The Importance of Code Readability and Maintainability
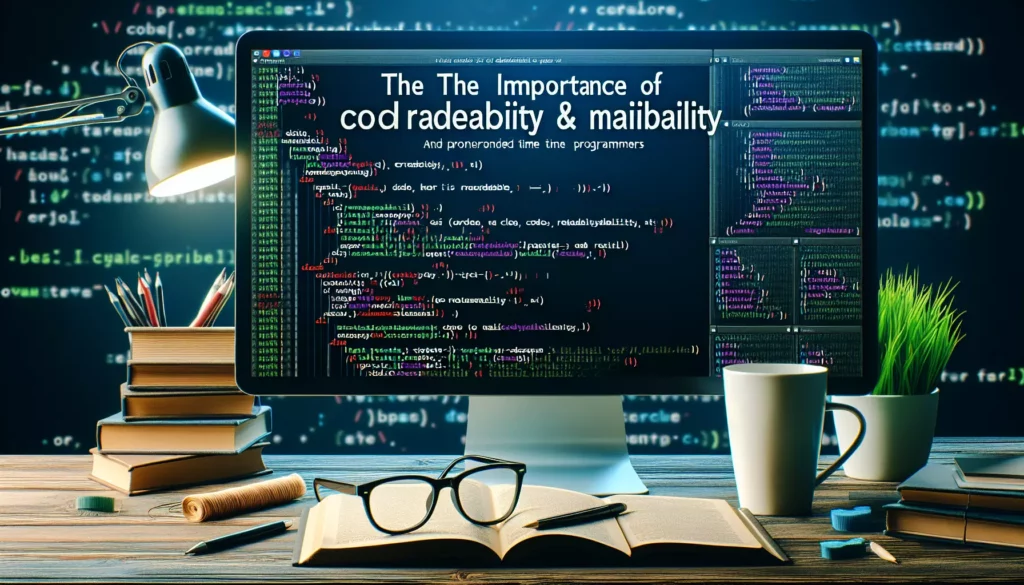
In the world of software development, writing code that works is only half the battle. The other half, which is often overlooked but equally crucial, is ensuring that the code is readable and maintainable. As developers, we spend far more time reading and maintaining existing code than writing new code from scratch. This is why code readability and maintainability are paramount in creating sustainable, scalable, and efficient software systems. In this comprehensive guide, we’ll explore the importance of these concepts and provide practical tips to improve your coding practices.
What is Code Readability?
Code readability refers to how easily human programmers can understand and interpret written code. Readable code is clear, concise, and self-explanatory. It follows logical patterns and uses meaningful names for variables, functions, and classes. When code is readable, it becomes easier for developers to:
- Understand the purpose and functionality of the code
- Identify and fix bugs
- Collaborate with other team members
- Maintain and update the codebase over time
What is Code Maintainability?
Code maintainability is the ease with which software can be modified, updated, or extended over time. Maintainable code is designed with future changes in mind, making it adaptable to new requirements or bug fixes. Key aspects of maintainable code include:
- Modularity: Breaking code into smaller, reusable components
- Loose coupling: Minimizing dependencies between different parts of the code
- Consistent coding standards: Following established best practices and style guides
- Proper documentation: Providing clear explanations of code functionality and design decisions
Why are Code Readability and Maintainability Important?
1. Reduced Development Time and Costs
When code is readable and maintainable, developers can quickly understand and modify it. This leads to faster development cycles, easier debugging, and more efficient use of resources. In the long run, this translates to reduced development costs and improved productivity.
2. Improved Collaboration
In most software projects, multiple developers work on the same codebase. Readable and maintainable code facilitates better collaboration by allowing team members to easily understand each other’s work. This leads to smoother code reviews, fewer misunderstandings, and more effective teamwork.
3. Enhanced Code Quality
Code that is easy to read and maintain is generally of higher quality. It tends to have fewer bugs, better performance, and improved security. When developers can easily understand the code, they’re more likely to spot potential issues and implement best practices.
4. Easier Onboarding of New Team Members
When new developers join a project, they need to quickly familiarize themselves with the existing codebase. Readable and maintainable code significantly reduces the learning curve, allowing new team members to become productive more quickly.
5. Future-Proofing
Software projects often evolve over time, with new features being added and existing ones being modified. Maintainable code is designed to accommodate these changes with minimal disruption, making it easier to adapt to new requirements and technologies.
Best Practices for Improving Code Readability and Maintainability
1. Use Meaningful Names
Choose descriptive and meaningful names for variables, functions, classes, and other code elements. This helps convey the purpose and functionality of the code at a glance.
Example of poor naming:
function f(x, y) {
return x + y;
}
let a = 5;
let b = 10;
let c = f(a, b);
Example of good naming:
function addNumbers(firstNumber, secondNumber) {
return firstNumber + secondNumber;
}
let baseValue = 5;
let incrementValue = 10;
let sum = addNumbers(baseValue, incrementValue);
2. Keep Functions Small and Focused
Write small, single-purpose functions that do one thing well. This makes the code easier to understand, test, and maintain.
Example of a function that does too much:
function processUserData(userData) {
// Validate user input
if (!userData.name || !userData.email || !userData.age) {
throw new Error("Invalid user data");
}
// Format user data
const formattedName = userData.name.toUpperCase();
const formattedEmail = userData.email.toLowerCase();
// Save user to database
const user = {
name: formattedName,
email: formattedEmail,
age: userData.age
};
database.saveUser(user);
// Send welcome email
sendWelcomeEmail(formattedEmail, formattedName);
return user;
}
Example of breaking the function into smaller, focused functions:
function validateUserData(userData) {
if (!userData.name || !userData.email || !userData.age) {
throw new Error("Invalid user data");
}
}
function formatUserData(userData) {
return {
name: userData.name.toUpperCase(),
email: userData.email.toLowerCase(),
age: userData.age
};
}
function saveUserToDatabase(user) {
database.saveUser(user);
}
function sendUserWelcomeEmail(user) {
sendWelcomeEmail(user.email, user.name);
}
function processUserData(userData) {
validateUserData(userData);
const formattedUser = formatUserData(userData);
saveUserToDatabase(formattedUser);
sendUserWelcomeEmail(formattedUser);
return formattedUser;
}
3. Use Consistent Formatting and Style
Adhere to a consistent coding style throughout your project. This includes things like indentation, bracket placement, and naming conventions. Many programming languages have established style guides, such as PEP 8 for Python or the Google Java Style Guide.
4. Write Self-Documenting Code
Strive to write code that is self-explanatory, reducing the need for extensive comments. Use clear variable and function names, and structure your code logically.
Example of code that requires comments:
// Calculate the total price including tax
let t = p + (p * r);
Example of self-documenting code:
let totalPrice = basePrice + (basePrice * taxRate);
5. Use Comments Judiciously
While self-documenting code is ideal, there are times when comments are necessary. Use comments to explain complex algorithms, clarify non-obvious decisions, or provide context that can’t be conveyed through code alone.
// Implement the Quicksort algorithm
function quicksort(arr, low, high) {
if (low < high) {
// pi is the partitioning index, arr[pi] is now at the right place
let pi = partition(arr, low, high);
// Recursively sort elements before and after partition
quicksort(arr, low, pi - 1);
quicksort(arr, pi + 1, high);
}
}
// This function takes the last element as pivot, places the pivot element at its
// correct position in sorted array, and places all smaller elements to left of
// pivot and all greater elements to right of pivot
function partition(arr, low, high) {
// Implementation details...
}
6. Follow the DRY Principle (Don’t Repeat Yourself)
Avoid duplicating code by extracting common functionality into reusable functions or classes. This reduces the amount of code that needs to be maintained and makes it easier to make changes across the entire codebase.
Example of repeated code:
function calculateCircleArea(radius) {
return 3.14159 * radius * radius;
}
function calculateCircleCircumference(radius) {
return 2 * 3.14159 * radius;
}
Example of DRY code:
const PI = 3.14159;
function calculateCircleArea(radius) {
return PI * radius * radius;
}
function calculateCircleCircumference(radius) {
return 2 * PI * radius;
}
7. Use Version Control
Implement a version control system like Git to track changes, collaborate with others, and maintain a history of your codebase. This helps in managing code changes and reverting to previous versions if needed.
8. Write Unit Tests
Create unit tests for your code to ensure its correctness and make it easier to refactor or modify in the future. Tests serve as documentation and help catch regressions when changes are made.
function add(a, b) {
return a + b;
}
// Unit test for the add function
function testAdd() {
console.assert(add(2, 3) === 5, "2 + 3 should equal 5");
console.assert(add(-1, 1) === 0, "-1 + 1 should equal 0");
console.assert(add(0, 0) === 0, "0 + 0 should equal 0");
}
testAdd();
9. Use Design Patterns and Principles
Familiarize yourself with common design patterns and principles like SOLID (Single Responsibility, Open-Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion). These concepts help in creating more maintainable and flexible code structures.
10. Refactor Regularly
Don’t be afraid to refactor your code when you see opportunities for improvement. Regular refactoring helps maintain code quality and prevents technical debt from accumulating.
Tools to Improve Code Readability and Maintainability
Several tools can help you enhance the readability and maintainability of your code:
1. Linters
Linters are static code analysis tools that can identify potential errors, stylistic issues, and suspicious constructs. Examples include ESLint for JavaScript, Pylint for Python, and RuboCop for Ruby.
2. Code Formatters
Automated code formatters ensure consistent styling across your codebase. Popular options include Prettier for JavaScript and Black for Python.
3. Integrated Development Environments (IDEs)
Modern IDEs like Visual Studio Code, IntelliJ IDEA, and PyCharm offer features like code completion, refactoring tools, and built-in linters that can significantly improve code quality and maintainability.
4. Documentation Generators
Tools like JSDoc for JavaScript and Sphinx for Python can generate documentation from code comments, encouraging developers to write clear and comprehensive documentation.
5. Code Review Tools
Platforms like GitHub, GitLab, and Bitbucket provide built-in code review functionality, making it easier for team members to collaborate and maintain code quality.
Conclusion
Code readability and maintainability are fundamental aspects of high-quality software development. By prioritizing these principles, developers can create more robust, efficient, and long-lasting applications. Remember that writing readable and maintainable code is an ongoing process that requires constant attention and refinement.
As you progress in your coding journey, whether you’re a beginner learning the basics or preparing for technical interviews at major tech companies, always keep the importance of code readability and maintainability in mind. These skills will not only make you a better developer but also a valuable asset to any development team.
Practice these principles in your daily coding tasks, and don’t hesitate to seek feedback from more experienced developers. Over time, you’ll develop a keen eye for clean, readable, and maintainable code, which will serve you well throughout your programming career.