The Illusion of Mastery: Why Understanding Isn’t Enough Without Application
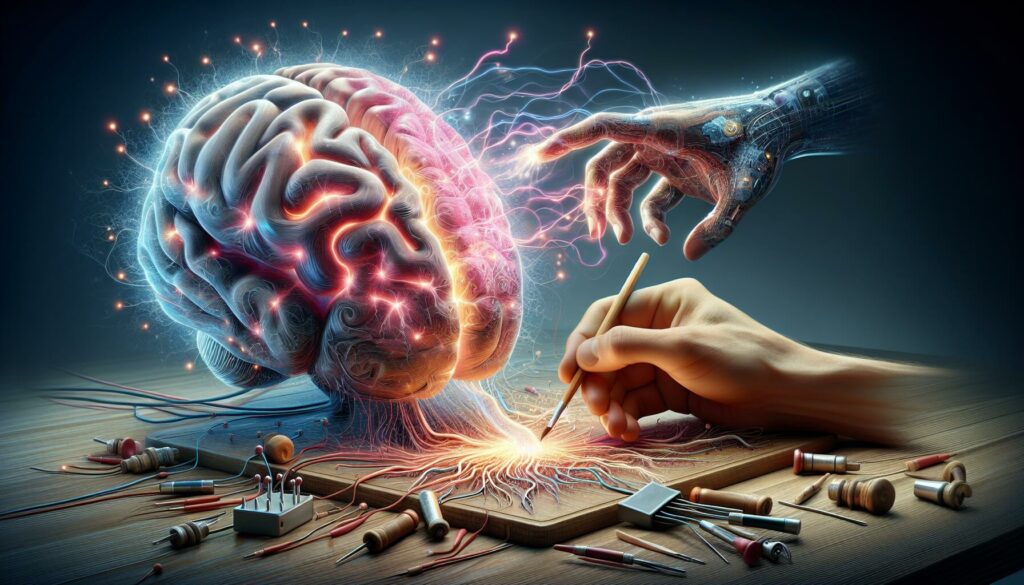
In the world of coding and programming, there’s a common misconception that plagues many aspiring developers: the illusion of mastery. This phenomenon occurs when learners believe they’ve fully grasped a concept or skill simply because they understand it theoretically. However, true mastery in programming requires more than just comprehension—it demands practical application and hands-on experience. In this article, we’ll explore why understanding alone isn’t sufficient and how platforms like AlgoCademy are addressing this gap in coding education.
The Gap Between Understanding and Application
When learning to code, it’s easy to fall into the trap of thinking that once you’ve read about a concept or watched a tutorial, you’ve mastered it. This is particularly true in the age of abundant online resources and video courses. While these materials are invaluable for introducing concepts and providing explanations, they often create a false sense of confidence.
Consider this scenario: You’ve just finished watching a series of videos on recursion in Python. The instructor’s explanations were clear, and you nodded along, feeling like you’ve got it all figured out. But when you sit down to solve a recursive problem on your own, you find yourself struggling to translate that understanding into working code.
This gap between understanding and application is where many learners stumble. It’s one thing to recognize and comprehend a concept when it’s presented to you; it’s another thing entirely to recall that information and apply it in a practical, problem-solving context.
The Importance of Active Learning in Coding
To bridge this gap, active learning is crucial. Active learning involves engaging with the material through practical exercises, coding challenges, and real-world projects. It’s the difference between passively consuming information and actively using that information to create something.
In the context of coding education, active learning might include:
- Writing code from scratch to solve specific problems
- Debugging existing code to find and fix errors
- Refactoring code to improve efficiency or readability
- Building small projects that incorporate multiple concepts
- Participating in coding challenges or hackathons
These activities force learners to apply their knowledge in practical scenarios, reinforcing their understanding and revealing areas where they may need more practice or clarification.
The Role of Deliberate Practice
Closely related to active learning is the concept of deliberate practice. Coined by psychologist K. Anders Ericsson, deliberate practice refers to a highly structured activity engaged in with the specific goal of improving performance. In coding, this means not just writing code, but doing so with intention and focus on improvement.
Deliberate practice in coding might involve:
- Setting specific goals for each coding session (e.g., implementing a particular algorithm)
- Focusing on challenging areas or concepts that you find difficult
- Seeking immediate feedback through code reviews or automated testing
- Reflecting on your code and identifying areas for improvement
- Gradually increasing the complexity of problems you tackle
By engaging in deliberate practice, learners can more effectively move from understanding to true mastery of coding concepts.
The AlgoCademy Approach: Bridging Understanding and Application
Recognizing the importance of practical application in coding education, platforms like AlgoCademy have developed approaches that go beyond passive learning. AlgoCademy focuses on providing interactive coding tutorials and resources that emphasize hands-on practice and real-world problem-solving.
Interactive Coding Tutorials
AlgoCademy’s interactive tutorials allow learners to write and run code directly in their browser. This immediate feedback loop helps reinforce concepts as they’re learned, bridging the gap between theory and practice. For example, when learning about sorting algorithms, users might be presented with this kind of interactive challenge:
def bubble_sort(arr):
# Your code here
pass
# Test your implementation
test_array = [64, 34, 25, 12, 22, 11, 90]
sorted_array = bubble_sort(test_array)
print(sorted_array)
Learners are encouraged to implement the bubble sort algorithm themselves, run the code, and see the results immediately. This hands-on approach helps solidify understanding and reveals any misconceptions or areas needing further study.
AI-Powered Assistance
One of AlgoCademy’s innovative features is its AI-powered assistance. This tool provides personalized guidance and hints when learners are stuck, mimicking the experience of having a tutor looking over your shoulder. For instance, if a learner is struggling with a recursive function, the AI might provide a hint like this:
# Hint: Remember, a recursive function should have:
# 1. A base case to stop the recursion
# 2. A recursive call that moves towards the base case
def factorial(n):
# Your code here
pass
This type of assistance helps learners apply their understanding in a guided manner, gradually building confidence and competence.
Step-by-Step Guidance
For more complex problems or algorithms, AlgoCademy offers step-by-step guidance. This approach breaks down problems into manageable chunks, allowing learners to tackle each part of a solution individually. For example, when learning about dynamic programming, a learner might encounter a problem like this:
# Problem: Implement the Fibonacci sequence using dynamic programming
def fibonacci_dp(n):
# Step 1: Initialize the base cases
# Your code here
# Step 2: Build the Fibonacci sequence bottom-up
# Your code here
# Step 3: Return the nth Fibonacci number
# Your code here
# Test your implementation
print(fibonacci_dp(10))
This structured approach helps learners apply their understanding systematically, reinforcing the problem-solving process that’s crucial in real-world programming scenarios.
Preparing for Technical Interviews: Beyond Theoretical Knowledge
One of AlgoCademy’s key focuses is preparing learners for technical interviews, particularly for major tech companies often referred to as FAANG (Facebook, Amazon, Apple, Netflix, Google). This preparation goes far beyond simply understanding algorithms and data structures—it requires the ability to apply this knowledge under pressure.
Technical interviews often involve solving coding problems on the spot, explaining your thought process, and optimizing your solutions. This requires not just theoretical knowledge, but the ability to quickly recall and apply that knowledge in a practical context.
To address this, AlgoCademy provides features like:
- Timed coding challenges that simulate interview conditions
- Problem sets that mimic the types of questions asked in FAANG interviews
- Opportunities to explain solutions verbally or in writing, practicing the communication skills needed in interviews
- Code optimization exercises to improve both time and space complexity
For example, a learner might encounter a challenge like this:
# You have 30 minutes to solve this problem:
# Given an array of integers, find two numbers such that they add up to a specific target number.
# Function Signature:
def two_sum(nums, target):
# Your code here
pass
# Example:
# Input: nums = [2, 7, 11, 15], target = 9
# Output: [0, 1] (because nums[0] + nums[1] = 2 + 7 = 9)
# Optimize your solution for time complexity.
# Be prepared to explain your approach and any trade-offs you considered.
This type of challenge not only tests the learner’s ability to implement a solution but also encourages them to think about optimization and be ready to articulate their thought process—key skills for succeeding in technical interviews.
The Power of Feedback and Iteration
Another crucial aspect of moving from understanding to mastery is the role of feedback and iteration. In traditional learning environments, feedback often comes long after the work is done, making it less effective. AlgoCademy and similar platforms address this by providing immediate feedback on code submissions.
This rapid feedback loop allows learners to:
- Identify and correct mistakes quickly
- Reinforce correct patterns and practices
- Iterate on their solutions to improve efficiency or readability
- Build confidence through successful implementations
For instance, after submitting a solution to a coding challenge, a learner might receive feedback like this:
# Feedback on your solution:
# Correctness: Passed all test cases
# Time Complexity: O(n^2) - Can be optimized
# Space Complexity: O(1) - Good job!
# Suggestion: Consider using a hash table to reduce time complexity to O(n).
# Example optimized solution:
def two_sum_optimized(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return []
This kind of detailed feedback not only confirms whether the solution works but also provides guidance on how to improve it. It encourages learners to revisit and refine their code, a crucial skill in real-world software development.
Building a Problem-Solving Mindset
Perhaps one of the most valuable outcomes of applying coding knowledge through platforms like AlgoCademy is the development of a problem-solving mindset. This goes beyond specific languages or algorithms—it’s about approaching challenges systematically and creatively.
Key aspects of this mindset include:
- Breaking down complex problems into smaller, manageable parts
- Identifying patterns and applying known solutions to new contexts
- Considering multiple approaches and evaluating trade-offs
- Debugging effectively by isolating issues and testing hypotheses
- Continuously learning and adapting to new technologies and paradigms
This problem-solving mindset is what separates proficient coders from true software engineers. It’s the ability to tackle unfamiliar challenges with confidence, even when the specific technology or problem domain is new.
The Continuous Journey of Learning
It’s important to recognize that in the field of programming, the journey from understanding to mastery is ongoing. Technology evolves rapidly, and new languages, frameworks, and paradigms emerge constantly. True mastery involves not just applying what you know, but continuously expanding your knowledge and skills.
Platforms like AlgoCademy support this continuous learning by:
- Regularly updating content to reflect current industry trends and best practices
- Providing resources for learning new languages and technologies
- Offering advanced topics and challenges for experienced developers
- Fostering a community where learners can share knowledge and collaborate
This emphasis on lifelong learning helps combat the illusion of mastery by reminding learners that there’s always more to discover and apply in the world of coding.
Conclusion: Embracing the Application of Knowledge
The illusion of mastery in coding education is a significant hurdle for many learners. Understanding concepts is undoubtedly important, but it’s only the first step on the path to true proficiency. To become skilled programmers and succeed in technical interviews, learners must actively apply their knowledge through practical coding, problem-solving, and real-world projects.
Platforms like AlgoCademy are at the forefront of addressing this challenge by providing interactive, hands-on learning experiences. Through features like AI-assisted coding tutorials, step-by-step problem-solving guidance, and interview preparation resources, they help bridge the gap between understanding and application.
As you continue your coding journey, remember that every line of code you write, every bug you fix, and every problem you solve is an opportunity to reinforce your understanding and move closer to true mastery. Embrace the challenges, seek out opportunities to apply your knowledge, and never stop learning. In the dynamic world of programming, the ability to apply and adapt your skills is what truly sets you apart.
So, the next time you feel confident after watching a coding tutorial or reading about a new algorithm, challenge yourself to put that knowledge into practice. Write some code, solve a problem, build a project. It’s in this application of knowledge that true learning occurs and the illusion of mastery gives way to genuine skill and confidence.