fundamentals, you’ll be ready to adapt, innovate, and excel in whatever direction your career takes you.