The Coder’s Nightmare Journal: Turning Anxiety Dreams into Problem-Solving Strategies
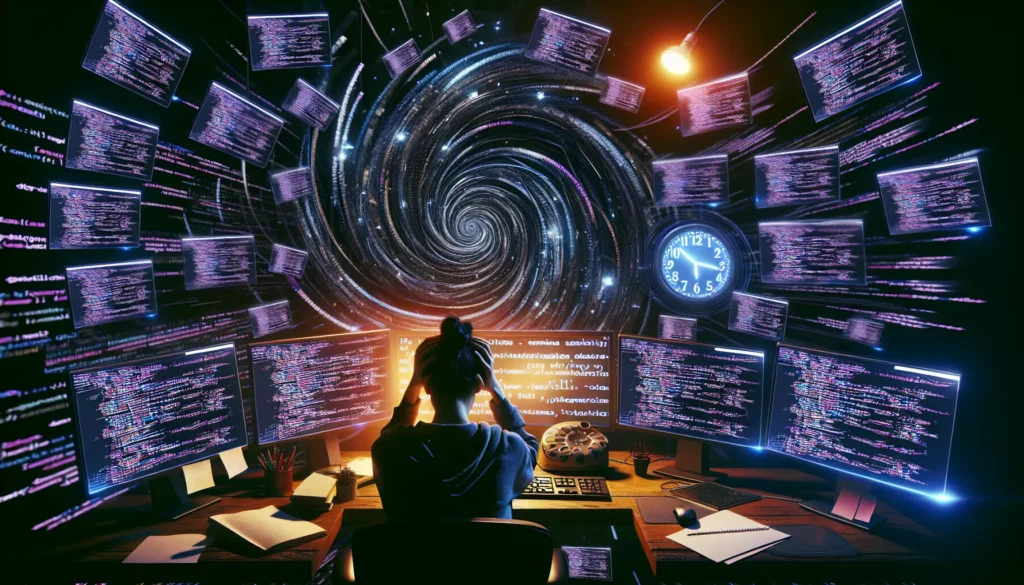
As programmers, we often find ourselves immersed in a world of logic, algorithms, and endless lines of code. But what happens when our subconscious mind takes over during sleep, weaving our coding challenges into bizarre and often anxiety-inducing dreams? Welcome to “The Coder’s Nightmare Journal,” where we explore the fascinating intersection of programming anxiety dreams and problem-solving strategies. In this comprehensive guide, we’ll delve into the psychology behind these dreams, analyze common coding nightmares, and discover how to transform these nocturnal experiences into powerful tools for improving your coding skills and conquering technical interviews.
Understanding the Psychology of Coding Anxiety Dreams
Before we dive into specific nightmares and their potential solutions, it’s essential to understand why programmers often experience anxiety dreams related to their work. Several factors contribute to this phenomenon:
- Cognitive load: Programming requires intense mental focus and problem-solving skills. The brain often processes this information during sleep, leading to coding-related dreams.
- Imposter syndrome: Many developers, especially those preparing for interviews with major tech companies, experience feelings of inadequacy or fear of being exposed as a “fraud.”
- Deadline pressure: Tight project deadlines and the constant need to deliver high-quality code can create stress that manifests in dreams.
- Perfectionism: The desire to write flawless code and solve complex problems efficiently can lead to anxiety about potential errors or suboptimal solutions.
- Continuous learning: The ever-evolving nature of technology means programmers must constantly update their skills, which can create anxiety about falling behind.
Understanding these underlying factors can help you contextualize your coding nightmares and approach them with a more analytical mindset. Now, let’s explore some common coding nightmares and how to transform them into problem-solving strategies.
Common Coding Nightmares and Their Solutions
1. The Infinite Loop Nightmare
The Dream: You find yourself trapped in an endless loop, unable to break free or terminate the program. The code keeps executing, consuming more and more resources, and you’re powerless to stop it.
The Solution: This nightmare often reflects feelings of being overwhelmed or stuck in a particular problem. To address this:
- Practice identifying and implementing proper exit conditions in your loops.
- Develop a habit of using debugging tools to step through your code and identify potential infinite loops.
- Study time complexity and optimize your algorithms to avoid unnecessary iterations.
Coding Exercise: Implement a function that detects and breaks out of potential infinite loops in a given piece of code. Here’s a simple example in Python:
def safe_execute(func, max_iterations=1000):
iteration_count = 0
def wrapper(*args, **kwargs):
nonlocal iteration_count
iteration_count += 1
if iteration_count > max_iterations:
raise Exception("Potential infinite loop detected")
return func(*args, **kwargs)
return wrapper
@safe_execute
def potentially_infinite_function(n):
while n != 1:
if n % 2 == 0:
n = n // 2
else:
n = 3 * n + 1
return n
try:
result = potentially_infinite_function(27)
print(f"Result: {result}")
except Exception as e:
print(f"Error: {e}")
2. The Syntax Error Apocalypse
The Dream: Your code is filled with an endless stream of syntax errors. No matter how many you fix, new ones keep appearing, and your program refuses to compile or run.
The Solution: This nightmare often stems from a fear of making mistakes or a lack of confidence in your coding skills. To overcome this:
- Regularly practice writing code without relying too heavily on auto-completion or syntax highlighting.
- Familiarize yourself with common syntax errors and their causes.
- Develop a systematic approach to debugging and error resolution.
Coding Exercise: Create a simple syntax checker that identifies common syntax errors in a given piece of code. Here’s a basic implementation in JavaScript:
function checkSyntax(code) {
const commonErrors = [
{ regex: /[{[(]\s*[})]\s*[})\]]/g, message: "Mismatched brackets or parentheses" },
{ regex: /=(?!=)/g, message: "Single equals used for comparison instead of double equals" },
{ regex: /\b(if|while|for)\s*[^(]/g, message: "Missing parentheses after control statement" },
{ regex: /[;,]\s*[;,]/g, message: "Unnecessary semicolon or comma" },
];
const errors = [];
commonErrors.forEach(({ regex, message }) => {
const matches = code.match(regex);
if (matches) {
errors.push(`${message} (${matches.length} occurrence${matches.length > 1 ? 's' : ''})`);
}
});
return errors.length ? errors : ["No common syntax errors detected"];
}
const code = `
function example() {
if (x = 5) {
console.log("Hello");
};
return [1, 2,];
}
`;
console.log(checkSyntax(code));
3. The Memory Leak Maze
The Dream: You’re lost in a labyrinth of objects and references, desperately trying to find and plug memory leaks. The more you search, the more complex the maze becomes, and your application’s memory usage keeps growing uncontrollably.
The Solution: This nightmare often reflects concerns about code efficiency and resource management. To address this:
- Study memory management techniques specific to your programming language.
- Learn to use memory profiling tools to identify and resolve leaks.
- Practice writing code with proper object disposal and reference management.
Coding Exercise: Implement a simple memory leak detector for a class that manages resources. Here’s an example in C++:
#include <iostream>
#include <unordered_set>
class ResourceManager {
private:
static std::unordered_set<ResourceManager*> instances;
int* resource;
public:
ResourceManager() {
resource = new int(0);
instances.insert(this);
}
~ResourceManager() {
delete resource;
instances.erase(this);
}
static void checkLeaks() {
if (!instances.empty()) {
std::cout << "Memory leak detected! " << instances.size() << " instance(s) not properly deleted." << std::endl;
} else {
std::cout << "No memory leaks detected." << std::endl;
}
}
};
std::unordered_set<ResourceManager*> ResourceManager::instances;
int main() {
ResourceManager* rm1 = new ResourceManager();
ResourceManager* rm2 = new ResourceManager();
delete rm1;
// Intentionally not deleting rm2 to simulate a memory leak
ResourceManager::checkLeaks();
return 0;
}
4. The Deadline Doomsday
The Dream: You’re racing against time to complete a crucial project. The deadline is approaching at an impossibly fast rate, and no matter how quickly you type, you can’t seem to make progress. Your code editor starts glitching, deleting your work, and the clock keeps ticking louder and louder.
The Solution: This nightmare often stems from time management anxiety and the pressure to deliver results quickly. To combat this:
- Develop strong time management skills and learn to break down large projects into manageable tasks.
- Practice estimating task durations more accurately to set realistic deadlines.
- Familiarize yourself with productivity techniques like the Pomodoro method to maintain focus and avoid burnout.
Coding Exercise: Create a simple task management system with deadline tracking. Here’s a basic implementation in Python:
import datetime
class Task:
def __init__(self, name, deadline):
self.name = name
self.deadline = deadline
self.completed = False
def complete(self):
self.completed = True
def time_remaining(self):
if self.completed:
return "Task completed"
now = datetime.datetime.now()
remaining = self.deadline - now
if remaining.total_seconds() < 0:
return "Deadline passed"
return str(remaining)
class ProjectManager:
def __init__(self):
self.tasks = []
def add_task(self, task):
self.tasks.append(task)
def view_tasks(self):
for task in self.tasks:
status = "Completed" if task.completed else "Pending"
print(f"{task.name}: {status} - Time remaining: {task.time_remaining()}")
# Usage example
pm = ProjectManager()
pm.add_task(Task("Implement login system", datetime.datetime.now() + datetime.timedelta(days=2)))
pm.add_task(Task("Write unit tests", datetime.datetime.now() + datetime.timedelta(days=1)))
pm.add_task(Task("Deploy to production", datetime.datetime.now() + datetime.timedelta(days=3)))
pm.view_tasks()
# Complete a task
pm.tasks[1].complete()
print("\nAfter completing a task:")
pm.view_tasks()
5. The Algorithm Abyss
The Dream: You’re standing at the edge of a deep, dark abyss filled with swirling algorithms and data structures. As you try to implement a solution, the abyss keeps shifting and changing, making it impossible to find a stable algorithm that works.
The Solution: This nightmare often reflects the challenge of mastering complex algorithms and data structures. To overcome this:
- Develop a strong foundation in fundamental algorithms and data structures.
- Practice implementing and analyzing various algorithms to understand their strengths and weaknesses.
- Learn to recognize patterns in problem-solving and map them to appropriate algorithmic solutions.
Coding Exercise: Implement a versatile algorithm selector that chooses the most appropriate sorting algorithm based on input characteristics. Here’s an example in Java:
import java.util.Arrays;
import java.util.Random;
public class AlgorithmSelector {
public static void sort(int[] arr) {
if (arr.length < 50) {
insertionSort(arr);
} else if (isNearlySorted(arr)) {
insertionSort(arr);
} else if (arr.length < 1000) {
quickSort(arr, 0, arr.length - 1);
} else {
mergeSort(arr, 0, arr.length - 1);
}
}
private static boolean isNearlySorted(int[] arr) {
int outOfOrder = 0;
for (int i = 0; i < arr.length - 1; i++) {
if (arr[i] > arr[i + 1]) {
outOfOrder++;
}
}
return outOfOrder < arr.length / 10; // Consider nearly sorted if less than 10% out of order
}
private static void insertionSort(int[] arr) {
for (int i = 1; i < arr.length; i++) {
int key = arr[i];
int j = i - 1;
while (j >= 0 && arr[j] > key) {
arr[j + 1] = arr[j];
j--;
}
arr[j + 1] = key;
}
}
private static void quickSort(int[] arr, int low, int high) {
if (low < high) {
int pi = partition(arr, low, high);
quickSort(arr, low, pi - 1);
quickSort(arr, pi + 1, high);
}
}
private static int partition(int[] arr, int low, int high) {
int pivot = arr[high];
int i = low - 1;
for (int j = low; j < high; j++) {
if (arr[j] < pivot) {
i++;
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
int temp = arr[i + 1];
arr[i + 1] = arr[high];
arr[high] = temp;
return i + 1;
}
private static void mergeSort(int[] arr, int left, int right) {
if (left < right) {
int mid = (left + right) / 2;
mergeSort(arr, left, mid);
mergeSort(arr, mid + 1, right);
merge(arr, left, mid, right);
}
}
private static void merge(int[] arr, int left, int mid, int right) {
int n1 = mid - left + 1;
int n2 = right - mid;
int[] L = new int[n1];
int[] R = new int[n2];
for (int i = 0; i < n1; ++i) L[i] = arr[left + i];
for (int j = 0; j < n2; ++j) R[j] = arr[mid + 1 + j];
int i = 0, j = 0, k = left;
while (i < n1 && j < n2) {
if (L[i] <= R[j]) {
arr[k] = L[i];
i++;
} else {
arr[k] = R[j];
j++;
}
k++;
}
while (i < n1) {
arr[k] = L[i];
i++;
k++;
}
while (j < n2) {
arr[k] = R[j];
j++;
k++;
}
}
public static void main(String[] args) {
Random rand = new Random();
int[] smallArray = rand.ints(30, 0, 100).toArray();
int[] nearlySortedArray = {1, 2, 3, 5, 4, 6, 8, 7, 9, 10};
int[] mediumArray = rand.ints(500, 0, 1000).toArray();
int[] largeArray = rand.ints(5000, 0, 10000).toArray();
System.out.println("Small array:");
System.out.println("Before: " + Arrays.toString(smallArray));
sort(smallArray);
System.out.println("After: " + Arrays.toString(smallArray));
System.out.println("\nNearly sorted array:");
System.out.println("Before: " + Arrays.toString(nearlySortedArray));
sort(nearlySortedArray);
System.out.println("After: " + Arrays.toString(nearlySortedArray));
System.out.println("\nMedium array:");
System.out.println("Before: " + Arrays.toString(mediumArray));
sort(mediumArray);
System.out.println("After: " + Arrays.toString(mediumArray));
System.out.println("\nLarge array:");
System.out.println("Before: " + Arrays.toString(largeArray));
sort(largeArray);
System.out.println("After: " + Arrays.toString(largeArray));
}
}
Turning Nightmares into Learning Opportunities
Now that we’ve explored some common coding nightmares and their potential solutions, let’s discuss how to transform these anxiety-inducing dreams into valuable learning opportunities:
- Keep a dream journal: Write down your coding nightmares as soon as you wake up. This practice helps you remember the details and identify recurring themes or anxieties.
- Analyze the underlying fears: Reflect on what each nightmare represents in terms of your coding challenges or career concerns. Are you worried about specific technologies, interview performance, or project deadlines?
- Create action plans: For each nightmare, develop a concrete plan to address the underlying issue. This might involve studying specific topics, practicing coding exercises, or seeking mentorship.
- Implement dream-inspired projects: Use elements from your coding nightmares as inspiration for personal projects. This approach helps you face your fears head-on and turn them into tangible learning experiences.
- Practice mindfulness and stress-reduction techniques: Incorporate meditation, deep breathing exercises, or other relaxation methods into your daily routine to reduce overall anxiety and improve sleep quality.
Preparing for Technical Interviews: From Nightmares to Success
Many coding nightmares stem from the pressure of technical interviews, especially when aiming for positions at major tech companies. Here are some strategies to transform your interview anxiety into preparation success:
- Mock interviews: Regularly participate in mock technical interviews with peers or mentors. This practice helps you become comfortable with the interview format and reduces anxiety.
- Time-boxed problem-solving: Set time limits for solving coding problems to simulate interview conditions. This approach helps you manage time pressure and improves your ability to think clearly under stress.
- Study common interview topics: Focus on mastering fundamental data structures, algorithms, and system design concepts frequently covered in technical interviews.
- Practice explaining your thought process: Work on articulating your problem-solving approach clearly and concisely. This skill is crucial for performing well in technical interviews.
- Build a portfolio of projects: Develop personal projects that showcase your skills and passion for coding. These projects can serve as talking points during interviews and boost your confidence.
Embracing Continuous Learning and Growth
Remember that coding nightmares often reflect our desire for growth and improvement as programmers. Embrace these challenges as opportunities to expand your skills and knowledge. Here are some final tips for continuous learning and professional development:
- Stay updated with industry trends: Regularly read tech blogs, attend conferences, and participate in online communities to stay informed about the latest developments in your field.
- Contribute to open-source projects: Participating in open-source development exposes you to diverse codebases and collaboration styles, enhancing your skills and expanding your network.
- Teach others: Sharing your knowledge through blog posts, tutorials, or mentoring junior developers can reinforce your understanding and boost your confidence.
- Set personal coding challenges: Regularly challenge yourself with coding exercises or projects that push you out of your comfort zone.
- Embrace failure as a learning opportunity: Remember that mistakes and setbacks are natural parts of the learning process. Analyze your errors, learn from them, and use them to fuel your growth as a programmer.
Conclusion
Coding nightmares may be unsettling, but they also offer valuable insights into our fears, aspirations, and areas for improvement as programmers. By understanding the psychology behind these dreams and implementing strategies to address them, we can transform our anxieties into powerful tools for growth and success.
Remember that every programmer, from beginners to seasoned professionals, faces challenges and moments of self-doubt. The key is to approach these experiences with curiosity, resilience, and a commitment to continuous learning. By doing so, you’ll not only conquer your coding nightmares but also evolve into a more skilled, confident, and adaptable developer.
So, the next time you find yourself trapped in a coding nightmare, take a deep breath, grab your dream journal, and get ready to turn that anxiety into your next big breakthrough. Happy coding, and sweet dreams!