The Coder’s Pilgrimage: Visiting the Birthplaces of Famous Algorithms
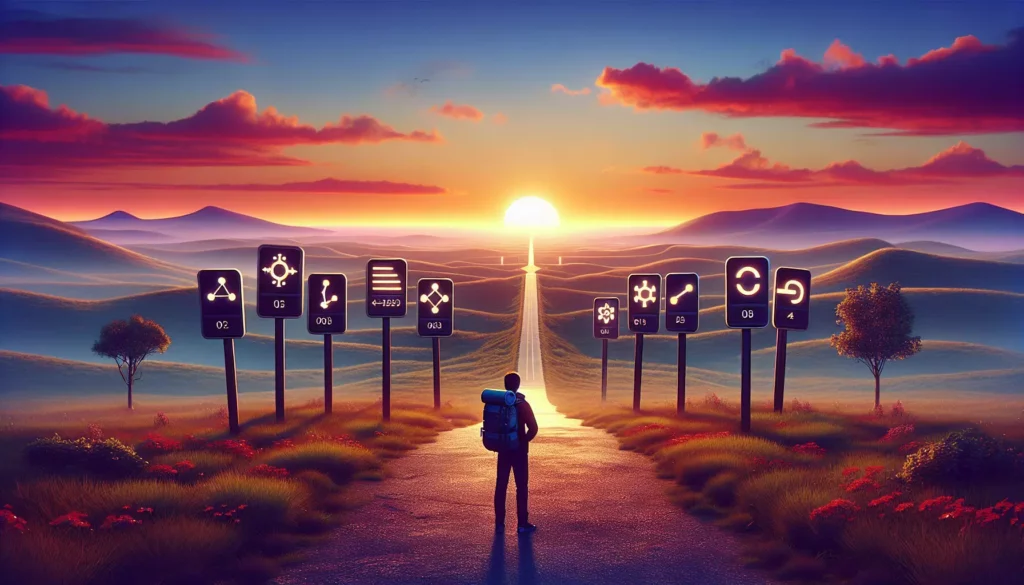
In the world of computer science and programming, algorithms are the building blocks that power our digital world. These ingenious solutions to complex problems have revolutionized technology and continue to shape our future. For coding enthusiasts and computer science aficionados, there’s a special thrill in tracing the origins of these groundbreaking algorithms. Join us on a virtual journey as we explore the birthplaces of some of the most famous algorithms in history.
1. The Dijkstra Algorithm – Eindhoven, Netherlands
Our first stop takes us to the charming city of Eindhoven in the Netherlands. This is where Edsger W. Dijkstra, one of the most influential computer scientists of all time, conceived his eponymous algorithm in 1956.
The Dijkstra algorithm is a graph search algorithm that solves the single-source shortest path problem for a graph with non-negative edge weights. It’s widely used in routing and as a subroutine in other graph algorithms.
Dijkstra came up with this algorithm while pondering how to demonstrate the capabilities of the ARMAC computer to the general public. He decided to compute the shortest route between two cities in the Netherlands, and in just about 20 minutes, he had designed the algorithm that would later bear his name.
Today, you can visit the Eindhoven University of Technology, where Dijkstra worked as a professor. The university has a computer science department that continues to honor Dijkstra’s legacy.
function dijkstra(graph, start, end):
distances = {node: float('inf') for node in graph}
distances[start] = 0
pq = [(0, start)]
while pq:
current_distance, current_node = heapq.heappop(pq)
if current_node == end:
return current_distance
if current_distance > distances[current_node]:
continue
for neighbor, weight in graph[current_node].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(pq, (distance, neighbor))
return float('inf')
2. The PageRank Algorithm – Stanford, California, USA
Our next destination is sunny Stanford, California, where Larry Page and Sergey Brin developed the PageRank algorithm in the late 1990s. This algorithm would become the foundation of Google’s search engine and revolutionize how we navigate the internet.
PageRank works by counting the number and quality of links to a page to determine a rough estimate of how important the website is. The underlying assumption is that more important websites are likely to receive more links from other websites.
You can visit Stanford University and take a stroll through the computer science department where Page and Brin were Ph.D. students when they developed this groundbreaking algorithm. The university offers guided tours that often include stops at significant locations in the history of Silicon Valley innovations.
def pagerank(graph, damping_factor=0.85, epsilon=1e-8, max_iterations=100):
num_pages = len(graph)
ranks = {page: 1 / num_pages for page in graph}
for _ in range(max_iterations):
new_ranks = {}
diff = 0
for page in graph:
rank = (1 - damping_factor) / num_pages
for linking_page in graph:
if page in graph[linking_page]:
rank += damping_factor * ranks[linking_page] / len(graph[linking_page])
new_ranks[page] = rank
diff += abs(new_ranks[page] - ranks[page])
ranks = new_ranks
if diff < epsilon:
break
return ranks
3. The Fast Fourier Transform – Princeton, New Jersey, USA
Our journey now takes us to Princeton, New Jersey, where James Cooley and John Tukey developed the Fast Fourier Transform (FFT) algorithm in 1965. The FFT is a highly efficient method for computing the Discrete Fourier Transform (DFT) of a sequence, or its inverse.
The FFT has numerous applications in digital signal processing, solving partial differential equations, and multiplying large integers. It’s a cornerstone algorithm in many fields, including audio and image processing, telecommunications, and scientific computing.
While working at Princeton University, Cooley and Tukey refined and published their algorithm, which dramatically reduced the complexity of computing Fourier transforms from O(n^2) to O(n log n). This improvement made many previously impractical computations feasible.
Today, you can visit Princeton University and its Department of Computer Science, which continues to be at the forefront of algorithmic research and development.
def fft(x):
n = len(x)
if n <= 1:
return x
even = fft(x[0::2])
odd = fft(x[1::2])
twiddle_factors = [cmath.exp(-2j * cmath.pi * k / n) for k in range(n // 2)]
return [even[k] + twiddle_factors[k] * odd[k] for k in range(n // 2)] + \
[even[k] - twiddle_factors[k] * odd[k] for k in range(n // 2)]
4. The RSA Algorithm – Cambridge, Massachusetts, USA
Our next stop is at the Massachusetts Institute of Technology (MIT) in Cambridge, where Ron Rivest, Adi Shamir, and Leonard Adleman invented the RSA algorithm in 1977. RSA (Rivest-Shamir-Adleman) is one of the first public-key cryptosystems and is widely used for secure data transmission.
The RSA algorithm is based on the practical difficulty of factoring the product of two large prime numbers. Its security relies on the fact that while it’s easy to multiply two prime numbers, it’s extremely challenging to determine the original primes if you only know their product.
MIT’s campus is steeped in the history of computer science and cryptography. Visitors can explore the Stata Center, which houses the Computer Science and Artificial Intelligence Laboratory (CSAIL) where groundbreaking research continues to this day.
def generate_keypair(p, q):
n = p * q
phi = (p-1) * (q-1)
e = random.randrange(1, phi)
g = math.gcd(e, phi)
while g != 1:
e = random.randrange(1, phi)
g = math.gcd(e, phi)
d = pow(e, -1, phi)
return ((e, n), (d, n))
def encrypt(pk, plaintext):
key, n = pk
cipher = [pow(ord(char), key, n) for char in plaintext]
return cipher
def decrypt(pk, ciphertext):
key, n = pk
plain = [chr(pow(char, key, n)) for char in ciphertext]
return ''.join(plain)
5. The Quicksort Algorithm – London, United Kingdom
Our pilgrimage now takes us across the Atlantic to London, where computer scientist Tony Hoare invented the Quicksort algorithm in 1959 while studying at Moscow State University. Quicksort is an efficient, in-place sorting algorithm that is commonly used as a benchmark for other sorting algorithms.
Hoare developed Quicksort as part of his work on machine translation for the National Physical Laboratory in the UK. The algorithm uses a divide-and-conquer strategy to sort elements, making it one of the fastest sorting algorithms in practice.
While you can’t visit the exact location where Quicksort was conceived (as Hoare was in Moscow at the time), you can visit the Department of Computer Science at University College London, where Hoare later worked as a Professor of Computer Science.
def quicksort(arr):
if len(arr) <= 1:
return arr
else:
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quicksort(left) + middle + quicksort(right)
6. The MapReduce Algorithm – Mountain View, California, USA
Our journey brings us back to California, this time to Mountain View, where Google engineers Jeffrey Dean and Sanjay Ghemawat developed the MapReduce algorithm in 2004. MapReduce is a programming model and an associated implementation for processing and generating big data sets with a parallel, distributed algorithm on a cluster.
MapReduce was designed to allow for distributed processing of massive datasets across a cluster of computers. It has become a foundational technique in big data processing and has influenced many modern big data frameworks.
While you can’t tour Google’s offices where MapReduce was developed, you can visit the Computer History Museum in Mountain View, which offers exhibits on the history of computing, including the evolution of big data processing techniques.
def map_function(data):
# This is a simple example that counts word occurrences
return [(word, 1) for word in data.split()]
def reduce_function(key, values):
# Sum up all the counts for each word
return key, sum(values)
def mapreduce(data, map_func, reduce_func):
# Map phase
mapped_data = []
for item in data:
mapped_data.extend(map_func(item))
# Shuffle phase
shuffled_data = {}
for key, value in mapped_data:
if key not in shuffled_data:
shuffled_data[key] = []
shuffled_data[key].append(value)
# Reduce phase
reduced_data = []
for key, values in shuffled_data.items():
reduced_data.append(reduce_func(key, values))
return reduced_data
7. The A* Search Algorithm – Palo Alto, California, USA
Our final stop on this algorithmic pilgrimage takes us to Palo Alto, California, where Peter Hart, Nils Nilsson, and Bertram Raphael developed the A* (A-star) search algorithm in 1968 at the Stanford Research Institute (now SRI International).
A* is a graph traversal and path search algorithm that is often used in many fields of computer science due to its completeness, optimality, and optimal efficiency. It’s particularly popular in artificial intelligence for pathfinding and graph traversal.
The algorithm was an extension of Edsger Dijkstra’s 1959 algorithm and achieves better performance by using heuristics to guide its search. Today, SRI International continues to be at the forefront of AI and robotics research.
While SRI International is not open to the public, you can visit the nearby Computer History Museum in Mountain View to learn more about the history of AI and pathfinding algorithms.
import heapq
def heuristic(a, b):
# Manhattan distance on a square grid
return abs(b[0] - a[0]) + abs(b[1] - a[1])
def a_star(start, goal, graph):
frontier = []
heapq.heappush(frontier, (0, start))
came_from = {}
cost_so_far = {}
came_from[start] = None
cost_so_far[start] = 0
while frontier:
current = heapq.heappop(frontier)[1]
if current == goal:
break
for next in graph[current]:
new_cost = cost_so_far[current] + graph[current][next]
if next not in cost_so_far or new_cost < cost_so_far[next]:
cost_so_far[next] = new_cost
priority = new_cost + heuristic(goal, next)
heapq.heappush(frontier, (priority, next))
came_from[next] = current
return came_from, cost_so_far
Conclusion: The Legacy of Algorithmic Innovation
Our journey through the birthplaces of these famous algorithms reveals more than just geographical locations. It’s a testament to the brilliant minds that have shaped the field of computer science and continue to influence how we solve problems in the digital age.
From Dijkstra’s graph search algorithm in Eindhoven to the A* search algorithm in Palo Alto, each of these locations holds a special place in the history of computer science. They serve as reminders of the power of human ingenuity and the far-reaching impact of algorithmic thinking.
For aspiring programmers and computer scientists, understanding these algorithms and their origins can provide invaluable insights into problem-solving and algorithm design. Each of these algorithms emerged from a specific need or challenge, demonstrating how innovative thinking can lead to solutions that stand the test of time.
As we continue to face new challenges in our increasingly digital world, we can draw inspiration from these algorithmic pioneers. Their work reminds us that with creativity, persistence, and a deep understanding of computational principles, we can develop solutions that not only solve immediate problems but also lay the groundwork for future innovations.
Whether you’re a seasoned developer or just starting your coding journey, taking the time to understand these fundamental algorithms can significantly enhance your problem-solving skills. Platforms like AlgoCademy offer interactive tutorials and resources to help you master these algorithms and prepare for technical interviews at major tech companies.
Remember, every great algorithm starts with a problem and a creative approach to solving it. As you continue your coding education and skills development, challenge yourself to think algorithmically and who knows? The next groundbreaking algorithm might just come from you.
So, while our virtual pilgrimage ends here, your journey in the world of algorithms is just beginning. Keep exploring, keep learning, and keep pushing the boundaries of what’s possible with code. The next chapter in the history of algorithms is waiting to be written, and you could be the one to write it.