The Importance of Asking Clarifying Questions in Interviews: A Guide for Aspiring Programmers
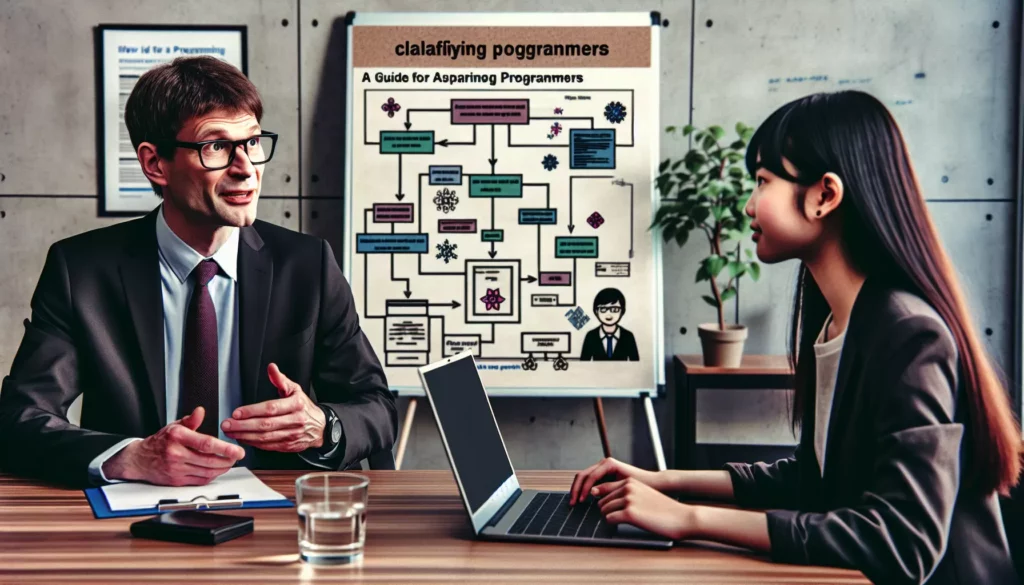
In the competitive world of tech interviews, particularly for coveted positions at major companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), your ability to solve complex coding problems is just one piece of the puzzle. A often overlooked but crucial skill is the art of asking clarifying questions. This skill not only demonstrates your analytical thinking but also ensures you fully understand the problem at hand before diving into a solution. In this comprehensive guide, we’ll explore why asking clarifying questions is essential, how to do it effectively, and how it can significantly improve your performance in technical interviews.
Why Asking Clarifying Questions Matters
Before we delve into the how-to’s, let’s understand why asking clarifying questions is so important in the context of coding interviews:
- Demonstrates critical thinking: By asking thoughtful questions, you show the interviewer that you approach problems analytically and don’t make assumptions.
- Prevents misunderstandings: Clarifying the problem statement helps avoid wasting time on incorrect solutions due to misinterpretations.
- Reveals hidden complexities: Often, interviewers intentionally leave out certain details to see if candidates will inquire about them.
- Builds rapport: Engaging in a dialogue with your interviewer can help create a more comfortable and collaborative atmosphere.
- Buys thinking time: Asking questions gives you a moment to gather your thoughts and start formulating a strategy.
- Showcases communication skills: Clear, concise questions demonstrate your ability to communicate effectively, a crucial skill in any tech role.
Types of Clarifying Questions to Ask
When faced with a coding problem in an interview, consider asking questions that fall into these categories:
1. Input Clarification
- What is the expected input format?
- Are there any constraints on the input size or values?
- How should I handle edge cases or invalid inputs?
2. Output Expectations
- What exactly should the function return?
- Is there a specific format required for the output?
- How should ties or multiple correct answers be handled?
3. Performance Requirements
- Are there any time or space complexity constraints?
- Is optimization a priority, or is a working solution sufficient?
- Should I focus on readability or efficiency?
4. Assumptions and Edge Cases
- Can I assume the input is always valid?
- How should I handle null or empty inputs?
- Are there any specific edge cases I should consider?
5. Problem-Specific Details
- Can you provide an example of the expected input and output?
- Are there any specific algorithms or data structures you’d like me to use or avoid?
- Is this problem similar to any well-known algorithmic problems?
How to Ask Clarifying Questions Effectively
Asking questions is an art, and doing it effectively can significantly enhance your interview performance. Here are some tips on how to ask clarifying questions in a way that impresses your interviewer:
1. Start with a Brief Analysis
Before jumping into questions, take a moment to analyze the problem statement. This shows the interviewer that you’re not asking questions blindly but have given some thought to the problem.
Example:
"I see that we're dealing with a string manipulation problem. Before I start, I'd like to clarify a few things to ensure I understand the requirements correctly."
2. Be Specific and Concise
Frame your questions clearly and concisely. Avoid vague or overly broad questions that might suggest you haven’t thought through the problem.
Good: “Should the function handle uppercase and lowercase letters differently?”
Bad: “How should I deal with the letters?”
3. Use Examples
When appropriate, use examples to illustrate your questions. This can help both you and the interviewer ensure you’re on the same page.
"For instance, if the input is '12345', should the output be [1,2,3,4,5] or ['1','2','3','4','5']?"
4. Group Related Questions
If you have multiple related questions, group them together to show organized thinking.
"Regarding the input constraints, I have a couple of questions:
1. Is there a maximum length for the input string?
2. Can the input contain special characters, or is it limited to alphanumeric characters?"
5. Listen Actively and Follow Up
Pay close attention to the interviewer’s responses and be prepared to ask follow-up questions if needed. This demonstrates active listening and thorough analysis.
6. Know When to Stop
While asking questions is important, don’t overdo it. Once you have a clear understanding of the problem, move on to solving it. If you’re unsure about something while coding, it’s okay to ask for clarification then as well.
Common Pitfalls to Avoid
While asking clarifying questions is generally positive, there are some pitfalls to be aware of:
- Asking questions answered in the problem statement: This suggests you didn’t read carefully.
- Asking too many questions: This can make you appear indecisive or lacking in confidence.
- Asking questions unrelated to the core problem: Stay focused on what’s relevant to solving the task at hand.
- Using questions as a delay tactic: While questions can buy you some thinking time, don’t use them solely for this purpose.
- Ignoring the interviewer’s hints: Sometimes, the way an interviewer responds to your questions can provide valuable hints about the expected approach.
Practical Examples
Let’s look at a couple of example scenarios to illustrate how effective questioning can improve your approach to a coding problem.
Example 1: String Reversal
Interviewer: “Write a function to reverse a string.”
Ineffective approach:
Candidate: "Okay, I'll write a function to reverse a string."
// Proceeds to write code without asking any questions
Effective approach:
Candidate: "Before I begin, I'd like to clarify a few points:
1. Should I handle an empty string as input?
2. Do we need to consider Unicode characters, or can I assume ASCII only?
3. Is in-place reversal required, or can I use additional space?
4. Should I handle null input, or can I assume the input is always a valid string?"
Interviewer: "Good questions. Assume ASCII characters only, null inputs won't be given, and you can use additional space if needed."
Candidate: "Thank you for the clarification. Based on this, I'll implement a solution that handles empty strings and uses additional space for simplicity."
Example 2: Array Manipulation
Interviewer: “Implement a function to remove duplicates from an array.”
Ineffective approach:
Candidate: "Alright, I'll remove duplicates from the array."
// Starts coding immediately, potentially missing important details
Effective approach:
Candidate: "I'd like to ask a few questions to ensure I understand the requirements:
1. What type of elements are in the array? Integers, strings, or mixed types?
2. Should the original order of elements be preserved?
3. Is there a constraint on the space complexity? Should I modify the array in-place?
4. How large can the input array be? Do we need to consider performance for very large arrays?
5. How should I handle an empty array or an array with no duplicates?"
Interviewer: "Great questions. Let's say we're dealing with integers, order doesn't matter, and you can use additional space if needed. The array size could be up to 10^5 elements."
Candidate: "Thank you. Given these parameters, I'll implement a solution using a hash set for efficiency, which will handle empty arrays and arrays with no duplicates naturally."
Incorporating Clarifying Questions into Your Problem-Solving Process
To make asking clarifying questions a natural part of your problem-solving approach, consider integrating it into a structured process:
- Read the problem statement carefully: Take your time to understand what’s being asked.
- Identify potential ambiguities: Note any areas where the problem statement might be unclear or where assumptions might be necessary.
- Formulate clear, concise questions: Based on your analysis, prepare your clarifying questions.
- Ask your questions: Present your questions to the interviewer, grouping related ones together.
- Summarize your understanding: After getting answers, briefly restate your understanding of the problem to ensure you’re on the right track.
- Begin problem-solving: With a clear understanding, start designing and implementing your solution.
- Ask follow-up questions if needed: If you encounter uncertainties while coding, don’t hesitate to ask for further clarification.
How AlgoCademy Can Help You Master This Skill
At AlgoCademy, we understand the importance of developing not just coding skills, but also the soft skills crucial for succeeding in technical interviews. Here’s how our platform can help you master the art of asking clarifying questions:
- Interactive Problem Solving: Our coding challenges are designed to mimic real interview scenarios, including intentionally ambiguous problem statements that require clarification.
- AI-Powered Interview Simulation: Practice with our AI interviewer, which is programmed to respond to your clarifying questions, helping you get comfortable with the back-and-forth of a real interview.
- Guided Learning Paths: Our curated learning paths include modules on soft skills, including effective communication and question-asking techniques.
- Peer Review System: Engage with other learners to review and discuss approaches to problem-solving, including strategies for asking effective questions.
- Expert Feedback: Receive personalized feedback from experienced programmers on your problem-solving approach, including your use of clarifying questions.
- Real-World Scenario Practice: Tackle problems based on actual interview questions from top tech companies, complete with guidance on what clarifying questions would be appropriate.
Conclusion
Mastering the art of asking clarifying questions is a valuable skill that can significantly enhance your performance in coding interviews. It demonstrates your analytical thinking, attention to detail, and communication skills – all highly prized attributes in the tech industry. By incorporating this skill into your problem-solving toolkit, you’ll not only improve your chances of solving coding challenges correctly but also leave a lasting positive impression on your interviewers.
Remember, the goal is not just to write code, but to solve problems effectively. Clarifying questions are your tool for understanding the problem deeply before you begin coding. With practice and the right approach, you’ll find that this skill becomes second nature, setting you apart in your journey towards landing your dream job in tech.
At AlgoCademy, we’re committed to helping you develop all the skills you need to excel in your coding career. From mastering algorithms to honing your soft skills, our platform provides the comprehensive support you need to tackle even the most challenging technical interviews with confidence. Start your journey with us today, and take the first step towards becoming not just a good coder, but an exceptional problem solver.