The Hardest Math Problems in the World and How They Relate to Coding
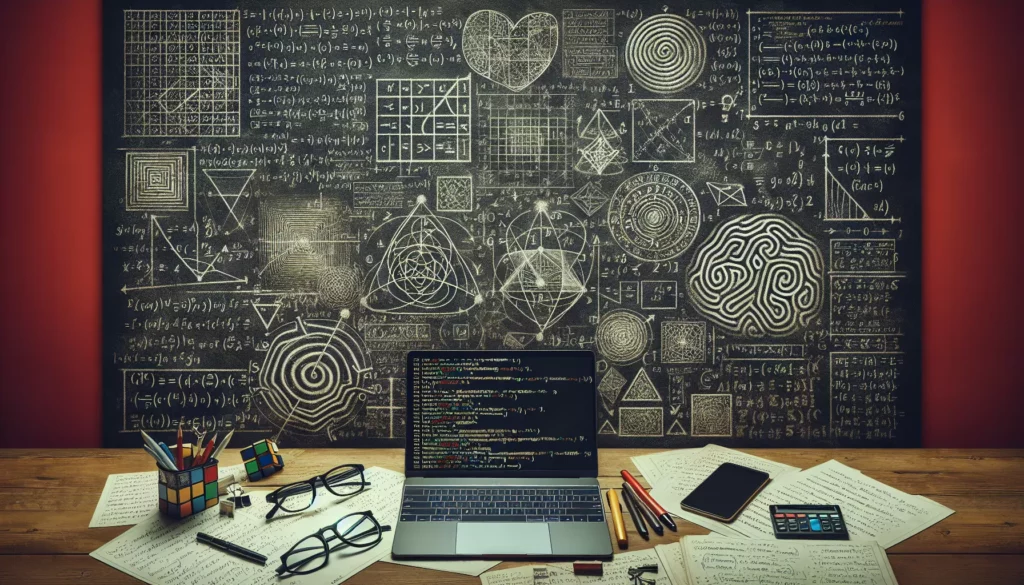
Mathematics has always been the foundation of computer science and coding. From the algorithms that power our daily applications to the complex systems that drive artificial intelligence, mathematical principles are at the heart of programming. In this article, we will explore some of the most challenging mathematical problems in the world and discuss how they connect to coding and computer science concepts.
For those learning to code or preparing for technical interviews at major tech companies, understanding these mathematical challenges can provide valuable insights into algorithmic thinking and problem solving approaches. Let’s dive into the world of mathematical complexity and see how it relates to our journey as programmers.
What Makes a Math Problem “Hard”?
Before diving into specific problems, it’s worth considering what makes a mathematical problem difficult. Hardness in mathematics can be characterized by several factors:
- Complexity of concepts involved
- Length of proof or solution
- Counterintuitive nature
- Historical resistance to solution
- Computational difficulty
Many of these same characteristics apply to challenging coding problems as well. The most difficult programming challenges often involve complex algorithms, require extensive code, produce surprising results, or have resisted efficient solutions for years.
The P vs NP Problem: Where Math Meets Computer Science
Perhaps no mathematical problem is more relevant to computer science than the P vs NP problem. This unsolved question asks whether every problem whose solution can be quickly verified by a computer can also be quickly solved by a computer.
In more technical terms: if a solution to a problem can be verified in polynomial time (P), can the solution also be found in polynomial time (NP)? This question has profound implications for algorithm design and efficiency.
For programmers, this problem directly relates to the efficiency of algorithms. When tackling coding challenges, we often face problems where:
- We can easily check if a proposed solution works (polynomial time verification)
- But finding that solution efficiently seems impossible (potentially exponential time solution)
Examples include many optimization problems like the Traveling Salesman Problem or the Knapsack Problem. These challenges appear frequently in technical interviews at major tech companies.
If P were proven equal to NP, it would revolutionize our approach to algorithm design, potentially making currently “intractable” problems solvable with efficient algorithms.
The Riemann Hypothesis: Prime Numbers and Cryptography
The Riemann Hypothesis is one of the most famous unsolved problems in mathematics. At its core, it deals with the distribution of prime numbers, which are fundamental to modern cryptography and secure communication protocols.
The hypothesis proposes that all non-trivial zeros of the Riemann zeta function have a real part equal to 1/2. While that may sound abstract, its implications for understanding prime number distribution are enormous.
For programmers, especially those working in cybersecurity, this has direct applications:
- Public key cryptography relies on the difficulty of factoring large numbers into primes
- RSA encryption, widely used in secure communications, depends on prime number properties
- Efficient algorithms for working with primes impact numerous applications
When implementing security features in your code, you’re leveraging the mathematical properties that the Riemann Hypothesis helps describe. A proof of this hypothesis could potentially lead to more efficient algorithms for working with prime numbers.
The Navier-Stokes Existence and Smoothness Problem
This problem concerns equations that describe the motion of fluid substances. While seemingly unrelated to coding, computational fluid dynamics is a major field in computer science that directly implements these equations.
The challenge asks whether solutions to the Navier-Stokes equations always exist in three dimensions and whether they remain smooth indefinitely or develop singularities.
For programmers working in:
- Physics simulations
- Game development (realistic fluid motion)
- Weather prediction systems
- Aerodynamics modeling
Understanding the mathematical limits of these equations directly impacts how we approach their implementation in code. The problem highlights the challenges of translating continuous mathematical models into discrete computational algorithms.
The Collatz Conjecture: Simple to State, Hard to Prove
Some of the hardest math problems are deceptively simple to state. The Collatz Conjecture involves a sequence defined by a simple rule:
- If a number is even, divide it by 2
- If a number is odd, multiply it by 3 and add 1
- Repeat this process with the resulting number
The conjecture states that no matter what positive integer you start with, this sequence will always eventually reach 1.
This problem is perfect for coding implementation. Here’s how you might code it in Python:
def collatz_sequence(n):
sequence = [n]
while n != 1:
if n % 2 == 0: # If n is even
n = n // 2
else: # If n is odd
n = 3 * n + 1
sequence.append(n)
return sequence
# Example usage
print(collatz_sequence(27))
Despite its simplicity, no one has been able to prove that this conjecture holds for all positive integers. It demonstrates how even seemingly straightforward recursive processes can lead to deep mathematical questions.
For programmers, this problem highlights:
- The power of recursion and iterative processes
- How simple rules can generate complex behavior
- The challenge of proving properties about algorithms
Computational Complexity and the Four Color Theorem
The Four Color Theorem states that any map can be colored using at most four colors in such a way that no adjacent regions share the same color. While this problem has been solved, its proof is significant because it was the first major mathematical theorem to be proved using a computer.
The proof required checking thousands of cases, which was only feasible with computational assistance. This represented a significant shift in how mathematical proofs could be approached.
For programmers, this theorem relates to graph coloring algorithms, which have applications in:
- Scheduling problems
- Register allocation in compilers
- Frequency assignment in wireless networks
- Resource allocation problems
Implementing a graph coloring algorithm is a common coding challenge:
def is_safe(graph, vertex, color, colors):
for i in range(len(graph)):
if graph[vertex][i] == 1 and colors[i] == color:
return False
return True
def graph_coloring(graph, m, vertex, colors, vertices):
if vertex == vertices:
return True
for color in range(1, m + 1):
if is_safe(graph, vertex, color, colors):
colors[vertex] = color
if graph_coloring(graph, m, vertex + 1, colors, vertices):
return True
colors[vertex] = 0
return False
# Example usage for a simple graph
graph = [
[0, 1, 1, 1],
[1, 0, 1, 0],
[1, 1, 0, 1],
[1, 0, 1, 0]
]
vertices = 4
colors = [0] * vertices
m = 3 # Number of colors
if graph_coloring(graph, m, 0, colors, vertices):
print("Solution exists: ", colors)
else:
print("No solution exists")
Fermat’s Last Theorem: Persistence Pays Off
Fermat’s Last Theorem states that no three positive integers a, b, and c can satisfy the equation a^n + b^n = c^n for any integer value of n greater than 2. This problem remained unsolved for over 350 years until Andrew Wiles finally proved it in 1994.
The journey to solve this problem led to the development of entire new branches of mathematics, including algebraic number theory and modular forms.
For programmers, this illustrates:
- The value of persistence in solving difficult problems
- How attempting to solve one problem can lead to new discoveries and approaches
- The importance of building on existing knowledge
When tackling complex coding challenges, we often find that breaking down problems and developing new approaches is essential for success.
The Halting Problem: Theoretical Limits of Computation
The Halting Problem, formulated by Alan Turing, asks whether it’s possible to determine, from a description of an arbitrary computer program and an input, whether the program will finish running (halt) or continue to run forever.
Turing proved that a general algorithm to solve the halting problem for all possible program-input pairs cannot exist. This was one of the first examples of a decision problem that is algorithmically unsolvable.
For programmers, this has profound implications:
- It establishes fundamental limits on what computers can do
- It demonstrates that not all well-defined problems are computable
- It relates to the challenges of debugging and analyzing code behavior
Understanding the Halting Problem helps programmers appreciate the theoretical boundaries of computation and the inherent challenges in program analysis and verification.
The Millennium Prize Problems: Mathematics’ Greatest Challenges
In 2000, the Clay Mathematics Institute established seven Millennium Prize Problems, each with a $1 million reward for solution. These represent some of the most difficult unsolved problems in mathematics:
- P vs NP (discussed earlier)
- Riemann Hypothesis (discussed earlier)
- Yang-Mills Existence and Mass Gap
- Navier-Stokes Existence and Smoothness (discussed earlier)
- Hodge Conjecture
- Poincaré Conjecture (solved by Grigori Perelman in 2003)
- Birch and Swinnerton-Dyer Conjecture
Of these, only the Poincaré Conjecture has been solved. These problems represent the frontier of mathematical research and have implications across various scientific fields, including computer science.
The Relationship Between Math and Coding Skills
For aspiring programmers and those preparing for technical interviews, understanding the relationship between mathematics and coding is crucial:
Algorithm Design and Analysis
Mathematical principles underpin algorithm design and analysis. Concepts like time complexity (Big O notation), space complexity, and algorithmic efficiency are fundamentally mathematical.
When preparing for technical interviews at major tech companies, candidates are often expected to analyze and optimize algorithms using these mathematical frameworks.
Problem Solving Approaches
Mathematics and coding share similar problem-solving approaches:
- Breaking complex problems into smaller, manageable parts
- Recognizing patterns and applying known solutions
- Using abstraction to simplify complex scenarios
- Applying logical reasoning to derive solutions
Developing mathematical thinking directly enhances your coding abilities and vice versa.
Specific Mathematical Areas Relevant to Coding
Several branches of mathematics are particularly relevant to programmers:
- Discrete Mathematics: Set theory, combinatorics, graph theory
- Linear Algebra: Essential for graphics programming, machine learning, and data analysis
- Probability and Statistics: Crucial for data science, AI, and algorithmic analysis
- Number Theory: Fundamental to cryptography and security
- Logic: The foundation of programming language design and formal verification
How to Approach Difficult Coding Problems
Drawing inspiration from how mathematicians tackle hard problems, here are strategies for approaching difficult coding challenges:
1. Understand the Problem Thoroughly
Before attempting a solution, make sure you fully understand the problem. Break it down, identify constraints, and clarify expected inputs and outputs.
2. Start with Simple Cases
Begin by solving the problem for simple, specific cases. This can provide insights into the general solution.
3. Look for Patterns and Analogies
Try to identify patterns or relate the problem to ones you’ve solved before. Many coding challenges are variations of classic problems.
4. Divide and Conquer
Break the problem into smaller subproblems that are easier to solve independently.
5. Use Visualization
Diagrams, flowcharts, and other visual aids can help clarify complex problems and potential solutions.
6. Consider Multiple Approaches
Don’t commit to the first solution that comes to mind. Consider different algorithms and data structures to find the most efficient approach.
7. Test and Refine
Implement your solution, test it thoroughly, and refine it based on the results.
Conclusion
The hardest math problems in the world share many characteristics with the most challenging coding problems. Both require deep analytical thinking, creativity, persistence, and a systematic approach.
For those developing their programming skills or preparing for technical interviews, studying these mathematical challenges can provide valuable insights into problem-solving approaches and algorithmic thinking. The connection between mathematics and computer science is not just theoretical; it’s practical and fundamental to becoming an effective programmer.
Remember that even the most difficult problems become approachable when broken down into manageable parts. Whether you’re tackling the Collatz Conjecture or a complex algorithm design challenge in your next coding interview, the principles of mathematical problem-solving will serve you well.
As you continue your journey in coding education, embrace the mathematical foundations that underpin computer science. They will not only help you solve immediate coding challenges but also provide a framework for addressing the complex programming problems you’ll encounter throughout your career.