The Coder’s Closet: Organizing Your Mental Models Like a Capsule Wardrobe
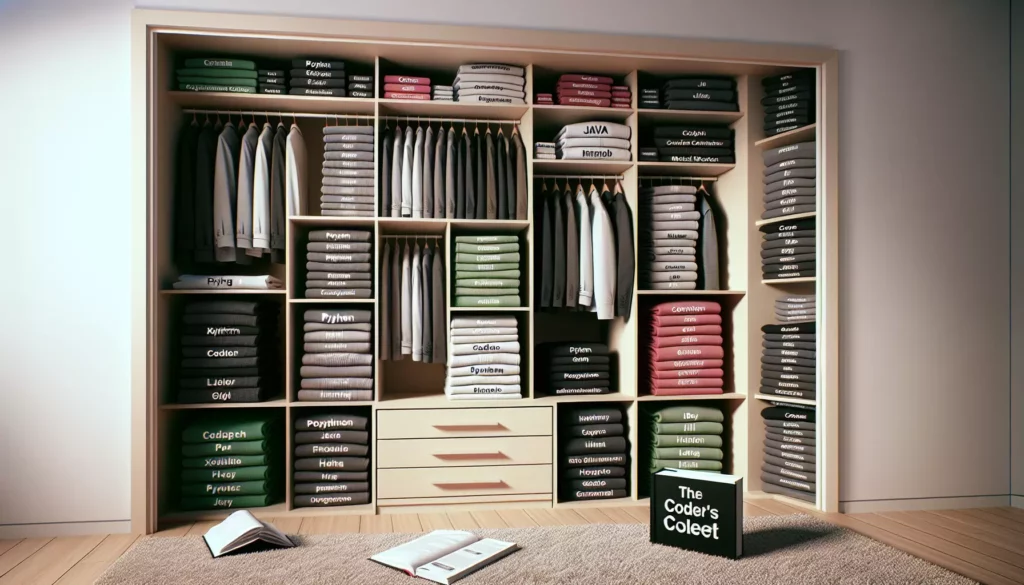
In the world of programming, just like in fashion, having a well-organized collection of essential elements can make all the difference. Enter the concept of the “Coder’s Closet” – a metaphorical wardrobe filled not with clothes, but with mental models and programming concepts. By organizing your coding knowledge like a capsule wardrobe, you can create a powerful toolkit that allows you to tackle a wide range of programming challenges with efficiency and style.
Understanding the Capsule Wardrobe Concept
Before we dive into the world of coding, let’s take a moment to understand the capsule wardrobe concept. A capsule wardrobe is a curated collection of versatile, timeless pieces that can be mixed and matched to create a variety of outfits. The idea is to have a small number of high-quality items that work well together, rather than a closet overflowing with clothes you rarely wear.
The benefits of a capsule wardrobe include:
- Simplicity and ease of choice
- Versatility and adaptability
- Quality over quantity
- Efficiency in decision-making
Now, let’s see how we can apply these principles to organize our coding knowledge and mental models.
The Coder’s Capsule Closet: Essential Mental Models
Just as a capsule wardrobe consists of essential clothing items, a coder’s mental model capsule should include fundamental concepts that can be applied across various programming scenarios. Here are some key “pieces” to include in your Coder’s Closet:
1. Data Structures
Data structures are the foundation of any programmer’s toolkit. They’re like the basic t-shirts and jeans of your coding wardrobe – versatile, essential, and applicable in numerous situations. Key data structures to master include:
- Arrays and Lists
- Stacks and Queues
- Hash Tables
- Trees (Binary Trees, Binary Search Trees)
- Graphs
- Heaps
Understanding these data structures allows you to organize and manipulate data efficiently, forming the basis for more complex algorithms and problem-solving techniques.
2. Algorithmic Patterns
Algorithmic patterns are like the versatile blazer or little black dress of your coding wardrobe. They can be adapted to solve a wide range of problems and form the backbone of efficient problem-solving. Some essential algorithmic patterns include:
- Two Pointers
- Sliding Window
- Binary Search
- Depth-First Search (DFS)
- Breadth-First Search (BFS)
- Dynamic Programming
- Greedy Algorithms
Mastering these patterns allows you to approach problems with a structured mindset, identifying similarities to known problem types and applying proven solution strategies.
3. Time and Space Complexity Analysis
Understanding time and space complexity is like knowing how to accessorize your outfits. It adds a layer of sophistication to your coding skills and helps you make informed decisions about algorithm choice and implementation. Key concepts include:
- Big O notation
- Best, average, and worst-case scenarios
- Space-time tradeoffs
- Amortized analysis
By incorporating complexity analysis into your mental model, you can evaluate and optimize your code for performance and efficiency.
4. Object-Oriented Programming (OOP) Principles
OOP principles are like the classic pieces in your wardrobe that never go out of style. They provide a structured approach to designing and organizing code, promoting reusability and maintainability. Key OOP concepts include:
- Encapsulation
- Inheritance
- Polymorphism
- Abstraction
- Singleton
- Factory
- Observer
- Strategy
- Decorator
- Model-View-Controller (MVC)
- Data manipulation (Data Structures, Algorithms)
- Code organization (OOP, Design Patterns)
- Problem-solving strategies (Algorithmic Patterns)
- Performance considerations (Time and Space Complexity)
- How does the choice of data structure affect the time complexity of an algorithm?
- How can design patterns be implemented using OOP principles?
- How do algorithmic patterns relate to specific data structures?
- Practicing coding problems to reinforce your understanding
- Staying updated with new programming paradigms and best practices
- Reflecting on past projects to identify areas for improvement
Understanding and applying these principles allows you to create modular, scalable, and easily maintainable code structures.
5. Design Patterns
Design patterns are like having a collection of pre-styled outfits in your closet. They provide tested solutions to common software design problems. Some essential design patterns include:
Familiarity with these patterns allows you to solve complex design problems efficiently and communicate solutions effectively with other developers.
Organizing Your Coder’s Closet
Now that we’ve identified the essential pieces of our Coder’s Closet, let’s discuss how to organize and maintain this mental model capsule wardrobe:
1. Categorize and Group
Just as you might organize your physical closet by type of clothing (shirts, pants, dresses), organize your mental models into categories. For example:
This categorization helps you quickly access the right mental model when faced with a specific type of problem.
2. Create Connections
In a capsule wardrobe, items are chosen for their ability to work well together. Similarly, look for connections between different mental models. For example:
Understanding these relationships enhances your ability to combine different concepts creatively to solve complex problems.
3. Regular Review and Update
Just as you might reassess your wardrobe each season, regularly review and update your mental models. This could involve:
This ongoing process ensures your Coder’s Closet remains relevant and effective.
4. Quality Over Quantity
Remember, the goal is not to memorize every possible algorithm or design pattern. Instead, focus on deeply understanding a core set of versatile concepts that can be adapted to various situations. It’s better to have a smaller number of well-understood mental models than a large collection of superficially known concepts.
Applying Your Coder’s Closet: A Practical Example
Let’s walk through an example of how you might use your Coder’s Closet to approach a coding problem. Consider the following problem statement:
Given an array of integers, find the contiguous subarray with the largest sum.
Here’s how you might “dress” this problem using items from your Coder’s Closet:
- Data Structure: The problem involves an array, so we’ll start with our understanding of array operations.
- Algorithmic Pattern: This problem can be solved using the “Sliding Window” pattern, which is efficient for finding subarrays that meet certain criteria.
- Time Complexity Consideration: We want to solve this in O(n) time to efficiently handle large arrays.
- Problem-Solving Strategy: We can use Kadane’s algorithm, which is a dynamic programming approach to this problem.
Now, let’s implement the solution:
def max_subarray_sum(arr):
max_sum = float('-inf')
current_sum = 0
for num in arr:
current_sum = max(num, current_sum + num)
max_sum = max(max_sum, current_sum)
return max_sum
# Example usage
arr = [-2, 1, -3, 4, -1, 2, 1, -5, 4]
result = max_subarray_sum(arr)
print(f"The maximum subarray sum is: {result}") # Output: The maximum subarray sum is: 6
In this solution, we’ve combined several concepts from our Coder’s Closet:
- We’re using our understanding of array traversal.
- We’re applying the sliding window concept by maintaining a “window” (current_sum) that expands and contracts as we move through the array.
- We’re using a dynamic programming approach by building our solution based on previous computations.
- The solution runs in O(n) time, meeting our efficiency requirements.
By drawing on these organized mental models, we were able to quickly identify an efficient approach to the problem and implement a clean solution.
Expanding Your Coder’s Closet
As you grow as a programmer, you’ll naturally want to expand your Coder’s Closet. Here are some ways to add new “pieces” to your collection:
1. Explore New Programming Paradigms
While object-oriented programming is a staple, consider exploring other paradigms like functional programming or reactive programming. These can add new dimensions to your problem-solving toolkit.
2. Learn Domain-Specific Models
Depending on your area of focus, you might want to add domain-specific models to your closet. For example:
- Web development: Understanding client-server architecture, RESTful API design, and front-end frameworks
- Data science: Familiarity with statistical models, machine learning algorithms, and data visualization techniques
- Systems programming: Knowledge of memory management, concurrency models, and network protocols
3. Stay Updated with New Technologies
The tech world is constantly evolving. Make it a habit to stay informed about new languages, frameworks, and tools. While you don’t need to master every new technology, having a high-level understanding can help you make informed decisions about when to incorporate new elements into your Coder’s Closet.
4. Practice, Practice, Practice
The best way to reinforce and expand your mental models is through consistent practice. Engage in coding challenges, contribute to open-source projects, or work on personal projects that push you out of your comfort zone.
The Benefits of a Well-Organized Coder’s Closet
Organizing your mental models like a capsule wardrobe offers numerous benefits:
1. Efficient Problem-Solving
With a well-organized set of mental models, you can quickly identify patterns in new problems and apply appropriate solutions. This efficiency is particularly valuable in coding interviews or when facing tight project deadlines.
2. Improved Learning and Retention
By focusing on a core set of versatile concepts and understanding their interconnections, you’re more likely to retain and deepen your knowledge over time. This approach aligns with cognitive science principles of effective learning and memory formation.
3. Enhanced Communication
A structured mental model allows you to communicate your thoughts and solutions more clearly to others. This is invaluable when collaborating with team members, mentoring junior developers, or explaining your approach in technical interviews.
4. Adaptability
Just as a capsule wardrobe allows you to mix and match pieces for different occasions, a well-organized set of mental models enables you to adapt to various programming challenges and environments. This flexibility is crucial in the ever-changing landscape of technology.
5. Confidence and Reduced Cognitive Load
Knowing that you have a reliable set of tools at your disposal can boost your confidence when approaching new problems. Additionally, having an organized mental framework reduces cognitive load, allowing you to focus more energy on the unique aspects of each challenge rather than constantly searching for basic concepts.
Conclusion: Curating Your Unique Coder’s Closet
The concept of organizing your mental models like a capsule wardrobe provides a powerful framework for developing and maintaining your programming skills. By curating a collection of essential, versatile concepts and understanding how they interconnect, you create a robust toolkit for tackling a wide range of coding challenges.
Remember, your Coder’s Closet is personal. While there are fundamental pieces that every programmer should have, the specific composition of your mental model wardrobe will depend on your interests, specializations, and the types of problems you most often encounter. The key is to focus on quality over quantity, ensuring that each “piece” in your collection is well-understood and can be applied flexibly.
As you continue your journey as a programmer, regularly assess and update your Coder’s Closet. Be open to adding new concepts, refining your understanding of existing ones, and occasionally “decluttering” by setting aside models that no longer serve you well. With a well-maintained Coder’s Closet, you’ll be prepared to face coding challenges with confidence, efficiency, and style.
So, take some time to organize your mental models, create connections between different concepts, and practice applying them to various problems. Your future self will thank you for the well-organized, versatile Coder’s Closet you’ve created!