The Critical Role of Data Structures and Algorithms in Coding Interviews: A Comprehensive Guide
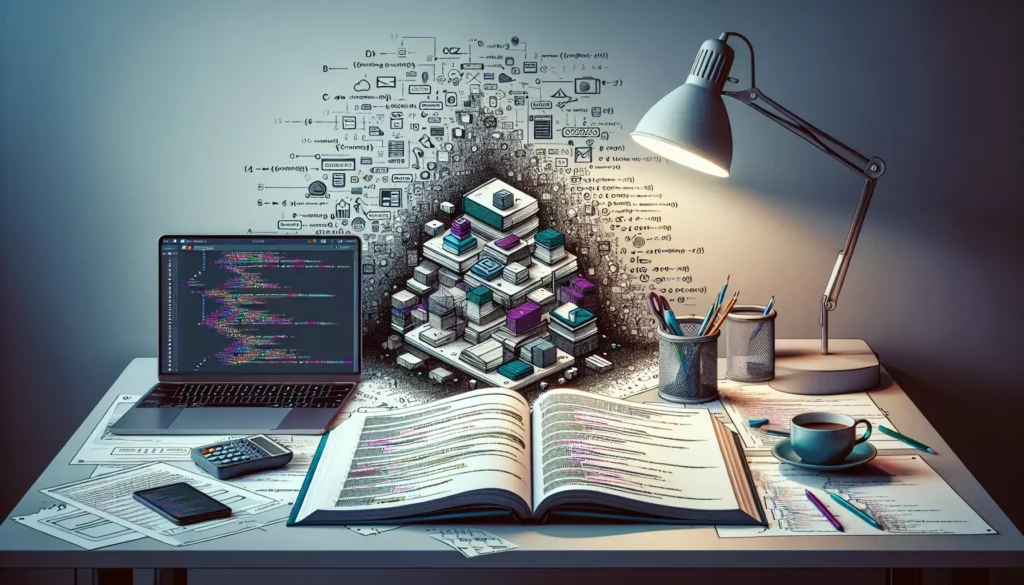
In the competitive world of software engineering, coding interviews have become a crucial gateway to landing coveted positions at top tech companies. Among the various skills assessed during these interviews, proficiency in data structures and algorithms (DSA) stands out as a fundamental requirement. But just how important are these concepts, and why do companies place such a heavy emphasis on them? In this comprehensive guide, we’ll explore the significance of data structures and algorithms in coding interviews, their practical applications, and how you can prepare effectively to ace your next technical interview.
1. The Fundamental Importance of Data Structures and Algorithms
Data structures and algorithms form the backbone of computer science and software engineering. They are essential tools that enable developers to solve complex problems efficiently and write optimized code. Here’s why they are considered crucial:
- Problem-Solving Skills: DSA challenges test a candidate’s ability to break down complex problems into manageable components and devise effective solutions.
- Efficiency: Knowledge of various data structures and algorithms allows developers to choose the most efficient approach for a given problem, leading to better performance and scalability.
- Foundation for Advanced Concepts: Understanding DSA is essential for grasping more advanced programming concepts and techniques.
- Language Agnostic: These concepts are universal and applicable across different programming languages, making them valuable skills for any developer.
2. Why Companies Focus on DSA in Interviews
Tech companies, especially those often referred to as FAANG (Facebook, Amazon, Apple, Netflix, Google), place a significant emphasis on data structures and algorithms during their interview process. Here’s why:
- Scalability Concerns: Large tech companies deal with massive amounts of data and users. Efficient algorithms are crucial for maintaining performance at scale.
- Problem-Solving Abilities: DSA questions test a candidate’s ability to think critically and solve complex problems under pressure.
- Code Quality: Proficiency in DSA often correlates with the ability to write clean, efficient, and maintainable code.
- Adaptability: Strong DSA skills indicate a candidate’s potential to learn and adapt to new technologies and challenges.
- Common Ground: DSA provides a standardized way to assess candidates from diverse backgrounds and experiences.
3. Common Data Structures and Algorithms in Interviews
While the specific topics can vary, certain data structures and algorithms frequently appear in coding interviews. Here’s a list of the most common ones:
Data Structures:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees (Binary Trees, Binary Search Trees, Balanced Trees)
- Graphs
- Hash Tables
- Heaps
Algorithms:
- Sorting (Quick Sort, Merge Sort, Heap Sort)
- Searching (Binary Search, Depth-First Search, Breadth-First Search)
- Dynamic Programming
- Greedy Algorithms
- Recursion and Backtracking
- Graph Algorithms (Shortest Path, Minimum Spanning Tree)
- Bit Manipulation
4. Real-World Applications of DSA
While some argue that DSA questions in interviews are disconnected from day-to-day programming tasks, the reality is that these concepts have numerous practical applications in software development:
- Database Optimization: Efficient data structures and algorithms are crucial for designing and optimizing database systems.
- Web Development: Algorithms play a role in routing, caching strategies, and front-end rendering optimizations.
- Machine Learning: Many machine learning algorithms rely on efficient data structures and algorithmic techniques.
- Operating Systems: Process scheduling, memory management, and file systems all utilize advanced data structures and algorithms.
- Network Protocols: Routing algorithms and data compression techniques are fundamental to network communications.
- Graphics and Gaming: Rendering engines and game logic heavily depend on efficient algorithms for performance.
5. How to Prepare for DSA in Coding Interviews
Mastering data structures and algorithms requires dedication and consistent practice. Here’s a step-by-step guide to help you prepare:
- Build a Strong Foundation:
- Start with basic data structures like arrays, linked lists, and hash tables.
- Learn fundamental algorithms such as binary search and basic sorting algorithms.
- Understand time and space complexity analysis (Big O notation).
- Practice Regularly:
- Solve problems on platforms like LeetCode, HackerRank, or Codeforces.
- Aim to solve at least one problem daily.
- Start with easy problems and gradually move to medium and hard difficulty levels.
- Study Advanced Topics:
- Dive deeper into trees, graphs, and dynamic programming.
- Learn advanced algorithms like Dijkstra’s, A*, and various tree balancing techniques.
- Understand the trade-offs between different data structures and algorithms.
- Implement from Scratch:
- Try implementing common data structures and algorithms from scratch.
- This deepens your understanding and prepares you for detailed questions in interviews.
- Mock Interviews:
- Practice with a friend or use platforms that offer mock interview services.
- Get comfortable explaining your thought process while solving problems.
- Review and Reflect:
- After solving a problem, review other solutions to learn different approaches.
- Reflect on your problem-solving process and identify areas for improvement.
6. Common Misconceptions about DSA in Interviews
Despite their importance, there are some misconceptions about the role of DSA in coding interviews:
- Myth: You need to memorize every algorithm.
Reality: Understanding core principles and problem-solving techniques is more important than memorization. - Myth: DSA skills are only important for interviews.
Reality: These skills are valuable throughout your career for writing efficient and scalable code. - Myth: You’ll never use these concepts in real work.
Reality: While you may not implement complex algorithms daily, the problem-solving skills and efficiency mindset are constantly applied. - Myth: Only FAANG companies focus on DSA.
Reality: Many companies across various industries value these skills in their hiring process.
7. Beyond DSA: Other Important Interview Skills
While data structures and algorithms are crucial, they’re not the only skills assessed in coding interviews. To present a well-rounded profile, also focus on:
- System Design: Understanding how to design scalable and efficient systems is crucial, especially for senior roles.
- Object-Oriented Programming: Solid grasp of OOP principles and design patterns.
- Coding Best Practices: Writing clean, readable, and maintainable code.
- Database Knowledge: Understanding of SQL, database design, and optimization techniques.
- Web Technologies: Familiarity with modern web development frameworks and practices.
- Soft Skills: Communication, teamwork, and problem-solving in a collaborative environment.
8. The Role of AI in Coding Education and Interview Preparation
As the field of software engineering evolves, so do the tools and resources available for learning and preparation. Artificial Intelligence is playing an increasingly significant role in coding education and interview preparation:
- AI-Powered Coding Assistants: Tools like GitHub Copilot offer real-time code suggestions, helping developers learn best practices and efficient implementations.
- Personalized Learning Paths: AI algorithms can analyze a learner’s progress and tailor content to address specific weaknesses in DSA knowledge.
- Automated Code Review: AI systems can provide instant feedback on code quality, efficiency, and potential improvements.
- Interview Simulation: Advanced AI can simulate realistic interview scenarios, providing a safe environment for practice.
Platforms like AlgoCademy leverage these AI capabilities to provide a more effective and personalized learning experience for aspiring software engineers.
9. The Future of DSA in Coding Interviews
As technology continues to advance, some wonder about the future relevance of DSA in coding interviews. While the specifics may evolve, the fundamental skills tested by DSA questions are likely to remain valuable:
- Evolving Focus: Interviews may shift towards more practical, real-world problem-solving scenarios that still require strong DSA foundations.
- Emphasis on Efficiency: As systems grow more complex, the ability to write efficient code becomes even more critical.
- Adaptation to New Technologies: DSA concepts may be applied to emerging fields like quantum computing or advanced AI algorithms.
- Balanced Assessment: Companies may adopt a more holistic approach, combining DSA with other practical skills assessments.
10. Conclusion: The Enduring Importance of DSA in Coding Interviews
In conclusion, data structures and algorithms play a crucial role in coding interviews, serving as a fundamental measure of a candidate’s problem-solving skills, efficiency mindset, and technical proficiency. While mastering these concepts requires significant effort, the benefits extend far beyond just passing interviews. A strong foundation in DSA equips you with the tools to tackle complex problems, write efficient code, and adapt to new challenges throughout your career in software engineering.
As you prepare for coding interviews, remember that consistent practice, a methodical approach to learning, and leveraging modern resources like AI-powered platforms can significantly enhance your skills. While DSA is a critical component, also focus on developing a well-rounded skill set that includes system design, practical coding experience, and soft skills.
The journey to mastering data structures and algorithms may be challenging, but it’s an investment that pays dividends throughout your career in technology. Whether you’re aiming for a position at a top tech company or looking to excel in any software development role, a strong grasp of DSA will serve as a solid foundation for your success.
Remember, the goal isn’t just to pass interviews but to become a proficient problem solver and efficient coder. Embrace the learning process, stay curious, and continually challenge yourself. With dedication and the right resources, you can develop the skills necessary to excel in coding interviews and thrive in your software engineering career.