The Importance of Accessibility in Software Development
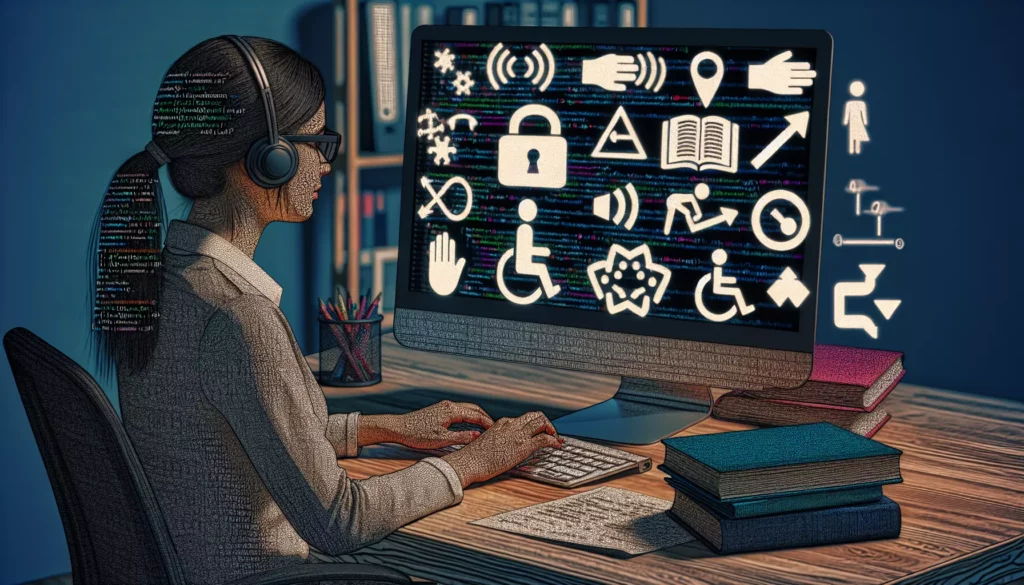
In today’s digital age, software applications have become an integral part of our daily lives. From smartphones to web browsers, we interact with various software products throughout the day. However, not all users have the same abilities or access to technology. This is where the concept of accessibility in software development comes into play. Accessibility ensures that software products are usable by people with diverse abilities, including those with disabilities. In this comprehensive guide, we’ll explore the importance of accessibility in software development and how it aligns with AlgoCademy’s mission of providing inclusive coding education.
What is Accessibility in Software Development?
Accessibility in software development refers to the practice of designing and developing software applications that can be used by people with various disabilities or limitations. This includes individuals with visual, auditory, motor, or cognitive impairments. The goal is to create inclusive software that can be accessed and used by the widest possible audience, regardless of their abilities or the devices they use.
Accessibility is not just about accommodating people with permanent disabilities. It also benefits users with temporary impairments (such as a broken arm) or situational limitations (like using a device in bright sunlight). By focusing on accessibility, developers can create more user-friendly and versatile applications that cater to a broader range of users and scenarios.
Why is Accessibility Important?
The importance of accessibility in software development cannot be overstated. Here are several key reasons why accessibility should be a priority for developers and organizations:
1. Inclusivity and Equal Access
Accessibility ensures that all users, regardless of their abilities, can access and use software applications. This promotes inclusivity and equal opportunities in the digital world. By making software accessible, we break down barriers and enable individuals with disabilities to participate fully in various aspects of life, including education, employment, and social interactions.
2. Legal Compliance
Many countries have laws and regulations that require digital products to be accessible. For example, in the United States, the Americans with Disabilities Act (ADA) and Section 508 of the Rehabilitation Act mandate accessibility for certain types of software and websites. Failure to comply with these regulations can result in legal consequences and financial penalties.
3. Expanded User Base
By making software accessible, developers can reach a wider audience. According to the World Health Organization, over 1 billion people worldwide live with some form of disability. That’s a significant portion of the population that could potentially use and benefit from accessible software. By catering to this demographic, businesses can expand their user base and increase their market share.
4. Improved User Experience
Accessibility features often lead to improved user experience for all users, not just those with disabilities. For example, clear and consistent navigation, well-structured content, and keyboard shortcuts can benefit everyone by making software easier to use and more efficient.
5. Better SEO and Discoverability
Many accessibility practices, such as proper use of headings, alternative text for images, and descriptive link text, also contribute to better search engine optimization (SEO). This can improve the discoverability of web-based applications and increase organic traffic.
6. Innovation and Adaptability
Designing for accessibility often leads to innovative solutions that can benefit all users. For instance, voice commands, originally developed for users with motor impairments, are now widely used by many people for convenience. Accessibility considerations can drive innovation and lead to more adaptable and flexible software designs.
Key Principles of Accessible Software Development
To create accessible software, developers should follow certain principles and guidelines. The Web Content Accessibility Guidelines (WCAG) provide a comprehensive set of recommendations for making web content more accessible. While these guidelines are primarily focused on web content, many of the principles can be applied to software development in general. Here are some key principles to consider:
1. Perceivable
Information and user interface components must be presentable to users in ways they can perceive. This means providing alternatives for non-text content, creating content that can be presented in different ways without losing meaning, and making it easier for users to see and hear content.
Examples:
- Providing alternative text for images
- Offering captions for audio and video content
- Ensuring sufficient color contrast for text and background
2. Operable
User interface components and navigation must be operable. This means making all functionality available from a keyboard, giving users enough time to read and use content, and helping users navigate and find content.
Examples:
- Implementing keyboard navigation
- Providing skip navigation links
- Avoiding time-based content that users can’t control
3. Understandable
Information and the operation of the user interface must be understandable. This involves making text readable and understandable, making content appear and operate in predictable ways, and helping users avoid and correct mistakes.
Examples:
- Using clear and simple language
- Providing consistent navigation across pages
- Offering clear error messages and suggestions for correction
4. Robust
Content must be robust enough that it can be interpreted reliably by a wide variety of user agents, including assistive technologies. This means maximizing compatibility with current and future user tools.
Examples:
- Using valid HTML and following web standards
- Ensuring compatibility with screen readers and other assistive technologies
- Providing name, role, and value for custom user interface components
Implementing Accessibility in Software Development
Implementing accessibility in software development requires a holistic approach that considers accessibility from the beginning of the development process. Here are some key steps and considerations:
1. Start with Accessibility in Mind
Incorporate accessibility considerations into the initial planning and design phases of software development. This proactive approach is more efficient and cost-effective than retrofitting accessibility features later in the development cycle.
2. Use Semantic HTML
For web-based applications, use semantic HTML elements to provide meaning and structure to your content. This helps assistive technologies understand and navigate the content more effectively.
Example of semantic HTML:
<header>
<h1>Welcome to Our Website</h1>
<nav>
<ul>
<li><a href="#home">Home</a></li>
<li><a href="#about">About</a></li>
<li><a href="#contact">Contact</a></li>
</ul>
</nav>
</header>
<main>
<article>
<h2>Main Content</h2>
<p>This is the main content of the page.</p>
</article>
</main>
<footer>
<p>© 2023 Our Company. All rights reserved.</p>
</footer>
3. Implement Keyboard Navigation
Ensure that all functionality is accessible via keyboard navigation. This is crucial for users who can’t use a mouse or touchscreen. Use proper focus management and provide visual indicators for focused elements.
Example of keyboard-accessible navigation:
<nav>
<ul>
<li><a href="#home" tabindex="0">Home</a></li>
<li><a href="#about" tabindex="0">About</a></li>
<li><a href="#contact" tabindex="0">Contact</a></li>
</ul>
</nav>
<style>
a:focus {
outline: 2px solid blue;
background-color: #f0f0f0;
}
</style>
4. Provide Alternative Text for Images
Include descriptive alternative text for images to convey their meaning to users who can’t see them. This is essential for users with visual impairments who use screen readers.
Example of an image with alternative text:
<img src="logo.png" alt="Company Logo: A blue circle with a white star inside">
5. Use ARIA Attributes When Necessary
Accessible Rich Internet Applications (ARIA) attributes can be used to provide additional information about the structure and functionality of web applications to assistive technologies. However, use ARIA judiciously and only when necessary, as native HTML elements are often sufficient.
Example of using ARIA for a custom toggle button:
<button id="toggleButton" aria-pressed="false">
Toggle Feature
</button>
<script>
const button = document.getElementById('toggleButton');
button.addEventListener('click', () => {
const isPressed = button.getAttribute('aria-pressed') === 'true';
button.setAttribute('aria-pressed', !isPressed);
});
</script>
6. Ensure Sufficient Color Contrast
Use color combinations that provide sufficient contrast between text and background. This helps users with visual impairments or color blindness to read the content easily. Tools like the WebAIM Contrast Checker can help you verify color contrast ratios.
7. Provide Captions and Transcripts
For audio and video content, provide captions and transcripts. This makes the content accessible to users who are deaf or hard of hearing, as well as those in noisy environments or who prefer reading to listening.
8. Use Clear and Consistent Navigation
Implement a clear and consistent navigation structure throughout your application. This helps users understand how to navigate your software and find the information they need.
9. Test with Assistive Technologies
Regularly test your software with various assistive technologies, such as screen readers, to ensure compatibility and identify any accessibility issues. Popular screen readers include NVDA (free) and JAWS for Windows, and VoiceOver for macOS and iOS.
10. Conduct User Testing
Involve users with disabilities in your testing process. Their feedback can provide invaluable insights into the real-world usability and accessibility of your software.
Tools and Resources for Accessible Software Development
There are numerous tools and resources available to help developers create accessible software. Here are some popular options:
1. Accessibility Testing Tools
- WAVE (Web Accessibility Evaluation Tool): A suite of evaluation tools for web accessibility
- axe: An accessibility testing engine for websites and applications
- Lighthouse: An open-source tool for improving web page quality, including accessibility
2. Color Contrast Checkers
- WebAIM Contrast Checker: A tool to check color contrast ratios
- Colour Contrast Analyser: A desktop application for checking color contrasts
3. Screen Readers
- NVDA: A free screen reader for Windows
- JAWS: A popular commercial screen reader for Windows
- VoiceOver: Built-in screen reader for macOS and iOS
4. Accessibility Guidelines and Standards
- Web Content Accessibility Guidelines (WCAG): Comprehensive guidelines for web accessibility
- Section 508: U.S. federal accessibility requirements
- WAI-ARIA: Specification for accessible rich internet applications
5. Learning Resources
- MDN Web Docs Accessibility Guide: Comprehensive guide to web accessibility
- Web Accessibility Initiative (WAI): Resources and strategies for web accessibility
- A11Y Project: A community-driven effort to make web accessibility easier
Accessibility and AlgoCademy
As a platform focused on coding education and programming skills development, AlgoCademy has a unique opportunity to incorporate accessibility principles into its curriculum and platform. Here are some ways AlgoCademy can emphasize the importance of accessibility:
1. Incorporate Accessibility into Coding Tutorials
Include accessibility best practices in coding tutorials and examples. This can help learners understand the importance of accessibility from the beginning of their coding journey.
2. Provide Accessibility-Focused Courses
Offer specific courses or modules on accessible software development. This can cover topics like WCAG guidelines, ARIA attributes, and testing with assistive technologies.
3. Emphasize Accessibility in Problem-Solving Exercises
Include accessibility considerations in algorithmic thinking and problem-solving exercises. For example, challenges could involve creating accessible user interfaces or optimizing code for screen reader compatibility.
4. Showcase Accessible Coding Practices
In coding examples and solutions, demonstrate accessible coding practices. This can include using semantic HTML, providing alternative text for images, and ensuring keyboard accessibility.
5. Integrate Accessibility Testing Tools
Incorporate accessibility testing tools into the AlgoCademy platform. This can help learners identify and fix accessibility issues in their code.
6. Highlight Accessibility in Technical Interview Preparation
Include accessibility-related questions and scenarios in technical interview preparation materials. This can help prepare learners for real-world development scenarios where accessibility is a key consideration.
Conclusion
Accessibility in software development is not just a nice-to-have feature; it’s a fundamental aspect of creating inclusive and user-friendly applications. By prioritizing accessibility, developers can ensure their software reaches a wider audience, complies with legal requirements, and provides a better user experience for everyone.
As the digital world continues to evolve, the importance of accessibility will only grow. By incorporating accessibility principles into coding education platforms like AlgoCademy, we can nurture a new generation of developers who understand and prioritize inclusive design from the outset of their careers.
Remember, accessibility is not a checklist to be completed at the end of development, but a mindset that should be integrated throughout the entire software development lifecycle. By embracing accessibility, we can create a more inclusive digital world that empowers all users, regardless of their abilities or limitations.
As you continue your journey in software development, whether through AlgoCademy or other resources, always keep accessibility in mind. It’s not just about compliance or reaching a wider audience; it’s about creating technology that truly serves and includes all members of our diverse global community.