The Importance of Continuous Integration and Deployment in Modern Development
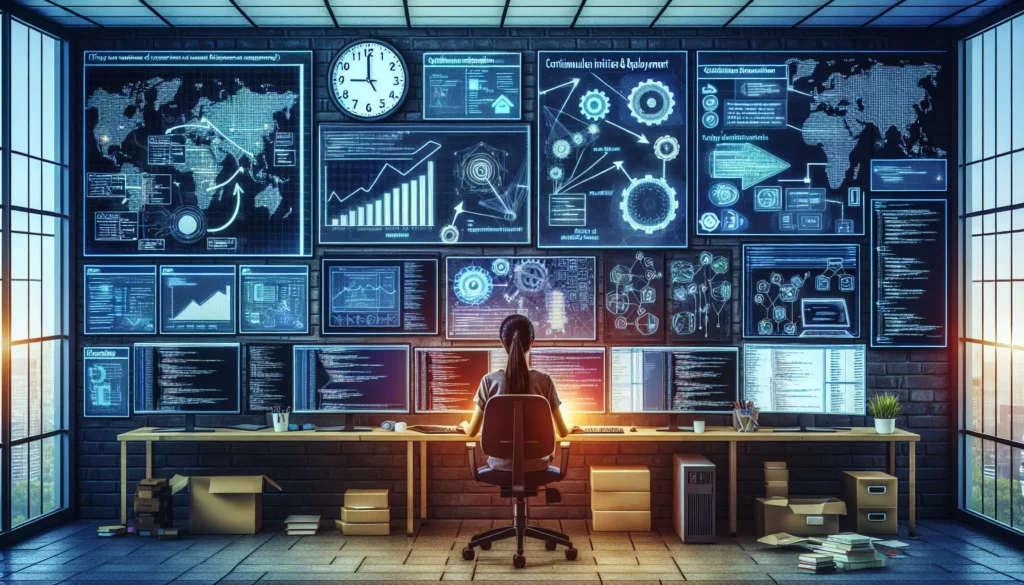
In today’s fast-paced software development landscape, the ability to deliver high-quality code quickly and efficiently is crucial. This is where Continuous Integration (CI) and Continuous Deployment (CD) come into play. These practices have revolutionized the way development teams work, enabling faster iterations, better collaboration, and more reliable software releases. In this comprehensive guide, we’ll explore the importance of CI/CD in modern development, its benefits, implementation strategies, and how it fits into the broader context of coding education and skill development.
What are Continuous Integration and Continuous Deployment?
Before diving into the importance of CI/CD, let’s define these terms:
Continuous Integration (CI)
Continuous Integration is the practice of frequently merging code changes into a central repository, followed by automated builds and tests. The main goal of CI is to detect and address integration issues early in the development process, ensuring that the codebase remains stable and functional as new features are added or existing ones are modified.
Continuous Deployment (CD)
Continuous Deployment takes CI a step further by automatically deploying every change that passes the automated tests to production. This means that every commit that passes the CI pipeline is automatically released to end-users without manual intervention.
It’s worth noting that some organizations prefer Continuous Delivery, which is similar to Continuous Deployment but includes a manual approval step before changes are pushed to production.
The Importance of CI/CD in Modern Development
Now that we understand what CI/CD is, let’s explore why it’s crucial in modern development:
1. Faster Time-to-Market
In today’s competitive landscape, getting features and bug fixes to users quickly can be a significant advantage. CI/CD pipelines automate much of the build, test, and deployment process, allowing developers to push changes to production much faster than traditional methods.
2. Improved Code Quality
By integrating code frequently and running automated tests on every change, CI helps catch bugs and integration issues early. This leads to higher overall code quality and reduces the likelihood of major issues making it to production.
3. Increased Developer Productivity
CI/CD automates many repetitive tasks, such as building, testing, and deploying code. This frees up developers to focus on writing code and solving problems rather than managing manual processes.
4. Better Collaboration
CI encourages developers to integrate their changes frequently, which promotes better communication and collaboration within the team. It also makes it easier to identify and resolve conflicts between different developers’ work.
5. Reduced Risk
By deploying smaller, incremental changes more frequently, CD reduces the risk associated with each individual deployment. If an issue does occur, it’s easier to identify and roll back a small change than a large, monolithic release.
6. Consistent Environments
CI/CD pipelines typically include steps to create consistent environments for testing and deployment. This reduces the “it works on my machine” problem and ensures that code behaves the same way in development, testing, and production environments.
7. Faster Feedback Loop
With automated tests running on every commit, developers get immediate feedback on their changes. This allows them to catch and fix issues quickly, rather than discovering problems later in the development cycle.
Implementing CI/CD: Best Practices and Tools
Implementing CI/CD requires careful planning and the right tools. Here are some best practices and popular tools to consider:
Best Practices
- Commit Often: Encourage developers to make small, frequent commits to the main branch.
- Automate Everything: Aim to automate as much of the build, test, and deployment process as possible.
- Use Version Control: A robust version control system like Git is essential for CI/CD.
- Write Good Tests: Comprehensive automated tests are crucial for catching issues early.
- Monitor and Optimize: Continuously monitor your CI/CD pipeline and optimize it for speed and reliability.
- Use Feature Flags: Feature flags allow you to deploy code to production without immediately activating new features, giving you more control over releases.
Popular CI/CD Tools
- Jenkins: An open-source automation server that supports building, deploying, and automating any project.
- GitLab CI/CD: Integrated CI/CD functionality within the GitLab platform.
- GitHub Actions: CI/CD and workflow automation tool integrated with GitHub.
- CircleCI: Cloud-based CI/CD platform that integrates with various version control systems.
- Travis CI: Distributed CI service used to build and test software projects hosted on GitHub and Bitbucket.
- Azure DevOps: Microsoft’s suite of DevOps tools, including Azure Pipelines for CI/CD.
CI/CD in the Context of Coding Education and Skill Development
Understanding and implementing CI/CD practices is becoming increasingly important for aspiring developers. Here’s how CI/CD fits into the broader context of coding education and skill development:
1. Industry-Relevant Skills
Many tech companies, including FAANG (Facebook, Amazon, Apple, Netflix, Google) and other major players, use CI/CD practices extensively. Familiarity with these concepts can give job seekers an edge in technical interviews and make them more valuable to potential employers.
2. Practical Application of Software Engineering Principles
CI/CD embodies many important software engineering principles, such as automation, frequent integration, and rapid feedback. Learning about CI/CD helps reinforce these concepts in a practical context.
3. Enhanced Problem-Solving Skills
Setting up and maintaining CI/CD pipelines often involves troubleshooting complex systems and integrations. This can help develop problem-solving skills that are valuable in many areas of software development.
4. Exposure to DevOps Concepts
CI/CD is a key component of DevOps practices. Learning about CI/CD can serve as an introduction to broader DevOps concepts and methodologies.
5. Improved Code Quality Awareness
Working with CI/CD pipelines encourages developers to think more about code quality, test coverage, and the importance of writing maintainable code.
Implementing CI/CD in Your Learning Journey
If you’re learning to code or looking to enhance your development skills, here are some ways to incorporate CI/CD concepts into your learning journey:
1. Set Up a Personal CI/CD Pipeline
Create a simple CI/CD pipeline for one of your personal projects. You can use free tools like GitHub Actions or GitLab CI/CD to get started. Here’s a basic example of a GitHub Actions workflow for a Python project:
name: Python CI
on: [push]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up Python
uses: actions/setup-python@v2
with:
python-version: '3.x'
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install -r requirements.txt
- name: Run tests
run: python -m unittest discover tests
2. Practice with Online Coding Platforms
Many online coding platforms and coding bootcamps now include CI/CD concepts in their curricula. Look for courses or tutorials that cover these topics and provide hands-on experience.
3. Contribute to Open Source Projects
Many open-source projects use CI/CD pipelines. Contributing to these projects can give you practical experience working with real-world CI/CD setups.
4. Learn Related Tools and Technologies
Familiarize yourself with related tools and technologies often used in CI/CD pipelines, such as Docker for containerization, Kubernetes for orchestration, and various testing frameworks.
5. Stay Updated with Industry Trends
CI/CD practices and tools are constantly evolving. Follow industry blogs, attend webinars, and participate in developer communities to stay up-to-date with the latest trends and best practices.
Challenges and Considerations in CI/CD Implementation
While CI/CD offers numerous benefits, it’s important to be aware of potential challenges and considerations:
1. Initial Setup Complexity
Setting up a robust CI/CD pipeline can be complex, especially for larger projects or teams new to the concept. It requires careful planning and may involve a significant initial time investment.
2. Maintaining Test Quality
The effectiveness of CI/CD relies heavily on the quality of automated tests. Writing and maintaining a comprehensive test suite requires ongoing effort and discipline from the development team.
3. Managing Build Times
As projects grow, CI/CD pipelines can become slower. Long build times can delay feedback and reduce the benefits of frequent integration. Optimizing build times often becomes an ongoing challenge.
4. Security Considerations
Automated deployments can potentially introduce security risks if not properly managed. It’s crucial to implement proper security measures and access controls in your CI/CD pipeline.
5. Cultural Shift
Adopting CI/CD often requires a cultural shift within an organization. It may involve changes to workflows and responsibilities, which can meet resistance from team members accustomed to traditional development practices.
6. Handling Database Changes
Managing database schema changes and data migrations in a CI/CD pipeline can be challenging. It requires careful planning and potentially specialized tools or practices.
Advanced CI/CD Concepts
As you become more comfortable with basic CI/CD practices, you may want to explore more advanced concepts:
1. Blue-Green Deployments
Blue-green deployment is a technique that reduces downtime and risk by running two identical production environments called Blue and Green. Here’s a simplified example of how it might work:
// Pseudo-code for blue-green deployment
function deploy() {
// Assume 'blue' is currently active
green = createNewEnvironment();
deployCodeTo(green);
runTests(green);
if (testsPass(green)) {
switchTrafficTo(green);
decommission(blue);
} else {
rollback();
}
}
2. Canary Releases
Canary releases involve gradually rolling out changes to a small subset of users before making them available to everyone. This allows you to test new features in the wild with minimal risk.
3. A/B Testing in CI/CD
Integrating A/B testing into your CI/CD pipeline allows you to automatically deploy different versions of your application to different user groups and analyze the results.
4. Infrastructure as Code (IaC)
IaC involves managing and provisioning infrastructure through code instead of manual processes. This aligns well with CI/CD practices and can be incorporated into your pipeline.
5. GitOps
GitOps is an operational framework that takes DevOps best practices used for application development such as version control, collaboration, compliance, and CI/CD, and applies them to infrastructure automation.
Conclusion
Continuous Integration and Continuous Deployment have become integral parts of modern software development. They enable teams to deliver high-quality software faster and more reliably, which is crucial in today’s competitive tech landscape. For aspiring developers and those looking to enhance their skills, understanding and implementing CI/CD practices can provide valuable experience and make them more attractive to potential employers.
As you continue your coding journey, whether you’re just starting out or preparing for technical interviews with major tech companies, consider incorporating CI/CD concepts into your learning and projects. Not only will this help you develop more robust and efficient coding practices, but it will also give you hands-on experience with tools and methodologies used in professional software development environments.
Remember, mastering CI/CD is an ongoing process. As with any aspect of software development, the key is to start small, practice regularly, and continually seek out new knowledge and best practices. By doing so, you’ll be well-equipped to tackle the challenges of modern software development and contribute effectively to projects of any scale.