The Ethics of Software Development: Navigating the Digital Moral Compass
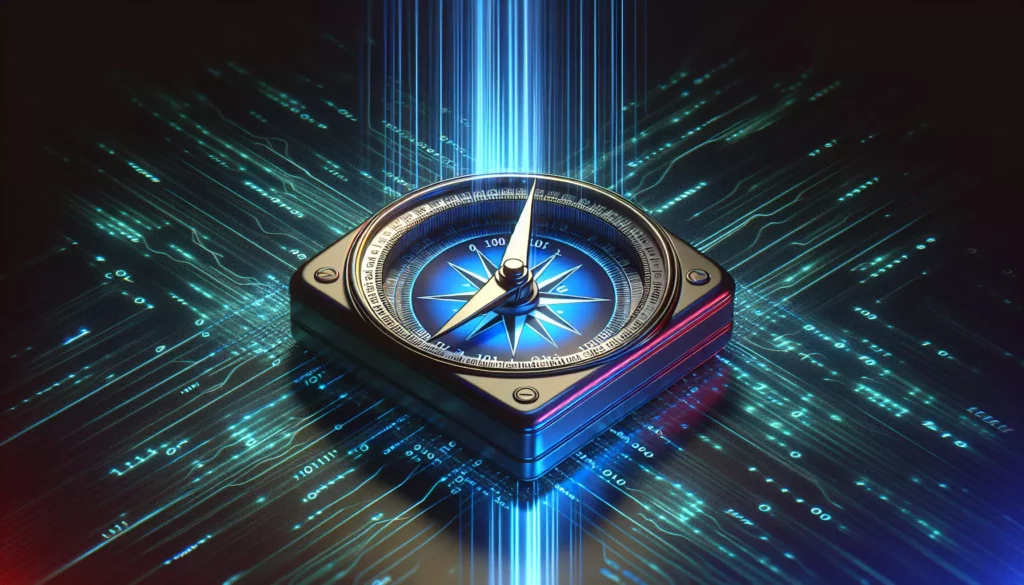
In the rapidly evolving world of technology, software development stands at the forefront of innovation, shaping the digital landscape that impacts billions of lives daily. As we progress in our coding education and hone our programming skills, it’s crucial to understand that with great power comes great responsibility. The ethics of software development is a topic that’s becoming increasingly important, not just for seasoned professionals but also for beginners embarking on their coding journey.
At AlgoCademy, where we focus on nurturing coding skills from beginner level to technical interview preparation, we believe that ethical considerations should be an integral part of a developer’s education. As you work through interactive coding tutorials and tackle algorithmic challenges, it’s essential to keep in mind the broader implications of the code you write. In this comprehensive guide, we’ll explore the multifaceted world of software development ethics, providing insights that will help you navigate the moral complexities of the digital realm.
Understanding the Importance of Ethics in Software Development
Before diving into specific ethical considerations, it’s crucial to understand why ethics matter in software development. In today’s interconnected world, software touches nearly every aspect of our lives – from the apps we use daily to the systems that manage our finances, healthcare, and even democratic processes. As developers, we’re not just writing code; we’re shaping the tools and platforms that influence human behavior, decision-making, and societal structures.
Consider these key reasons why ethics are paramount in software development:
- Impact on Society: Software can influence social norms, communication patterns, and even political landscapes.
- Privacy Concerns: With the increasing collection and use of personal data, developers must be mindful of privacy implications.
- Security Responsibilities: As cyber threats evolve, ethical coding practices are crucial for protecting users and systems.
- Algorithmic Bias: AI and machine learning algorithms can perpetuate or amplify societal biases if not carefully designed.
- Accessibility: Ethical development ensures that software is inclusive and accessible to all users, including those with disabilities.
Core Ethical Principles in Software Development
As you progress in your coding education, whether you’re working through AlgoCademy’s interactive tutorials or preparing for technical interviews with major tech companies, it’s essential to internalize these core ethical principles:
1. Transparency
Transparency in software development means being open about what your software does, how it works, and what data it collects. This principle is crucial for building trust with users and stakeholders.
Practical Application: When developing a feature that collects user data, clearly communicate this to users and explain how the data will be used. For example:
<!-- User data collection notice -->
<div class="data-notice">
<p>This feature collects your location data to improve local recommendations. Your data is anonymized and never sold to third parties.</p>
<button onclick="acceptDataCollection()">Accept</button>
<button onclick="declineDataCollection()">Decline</button>
</div>
2. Privacy
Respecting user privacy is not just about compliance with regulations like GDPR; it’s about fundamentally valuing and protecting user data.
Practical Application: Implement data minimization principles, collecting only what’s necessary for the function of your software. Use encryption for sensitive data:
// Example of encrypting user data before storage
const crypto = require('crypto');
function encryptUserData(data, key) {
const cipher = crypto.createCipher('aes-256-cbc', key);
let encrypted = cipher.update(data, 'utf8', 'hex');
encrypted += cipher.final('hex');
return encrypted;
}
3. Accessibility
Ethical software development means creating applications that are usable by people of all abilities. This includes following web accessibility guidelines and considering diverse user needs.
Practical Application: Implement proper semantic HTML and ARIA attributes to make your web applications screen-reader friendly:
<!-- Accessible navigation menu -->
<nav aria-label="Main Navigation">
<ul>
<li><a href="#home" aria-current="page">Home</a></li>
<li><a href="#about">About</a></li>
<li><a href="#contact">Contact</a></li>
</ul>
</nav>
4. Security
Ethical developers prioritize the security of their software, protecting user data and system integrity from potential threats.
Practical Application: Implement secure coding practices, such as input validation to prevent SQL injection attacks:
// Example of parameterized query to prevent SQL injection
const mysql = require('mysql');
const connection = mysql.createConnection({
// connection details
});
function getUserData(userId) {
return new Promise((resolve, reject) => {
const query = 'SELECT * FROM users WHERE id = ?';
connection.query(query, [userId], (error, results) => {
if (error) reject(error);
resolve(results);
});
});
}
5. Fairness and Non-Discrimination
Ethical software should be free from bias and discrimination, treating all users fairly regardless of their background or characteristics.
Practical Application: When developing AI or machine learning models, regularly audit your training data and results for potential biases:
// Example of a simple bias check in a machine learning model
function checkForBias(predictions, protectedAttribute) {
const groups = {};
predictions.forEach((pred, index) => {
const group = protectedAttribute[index];
if (!groups[group]) groups[group] = [];
groups[group].push(pred);
});
const groupAverages = Object.entries(groups).map(([group, preds]) => {
return { group, average: preds.reduce((a, b) => a + b) / preds.length };
});
console.log('Group averages:', groupAverages);
// Further analysis would be needed to determine if differences are significant
}
Ethical Challenges in Modern Software Development
As you advance in your coding skills and prepare for technical interviews, you’ll encounter more complex ethical challenges. Here are some of the pressing issues in modern software development:
1. AI and Machine Learning Ethics
The rise of AI and machine learning brings unprecedented ethical challenges. As developers, we must be aware of:
- Algorithmic Bias: Ensuring that AI systems don’t perpetuate or amplify societal biases.
- Transparency in AI Decision-Making: Making AI systems explainable and accountable.
- Data Privacy in AI: Balancing the need for large datasets with individual privacy rights.
Practical Consideration: When developing AI systems, implement techniques for fairness-aware machine learning and explainable AI (XAI). For instance, use tools like IBM’s AI Fairness 360 toolkit to detect and mitigate bias in your models.
2. Data Ethics and Big Data
The era of big data presents unique ethical challenges:
- Data Collection Ethics: Ensuring that data is collected ethically and with informed consent.
- Data Usage and Sharing: Being responsible with how collected data is used and shared.
- Data Retention: Implementing ethical policies for data storage and deletion.
Practical Consideration: Implement data governance frameworks in your projects. For example, create clear data lifecycle policies that include regular audits of data usage and deletion of unnecessary data:
// Example of a simple data retention policy implementation
class DataRetentionPolicy {
constructor(retentionPeriodDays) {
this.retentionPeriodDays = retentionPeriodDays;
}
shouldDeleteData(dataCreationDate) {
const currentDate = new Date();
const dataAge = (currentDate - dataCreationDate) / (1000 * 60 * 60 * 24);
return dataAge > this.retentionPeriodDays;
}
auditAndCleanData(database) {
database.forEach(record => {
if (this.shouldDeleteData(record.creationDate)) {
// Implement secure deletion logic here
console.log(`Deleting record ${record.id} due to retention policy`);
}
});
}
}
// Usage
const retentionPolicy = new DataRetentionPolicy(365); // 1 year retention
retentionPolicy.auditAndCleanData(myDatabase);
3. Privacy in the Age of Surveillance Capitalism
As developers, we often work on projects that involve user data. It’s crucial to consider:
- Data Minimization: Collecting only the data necessary for the application’s function.
- User Control: Giving users control over their data and how it’s used.
- Transparency in Data Practices: Clearly communicating data collection and usage policies.
Practical Consideration: Implement privacy by design principles in your development process. For example, create user-friendly privacy controls:
<!-- Example of user privacy controls in HTML/JS -->
<div id="privacy-settings">
<h2>Privacy Settings</h2>
<label>
<input type="checkbox" id="allow-analytics" onchange="updatePrivacySettings()">
Allow analytics tracking
</label>
<label>
<input type="checkbox" id="allow-personalization" onchange="updatePrivacySettings()">
Allow personalized recommendations
</label>
</div>
<script>
function updatePrivacySettings() {
const allowAnalytics = document.getElementById('allow-analytics').checked;
const allowPersonalization = document.getElementById('allow-personalization').checked;
// Update user preferences in backend
fetch('/api/update-privacy-settings', {
method: 'POST',
body: JSON.stringify({ allowAnalytics, allowPersonalization }),
headers: { 'Content-Type': 'application/json' }
});
}
</script>
4. Ethical Considerations in Open Source Development
Open source development brings its own set of ethical considerations:
- License Compliance: Ensuring proper use and attribution of open source components.
- Community Responsibility: Contributing back to the open source community.
- Ethical Use of Open Source Software: Considering the potential uses and misuses of your open source contributions.
Practical Consideration: When using open source components, always respect the licenses and contribute back when possible. For example, include a clear license file in your projects:
// Example LICENSE file content (MIT License)
MIT License
Copyright (c) [year] [fullname]
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE.
Implementing Ethical Practices in Your Development Process
As you progress in your coding education and prepare for technical interviews, it’s important to integrate ethical considerations into your development process. Here are some practical steps:
1. Ethical Code Reviews
Incorporate ethical considerations into your code review process. Along with checking for bugs and efficiency, review code for potential ethical issues:
- Privacy concerns in data handling
- Accessibility features
- Potential for bias in algorithms
- Security vulnerabilities
Practical Tip: Create an ethical code review checklist to ensure consistent evaluation:
// Ethical Code Review Checklist
1. Data Handling:
[ ] Is user data properly encrypted?
[ ] Is data collection minimized to only what's necessary?
[ ] Are there clear user consent mechanisms for data collection?
2. Accessibility:
[ ] Does the code follow WCAG guidelines?
[ ] Are there appropriate ARIA attributes for complex UI elements?
[ ] Is the color contrast sufficient for readability?
3. Algorithmic Fairness:
[ ] Have we checked for potential biases in our algorithms?
[ ] Is there a process for regular auditing of AI/ML models?
4. Security:
[ ] Are inputs properly sanitized to prevent injection attacks?
[ ] Are authentication and authorization mechanisms robust?
[ ] Is sensitive information properly protected in logs and error messages?
5. Transparency:
[ ] Is the functionality of the code clearly documented?
[ ] Are there clear explanations for users about how their data is used?
6. Open Source Compliance:
[ ] Are all open source libraries properly attributed?
[ ] Do we comply with the licenses of all used libraries?
2. Ethical Design Thinking
Incorporate ethical considerations from the very beginning of your design process:
- Conduct stakeholder analysis to understand the impact of your software on different groups
- Use ethical frameworks like the Ethical OS Toolkit to anticipate potential issues
- Implement user stories that include ethical considerations
Practical Tip: Include ethical considerations in your user stories:
// Example of an ethically-conscious user story
As a user with visual impairments,
I want to be able to navigate the application using a screen reader,
So that I can access all features independently.
Acceptance Criteria:
1. All interactive elements are properly labeled with ARIA attributes
2. The application passes WCAG 2.1 AA compliance checks
3. Color contrast ratios meet accessibility standards
4. Keyboard navigation is fully supported for all features
3. Continuous Ethical Education
The field of technology ethics is constantly evolving. Stay informed about the latest ethical issues and best practices:
- Attend ethics in technology conferences and workshops
- Read publications on tech ethics and responsible innovation
- Participate in online courses focused on ethics in software development
Practical Tip: Set up a regular learning schedule for ethical education. For example:
// Example of a monthly ethical learning plan
Month 1: AI Ethics
- Read: "Weapons of Math Destruction" by Cathy O'Neil
- Course: "Ethics and Law in Data and Analytics" on Coursera
- Project: Implement a bias detection algorithm in a machine learning model
Month 2: Privacy and Data Protection
- Read: "The Age of Surveillance Capitalism" by Shoshana Zuboff
- Workshop: Attend a GDPR compliance workshop
- Project: Conduct a privacy impact assessment on a current project
Month 3: Accessibility in Software Design
- Read: "A Web for Everyone" by Sarah Horton and Whitney Quesenbery
- Course: "Web Accessibility" on edX
- Project: Perform an accessibility audit on your portfolio website
4. Ethical Decision-Making Frameworks
Develop and use ethical decision-making frameworks to guide your development process. These frameworks can help you navigate complex ethical dilemmas:
- Utilize ethical matrices to weigh different stakeholder interests
- Implement ethics-based risk assessment in your project planning
- Create ethical guidelines specific to your team or organization
Practical Tip: Create an ethical decision-making flowchart for your development team:
<!-- Example of an ethical decision-making flowchart in HTML -->
<div class="flowchart">
<div class="step">
<p>Identify the ethical issue</p>
<span class="arrow">↓</span>
</div>
<div class="step">
<p>Gather relevant information</p>
<span class="arrow">↓</span>
</div>
<div class="step">
<p>Consider stakeholder perspectives</p>
<span class="arrow">↓</span>
</div>
<div class="step">
<p>Evaluate options using ethical principles</p>
<span class="arrow">↓</span>
</div>
<div class="step">
<p>Make a decision</p>
<span class="arrow">↓</span>
</div>
<div class="step">
<p>Implement and monitor the decision</p>
<span class="arrow">↓</span>
</div>
<div class="step">
<p>Reflect and learn from the outcome</p>
</div>
</div>
The Role of Ethics in Technical Interviews and Job Searches
As you prepare for technical interviews, especially with major tech companies, it’s important to understand that ethical considerations are increasingly becoming part of the interview process. Here’s how to incorporate ethics into your interview preparation:
1. Be Prepared to Discuss Ethical Scenarios
Interviewers may present ethical dilemmas related to software development. Practice discussing these scenarios:
- How would you handle a request to implement a feature that could potentially violate user privacy?
- What steps would you take if you discovered a bias in an AI algorithm your team developed?
- How would you approach a situation where you’re asked to cut corners on security to meet a deadline?
Practical Tip: Develop a framework for answering ethical questions in interviews:
// Framework for Answering Ethical Questions in Interviews
1. Acknowledge the ethical dilemma
2. Identify the stakeholders involved
3. Consider the potential consequences of different actions
4. Reference relevant ethical principles or guidelines
5. Propose a solution that balances ethical considerations with practical needs
6. Explain how you would implement and monitor the solution
7. Show willingness to seek advice or escalate if necessary
2. Showcase Ethical Projects in Your Portfolio
When building your coding portfolio, include projects that demonstrate your commitment to ethical development:
- Develop an open-source tool that promotes digital privacy
- Create an accessible web application that adheres to WCAG guidelines
- Build a demo of an ethically-designed AI system with explainable decision-making
Practical Tip: Add an “Ethical Considerations” section to your project documentation:
<!-- Example of Ethical Considerations in Project README -->
# Project Name
## Ethical Considerations
This project was developed with the following ethical principles in mind:
1. **Privacy**: User data is encrypted and stored securely. We implement data minimization practices.
2. **Accessibility**: The application meets WCAG 2.1 AA standards and has been tested with screen readers.
3. **Fairness**: Our recommendation algorithm has been audited for bias and includes diverse representation in its training data.
4. **Transparency**: We provide clear explanations of how user data is used and how AI-driven features make decisions.
5. **Security**: Regular security audits are conducted, and we have a responsible disclosure policy for vulnerabilities.
For more details on our ethical practices, see [ETHICS.md](./ETHICS.md).
3. Ask Ethical Questions During Interviews
Demonstrate your commitment to ethical development by asking thoughtful questions about the company’s ethical practices:
- How does the company approach issues of algorithmic bias?
- What processes are in place for ethical decision-making in product development?
- How does the company balance user privacy with data-driven features?
Practical Tip: Prepare a list of ethical questions for different interview scenarios:
// Example Ethical Questions for Tech Interviews
For Product Managers:
1. How do you incorporate ethical considerations into the product roadmap?
2. What processes are in place to ensure diverse perspectives are considered in product decisions?
For Engineering Managers:
1. How does the team approach ethical code reviews?
2. What training or resources are provided to engineers on ethical development practices?
For Data Scientists:
1. How do you ensure fairness and prevent bias in your machine learning models?
2. What ethical guidelines do you follow when collecting and using user data?
For UX Designers:
1. How do you ensure your designs are accessible to users with diverse abilities?
2. What methods do you use to get informed consent for user research and testing?
For C-level Executives:
1. How does ethics factor into the company's long-term strategy and vision?
2. What mechanisms are in place to ensure ethical accountability across the organization?
Conclusion: Embracing Ethical Software Development
As we’ve explored throughout this guide, the ethics of software development is a crucial aspect of our field that goes hand in hand with technical proficiency. As you continue your journey with AlgoCademy, from mastering coding basics to preparing for technical interviews with top tech companies, remember that ethical considerations should be woven into every line of code you write and every system you design.
Ethical software development is not just about following rules or avoiding negative consequences; it’s about actively contributing to a better digital world. It’s about creating technology that respects human rights, promotes fairness, and enhances the well-being of users and society at large.
By embracing ethical practices in your development process, you’re not only becoming a better programmer but also a responsible digital citizen. You’re preparing yourself for a career where you can make a positive impact, tackle complex challenges, and help shape the future of technology in a way that aligns with human values and societal good.
Remember, the code you write has the power to influence lives. Use that power wisely, ethically, and always with the best interests of users and society in mind. As you continue to grow in your coding skills, let ethics be your guiding principle, your north star in the vast and exciting world of software development.
Keep learning, keep coding, and most importantly, keep questioning the ethical implications of your work. The future of technology – and in many ways, the future of our society – depends on developers like you who are committed to not just writing good code, but writing code for good.