The Comprehensive Guide to Setting Variables in C++
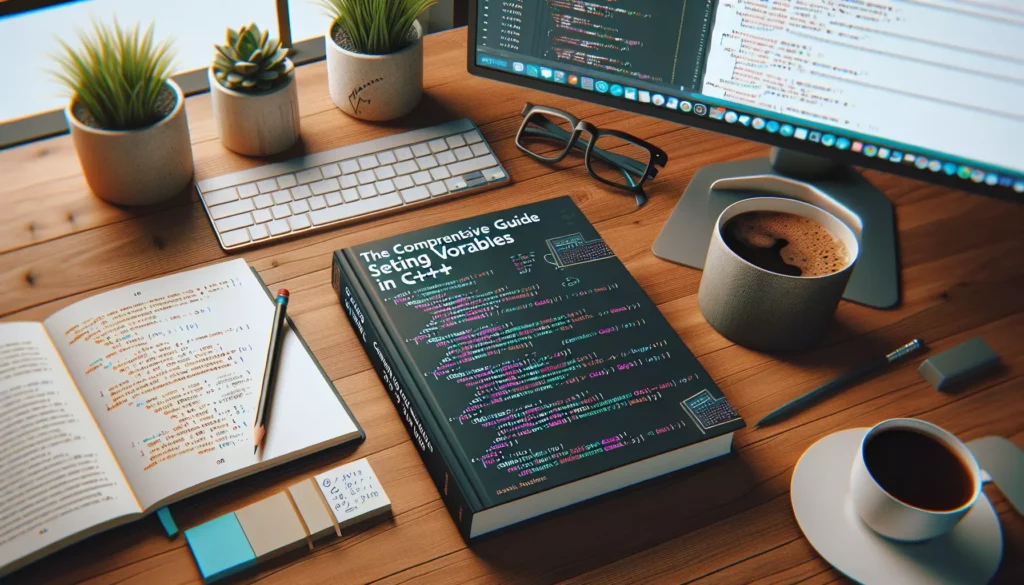
C++ is a powerful and versatile programming language that has been widely used for decades. One of the fundamental concepts in C++ is setting variables, which allows programmers to store and manipulate data within their programs. In this comprehensive guide, we’ll explore everything you need to know about setting variables in C++, from basic concepts to advanced techniques.
Table of Contents
- Introduction to Variables in C++
- Basic Variable Types
- Declaring and Initializing Variables
- Variable Scope and Lifetime
- Constants and Immutable Variables
- Type Inference with auto
- Reference Variables
- Pointers
- Arrays and Vectors
- Structures and Classes
- Enumerations
- Type Aliases
- Best Practices for Variable Naming and Usage
- Common Pitfalls and How to Avoid Them
- Advanced Topics in Variable Management
1. Introduction to Variables in C++
Variables are fundamental building blocks in C++ programming. They serve as containers for storing data that can be manipulated and accessed throughout a program’s execution. Understanding how to properly set and use variables is crucial for writing efficient and effective C++ code.
In C++, variables have several important characteristics:
- Type: Defines the kind of data the variable can hold (e.g., integers, floating-point numbers, characters)
- Name: A unique identifier used to reference the variable in the code
- Value: The actual data stored in the variable
- Memory location: The address in computer memory where the variable’s value is stored
2. Basic Variable Types
C++ provides several built-in data types for variables. Here are some of the most commonly used ones:
- int: Integer values (e.g., -1, 0, 42)
- float: Single-precision floating-point numbers (e.g., 3.14f)
- double: Double-precision floating-point numbers (e.g., 3.14159265359)
- char: Single characters (e.g., ‘A’, ‘7’, ‘$’)
- bool: Boolean values (true or false)
- string: Sequences of characters (e.g., “Hello, World!”)
Each of these types has a specific size and range of values it can represent. For example:
int myInteger = 42;
float myFloat = 3.14f;
double myDouble = 3.14159265359;
char myChar = 'A';
bool myBoolean = true;
std::string myString = "Hello, World!";
3. Declaring and Initializing Variables
In C++, you must declare a variable before using it. Declaration involves specifying the variable’s type and name. You can also initialize the variable with a value at the time of declaration.
Here are some examples of variable declaration and initialization:
// Declaration only
int age;
// Declaration with initialization
int score = 100;
// Multiple declarations
int x, y, z;
// Multiple declarations with initialization
int a = 1, b = 2, c = 3;
// Declaration and initialization using uniform initialization syntax (C++11 and later)
int value{42};
float pi{3.14159f};
It’s generally a good practice to initialize variables when you declare them to avoid using uninitialized variables, which can lead to undefined behavior.
4. Variable Scope and Lifetime
The scope of a variable determines where in the code the variable can be accessed. C++ has several levels of scope:
- Global scope: Variables declared outside of any function or class
- Namespace scope: Variables declared within a namespace
- Local scope: Variables declared within a function or a block
- Class scope: Variables declared as members of a class
Here’s an example illustrating different scopes:
int globalVar = 10; // Global scope
namespace MyNamespace {
int namespaceVar = 20; // Namespace scope
}
void myFunction() {
int localVar = 30; // Local scope
{
int blockVar = 40; // Block scope
}
// blockVar is not accessible here
}
class MyClass {
int classVar; // Class scope
public:
MyClass() : classVar(50) {}
};
The lifetime of a variable is the duration for which it exists in memory. Global and static variables have a lifetime that spans the entire program execution, while local variables exist only within their scope.
5. Constants and Immutable Variables
C++ provides ways to create variables whose values cannot be changed after initialization. These are useful for representing fixed values or preventing accidental modifications.
The const
keyword is used to declare constants:
const int MAX_SCORE = 100;
const double PI = 3.14159265359;
// Attempting to modify a const variable will result in a compilation error
// MAX_SCORE = 200; // Error!
In C++11 and later, you can use constexpr
for compile-time constants:
constexpr int DAYS_IN_WEEK = 7;
constexpr double SPEED_OF_LIGHT = 299792458.0; // meters per second
6. Type Inference with auto
C++11 introduced the auto
keyword, which allows the compiler to automatically deduce the type of a variable based on its initializer. This can make code more concise and easier to maintain, especially when dealing with complex types:
auto i = 42; // int
auto f = 3.14f; // float
auto d = 3.14; // double
auto b = true; // bool
auto c = 'A'; // char
auto s = "Hello"; // const char*
auto str = std::string("Hello"); // std::string
While auto
can be convenient, it’s important to use it judiciously and ensure that the inferred type is what you expect.
7. Reference Variables
References in C++ provide an alternative name for an existing variable. They are declared using the &
symbol and must be initialized when declared:
int x = 10;
int& ref = x; // ref is a reference to x
ref = 20; // This changes the value of x
std::cout << x; // Outputs 20
References are often used in function parameters to avoid copying large objects and to allow functions to modify the original variables:
void incrementValue(int& value) {
value++;
}
int main() {
int num = 5;
incrementValue(num);
std::cout << num; // Outputs 6
return 0;
}
8. Pointers
Pointers are variables that store memory addresses. They are powerful but can be a source of errors if not used carefully. Pointers are declared using the *
symbol:
int x = 10;
int* ptr = &x; // ptr holds the address of x
std::cout << *ptr; // Outputs 10 (dereferencing)
*ptr = 20; // Changes the value of x to 20
Pointers can also be used with dynamic memory allocation:
int* dynamicInt = new int(42);
// Use dynamicInt...
delete dynamicInt; // Don't forget to free the memory!
9. Arrays and Vectors
Arrays and vectors are used to store collections of elements of the same type.
C-style arrays have a fixed size determined at compile-time:
int numbers[5] = {1, 2, 3, 4, 5};
char name[] = "John"; // Null-terminated string
Vectors, from the C++ Standard Library, offer more flexibility and safety:
#include <vector>
std::vector<int> numbers = {1, 2, 3, 4, 5};
numbers.push_back(6); // Add an element
std::cout << numbers.size(); // Outputs 6
10. Structures and Classes
Structures and classes allow you to create custom data types that group related variables:
struct Point {
int x;
int y;
};
Point p1 = {10, 20};
class Person {
private:
std::string name;
int age;
public:
Person(const std::string& n, int a) : name(n), age(a) {}
void introduce() {
std::cout << "I'm " << name << " and I'm " << age << " years old.";
}
};
Person john("John", 30);
john.introduce();
11. Enumerations
Enumerations allow you to define a set of named constants:
enum Color { RED, GREEN, BLUE };
Color myColor = GREEN;
// C++11 introduced strongly-typed enums
enum class Day : char { MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY };
Day today = Day::WEDNESDAY;
12. Type Aliases
Type aliases allow you to create alternative names for existing types:
typedef unsigned long long ULL; // Old style
using LongString = std::string; // New style (C++11 and later)
ULL bigNumber = 18446744073709551615ULL;
LongString message = "This is a long string.";
13. Best Practices for Variable Naming and Usage
- Use descriptive and meaningful names for variables
- Follow a consistent naming convention (e.g., camelCase or snake_case)
- Initialize variables when declaring them
- Use const for variables that shouldn’t be modified
- Limit the scope of variables as much as possible
- Avoid global variables when possible
- Use auto judiciously, especially when the type is not obvious
14. Common Pitfalls and How to Avoid Them
- Uninitialized variables: Always initialize variables before using them
- Integer overflow: Be aware of the limits of integer types
- Dangling pointers: Avoid using pointers to objects that have been deleted
- Memory leaks: Always free dynamically allocated memory
- Type mismatches: Be careful when mixing different types in expressions
- Shadowing: Avoid declaring variables with the same name in nested scopes
15. Advanced Topics in Variable Management
Move Semantics and Rvalue References
C++11 introduced move semantics, which can improve performance by allowing resources to be transferred between objects instead of copied:
#include <utility>
class MyClass {
// ...
public:
MyClass(MyClass&& other) noexcept { // Move constructor
// Transfer resources from other to this
}
MyClass& operator=(MyClass&& other) noexcept { // Move assignment operator
if (this != &other) {
// Transfer resources from other to this
}
return *this;
}
};
MyClass createObject() {
MyClass obj;
// ... initialize obj
return obj;
}
MyClass myObj = createObject(); // Move construction
Smart Pointers
Smart pointers provide automatic memory management, helping to prevent memory leaks and other pointer-related issues:
#include <memory>
std::unique_ptr<int> uniquePtr = std::make_unique<int>(42);
std::shared_ptr<int> sharedPtr = std::make_shared<int>(100);
// No need to manually delete; memory is automatically managed
Thread-Local Storage
For multi-threaded programs, you can use thread-local storage to create variables that are unique to each thread:
#include <thread>
thread_local int threadId = 0;
void threadFunction() {
threadId = std::this_thread::get_id().hash();
std::cout << "Thread ID: " << threadId << std::endl;
}
int main() {
std::thread t1(threadFunction);
std::thread t2(threadFunction);
t1.join();
t2.join();
return 0;
}
Variadic Templates
Variadic templates allow you to write functions and classes that can work with any number of arguments:
template<typename... Args>
void printAll(Args... args) {
(std::cout << ... << args) << std::endl;
}
printAll(1, 2.0, "three", '4');
Concepts (C++20)
Concepts provide a way to specify constraints on template parameters, making template code more readable and easier to debug:
#include <concepts>
template<typename T>
concept Numeric = std::integral<T> || std::floating_point<T>;
template<Numeric T>
T add(T a, T b) {
return a + b;
}
// Usage
auto result1 = add(5, 3); // OK
auto result2 = add(3.14, 2.5); // OK
// auto result3 = add("a", "b"); // Error: doesn't satisfy Numeric concept
In conclusion, setting variables in C++ is a fundamental skill that underpins all C++ programming. From basic types to advanced concepts like move semantics and smart pointers, understanding how to declare, initialize, and manage variables is crucial for writing efficient, safe, and maintainable C++ code. By following best practices and being aware of common pitfalls, you can leverage the full power of C++’s variable system in your programs.