The Importance of Learning Object-Oriented Programming
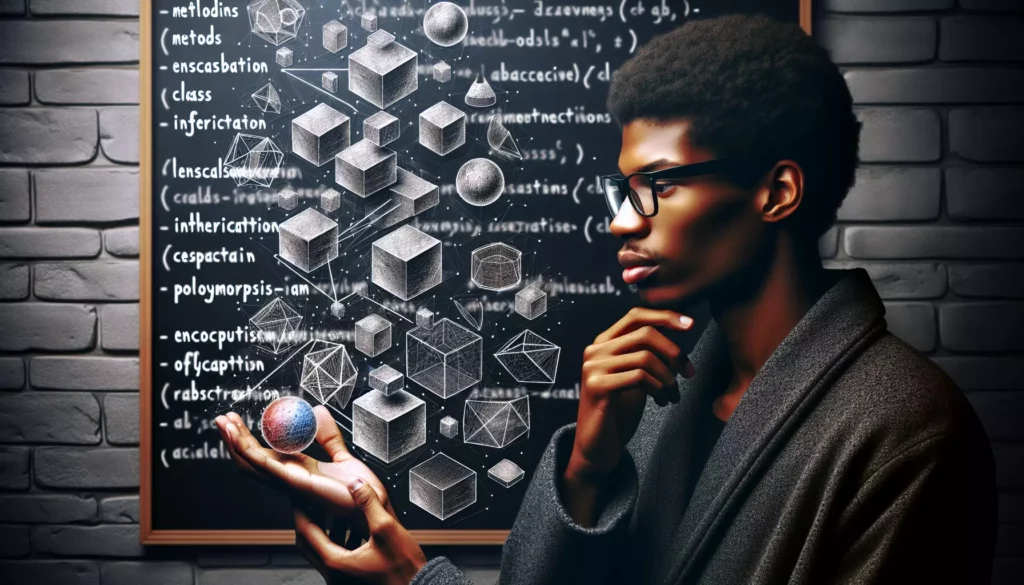
In the ever-evolving world of software development, Object-Oriented Programming (OOP) stands as a cornerstone of modern coding practices. As aspiring developers and seasoned programmers alike navigate the complex landscape of programming paradigms, understanding and mastering OOP has become increasingly crucial. This comprehensive guide will delve into the importance of learning Object-Oriented Programming, its fundamental concepts, and how it can significantly enhance your coding skills and career prospects.
What is Object-Oriented Programming?
Object-Oriented Programming is a programming paradigm based on the concept of “objects,” which can contain data in the form of fields (often known as attributes or properties) and code in the form of procedures (often known as methods). OOP is designed to make it easier to develop, debug, reuse, and maintain software.
The four main principles of OOP are:
- Encapsulation: Bundling data and methods that operate on that data within a single unit (class).
- Inheritance: Allowing new classes to be based on existing classes, inheriting their properties and methods.
- Polymorphism: The ability of different classes to be treated as instances of the same class through inheritance.
- Abstraction: Hiding complex implementation details and showing only the necessary features of an object.
Why is Learning OOP Important?
1. Improved Code Organization and Structure
One of the primary benefits of OOP is its ability to organize code into reusable, modular components. This organization leads to cleaner, more maintainable code structures. By encapsulating related data and behaviors into objects, developers can create more intuitive and logical program architectures.
For example, consider a simple banking application. Using OOP, we can create a BankAccount
class:
class BankAccount:
def __init__(self, account_number, balance):
self.account_number = account_number
self.balance = balance
def deposit(self, amount):
self.balance += amount
def withdraw(self, amount):
if self.balance >= amount:
self.balance -= amount
return True
return False
def get_balance(self):
return self.balance
This structure neatly organizes all the data and operations related to a bank account into a single, cohesive unit.
2. Code Reusability and Reduced Redundancy
OOP promotes the reuse of code through inheritance and polymorphism. This reusability not only saves time but also reduces the likelihood of errors that can occur when duplicating code. By creating a hierarchy of classes, developers can write code once and reuse it multiple times.
Extending our banking example, we can create specialized account types:
class SavingsAccount(BankAccount):
def __init__(self, account_number, balance, interest_rate):
super().__init__(account_number, balance)
self.interest_rate = interest_rate
def apply_interest(self):
interest = self.balance * (self.interest_rate / 100)
self.deposit(interest)
class CheckingAccount(BankAccount):
def __init__(self, account_number, balance, overdraft_limit):
super().__init__(account_number, balance)
self.overdraft_limit = overdraft_limit
def withdraw(self, amount):
if self.balance + self.overdraft_limit >= amount:
self.balance -= amount
return True
return False
Here, both SavingsAccount
and CheckingAccount
inherit from BankAccount
, reusing its core functionality while adding their own specialized features.
3. Easier Maintenance and Debugging
The modular nature of OOP makes it easier to isolate and fix issues within specific components of a program. When bugs occur, developers can focus on the relevant objects and their interactions, rather than sifting through monolithic code blocks. This compartmentalization also allows for easier updates and modifications to specific parts of the program without affecting the entire system.
4. Better Modeling of Real-World Scenarios
OOP allows developers to create more intuitive representations of real-world entities and their relationships. This natural mapping between code structure and real-world concepts makes it easier to design and understand complex systems. For instance, in a game development scenario, objects like characters, items, and environments can be modeled as classes with their own properties and behaviors.
5. Enhanced Collaboration and Teamwork
The modular nature of OOP facilitates better collaboration among team members. Different team members can work on different classes or modules simultaneously without interfering with each other’s work. This parallel development process can significantly speed up project timelines and improve overall productivity.
6. Industry Standard and Job Market Demand
OOP is widely used in the software industry, and proficiency in OOP concepts is often a requirement for many programming jobs. Languages like Java, C++, Python, and C# heavily rely on OOP principles. Understanding OOP can significantly enhance your employability and career prospects in the tech industry.
Key OOP Concepts to Master
1. Classes and Objects
Classes are the blueprints for creating objects. They define the structure and behavior that the objects of that class will have. Objects are instances of classes, representing specific entities with their own unique data.
Example in Python:
class Car:
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
def display_info(self):
return f"{self.year} {self.make} {self.model}"
# Creating an object
my_car = Car("Toyota", "Corolla", 2022)
print(my_car.display_info()) # Output: 2022 Toyota Corolla
2. Encapsulation
Encapsulation is the bundling of data and the methods that operate on that data within a single unit (class). It also involves restricting direct access to some of an object’s components, which is a means of preventing accidental interference and misuse of the methods and data.
Example in Python:
class BankAccount:
def __init__(self, balance):
self.__balance = balance # Private attribute
def deposit(self, amount):
if amount > 0:
self.__balance += amount
def withdraw(self, amount):
if 0 < amount <= self.__balance:
self.__balance -= amount
def get_balance(self):
return self.__balance
account = BankAccount(1000)
account.deposit(500)
print(account.get_balance()) # Output: 1500
# print(account.__balance) # This would raise an AttributeError
3. Inheritance
Inheritance allows a new class to be based on an existing class, inheriting its properties and methods. This promotes code reuse and establishes a relationship between parent and child classes.
Example in Python:
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
pass
class Dog(Animal):
def speak(self):
return f"{self.name} says Woof!"
class Cat(Animal):
def speak(self):
return f"{self.name} says Meow!"
dog = Dog("Buddy")
cat = Cat("Whiskers")
print(dog.speak()) # Output: Buddy says Woof!
print(cat.speak()) # Output: Whiskers says Meow!
4. Polymorphism
Polymorphism allows objects of different classes to be treated as objects of a common base class. It enables the use of a single interface to represent different underlying forms (data types or classes).
Example in Python:
def animal_sound(animal):
return animal.speak()
# Using the Animal, Dog, and Cat classes from the previous example
dog = Dog("Rex")
cat = Cat("Luna")
print(animal_sound(dog)) # Output: Rex says Woof!
print(animal_sound(cat)) # Output: Luna says Meow!
5. Abstraction
Abstraction involves hiding complex implementation details and showing only the necessary features of an object. It helps in managing complexity by hiding unnecessary details from the user.
Example in Python using abstract base classes:
from abc import ABC, abstractmethod
class Shape(ABC):
@abstractmethod
def area(self):
pass
class Rectangle(Shape):
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def area(self):
return 3.14 * self.radius ** 2
# Usage
rectangle = Rectangle(5, 4)
circle = Circle(3)
print(rectangle.area()) # Output: 20
print(circle.area()) # Output: 28.26
Practical Applications of OOP
Understanding the importance of OOP becomes even clearer when we look at its practical applications in various domains of software development:
1. Web Development
In web development, OOP principles are extensively used in frameworks like Django (Python), Ruby on Rails, and ASP.NET. These frameworks use OOP to create modular, reusable components for building web applications. For instance, in Django, models (which represent database tables) are defined as classes, and views (which handle HTTP requests) can be written as class-based views.
2. Game Development
Game development heavily relies on OOP concepts. Games often involve complex systems with many interacting entities, such as characters, items, and environments. OOP allows developers to model these entities as objects with their own properties and behaviors. For example, in a role-playing game, you might have a base Character
class, with subclasses like Warrior
, Mage
, and Archer
, each with their unique abilities.
3. Mobile App Development
Mobile app frameworks like Android (Java/Kotlin) and iOS (Swift) are built around OOP principles. In these frameworks, UI components, data models, and controllers are typically represented as objects. For instance, in Android development, activities and fragments (which represent screens or portions of screens) are classes that developers extend to create custom behavior.
4. Desktop Application Development
Desktop application frameworks such as JavaFX, Qt (C++), and Windows Presentation Foundation (C#) use OOP to create user interfaces and manage application logic. These frameworks often use a Model-View-Controller (MVC) or Model-View-ViewModel (MVVM) architecture, which are patterns that heavily rely on OOP concepts.
5. Data Science and Machine Learning
While data science and machine learning often use functional programming paradigms, OOP still plays a crucial role. Libraries like scikit-learn in Python use OOP to create consistent interfaces for various machine learning algorithms. For example, all classifiers in scikit-learn have fit()
and predict()
methods, making it easy to switch between different algorithms.
OOP and Software Design Patterns
One of the most significant advantages of mastering OOP is the ability to implement and understand software design patterns. Design patterns are typical solutions to common problems in software design. They are templates for how to solve a problem that can be used in many different situations. Many of these patterns are based on OOP principles:
1. Singleton Pattern
This pattern ensures a class has only one instance and provides a global point of access to it. It’s useful for managing shared resources, such as a database connection pool.
class Singleton:
_instance = None
def __new__(cls):
if cls._instance is None:
cls._instance = super(Singleton, cls).__new__(cls)
return cls._instance
# Usage
s1 = Singleton()
s2 = Singleton()
print(s1 is s2) # Output: True
2. Factory Pattern
This pattern provides an interface for creating objects in a superclass, but allows subclasses to alter the type of objects that will be created.
from abc import ABC, abstractmethod
class Animal(ABC):
@abstractmethod
def speak(self):
pass
class Dog(Animal):
def speak(self):
return "Woof!"
class Cat(Animal):
def speak(self):
return "Meow!"
class AnimalFactory:
def create_animal(self, animal_type):
if animal_type == "dog":
return Dog()
elif animal_type == "cat":
return Cat()
else:
raise ValueError("Unknown animal type")
# Usage
factory = AnimalFactory()
dog = factory.create_animal("dog")
cat = factory.create_animal("cat")
print(dog.speak()) # Output: Woof!
print(cat.speak()) # Output: Meow!
3. Observer Pattern
This pattern defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically.
class Subject:
def __init__(self):
self._observers = []
self._state = None
def attach(self, observer):
self._observers.append(observer)
def detach(self, observer):
self._observers.remove(observer)
def notify(self):
for observer in self._observers:
observer.update(self._state)
def set_state(self, state):
self._state = state
self.notify()
class Observer:
def update(self, state):
pass
class ConcreteObserver(Observer):
def update(self, state):
print(f"State changed to: {state}")
# Usage
subject = Subject()
observer1 = ConcreteObserver()
observer2 = ConcreteObserver()
subject.attach(observer1)
subject.attach(observer2)
subject.set_state("New State")
# Output:
# State changed to: New State
# State changed to: New State
Challenges in Learning OOP
While learning OOP offers numerous benefits, it also comes with its own set of challenges:
1. Conceptual Complexity
OOP introduces abstract concepts like classes, objects, inheritance, and polymorphism, which can be challenging for beginners to grasp. It requires a shift in thinking from procedural programming to thinking in terms of objects and their interactions.
2. Design Decisions
Deciding how to structure classes and their relationships can be difficult, especially for complex systems. Poor design decisions can lead to inflexible or hard-to-maintain code.
3. Overuse of Inheritance
While inheritance is a powerful feature, overusing it can lead to complex class hierarchies that are difficult to understand and maintain. This is often referred to as the “diamond problem” in languages that support multiple inheritance.
4. Performance Overhead
In some cases, OOP can introduce performance overhead due to the additional layers of abstraction. This is generally not a significant issue with modern hardware and optimized runtimes, but it can be a concern in performance-critical applications.
Overcoming OOP Learning Challenges
To overcome these challenges and effectively learn OOP, consider the following strategies:
1. Start with Simple Projects
Begin with small, manageable projects that demonstrate OOP concepts. As you gain confidence, gradually increase the complexity of your projects.
2. Practice Regularly
Consistent practice is key to mastering OOP. Try to implement OOP concepts in your daily coding tasks, even if it’s not strictly necessary. This will help reinforce your understanding.
3. Study Design Patterns
Familiarize yourself with common design patterns. They provide tested solutions to recurring design problems and can help you make better design decisions.
4. Code Review and Collaboration
Engage in code reviews with more experienced developers. Their feedback can provide valuable insights into best practices and potential improvements in your OOP designs.
5. Refactor Existing Code
Practice refactoring procedural code into object-oriented code. This exercise can help you understand the benefits of OOP and how to apply its principles effectively.
Conclusion
Learning Object-Oriented Programming is not just about mastering a programming paradigm; it’s about adopting a powerful approach to software design and problem-solving. The importance of OOP in modern software development cannot be overstated. It provides a structured approach to creating scalable, maintainable, and reusable code – skills that are highly valued in the software industry.
As you embark on your journey to master OOP, remember that it’s a gradual process. Start with the basics, practice regularly, and don’t be afraid to make mistakes. Each challenge you overcome will deepen your understanding and make you a more proficient programmer.
In the context of coding education platforms like AlgoCademy, understanding OOP is crucial. It forms the foundation for advanced topics in software development, algorithm design, and even in preparing for technical interviews at major tech companies. By mastering OOP, you’re not just learning a programming style; you’re equipping yourself with a mindset that will serve you throughout your programming career.
Whether you’re aiming to develop complex software systems, create intuitive user interfaces, or dive into emerging fields like artificial intelligence and machine learning, a solid grounding in OOP will be invaluable. So, embrace the challenge, practice diligently, and watch as the world of software development opens up before you, one object at a time.