The Coder’s Emotional Intelligence: Developing Empathy for Your Future Self Through Comments
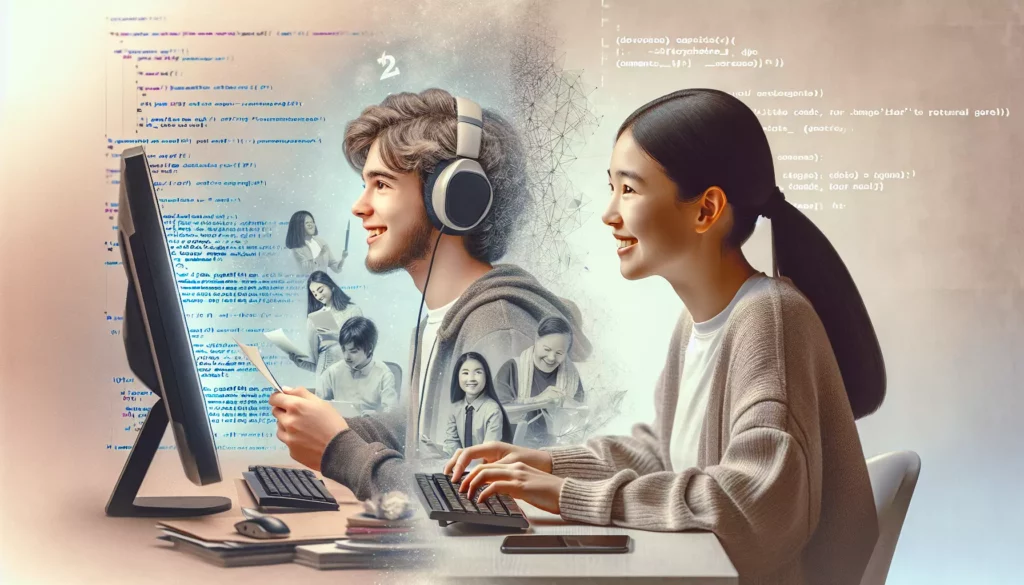
In the fast-paced world of software development, where lines of code flow like rivers and algorithms bloom like digital flowers, it’s easy to get lost in the immediacy of problem-solving. We often forget that coding is not just a dialogue with the computer but a conversation with our future selves and our fellow developers. This is where the concept of emotional intelligence in coding comes into play, particularly through the art of writing comments.
The Importance of Emotional Intelligence in Coding
Emotional intelligence, often abbreviated as EQ, is the ability to understand, use, and manage your own emotions in positive ways to relieve stress, communicate effectively, empathize with others, overcome challenges and defuse conflict. In the context of coding, emotional intelligence manifests in various ways, but one of the most crucial is in how we communicate our thought processes and decisions through code comments.
When we write code, we’re not just instructing a machine; we’re leaving a trail of breadcrumbs for our future selves and other developers who will interact with our code. This is where empathy comes into play – the ability to understand and share the feelings of another, even if that “other” is you, six months from now, trying to decipher why you made a particular coding decision.
Comments as a Form of Time Travel
Imagine you’re working on a complex algorithm for a technical interview preparation platform like AlgoCademy. You’ve just spent hours optimizing a sorting function for large datasets. In the moment, every line of code makes perfect sense to you. But will it make sense when you revisit it weeks or months later?
This is where well-crafted comments become your time machine. They allow you to communicate across time, explaining your reasoning, highlighting potential pitfalls, and providing context that might not be immediately apparent from the code alone.
Example: Commenting on a Complex Algorithm
def optimized_quick_sort(arr, low, high):
# This implementation of QuickSort uses a three-way partitioning scheme
# to handle arrays with many duplicate elements more efficiently.
# It divides the array into three parts: elements smaller than the pivot,
# elements equal to the pivot, and elements larger than the pivot.
if low < high:
# Choose the middle element as the pivot to avoid worst-case scenarios
# in already sorted or reverse sorted arrays
pivot = arr[(low + high) // 2]
i, j, k = low, low, high
while j <= k:
if arr[j] < pivot:
arr[i], arr[j] = arr[j], arr[i]
i += 1
j += 1
elif arr[j] > pivot:
arr[j], arr[k] = arr[k], arr[j]
k -= 1
else:
j += 1
# Recursively sort the partitions
optimized_quick_sort(arr, low, i - 1)
optimized_quick_sort(arr, k + 1, high)
return arr
In this example, the comments provide crucial context about the algorithm’s implementation, its advantages, and the reasoning behind certain choices (like pivot selection). This information is invaluable for anyone trying to understand or modify the code in the future.
The Psychology of Self-Compassion in Coding
Writing comments is an act of self-compassion. It’s acknowledging that your future self (or another developer) might struggle with understanding the code, and proactively providing help. This practice not only makes the codebase more maintainable but also fosters a mindset of continuous learning and improvement.
Consider the following scenarios:
- Debugging Under Pressure: You’re faced with a critical bug in a production system. Clear comments can significantly reduce the time and stress involved in identifying and fixing the issue.
- Onboarding New Team Members: Well-commented code acts as documentation, helping new developers understand the project more quickly and feel more confident in contributing.
- Code Reviews: Comments that explain the “why” behind certain decisions can lead to more constructive code reviews, focusing on architectural decisions rather than getting bogged down in implementation details.
Best Practices for Empathetic Commenting
To truly develop empathy for your future self and other developers through comments, consider these best practices:
1. Explain the Why, Not Just the What
Instead of merely describing what the code does, focus on explaining why it does it. This context is often what’s missing when trying to understand code months or years later.
2. Use Comments to Tell a Story
Think of your comments as telling the story of your code. What problem were you trying to solve? What constraints were you working under? What alternatives did you consider?
3. Keep Comments Updated
Outdated comments are often worse than no comments at all. Make updating comments part of your code modification process.
4. Use Clear and Concise Language
Avoid jargon or overly complex explanations. Write comments as if you’re explaining the code to a junior developer who is bright but may not have the same context you do.
5. Comment on Non-Obvious Edge Cases
If your code handles specific edge cases or has particular limitations, make sure to comment on these. It can save hours of debugging in the future.
6. Use TODO Comments Wisely
TODO comments can be helpful for marking areas that need future attention, but don’t let them become a graveyard of good intentions. Regularly review and address TODO items.
Example: Applying These Practices
def calculate_interview_score(coding_score, communication_score, problem_solving_score):
# We use a weighted average to calculate the overall interview score
# Rationale for weights:
# - Coding (50%): Core skill for software development
# - Communication (30%): Critical for team collaboration and client interaction
# - Problem Solving (20%): Important, but often reflected in coding score as well
weights = {
'coding': 0.5,
'communication': 0.3,
'problem_solving': 0.2
}
total_score = (
coding_score * weights['coding'] +
communication_score * weights['communication'] +
problem_solving_score * weights['problem_solving']
)
# TODO: Consider adding a normalization step if scores are not on the same scale
return total_score
In this example, the comments provide context for the weighting decision, explain the rationale, and include a TODO for a potential future improvement.
The Role of Comments in Learning and Interview Preparation
For platforms like AlgoCademy that focus on coding education and interview preparation, the practice of writing empathetic comments serves a dual purpose:
- Enhancing Learning: By explaining complex algorithms or data structures in comments, learners reinforce their own understanding and create valuable study resources.
- Interview Readiness: The ability to clearly explain code and decisions is a crucial skill in technical interviews. Practicing this through comments helps prepare candidates for the communication aspects of coding interviews.
Example: Commenting for Learning and Interviews
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def is_balanced(root):
# This function checks if a binary tree is height-balanced
# A height-balanced binary tree is one where the depths of any two leaf nodes differ by at most one
def check_balance(node):
# This helper function returns a tuple: (is_balanced, height)
# Using a tuple allows us to check balance and calculate height in one pass,
# reducing time complexity from O(n^2) to O(n)
if not node:
return True, 0
left_balanced, left_height = check_balance(node.left)
right_balanced, right_height = check_balance(node.right)
# The tree is balanced if:
# 1. Both subtrees are balanced
# 2. The height difference between left and right subtrees is at most 1
is_balanced = left_balanced and right_balanced and abs(left_height - right_height) <= 1
# The height of the current node is the max of its subtrees, plus 1 for itself
height = max(left_height, right_height) + 1
return is_balanced, height
# We only need to return the balance status, not the height
return check_balance(root)[0]
In this example, the comments not only explain the algorithm but also touch on its efficiency, which is a common topic in coding interviews. This style of commenting helps learners understand the problem-solving approach and prepares them to articulate their thoughts during interviews.
The Impact of Empathetic Commenting on Team Dynamics
The practice of writing empathetic comments extends beyond individual benefits; it significantly impacts team dynamics and overall code quality:
1. Fostering a Culture of Knowledge Sharing
When developers consistently write clear, informative comments, they create an environment where knowledge is freely shared. This can lead to faster onboarding of new team members and more efficient collaboration on complex projects.
2. Reducing Technical Debt
Well-commented code is easier to maintain and refactor. By investing time in writing good comments, teams can reduce the accumulation of technical debt that often results from poorly understood or documented code.
3. Enhancing Code Reviews
Comments that explain the reasoning behind coding decisions can lead to more productive code reviews. Reviewers can focus on architectural and algorithmic choices rather than getting bogged down in trying to understand the basic functionality of the code.
4. Improving Overall Code Quality
The process of writing comments often leads developers to reflect on their code, potentially identifying areas for improvement or simplification. This reflective practice can result in cleaner, more efficient code.
Balancing Comment Quantity and Quality
While the benefits of commenting are clear, it’s important to strike a balance. Over-commenting can be as problematic as under-commenting. Here are some guidelines:
1. Let the Code Speak for Itself
Well-written code should be largely self-explanatory. Use comments to provide context and explain complex logic, not to describe obvious operations.
2. Use Meaningful Variable and Function Names
Descriptive names can reduce the need for comments. For example, calculate_total_price()
is self-explanatory and may not need a comment, whereas ct()
would benefit from an explanation.
3. Comment at the Right Level of Abstraction
High-level comments explaining the overall purpose and structure of a module or class are often more valuable than line-by-line explanations.
4. Use Code Documentation Tools
Tools like Javadoc for Java or Docstrings in Python provide structured ways to document code. These can be especially useful for API documentation.
Example: Balanced Commenting
class InterviewSimulator:
def __init__(self, difficulty='medium'):
self.difficulty = difficulty
self.questions = self._load_questions()
self.current_question = None
def _load_questions(self):
# Load questions from a database or file based on difficulty
# This is a placeholder implementation
return [f"Sample question {i} for {self.difficulty} difficulty" for i in range(10)]
def start_interview(self):
# Initialize the interview session
self.current_question = 0
return self.get_next_question()
def get_next_question(self):
if self.current_question < len(self.questions):
question = self.questions[self.current_question]
self.current_question += 1
return question
else:
return None # Interview is complete
def submit_answer(self, answer):
# In a real implementation, this would evaluate the answer
# For now, we'll just acknowledge receipt
return f"Answer received for question {self.current_question}"
def end_interview(self):
# Clean up and possibly generate a report
self.current_question = None
return "Interview completed"
In this example, comments are used sparingly but effectively. They provide context where necessary (like explaining the placeholder implementation in _load_questions
) but don’t overexplain simple methods like get_next_question
.
The Future of Code Comments: AI and Natural Language Processing
As we look to the future, the role of comments in code is likely to evolve with advancements in AI and natural language processing:
1. Automated Comment Generation
AI tools may be able to generate initial comments based on code analysis, which developers can then refine. This could help ensure a baseline level of documentation across projects.
2. Natural Language Queries
Future IDEs might allow developers to ask questions about code in natural language, with AI interpreting both the code and its comments to provide answers.
3. Comment Quality Analysis
AI tools could analyze comments for clarity, completeness, and consistency with the code, suggesting improvements or flagging outdated comments.
4. Context-Aware Comment Assistance
IDEs might offer context-aware suggestions for comments based on the complexity of the code, coding patterns, and project-specific documentation requirements.
Conclusion: Empathy as a Coding Superpower
In the world of coding, empathy – particularly empathy for your future self and fellow developers – is a superpower. It’s a skill that extends beyond technical proficiency, touching on communication, foresight, and emotional intelligence. By cultivating this empathy through thoughtful, context-rich comments, we not only make our code more maintainable and our teams more efficient but also contribute to a more collaborative and understanding development community.
As you continue your coding journey, whether you’re preparing for interviews with platforms like AlgoCademy or working on complex projects, remember that every line of code you write is a message to the future. Make it a message of clarity, insight, and empathy. Your future self – and your fellow developers – will thank you.
In embracing this practice, we elevate coding from a mere technical skill to an art form – one where we not only solve problems but also tell stories, share knowledge, and build bridges across time and teams. So the next time you sit down to code, take a moment to consider: How can you make your code speak not just to the computer, but to the humans who will interact with it in the future? In doing so, you’ll not only become a better coder but a better collaborator and, ultimately, a more well-rounded professional in the ever-evolving world of software development.