The Importance of Edge Cases and How to Identify Them
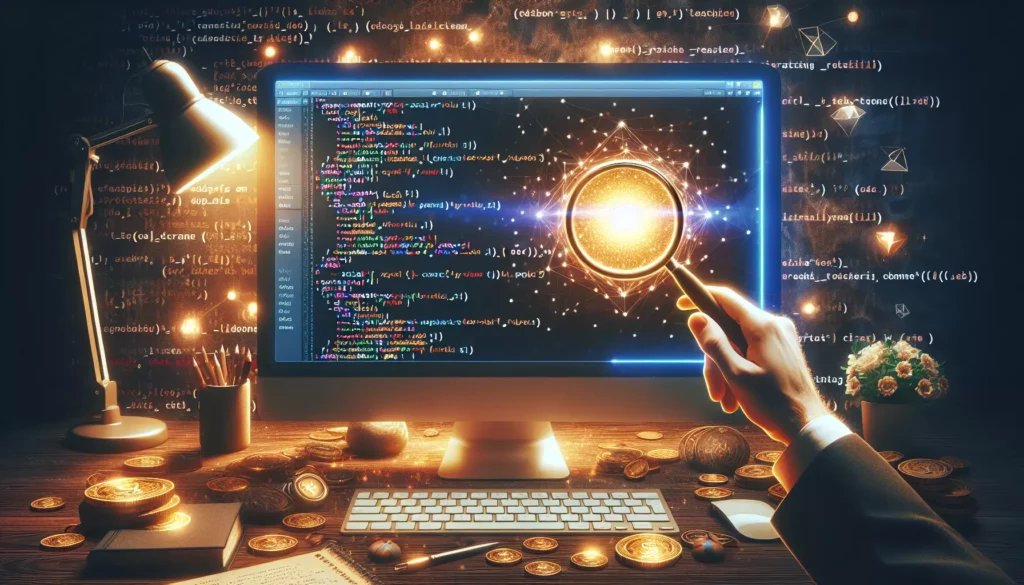
In the world of software development and coding, edge cases play a crucial role in ensuring the robustness and reliability of our programs. As aspiring developers or seasoned professionals preparing for technical interviews at major tech companies, understanding and effectively handling edge cases can make the difference between a mediocre solution and an exceptional one. In this comprehensive guide, we’ll explore the importance of edge cases, how to identify them, and strategies for incorporating them into your problem-solving approach.
What Are Edge Cases?
Edge cases, also known as corner cases, are situations that occur at the extreme ends of the possible range of inputs or conditions for a given problem or system. These are scenarios that are often rare, unexpected, or outside the normal operating parameters. Edge cases test the boundaries of your code and can reveal potential vulnerabilities or limitations in your solution.
For example, consider a function that calculates the average of a list of numbers. Some edge cases might include:
- An empty list
- A list with a single element
- A list with very large numbers that might cause overflow
- A list with negative numbers
Handling these edge cases properly ensures that your function works correctly across all possible inputs, not just the typical or expected ones.
Why Are Edge Cases Important?
Understanding and addressing edge cases is crucial for several reasons:
1. Robustness and Reliability
By considering edge cases, you make your code more robust and reliable. It can handle unexpected inputs or situations without crashing or producing incorrect results. This is especially important in production environments where failures can be costly.
2. Bug Prevention
Many bugs in software are caused by edge cases that weren’t considered during development. By identifying and handling these cases early, you can prevent a significant number of potential issues before they occur in production.
3. Improved User Experience
Properly handling edge cases leads to a better user experience. Users won’t encounter unexpected errors or behavior when using your software, even in unusual situations.
4. Code Quality and Maintainability
Considering edge cases often leads to more thoughtful and comprehensive code design. This results in higher quality code that is easier to maintain and extend in the future.
5. Interview Performance
In technical interviews, especially at major tech companies, the ability to identify and handle edge cases is often a key factor in evaluating candidates. It demonstrates thorough problem-solving skills and attention to detail.
How to Identify Edge Cases
Identifying edge cases requires a systematic approach and practice. Here are some strategies to help you spot potential edge cases:
1. Understand the Problem Domain
Before diving into the code, make sure you have a thorough understanding of the problem domain. This includes knowing the expected inputs, outputs, and any constraints or assumptions.
2. Consider Extreme Values
Think about the minimum and maximum possible values for your inputs. What happens at these extremes? For example:
- For integer inputs: What if the input is 0, negative, or the maximum possible integer value?
- For string inputs: What if the string is empty or very long?
- For collections: What if the collection is empty or contains only one element?
3. Think About Invalid Inputs
Consider inputs that are technically possible but may not be valid for your specific problem. For example:
- Null or undefined values
- Incorrect data types (e.g., a string where a number is expected)
- Malformed data (e.g., an improperly formatted date string)
4. Analyze Boundary Conditions
Look for situations where the behavior of your code might change. These often occur at the boundaries between different conditions or states. For example:
- In a sorting algorithm, what happens when two elements are equal?
- In a search function, what if the target element is at the beginning or end of the list?
5. Consider Performance Edge Cases
Think about inputs that might cause performance issues:
- Very large inputs that might cause time or space complexity problems
- Inputs that trigger worst-case scenarios for your algorithm
6. Use the “What If” Technique
Ask yourself “What if…” questions about your inputs and problem conditions. For example:
- What if the input is already sorted?
- What if all elements are the same?
- What if the input contains duplicate elements?
7. Draw from Experience
As you gain more experience, you’ll start to recognize common edge cases across different problems. Keep a mental (or physical) list of edge cases you’ve encountered in the past.
Practical Examples of Edge Case Identification
Let’s look at some practical examples to illustrate the process of identifying edge cases:
Example 1: Binary Search
Consider implementing a binary search algorithm. Some edge cases to consider might include:
- An empty array
- An array with a single element
- The target element is at the beginning or end of the array
- The target element is not in the array
- All elements in the array are the same
- The array contains duplicate elements
Example 2: String Reversal
For a function that reverses a string, edge cases might include:
- An empty string
- A string with a single character
- A string with only whitespace characters
- A very long string (consider performance)
- A string with special characters or Unicode characters
Example 3: Linked List Operations
When working with linked list operations (e.g., insertion, deletion), consider these edge cases:
- Operations on an empty list
- Operations on a list with a single node
- Inserting/deleting at the head of the list
- Inserting/deleting at the tail of the list
- Operations involving the last node
Strategies for Handling Edge Cases
Once you’ve identified potential edge cases, you need to handle them effectively in your code. Here are some strategies:
1. Input Validation
Always validate your inputs at the beginning of your function. This can prevent many issues caused by invalid or unexpected inputs. For example:
function calculateAverage(numbers) {
if (!Array.isArray(numbers) || numbers.length === 0) {
throw new Error("Input must be a non-empty array of numbers");
}
// ... rest of the function
}
2. Defensive Programming
Use defensive programming techniques to handle potential edge cases throughout your code. This might include checking for null values, handling unexpected types, or setting default values. For example:
function getFirstElement(arr) {
return (arr && arr.length > 0) ? arr[0] : null;
}
3. Use Built-in Language Features
Many programming languages have built-in features or libraries that can help handle common edge cases. For example, in JavaScript, you can use the `??` (nullish coalescing) operator to provide default values:
function greet(name) {
return `Hello, ${name ?? "Guest"}!`;
}
4. Write Clear Error Messages
When an edge case occurs that can’t be handled gracefully, throw an error with a clear and informative message. This helps with debugging and improves the overall robustness of your code.
5. Use Try-Catch Blocks
For operations that might throw exceptions, use try-catch blocks to handle potential errors gracefully:
function parseJSON(jsonString) {
try {
return JSON.parse(jsonString);
} catch (error) {
console.error("Invalid JSON string:", error);
return null;
}
}
6. Write Unit Tests for Edge Cases
Include unit tests that specifically target edge cases. This ensures that your code handles these cases correctly and helps prevent regressions in the future.
Edge Cases in Technical Interviews
In technical interviews, especially at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), the ability to identify and handle edge cases is often a key factor in evaluating candidates. Here are some tips for addressing edge cases during interviews:
1. Think Aloud
As you’re solving a problem, verbalize your thought process about potential edge cases. This demonstrates your problem-solving approach and attention to detail.
2. Ask Clarifying Questions
Don’t hesitate to ask the interviewer about potential edge cases or assumptions you should make. This shows that you’re considering these scenarios and helps ensure you’re solving the right problem.
3. Mention Edge Cases Early
Bring up potential edge cases early in your solution discussion. You can say something like, “Before we dive into the main algorithm, let’s consider some edge cases we might need to handle.”
4. Prioritize
If time is limited, prioritize the most important or likely edge cases. You can mention other potential edge cases you’ve thought of, even if you don’t have time to fully implement them.
5. Test Your Solution
After implementing your solution, walk through it with a few test cases, including edge cases. This demonstrates that you’re thinking about code correctness and not just the happy path.
Common Edge Cases to Consider
While edge cases can vary widely depending on the specific problem, here are some common categories of edge cases to keep in mind:
1. Empty or Null Inputs
Always consider what should happen if the input is empty, null, or undefined. This is especially important for collections (arrays, lists, strings) and objects.
2. Boundary Values
Consider the minimum and maximum possible values for your inputs. This includes things like integer overflow, floating-point precision issues, and the limits of data structures.
3. Single-Element Collections
Collections with only one element can often behave differently than those with multiple elements. Make sure your code handles these cases correctly.
4. Duplicate or Repeated Values
If your algorithm assumes unique values, consider what happens when there are duplicates. This is especially important in sorting and searching algorithms.
5. Negative Numbers
For numerical inputs, consider how negative numbers might affect your algorithm. This is particularly important for mathematical operations and array indices.
6. Large Inputs
Consider how your algorithm performs with very large inputs. This can reveal performance issues or potential stack overflow problems in recursive solutions.
7. Invalid or Unexpected Input Types
If your function expects a certain type of input, consider what happens if it receives a different type. This is especially important in dynamically-typed languages.
8. Concurrent Access
In multi-threaded environments, consider edge cases related to concurrent access or modification of shared resources.
Conclusion
Mastering the art of identifying and handling edge cases is a crucial skill for any programmer, especially those aiming for positions at top tech companies. It’s not just about writing code that works for the typical case, but about creating robust, reliable solutions that can handle any input or situation.
By systematically considering potential edge cases, you’ll write better code, prevent bugs, and demonstrate your thoroughness and attention to detail in technical interviews. Remember, the ability to anticipate and handle edge cases is often what separates good programmers from great ones.
As you continue to practice and develop your coding skills, make identifying edge cases a core part of your problem-solving process. Over time, it will become second nature, and you’ll find yourself naturally considering these scenarios as you design and implement your solutions.
Keep in mind that while it’s important to handle edge cases, it’s also crucial to balance this with the need for clean, readable code. Overcomplicating your solution to handle extremely unlikely edge cases can sometimes do more harm than good. Use your judgment to decide which edge cases are truly important for your specific problem and context.
By incorporating edge case analysis into your coding practice and interview preparation, you’ll be well-equipped to tackle complex problems and shine in technical interviews at even the most competitive tech companies. Remember, in the world of coding, it’s often the edge cases that make the difference between a good solution and a great one.