The Importance of Learning JavaScript in the Modern Development Ecosystem
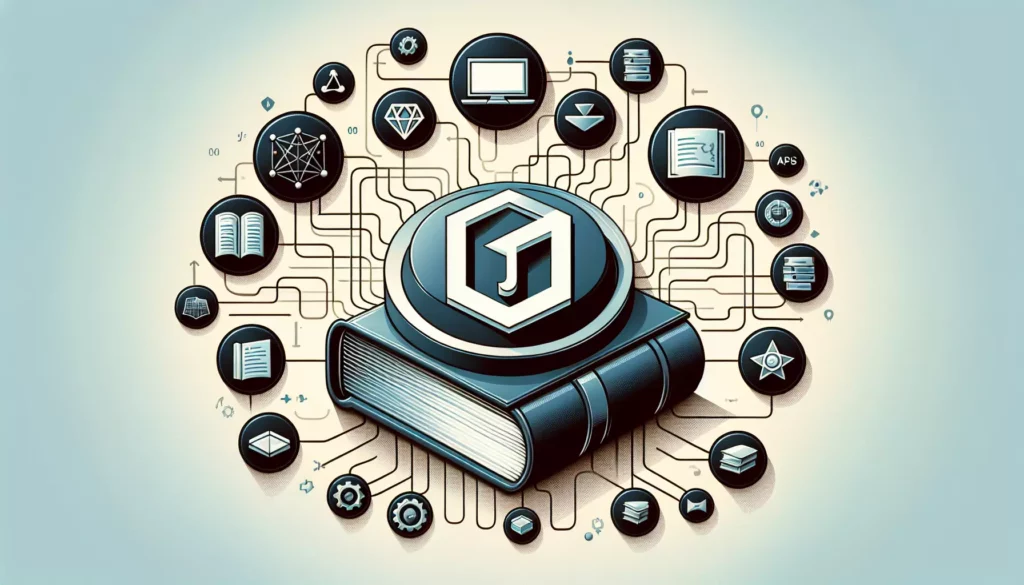
In today’s rapidly evolving digital landscape, JavaScript has emerged as a cornerstone of web development and beyond. Its versatility, widespread adoption, and continuous evolution make it an essential language for aspiring developers and seasoned professionals alike. This article delves into the significance of JavaScript in the modern development ecosystem, exploring its impact, applications, and why mastering this language is crucial for success in the tech industry.
The Rise of JavaScript: A Brief History
JavaScript was created by Brendan Eich in 1995 while he was working at Netscape Communications. Initially designed as a simple scripting language for web browsers, JavaScript has since grown into a powerful, multi-paradigm language that powers much of the modern web and beyond. Let’s take a look at some key milestones in JavaScript’s history:
- 1995: JavaScript is created and implemented in Netscape Navigator
- 1996: Microsoft releases JScript, its own version of JavaScript
- 1997: ECMAScript 1 is released, standardizing the language
- 2009: Node.js is created, allowing JavaScript to run on the server-side
- 2015: ECMAScript 6 (ES6) is released, introducing significant new features
- 2022: ECMAScript 2022, the latest version, is published
This evolution has transformed JavaScript from a simple client-side scripting language into a versatile tool for building complex web applications, server-side programming, mobile app development, and even desktop applications.
Why JavaScript is Essential in Modern Development
1. Ubiquity in Web Development
JavaScript is the only programming language natively supported by all major web browsers. This ubiquity makes it an indispensable tool for front-end web development. Whether you’re building a simple website or a complex web application, JavaScript is essential for creating interactive and dynamic user interfaces.
2. Full-Stack Development with Node.js
The introduction of Node.js in 2009 revolutionized the JavaScript ecosystem by allowing developers to use JavaScript on the server-side. This breakthrough enabled full-stack JavaScript development, where a single language can be used for both client-side and server-side programming. This consistency across the stack can lead to increased productivity and easier code maintenance.
3. Rich Ecosystem and Libraries
JavaScript boasts one of the largest and most active developer communities in the world. This has resulted in a vast ecosystem of libraries, frameworks, and tools that can significantly speed up development and solve complex problems. Popular frameworks and libraries include:
- React.js for building user interfaces
- Angular for creating dynamic web applications
- Vue.js for flexible front-end development
- Express.js for building web applications on Node.js
- Three.js for 3D graphics
- TensorFlow.js for machine learning in the browser
4. Mobile App Development
JavaScript has become a popular choice for mobile app development, thanks to frameworks like React Native and Ionic. These tools allow developers to create native mobile applications using JavaScript, significantly reducing development time and costs by enabling code reuse across different platforms.
5. Desktop Application Development
With frameworks like Electron, JavaScript can be used to build cross-platform desktop applications. Popular applications like Visual Studio Code, Atom, and Discord are built using Electron, showcasing the power and flexibility of JavaScript beyond the web.
6. Internet of Things (IoT)
JavaScript’s versatility extends to the Internet of Things. Platforms like Johnny-Five allow developers to program IoT devices using JavaScript, making it easier for web developers to transition into this growing field.
7. Constantly Evolving Language
JavaScript continues to evolve with annual ECMAScript updates. These updates introduce new features and improvements, keeping the language modern and relevant. This constant evolution ensures that JavaScript remains at the forefront of programming languages, adapting to new development paradigms and industry needs.
Key JavaScript Concepts Every Developer Should Know
To truly harness the power of JavaScript, developers need to master several key concepts. Here are some fundamental areas to focus on:
1. Variables and Data Types
Understanding how to declare variables using var
, let
, and const
, as well as grasping JavaScript’s dynamic typing system, is crucial. JavaScript has several primitive data types (such as number, string, boolean) and complex data types (like objects and arrays) that developers must be comfortable working with.
2. Functions and Scope
Functions are first-class citizens in JavaScript, meaning they can be assigned to variables, passed as arguments, and returned from other functions. Understanding function declarations, expressions, and arrow functions is essential. Additionally, grasping the concept of scope (global, function, and block scope) is crucial for writing efficient and bug-free code.
3. Object-Oriented Programming (OOP) in JavaScript
While JavaScript is a multi-paradigm language, it has strong support for object-oriented programming. Developers should be familiar with creating and working with objects, prototypal inheritance, and the newer class syntax introduced in ES6.
4. Asynchronous Programming
Asynchronous programming is a cornerstone of JavaScript, especially in web development. Understanding callbacks, promises, and async/await is crucial for handling operations like API calls, file I/O, and other time-consuming tasks without blocking the main thread.
5. DOM Manipulation
For front-end development, understanding how to interact with the Document Object Model (DOM) is essential. This includes selecting elements, modifying content and attributes, and handling events.
6. ES6+ Features
Staying up-to-date with the latest JavaScript features is important. Some key ES6+ features to master include:
- Arrow functions
- Template literals
- Destructuring assignment
- Spread and rest operators
- Modules (import/export)
- Classes
- Promises and async/await
7. Functional Programming Concepts
JavaScript supports functional programming paradigms. Understanding concepts like pure functions, immutability, and higher-order functions can lead to cleaner, more maintainable code.
JavaScript in the Context of AlgoCademy
At AlgoCademy, we recognize the critical role JavaScript plays in modern software development. Our platform is designed to help learners master JavaScript along with other essential programming skills. Here’s how we approach JavaScript education:
1. Interactive Coding Tutorials
Our platform offers hands-on, interactive JavaScript tutorials that cover everything from basic syntax to advanced concepts. These tutorials allow learners to write and execute code in real-time, providing immediate feedback and reinforcing learning.
2. Problem-Solving and Algorithmic Thinking
We emphasize the importance of problem-solving skills and algorithmic thinking in our JavaScript curriculum. Through carefully crafted coding challenges, learners develop the ability to break down complex problems and implement efficient solutions using JavaScript.
3. Real-World Projects
To bridge the gap between theory and practice, we offer real-world project tutorials that simulate actual development scenarios. These projects cover various aspects of JavaScript development, including front-end frameworks, Node.js applications, and full-stack development.
4. Technical Interview Preparation
Given JavaScript’s popularity in technical interviews, especially for front-end and full-stack roles, we provide specialized resources to help learners prepare for coding interviews at top tech companies. This includes JavaScript-specific data structure and algorithm challenges, as well as system design questions relevant to JavaScript ecosystems.
5. AI-Powered Assistance
Our AI-powered coding assistant provides personalized help and feedback as learners work through JavaScript challenges. This feature helps identify areas for improvement and offers tailored suggestions to enhance coding skills.
6. Community and Collaboration
We foster a community of JavaScript learners and professionals where members can share knowledge, collaborate on projects, and seek help. This collaborative environment enhances the learning experience and helps build a network of like-minded developers.
JavaScript Best Practices and Tips for Efficient Development
As you embark on your JavaScript learning journey, it’s crucial to adopt best practices that will help you write clean, efficient, and maintainable code. Here are some tips to keep in mind:
1. Use Strict Mode
Enable strict mode in your JavaScript files by adding "use strict";
at the beginning of your scripts or functions. This helps catch common coding errors and prevents the use of certain error-prone features.
"use strict";
function exampleFunction() {
// Your code here
}
2. Follow a Consistent Naming Convention
Adopt a consistent naming convention for variables, functions, and classes. The most common conventions in JavaScript are camelCase for variables and functions, and PascalCase for classes.
// Variables and functions
let userName = "John Doe";
function calculateTotal() { /* ... */ }
// Classes
class UserProfile { /* ... */ }
3. Use Meaningful Variable and Function Names
Choose descriptive names that clearly indicate the purpose or content of variables and functions. This improves code readability and makes it easier for others (including your future self) to understand your code.
// Bad
let x = 5;
// Good
let numberOfAttempts = 5;
// Bad
function do_stuff() { /* ... */ }
// Good
function validateUserInput() { /* ... */ }
4. Avoid Global Variables
Minimize the use of global variables to prevent naming conflicts and unexpected behavior. Instead, use local variables and pass them as parameters when needed.
5. Use Modern JavaScript Features
Take advantage of modern JavaScript features like let
and const
for variable declarations, arrow functions for concise function syntax, and template literals for string interpolation.
// Using let and const
const PI = 3.14159;
let radius = 5;
// Arrow function
const calculateArea = (r) => PI * r * r;
// Template literal
console.log(`The area of the circle is ${calculateArea(radius)}`);
6. Handle Errors Properly
Use try-catch blocks to handle exceptions and prevent your application from crashing. This is especially important when working with asynchronous operations.
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
return data;
} catch (error) {
console.error('Error fetching data:', error);
// Handle the error appropriately
}
}
7. Use Modules for Code Organization
Organize your code into modules using the ES6 module syntax. This helps in maintaining a clean and modular codebase, making it easier to manage and scale your projects.
// math.js
export function add(a, b) {
return a + b;
}
// main.js
import { add } from './math.js';
console.log(add(5, 3)); // Output: 8
8. Optimize Performance
Be mindful of performance when writing JavaScript. Some tips include:
- Avoid unnecessary DOM manipulations
- Use efficient data structures and algorithms
- Minimize the use of global variables
- Use debouncing or throttling for frequent events like scrolling or resizing
9. Write Self-Documenting Code
Strive to write code that is self-explanatory. Use clear variable names, break complex operations into smaller, well-named functions, and add comments only when necessary to explain complex logic or algorithms.
10. Stay Updated
Keep yourself updated with the latest JavaScript features and best practices. The language evolves rapidly, and staying current will help you write more efficient and modern code.
The Future of JavaScript
As we look to the future, JavaScript’s role in the development ecosystem is likely to continue growing and evolving. Here are some trends and areas to watch:
1. WebAssembly Integration
WebAssembly (Wasm) is gaining traction as a way to run high-performance code in the browser. JavaScript’s integration with WebAssembly is likely to increase, allowing developers to combine the flexibility of JavaScript with the performance of lower-level languages.
2. Artificial Intelligence and Machine Learning
Libraries like TensorFlow.js are bringing machine learning capabilities to the browser. As AI and ML become more prevalent, JavaScript’s role in implementing these technologies on the client-side is expected to grow.
3. Progressive Web Apps (PWAs)
PWAs, which offer app-like experiences in the browser, are becoming increasingly popular. JavaScript plays a crucial role in building PWAs, and this trend is likely to continue.
4. Serverless Architecture
Serverless computing is gaining popularity, and JavaScript is well-suited for writing serverless functions. This trend is likely to drive further adoption of JavaScript in backend development.
5. Continued Evolution of the Language
JavaScript will continue to evolve with new features and improvements. Staying updated with these changes will be crucial for developers to write modern, efficient code.
Conclusion
Learning JavaScript is not just about mastering a programming language; it’s about equipping yourself with a versatile tool that opens doors to numerous opportunities in the tech industry. From web development to server-side programming, mobile apps to IoT, JavaScript’s reach is vast and continually expanding.
At AlgoCademy, we’re committed to helping you navigate this exciting landscape. Our platform provides the resources, guidance, and practice you need to master JavaScript and prepare for a successful career in tech. Whether you’re a beginner taking your first steps in coding or an experienced developer looking to sharpen your skills, JavaScript proficiency is a valuable asset in your programming toolkit.
Remember, the journey of learning JavaScript is ongoing. The language and its ecosystem are constantly evolving, presenting new challenges and opportunities. Embrace this dynamic nature, stay curious, and never stop learning. With dedication and practice, you’ll be well-equipped to tackle the most complex programming challenges and build innovative solutions that shape the digital world.
So, dive in, start coding, and unlock the full potential of JavaScript in your development journey. The world of modern software development awaits, and with JavaScript in your skillset, you’re well-prepared to make your mark.