The Difference Between a Frontend vs Backend Developer Interview
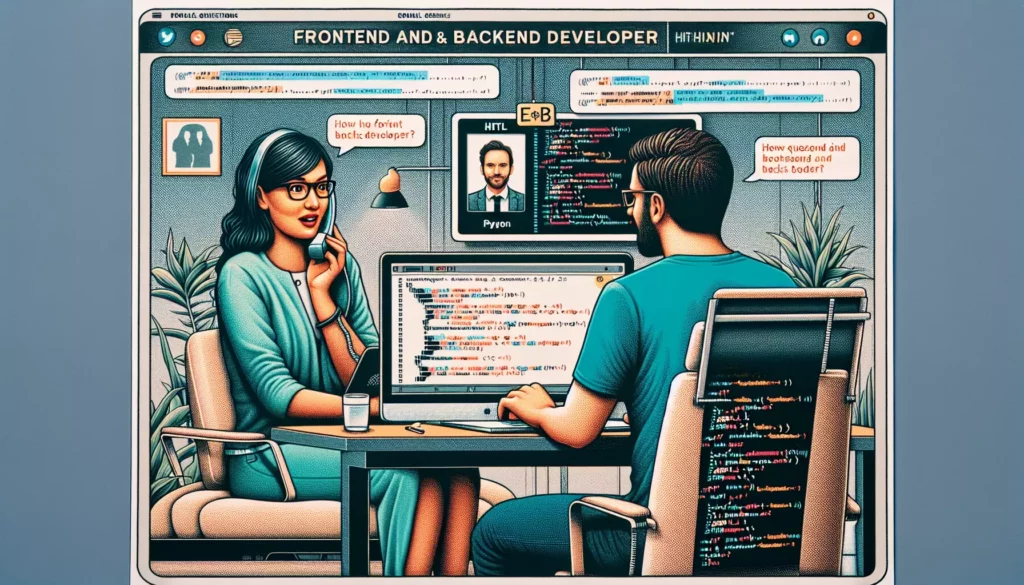
In the world of web development, there are two primary domains that developers specialize in: frontend and backend. While both are crucial for creating functional and user-friendly websites and applications, the skills required and the interview processes for these roles can differ significantly. In this comprehensive guide, we’ll explore the key differences between frontend and backend developer interviews, helping you prepare effectively for your next career move in the tech industry.
Understanding Frontend and Backend Development
Before diving into the interview differences, let’s briefly recap what frontend and backend development entail:
Frontend Development
Frontend development focuses on the user-facing side of web applications. It involves creating the visual elements that users interact with directly in their web browsers. Frontend developers work with technologies such as:
- HTML (Hypertext Markup Language)
- CSS (Cascading Style Sheets)
- JavaScript
- Frontend frameworks and libraries (e.g., React, Vue.js, Angular)
Backend Development
Backend development deals with the server-side of web applications. It involves managing databases, server logic, and application programming interfaces (APIs). Backend developers typically work with:
- Server-side programming languages (e.g., Python, Java, Ruby, Node.js)
- Databases (e.g., MySQL, PostgreSQL, MongoDB)
- Server management and deployment
- API development and integration
Key Differences in Interview Focus
When it comes to interviews, the focus areas for frontend and backend developers can vary significantly. Here are the main differences you can expect:
1. Technical Skills Assessment
Frontend Developer Interviews
Frontend interviews often emphasize:
- HTML/CSS proficiency
- JavaScript knowledge and DOM manipulation
- Responsive design principles
- Frontend framework expertise (e.g., React, Vue.js, Angular)
- Browser compatibility issues
- Web performance optimization
Backend Developer Interviews
Backend interviews typically focus on:
- Server-side programming language proficiency
- Database design and management
- API development and RESTful principles
- Server architecture and scalability
- Security best practices
- Data structures and algorithms
2. Coding Challenges
Frontend Developer Interviews
Coding challenges for frontend developers often involve:
- Building responsive layouts
- Implementing interactive UI components
- Solving JavaScript puzzles
- Optimizing frontend performance
- Debugging CSS issues
Example frontend coding challenge:
<!-- Create a responsive navigation menu that collapses into a hamburger menu on mobile devices -->
<nav>
<ul>
<li><a href="#">Home</a></li>
<li><a href="#">About</a></li>
<li><a href="#">Services</a></li>
<li><a href="#">Contact</a></li>
</ul>
</nav>
Backend Developer Interviews
Coding challenges for backend developers typically include:
- Designing and implementing APIs
- Optimizing database queries
- Solving algorithmic problems
- Implementing authentication and authorization
- Handling concurrent requests and scalability issues
Example backend coding challenge:
// Implement a function to find the nth Fibonacci number using dynamic programming
function fibonacci(n) {
// Your code here
}
console.log(fibonacci(10)); // Should output 55
3. System Design Questions
Frontend Developer Interviews
Frontend system design questions often focus on:
- Component architecture
- State management
- Application performance optimization
- Progressive enhancement and graceful degradation
- Accessibility considerations
Example frontend system design question:
“Design a scalable and performant image gallery component that can handle thousands of images with lazy loading and infinite scrolling.”
Backend Developer Interviews
Backend system design questions typically involve:
- Distributed systems architecture
- Database sharding and replication
- Caching strategies
- Load balancing and scaling
- Microservices architecture
Example backend system design question:
“Design a URL shortening service like bit.ly that can handle millions of requests per day.”
4. Tools and Technologies
Frontend Developer Interviews
Frontend interviews may inquire about experience with:
- Version control systems (e.g., Git)
- Package managers (e.g., npm, Yarn)
- Build tools (e.g., Webpack, Babel)
- CSS preprocessors (e.g., Sass, Less)
- Frontend testing frameworks (e.g., Jest, Cypress)
- Browser developer tools
Backend Developer Interviews
Backend interviews often explore knowledge of:
- Server management tools (e.g., Docker, Kubernetes)
- CI/CD pipelines
- Message queues (e.g., RabbitMQ, Kafka)
- Caching systems (e.g., Redis, Memcached)
- Backend testing frameworks (e.g., JUnit, pytest)
- Monitoring and logging tools (e.g., Prometheus, ELK stack)
5. Soft Skills and Collaboration
While both frontend and backend developer interviews assess soft skills, there may be slight differences in emphasis:
Frontend Developer Interviews
May focus more on:
- Collaboration with designers
- User empathy and understanding of UX principles
- Ability to translate design mockups into functional interfaces
- Communication skills for explaining technical concepts to non-technical stakeholders
Backend Developer Interviews
May emphasize:
- Collaboration with system administrators and DevOps teams
- Ability to optimize for performance and scalability
- Understanding of security best practices
- Communication skills for discussing system architecture and trade-offs
Preparing for Your Interview
Regardless of whether you’re interviewing for a frontend or backend position, there are some common strategies you can employ to increase your chances of success:
1. Review Fundamentals
Ensure you have a solid grasp of the core concepts in your area of expertise. For frontend developers, this means mastering HTML, CSS, and JavaScript. For backend developers, focus on your chosen server-side language, database concepts, and API design principles.
2. Practice Coding Challenges
Regularly solve coding problems on platforms like LeetCode, HackerRank, or CodeSignal. Focus on problems relevant to your domain, but don’t neglect general algorithmic challenges, as they’re often part of both frontend and backend interviews.
3. Build Projects
Create personal projects that showcase your skills. For frontend developers, this could be a responsive portfolio website or a complex UI component. Backend developers might build a RESTful API or a small web application demonstrating database interactions.
4. Stay Updated with Industry Trends
Keep abreast of the latest developments in your field. Follow relevant blogs, attend webinars, and experiment with new technologies to demonstrate your passion for continuous learning.
5. Prepare for Behavioral Questions
Be ready to discuss your past experiences, challenges you’ve overcome, and how you work in a team. Use the STAR method (Situation, Task, Action, Result) to structure your responses effectively.
6. Mock Interviews
Practice with friends, mentors, or use online platforms that offer mock technical interviews. This will help you get comfortable with the interview format and improve your ability to communicate technical concepts clearly.
Common Interview Questions
To help you prepare further, here are some common interview questions for both frontend and backend developers:
Frontend Developer Interview Questions
- Explain the box model in CSS and how it affects layout.
- What are the differences between var, let, and const in JavaScript?
- How does React’s virtual DOM work, and what are its benefits?
- Describe the concept of CSS specificity and how it’s calculated.
- What are closures in JavaScript, and how can they be useful?
- Explain the differences between responsive and adaptive design.
- How would you optimize the loading speed of a web page?
- What are Web Components, and how do they work?
- Describe the purpose and usage of the `async` and `defer` attributes in script tags.
- How does CSS Grid differ from Flexbox, and when would you use one over the other?
Backend Developer Interview Questions
- What are the ACID properties in database transactions?
- Explain the differences between SQL and NoSQL databases.
- What is the purpose of an index in a database, and how does it improve query performance?
- Describe the concept of dependency injection and its benefits.
- What are the main HTTP methods used in RESTful APIs?
- Explain the differences between synchronous and asynchronous programming.
- What is the purpose of a message queue in a distributed system?
- How would you handle race conditions in a multi-threaded environment?
- Describe the CAP theorem and its implications for distributed systems.
- What are the key considerations when designing a scalable web application?
Conclusion
While frontend and backend developer interviews share some common elements, they each have distinct focus areas that reflect the specific skills and knowledge required for these roles. Frontend interviews tend to emphasize visual implementation, user interface design, and browser-side performance, while backend interviews focus more on server-side logic, database management, and system architecture.
Regardless of which path you choose, thorough preparation is key to success in technical interviews. By understanding the specific requirements of your chosen domain and honing both your technical and soft skills, you’ll be well-equipped to tackle any interview challenge that comes your way.
Remember that the tech industry is constantly evolving, so staying curious and committed to continuous learning is crucial for long-term success in either frontend or backend development. Good luck with your interviews, and may your coding journey be both challenging and rewarding!