The Importance of Full Stops in Programming: Why Every Character Matters
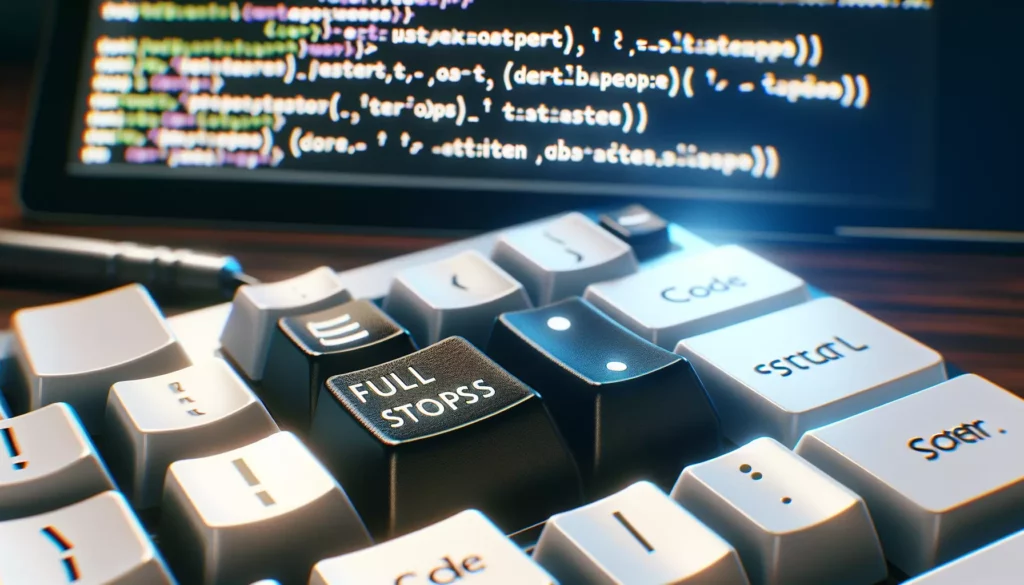
In the world of programming, every character matters. From curly braces to semicolons, each symbol plays a crucial role in creating functional and error-free code. Among these characters, the humble full stop (also known as a period or dot) holds a special place. While it may seem insignificant at first glance, the full stop is a powerful tool that can make or break your code. In this comprehensive guide, we’ll explore the importance of full stops in programming and why paying attention to every character is essential for success.
The Role of Full Stops in Programming Languages
Full stops serve various purposes in different programming languages. Let’s examine some of the most common uses:
1. Object-Oriented Programming: Accessing Methods and Properties
In object-oriented programming languages like Java, Python, and C#, the full stop is used to access methods and properties of objects. This notation is known as dot notation.
For example, in Python:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greet(self):
print(f"Hello, my name is {self.name} and I'm {self.age} years old.")
person = Person("Alice", 30)
person.greet() # Calls the greet() method of the Person object
print(person.name) # Accesses the name property of the Person object
In this example, the full stops are used to call the greet()
method and access the name
property of the person
object.
2. Package and Module Imports
Many programming languages use full stops to separate package and module names when importing or referencing them. This hierarchical structure helps organize code and prevent naming conflicts.
For instance, in Java:
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
List<String> names = new ArrayList<>();
names.add("Alice");
names.add("Bob");
System.out.println(names);
}
}
Here, the full stops in java.util.ArrayList
and java.util.List
indicate the package structure. The System.out.println()
method call also uses full stops to access the out
object and its println()
method.
3. File Extensions
While not directly related to code syntax, full stops are crucial in specifying file extensions. These extensions help identify the type of file and the programming language used.
Common examples include:
.py
for Python files.java
for Java source files.js
for JavaScript files.cpp
for C++ source files.html
for HTML documents
Using the correct file extension is essential for proper interpretation and execution of your code.
4. Decimal Points in Numeric Values
In most programming languages, full stops are used to represent decimal points in floating-point numbers.
For example, in JavaScript:
let pi = 3.14159;
let price = 19.99;
console.log(pi + price); // Output: 23.13159
Accurate representation of decimal numbers is crucial for mathematical operations and data processing in many applications.
The Consequences of Misplaced or Missing Full Stops
Now that we understand the importance of full stops in programming, let’s explore what can happen when they are misplaced or omitted.
1. Syntax Errors
One of the most common consequences of a missing or misplaced full stop is a syntax error. These errors prevent your code from compiling or running altogether.
Consider this Python example:
import random
def generate_random_number():
return random.randint(1, 100)
print(generate_random_number())
If we accidentally omit the full stop in random.randint()
, we’ll get a syntax error:
def generate_random_number():
return randomrandint(1, 100) # NameError: name 'randomrandint' is not defined
This error occurs because Python interprets randomrandint
as a single identifier, which doesn’t exist, instead of accessing the randint()
function from the random
module.
2. Incorrect Method or Property Access
Misplaced full stops can lead to accessing the wrong methods or properties, causing unexpected behavior in your program.
For instance, in JavaScript:
const user = {
name: "Alice",
age: 30,
greet: function() {
console.log(`Hello, I'm ${this.name}`);
}
};
user.greet(); // Correct: Outputs "Hello, I'm Alice"
user.name.greet(); // Incorrect: TypeError: user.name.greet is not a function
In this example, adding an extra full stop changes the context of the method call, resulting in an error because name
is a string and doesn’t have a greet()
method.
3. Unintended Object Creation
In some languages, like JavaScript, accidentally adding a full stop at the end of a statement can create an empty object, potentially leading to subtle bugs.
let x = 5.; // Creates an object instead of assigning the number 5
console.log(typeof x); // Output: "object"
let y = 5; // Correct assignment
console.log(typeof y); // Output: "number"
This behavior can be particularly tricky to debug, as it doesn’t always cause immediate errors but can lead to unexpected results in calculations or comparisons.
4. Import Errors
When working with modules and packages, incorrect placement of full stops can result in import errors.
For example, in Python:
from math import pi # Correct import
print(pi) # Output: 3.141592653589793
from math.pi import pi # Incorrect import
# ImportError: No module named 'math.pi'
In this case, adding an extra full stop changes the import statement’s meaning, causing Python to look for a non-existent module named math.pi
.
Best Practices for Using Full Stops in Programming
To avoid the pitfalls associated with misused full stops, follow these best practices:
1. Be Consistent with Naming Conventions
Adhere to the naming conventions of your chosen programming language. For example, in Python, use snake_case for function and variable names, and PascalCase for class names. This consistency helps prevent accidental misuse of full stops.
def calculate_average(numbers): # Correct
return sum(numbers) / len(numbers)
def calculate.average(numbers): # Incorrect
return sum(numbers) / len(numbers)
2. Use Code Linters and Formatters
Employ code linting tools and formatters specific to your programming language. These tools can automatically detect and sometimes fix issues related to full stops and other punctuation.
For instance, in JavaScript, you can use ESLint with a configuration like:
{
"rules": {
"no-unexpected-multiline": "error",
"dot-notation": "error"
}
}
This configuration helps catch unexpected line breaks and encourages the use of dot notation for property access.
3. Pay Attention to Auto-Completion
While auto-completion features in modern IDEs can be helpful, they can sometimes introduce errors. Always double-check auto-completed code, especially when it involves full stops.
4. Use Meaningful Names for Methods and Properties
Choose clear and descriptive names for methods and properties. This practice not only improves code readability but also makes it easier to spot misplaced full stops.
user.getFullName() // Clear and descriptive
user.gfn() // Unclear and prone to errors
5. Properly Format Your Code
Maintain consistent and clean code formatting. Proper indentation and spacing can make it easier to identify misplaced full stops and other punctuation errors.
// Good formatting
const user = {
name: "Alice",
age: 30,
greet() {
console.log(`Hello, I'm ${this.name}`);
}
};
// Poor formatting (harder to spot errors)
const user={name:"Alice",age:30,greet(){console.log(`Hello, I'm ${this.name}`)}};
Advanced Topics: Full Stops in Different Programming Paradigms
As we delve deeper into the world of programming, it’s important to understand how full stops are used in different programming paradigms and advanced scenarios.
1. Functional Programming
In functional programming languages or paradigms, full stops can be used for function composition and chaining. This approach allows for the creation of complex operations by combining simpler functions.
For example, in JavaScript using a library like Ramda:
const R = require('ramda');
const getName = R.prop('name');
const getUpperCase = R.toUpper;
const greet = R.concat('Hello, ');
const greetUser = R.pipe(
getName,
getUpperCase,
greet
);
const user = { name: 'alice' };
console.log(greetUser(user)); // Output: "Hello, ALICE"
In this example, the full stop is used to access methods from the Ramda library (R
), and the pipe
function chains multiple operations together.
2. Method Chaining in Object-Oriented Programming
Method chaining is a common technique in object-oriented programming where multiple methods are called in a single statement. This approach relies heavily on the proper use of full stops.
Consider this example in Java using the StringBuilder class:
StringBuilder sb = new StringBuilder();
String result = sb.append("Hello")
.append(" ")
.append("World")
.append("!")
.toString();
System.out.println(result); // Output: "Hello World!"
Each method call is chained using a full stop, allowing for a concise and readable way to perform multiple operations on the same object.
3. Namespaces and Scoping
In languages that support namespaces, full stops are often used to define and access elements within specific scopes. This feature helps organize code and prevent naming conflicts in large projects.
For instance, in C#:
namespace MyCompany.MyProject.Utilities
{
public class StringHelper
{
public static string Reverse(string input)
{
char[] charArray = input.ToCharArray();
Array.Reverse(charArray);
return new string(charArray);
}
}
}
// Using the namespace
using MyCompany.MyProject.Utilities;
class Program
{
static void Main(string[] args)
{
string reversed = StringHelper.Reverse("Hello");
Console.WriteLine(reversed); // Output: "olleH"
}
}
In this example, full stops are used to define the nested namespace structure and to access the Reverse
method within the StringHelper
class.
The Future of Full Stops in Programming
As programming languages evolve, the role of full stops may change or expand. Here are some trends and potential future developments:
1. Optional Chaining Operators
Some modern languages are introducing optional chaining operators, which build upon the traditional use of full stops. For example, JavaScript has introduced the ?.
operator:
const user = {
name: "Alice",
address: {
street: "123 Main St"
}
};
console.log(user?.address?.street); // Output: "123 Main St"
console.log(user?.phone?.number); // Output: undefined (no error thrown)
This operator allows for safer property access, especially when dealing with potentially undefined or null values.
2. Increased Use in Functional Programming
As functional programming gains popularity, we may see more creative uses of full stops for function composition and data transformation pipelines.
3. New Language Features
Future programming languages may introduce new syntax or features that utilize full stops in novel ways, potentially expanding their role beyond current uses.
Conclusion
The full stop, despite its small size, plays a crucial role in programming. From object-oriented programming to functional paradigms, this tiny character significantly impacts code structure, readability, and functionality. As we’ve seen, misusing or omitting full stops can lead to a variety of errors and unexpected behaviors.
By understanding the importance of full stops and following best practices, programmers can write cleaner, more efficient, and error-free code. As programming languages continue to evolve, the role of full stops may expand, but their fundamental importance in connecting and structuring code elements is likely to remain.
Remember, in the world of programming, every character matters. Paying attention to details like full stops not only helps prevent bugs but also contributes to creating more robust and maintainable software. So the next time you’re coding, give the humble full stop the respect it deserves – your future self (and your fellow developers) will thank you for it!