The Coder’s Phonebook: Memorizing Important Methods by Assigning Them Phone Numbers
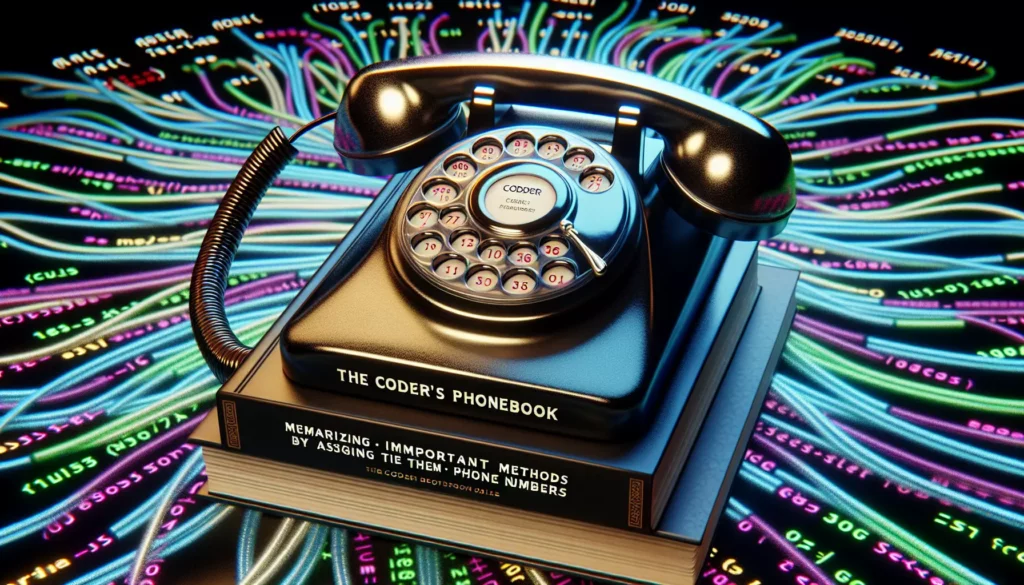
As programmers, we’re constantly juggling a vast array of methods, functions, and algorithms in our minds. Whether you’re a beginner just starting your coding journey or a seasoned developer preparing for technical interviews at top tech companies, having quick recall of important coding concepts can be a game-changer. Today, we’re going to explore a unique and effective memorization technique: assigning phone numbers to important coding methods. This approach, which we’ll call “The Coder’s Phonebook,” can help you internalize crucial programming knowledge and ace your next coding challenge or interview.
Why Use Phone Numbers for Memorization?
Before we dive into the specifics of this technique, let’s consider why phone numbers make an excellent memorization tool:
- Familiarity: Most of us are already accustomed to remembering phone numbers, making it a natural extension of our existing memory skills.
- Structure: Phone numbers have a consistent format (e.g., XXX-XXX-XXXX in the US), providing a structured framework for organizing information.
- Mnemonics: Phone numbers can easily be converted into memorable words or phrases using the letters associated with each number on a phone keypad.
- Chunking: The grouping of digits in phone numbers aligns with the psychological principle of chunking, which helps our brains process and retain information more effectively.
How to Create Your Coder’s Phonebook
Here’s a step-by-step guide to building your personalized Coder’s Phonebook:
1. Identify Key Methods and Concepts
Start by listing the most important methods, algorithms, or concepts you want to remember. Focus on those that are frequently used in coding interviews or are fundamental to your programming language of choice.
2. Assign Phone Numbers
For each method or concept, create a unique phone number. You can use various strategies to make these numbers meaningful:
- Use the method’s time or space complexity as part of the number (e.g., O(n) could be represented by 6(66) for Big O notation)
- Incorporate the first letters of the method name using the phone keypad
- Include numbers that represent key aspects of the algorithm (e.g., 2 for binary operations)
3. Create Mnemonics
Develop memorable phrases or words from the phone numbers using the letters on a phone keypad. This adds an extra layer of memorability to your Coder’s Phonebook entries.
4. Organize Your Phonebook
Group related methods or concepts together, just as you might organize contacts in a real phone book. This can help you see connections between different programming concepts.
Example Entries for Your Coder’s Phonebook
Let’s look at some example entries to illustrate how this system might work:
1. Binary Search
Phone Number: 246-569-2474 (BIN-LOG-BISE)
Mnemonic: “BINary LOGarithm BInary SEarch”
Explanation: The “246” represents the binary nature of the algorithm, “569” (LOG) reminds us of the logarithmic time complexity, and “2474” spells out “BISE” for BInary SEarch.
2. Quicksort
Phone Number: 784-256-7678 (QUI-CLO-SORT)
Mnemonic: “QUIck CLOse SORT”
Explanation: “784” spells “QUI” for Quick, “256” represents the average-case time complexity of O(n log n), and “7678” spells “SORT”.
3. Depth-First Search (DFS)
Phone Number: 337-386-3377 (DFS-FUL-DFSR)
Mnemonic: “DFS FULly Deep FiRst Search”
Explanation: “337” spells “DFS”, “386” (FUL) reminds us it fully explores branches before backtracking, and “3377” reinforces DFS and adds “R” for recursion, a common implementation approach.
Practical Application in Coding Interviews
Now that we’ve established the concept of the Coder’s Phonebook, let’s explore how this memorization technique can be applied in real-world coding interview scenarios. We’ll walk through a common interview question and demonstrate how having quick access to your mental “phonebook” can help you solve problems more efficiently.
Interview Question: Find the First Non-Repeating Character
Imagine you’re in a technical interview, and you’re presented with the following question:
Given a string, find the first non-repeating character in it and return its index. If it doesn’t exist, return -1.
This is a classic problem that tests your understanding of string manipulation and data structures. Let’s see how our Coder’s Phonebook can help us approach this problem step-by-step.
Step 1: Recognize the Problem Type
As soon as you hear this question, you might recall your Coder’s Phonebook entry for hash tables:
Hash Table
Phone Number: 427-428-2253 (HAS-HAT-ABLE)
Mnemonic: “HASh HAshTable ABLE”
This entry reminds you that hash tables are excellent for solving problems involving character frequency or uniqueness in strings.
Step 2: Outline the Solution
With hash tables in mind, you can quickly outline a two-pass solution:
- First pass: Count the frequency of each character using a hash table.
- Second pass: Find the first character with a frequency of 1.
Step 3: Implement the Solution
Now, let’s write the code for this solution. As you implement, you might recall another Coder’s Phonebook entry:
String Iteration
Phone Number: 787-484-8377 (STR-ITI-TESR)
Mnemonic: “STRing ITeration ITERator”
This reminds you of efficient ways to iterate through strings in your chosen programming language. Here’s a Python implementation of the solution:
def first_non_repeating_char(s):
char_count = {}
# First pass: Count character frequencies
for char in s:
char_count[char] = char_count.get(char, 0) + 1
# Second pass: Find first character with frequency 1
for i, char in enumerate(s):
if char_count[char] == 1:
return i
return -1
# Test the function
print(first_non_repeating_char("leetcode")) # Output: 0
print(first_non_repeating_char("loveleetcode")) # Output: 2
print(first_non_repeating_char("aabb")) # Output: -1
Step 4: Analyze Time and Space Complexity
After implementing the solution, you should be prepared to discuss its time and space complexity. Your Coder’s Phonebook might include an entry like this:
Time Complexity Analysis
Phone Number: 843-668-2669 (TIME-NOT-ANOY)
Mnemonic: “TIME NOTation ANalYsis”
This reminds you to consider both time and space complexity in your analysis:
- Time Complexity: O(n), where n is the length of the string. We make two passes through the string.
- Space Complexity: O(k), where k is the size of the character set. In the worst case, this could be O(n) if all characters are unique.
Expanding Your Coder’s Phonebook
As you progress in your coding journey, you’ll want to continually expand and refine your Coder’s Phonebook. Here are some strategies to help you grow your mental repository of programming knowledge:
1. Add Language-Specific Methods
Include important built-in methods and functions for the programming languages you use most frequently. For example, if you’re a Python developer, you might add entries like:
List Comprehension
Phone Number: 547-826-6773 (LIS-COM-PREH)
Mnemonic: “LISt COMPREHension”
Dictionary Get Method
Phone Number: 343-438-4386 (DIC-GET-GURU)
Mnemonic: “DICt GET GURU”
2. Incorporate Data Structure Operations
For each major data structure, create entries that help you remember their key operations and time complexities. For instance:
Binary Heap Insert
Phone Number: 243-564-4678 (BHE-LOG-INST)
Mnemonic: “Binary HEap LOGarithmic INSerT”
3. Add Algorithm Design Paradigms
Include entries for important algorithm design techniques, such as:
Dynamic Programming
Phone Number: 396-726-3962 (DYN-PRO-DYMA)
Mnemonic: “DYNamic PROgramming DYnaMic Approach”
4. Remember System Design Concepts
For more advanced interviews, add entries related to system design principles:
Load Balancing
Phone Number: 562-322-5262 (LOA-BAL-LANC)
Mnemonic: “LOAd BALancing BALaNCe”
Practicing with Your Coder’s Phonebook
Creating your Coder’s Phonebook is just the first step. To truly internalize this knowledge and make it useful during high-pressure situations like coding interviews, you need to practice regularly. Here are some effective ways to reinforce your Coder’s Phonebook entries:
1. Daily Review
Set aside a few minutes each day to review a subset of your Coder’s Phonebook entries. You could use a spaced repetition system to ensure you’re reviewing entries at optimal intervals for long-term retention.
2. Coding Challenges
As you work through coding challenges on platforms like AlgoCademy, LeetCode, or HackerRank, consciously try to recall relevant Coder’s Phonebook entries before you start coding. This will help you build the mental habit of accessing your phonebook when faced with real problems.
3. Teach Others
Explaining concepts to others is an excellent way to solidify your own understanding. Try teaching a coding concept to a friend or writing a blog post, using your Coder’s Phonebook entries as a guide.
4. Mock Interviews
Practice mock interviews with friends or use online platforms that offer this service. During these interviews, challenge yourself to quickly recall and apply relevant information from your Coder’s Phonebook.
5. Visualization Exercises
Regularly visualize yourself in a coding interview, mentally “dialing” the numbers in your Coder’s Phonebook to access the information you need. This mental rehearsal can help reduce anxiety and improve recall during actual interviews.
Adapting the Coder’s Phonebook for Different Learning Styles
While the phone number system can be highly effective, it’s important to recognize that everyone has different learning preferences. Here are some ways to adapt the Coder’s Phonebook concept for various learning styles:
Visual Learners
If you’re a visual learner, you might enhance your Coder’s Phonebook by:
- Creating a mind map that visually represents your “phonebook,” with branches for different categories of programming concepts.
- Designing custom icons or images to represent each entry, incorporating elements of the phone number and mnemonic.
- Using color coding to group related concepts or to highlight different parts of each entry (e.g., one color for the method name, another for the time complexity).
Auditory Learners
For those who learn best through hearing, consider these adaptations:
- Record yourself reading out each Coder’s Phonebook entry, including the method name, phone number, and mnemonic.
- Create short jingles or rhymes for each entry to make them more memorable.
- Use a text-to-speech tool to generate audio versions of your entries that you can listen to while commuting or exercising.
Kinesthetic Learners
If you’re a hands-on learner, try these approaches:
- Create physical flashcards for each entry, with the method name on one side and the phone number and mnemonic on the other.
- Use a real phone or phone app to “dial” the numbers as you review your entries, engaging your muscle memory.
- Act out or use hand gestures to represent different algorithms or data structures as you review their associated phone numbers.
Leveraging AI for Your Coder’s Phonebook
As AI continues to advance, there are exciting possibilities for incorporating it into your Coder’s Phonebook practice. Here are some ways you might use AI to enhance your learning:
1. AI-Generated Mnemonics
Use AI language models to generate creative and memorable mnemonics for your phone number entries. This can help you come up with unique and effective memory aids more quickly.
2. Personalized Review Schedules
Implement an AI system that tracks your review history and performance, then generates personalized schedules for reviewing your Coder’s Phonebook entries. This could optimize your learning by ensuring you review concepts at the most effective intervals for long-term retention.
3. Interactive Quizzing
Create an AI-powered chatbot that quizzes you on your Coder’s Phonebook entries, adapting its questions based on your performance and focusing on areas where you need more practice.
4. Code Analysis and Entry Suggestions
Develop an AI tool that analyzes your coding projects and suggests new entries for your Coder’s Phonebook based on the methods and concepts you frequently use or struggle with.
Conclusion: Your Personal Roadmap to Coding Mastery
The Coder’s Phonebook is more than just a memorization technique – it’s a personalized roadmap to coding mastery. By assigning phone numbers to important programming concepts, you’re creating a unique and powerful mental framework that can serve you throughout your coding career.
As you build and refine your Coder’s Phonebook, remember that the true value lies not just in memorization, but in the deep understanding that comes from actively engaging with these concepts. Each “phone number” should serve as a gateway to a wealth of knowledge about the associated method or algorithm.
Whether you’re preparing for technical interviews at top tech companies, working on complex coding projects, or simply striving to become a better programmer, your Coder’s Phonebook can be an invaluable tool. It provides quick access to critical information, helps you make connections between different programming concepts, and builds your confidence in tackling a wide range of coding challenges.
So, start building your Coder’s Phonebook today. Assign those phone numbers, create memorable mnemonics, and practice regularly. With time and dedication, you’ll develop a mental repository of programming knowledge that’s always at your fingertips, ready to be “dialed up” whenever you need it.
Happy coding, and may your Coder’s Phonebook lead you to success in all your programming endeavors!