The Coder’s Palate: Developing a Taste for Clean and Efficient Code
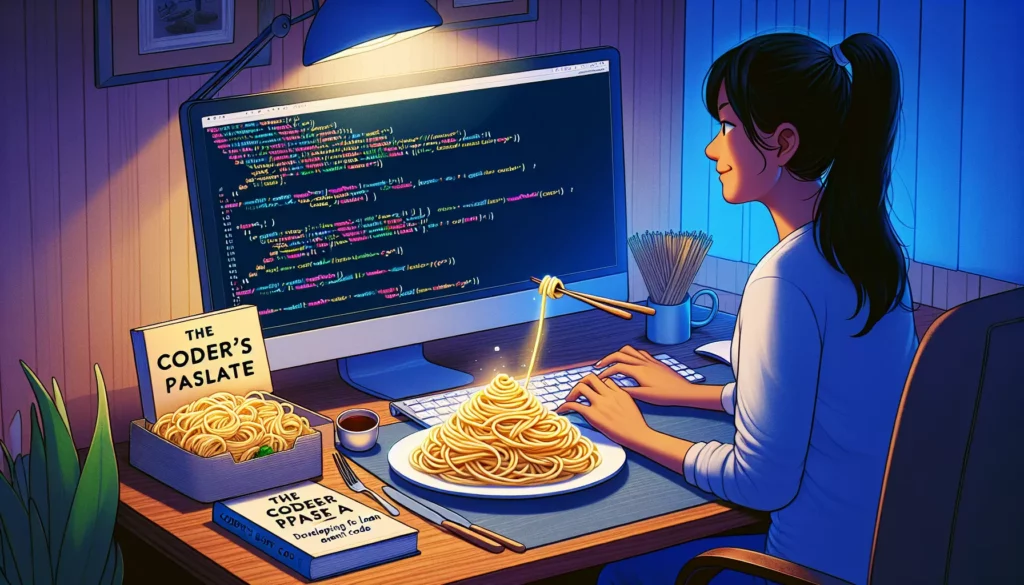
In the world of programming, writing code is akin to crafting a culinary masterpiece. Just as a chef refines their palate to discern the subtleties of flavors, a programmer must develop a keen sense for clean, efficient, and elegant code. This “coder’s palate” is not innate but cultivated through experience, practice, and a deep understanding of programming principles. In this comprehensive guide, we’ll explore how to develop your coder’s palate, enabling you to create code that’s not just functional, but truly exemplary.
1. Understanding the Ingredients: The Basics of Clean Code
Before we dive into the more complex aspects of code quality, let’s start with the fundamental ingredients that make up clean code:
1.1 Readability
Clean code should be easily readable, not just by machines, but by humans. This means using clear and descriptive variable names, consistent indentation, and logical structure. For example:
// Poor readability
function calc(a,b,c) {
return a*b+c;
}
// Good readability
function calculateTotalPrice(basePrice, taxRate, shippingCost) {
return basePrice * (1 + taxRate) + shippingCost;
}
1.2 Simplicity
The KISS principle (Keep It Simple, Stupid) applies strongly to coding. Avoid unnecessary complexity. Simple code is easier to understand, maintain, and debug.
1.3 DRY (Don’t Repeat Yourself)
Avoid duplicating code. If you find yourself writing the same logic in multiple places, it’s time to abstract it into a function or class.
1.4 Single Responsibility Principle
Each function or class should have a single, well-defined purpose. This makes your code more modular and easier to maintain.
2. Developing Your Palate: Practices for Writing Better Code
Now that we’ve covered the basics, let’s explore practices that will help you develop a more refined coder’s palate:
2.1 Code Review
Regularly participating in code reviews, both as a reviewer and a reviewee, can significantly improve your coding skills. It exposes you to different coding styles and problem-solving approaches.
2.2 Refactoring
Refactoring is the process of restructuring existing code without changing its external behavior. It’s a crucial skill for improving code quality. Here’s a simple example:
// Before refactoring
function getFullName(user) {
return user.firstName + " " + user.lastName;
}
// After refactoring
function getFullName({ firstName, lastName }) {
return `${firstName} ${lastName}`;
}
2.3 Learning Design Patterns
Design patterns are reusable solutions to common programming problems. Familiarizing yourself with these patterns can help you write more efficient and maintainable code.
2.4 Writing Tests
Writing unit tests for your code not only ensures its correctness but also encourages you to write more modular and testable code. Here’s a simple example using Jest:
// Function to test
function add(a, b) {
return a + b;
}
// Test
test('adds 1 + 2 to equal 3', () => {
expect(add(1, 2)).toBe(3);
});
3. Acquiring a Taste for Efficiency: Optimizing Your Code
Efficiency is a crucial aspect of good code. Let’s explore some ways to optimize your code:
3.1 Time Complexity
Understanding Big O notation and being able to analyze the time complexity of your algorithms is crucial. For example, consider these two implementations of finding the maximum number in an array:
// O(n^2) time complexity
function findMax(arr) {
for (let i = 0; i < arr.length; i++) {
let isMax = true;
for (let j = 0; j < arr.length; j++) {
if (arr[j] > arr[i]) {
isMax = false;
break;
}
}
if (isMax) return arr[i];
}
}
// O(n) time complexity
function findMaxEfficient(arr) {
let max = arr[0];
for (let i = 1; i < arr.length; i++) {
if (arr[i] > max) max = arr[i];
}
return max;
}
The second implementation is much more efficient, especially for large arrays.
3.2 Space Complexity
In addition to time complexity, it’s important to consider space complexity. Sometimes, you can trade space for time or vice versa. For example, memoization can significantly speed up recursive algorithms at the cost of additional memory usage.
3.3 Choosing the Right Data Structures
Selecting the appropriate data structure can greatly impact your code’s efficiency. For instance, using a Set instead of an Array for checking element existence can improve performance:
// Using Array (O(n) time complexity for checking existence)
const arr = [1, 2, 3, 4, 5];
console.log(arr.includes(3)); // true
// Using Set (O(1) time complexity for checking existence)
const set = new Set([1, 2, 3, 4, 5]);
console.log(set.has(3)); // true
4. The Art of Seasoning: Adding Elegance to Your Code
Elegant code goes beyond being clean and efficient; it demonstrates a deep understanding of the language and problem domain. Here are some ways to add elegance to your code:
4.1 Functional Programming Techniques
Functional programming can lead to more concise and elegant code. For example, using map, filter, and reduce can often replace complex loops:
// Imperative approach
const numbers = [1, 2, 3, 4, 5];
let sum = 0;
for (let i = 0; i < numbers.length; i++) {
if (numbers[i] % 2 === 0) {
sum += numbers[i] * 2;
}
}
// Functional approach
const sum = numbers
.filter(n => n % 2 === 0)
.map(n => n * 2)
.reduce((acc, n) => acc + n, 0);
4.2 Expressive Code
Strive to make your code self-explanatory. Use meaningful variable and function names, and structure your code in a way that clearly expresses its intent.
4.3 Consistent Style
Adhere to a consistent coding style throughout your project. This includes things like indentation, naming conventions, and code organization. Many languages have style guides (e.g., PEP 8 for Python) that you can follow.
4.4 Smart Use of Language Features
Take advantage of language-specific features to write more elegant code. For example, in JavaScript, you can use destructuring and the spread operator:
// Without destructuring
function printUserInfo(user) {
console.log(`${user.name} (${user.age})`);
}
// With destructuring
function printUserInfo({ name, age }) {
console.log(`${name} (${age})`);
}
// Using spread operator
const arr1 = [1, 2, 3];
const arr2 = [4, 5, 6];
const combined = [...arr1, ...arr2]; // [1, 2, 3, 4, 5, 6]
5. Tasting Notes: Recognizing Code Smells
Just as a sommelier can detect flaws in wine, a skilled programmer can identify “code smells” – indicators of potential problems in code. Here are some common code smells to watch out for:
5.1 Duplicated Code
If you see the same code repeated in multiple places, it’s a sign that you should refactor it into a reusable function or class.
5.2 Long Methods
Methods that are too long are often trying to do too much. Break them down into smaller, more focused methods.
5.3 Large Classes
Classes with too many responsibilities violate the Single Responsibility Principle. Consider splitting them into smaller, more focused classes.
5.4 Long Parameter List
If a function has too many parameters, it might be a sign that you need to restructure your code. Consider using an object to group related parameters:
// Before
function createUser(name, age, email, address, phone) {
// ...
}
// After
function createUser({ name, age, email, address, phone }) {
// ...
}
createUser({
name: "John Doe",
age: 30,
email: "john@example.com",
address: "123 Main St",
phone: "555-1234"
});
6. The Coder’s Cookbook: Recipes for Common Programming Tasks
As you develop your coder’s palate, you’ll start to recognize common patterns and solutions. Here are a few “recipes” for common programming tasks:
6.1 Implementing a Singleton
A Singleton is a design pattern that restricts a class to a single instance. Here’s how you might implement it in JavaScript:
class Singleton {
constructor() {
if (Singleton.instance) {
return Singleton.instance;
}
Singleton.instance = this;
}
// Methods...
}
const instance1 = new Singleton();
const instance2 = new Singleton();
console.log(instance1 === instance2); // true
6.2 Debouncing a Function
Debouncing is a technique used to limit the rate at which a function gets called. It’s particularly useful for optimizing event handlers:
function debounce(func, delay) {
let timeoutId;
return function (...args) {
clearTimeout(timeoutId);
timeoutId = setTimeout(() => func.apply(this, args), delay);
};
}
const debouncedSearch = debounce((query) => {
// Perform search operation
console.log(`Searching for: ${query}`);
}, 300);
// Usage
searchInput.addEventListener('input', (e) => debouncedSearch(e.target.value));
6.3 Implementing a Basic Promise
Understanding how Promises work is crucial for modern JavaScript development. Here’s a simple implementation of a Promise-like object:
class MyPromise {
constructor(executor) {
this.state = 'pending';
this.value = undefined;
this.callbacks = [];
const resolve = (value) => {
if (this.state === 'pending') {
this.state = 'fulfilled';
this.value = value;
this.callbacks.forEach(callback => callback(value));
}
};
executor(resolve);
}
then(onFulfilled) {
if (this.state === 'fulfilled') {
onFulfilled(this.value);
} else {
this.callbacks.push(onFulfilled);
}
}
}
// Usage
const promise = new MyPromise((resolve) => {
setTimeout(() => resolve('Hello, World!'), 1000);
});
promise.then((value) => console.log(value)); // Logs "Hello, World!" after 1 second
7. Cultivating Your Palate: Continuous Learning and Improvement
Developing your coder’s palate is an ongoing process. Here are some strategies for continuous improvement:
7.1 Read High-Quality Code
Study the source code of well-regarded open-source projects. This exposes you to best practices and elegant solutions to complex problems.
7.2 Practice Coding Challenges
Platforms like LeetCode, HackerRank, and CodeWars offer a wide range of coding challenges that can help you hone your problem-solving skills and learn new algorithms and data structures.
7.3 Contribute to Open Source
Contributing to open-source projects allows you to work on real-world problems and receive feedback from experienced developers.
7.4 Stay Updated
Keep up with the latest developments in your programming languages and frameworks. Follow relevant blogs, podcasts, and attend conferences or meetups when possible.
7.5 Teach Others
Teaching is an excellent way to solidify your understanding. Consider starting a blog, giving presentations, or mentoring junior developers.
Conclusion: Savoring the Art of Coding
Developing a coder’s palate is about more than just writing functional code; it’s about crafting elegant solutions that are a joy to read and maintain. It’s about understanding the nuances of your programming language and using them effectively. It’s about constantly refining your skills and striving for excellence.
Remember, like any skill, developing your coder’s palate takes time and practice. Be patient with yourself, stay curious, and never stop learning. With dedication and persistence, you’ll find yourself writing code that’s not just correct, but truly delightful – a feast for the coder’s palate.
As you continue on your journey to mastery, platforms like AlgoCademy can be invaluable resources. With its focus on algorithmic thinking, problem-solving, and practical coding skills, AlgoCademy provides the perfect environment to refine your coder’s palate. From interactive coding tutorials to AI-powered assistance, it offers the tools and guidance you need to progress from beginner-level coding to tackling complex technical interviews.
So, keep coding, keep learning, and keep refining your taste for clean, efficient, and elegant code. Your future self – and your fellow developers – will thank you for it.