The Code Skeleton: Why You Should Outline Your Solution Before Filling in the Details
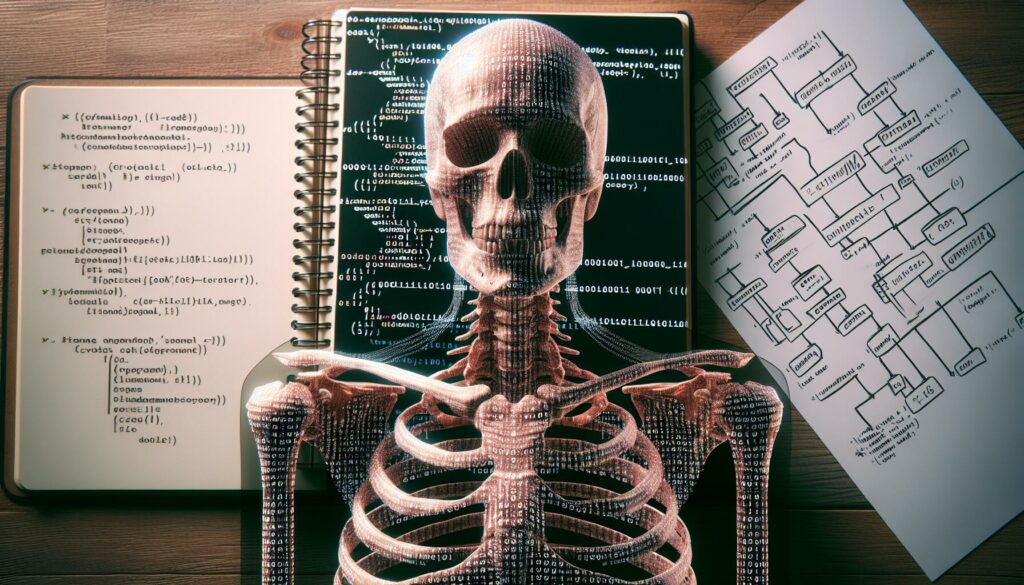
In the world of coding and software development, there’s a common saying: “Measure twice, cut once.” This carpentry wisdom applies just as well to writing code. Before diving into the nitty-gritty details of implementation, taking the time to outline your solution can save you countless hours of debugging, refactoring, and headaches. This practice, often referred to as creating a “code skeleton,” is a crucial step in the problem-solving process that many beginners overlook but seasoned developers swear by.
What is a Code Skeleton?
A code skeleton, also known as a program outline or pseudocode, is a high-level description of your solution to a coding problem. It’s not actual code, but rather a blueprint that outlines the structure and logic of your program without getting bogged down in syntax details. Think of it as the architectural plans for a house – you wouldn’t start building without a clear idea of the layout, would you?
Components of a Code Skeleton
- Main functions or methods
- Key variables and data structures
- Control flow (loops, conditionals)
- Basic algorithm steps
- Input and output expectations
The Benefits of Creating a Code Skeleton
1. Clarifies Your Thinking
When you outline your solution before coding, you’re forced to think through the problem systematically. This process helps you identify potential issues, edge cases, and logical flaws before you’ve written a single line of code. It’s much easier to spot and fix problems at this stage than when you’re knee-deep in implementation details.
2. Improves Problem-Solving Skills
Creating a code skeleton exercises your problem-solving muscles. It encourages you to break down complex problems into smaller, manageable parts. This skill is invaluable not just in coding, but in all aspects of life and work.
3. Saves Time in the Long Run
While it might seem like an extra step, outlining your solution actually saves time overall. By having a clear roadmap, you’re less likely to get lost in the weeds or write code that you’ll need to completely overhaul later. This is especially true for larger, more complex projects.
4. Facilitates Collaboration
If you’re working on a team, a code skeleton can serve as a communication tool. It allows you to share your approach with colleagues and get feedback before you’ve invested time in writing actual code. This can lead to better designs and fewer misunderstandings.
5. Enhances Code Quality
With a well-thought-out skeleton, your final code is likely to be more organized, modular, and easier to maintain. You’re essentially creating a structure that guides your coding process, leading to cleaner, more efficient code.
How to Create an Effective Code Skeleton
Step 1: Understand the Problem
Before you can outline a solution, you need to fully grasp the problem at hand. Read the problem statement carefully, identify the inputs and expected outputs, and clarify any ambiguities.
Step 2: Break Down the Problem
Divide the problem into smaller, manageable sub-problems. This is where you start to see the main components of your solution taking shape.
Step 3: Outline the Main Functions
Identify the key functions or methods your solution will need. Don’t worry about the implementation details yet – focus on what each function should accomplish.
Step 4: Sketch Out the Algorithm
Write out the basic steps of your algorithm in plain language or pseudocode. This is where you’ll outline your approach to solving the problem.
Step 5: Consider Edge Cases
Think about potential edge cases or unusual inputs that your solution needs to handle. Incorporating these considerations early can save you debugging time later.
Step 6: Review and Refine
Look over your skeleton and see if it makes logical sense. Are there any steps you’ve missed? Can anything be simplified or optimized?
Code Skeleton Example
Let’s look at an example of how you might create a code skeleton for a common coding problem: reversing a string.
// Function to reverse a string
function reverseString(input):
// Check if input is valid
// Initialize empty result string
// Loop through input string from end to beginning
// Add each character to result string
// Return result string
// Main function
function main():
// Get input string from user
// Call reverseString function
// Print result
This skeleton outlines the main functions, the basic algorithm steps, and considers input validation. It provides a clear structure for the solution without getting into the specifics of any particular programming language.
Common Mistakes to Avoid
1. Getting Too Detailed
Remember, a code skeleton is meant to be a high-level outline. Don’t get bogged down in language-specific syntax or intricate implementation details at this stage.
2. Skipping Edge Cases
While you don’t need to solve for every edge case in your skeleton, it’s important to at least note them. This will help you remember to address them when you start coding.
3. Ignoring Time and Space Complexity
Even at the skeleton stage, it’s good to have a general idea of the time and space complexity of your solution. This can help you identify potential efficiency issues early on.
4. Not Considering Alternative Approaches
Don’t settle for your first idea. Consider multiple approaches and outline the pros and cons of each before deciding on your final solution.
5. Rushing Through the Process
Creating a code skeleton might feel like it’s slowing you down, especially when you’re eager to start coding. Resist the urge to rush. The time invested here will pay off in the coding phase.
Code Skeletons in Technical Interviews
If you’re preparing for technical interviews, especially for major tech companies like those in FAANG (Facebook, Amazon, Apple, Netflix, Google), mastering the art of creating code skeletons can give you a significant advantage.
Benefits in Interview Settings
- Demonstrates Systematic Thinking: Interviewers want to see how you approach problems. By starting with a skeleton, you show that you can think through a problem methodically.
- Facilitates Communication: A code skeleton allows you to explain your approach clearly to the interviewer before diving into implementation. This can help you get early feedback and guidance.
- Manages Time Effectively: In the high-pressure environment of an interview, a skeleton can help you stay on track and use your limited time wisely.
- Shows Attention to Detail: By considering edge cases and potential issues in your skeleton, you demonstrate thoroughness and foresight.
- Reduces Stress: Having a clear plan can help calm your nerves and give you confidence as you tackle the problem.
How to Use Code Skeletons in Interviews
- Start by Clarifying the Problem: Ask questions to ensure you fully understand the requirements.
- Verbalize Your Thought Process: As you create your skeleton, explain your thinking to the interviewer. This gives them insight into your problem-solving approach.
- Use the Whiteboard: If given a whiteboard, use it to sketch out your skeleton. This visual aid can help both you and the interviewer.
- Be Open to Feedback: If the interviewer suggests modifications to your approach, be ready to adapt your skeleton accordingly.
- Transition Smoothly to Coding: Once you and the interviewer are satisfied with the skeleton, use it as a guide for writing your actual code.
Tools and Techniques for Creating Code Skeletons
While code skeletons can be created with just pen and paper (or a whiteboard in interview settings), there are various tools and techniques that can enhance this process, especially for more complex problems or larger projects.
1. Flowcharts
Flowcharts are visual representations of your algorithm’s logic flow. They can be particularly useful for visualizing complex decision trees or process flows.
Tools for Creating Flowcharts:
- Lucidchart
- Draw.io
- Microsoft Visio
2. UML Diagrams
Unified Modeling Language (UML) diagrams are great for outlining the structure of object-oriented programs. They can help you visualize classes, methods, and their relationships.
UML Diagram Tools:
- PlantUML
- StarUML
- Visual Paradigm
3. Mind Mapping Software
Mind maps can be an excellent way to brainstorm and organize the different components of your solution.
Mind Mapping Tools:
- MindMeister
- XMind
- Coggle
4. Pseudocode Editors
While pseudocode can be written in any text editor, there are some tools designed specifically for writing and formatting pseudocode.
Pseudocode Tools:
- PseudoCode.io
- Code2flow
5. Version Control for Skeletons
For larger projects, you might want to keep track of different versions of your code skeleton as your ideas evolve. Version control systems like Git can be useful for this, even at the planning stage.
Incorporating Code Skeletons into Your Development Workflow
To truly benefit from code skeletons, it’s important to integrate them into your regular development process. Here are some tips for making code skeletons a natural part of your workflow:
1. Start with the Skeleton
Make it a habit to begin every new project or significant feature with a code skeleton. Resist the urge to jump straight into coding.
2. Use Comments in Your Code
Translate your code skeleton into comments in your actual code file. This creates a natural structure for your implementation and serves as a to-do list as you code.
3. Review and Refine
As you implement your solution, continually refer back to your skeleton. If you find that your implementation is deviating significantly from your original plan, take a step back and consider whether you need to update your skeleton or if you’re going off track.
4. Collaborate on Skeletons
If you’re working in a team, consider reviewing code skeletons together before implementation begins. This can catch potential issues early and ensure everyone is on the same page.
5. Keep Skeletons for Future Reference
Save your code skeletons along with your finished projects. They can be valuable references for similar problems in the future and can help you reflect on your problem-solving progress over time.
Code Skeletons and Algorithmic Thinking
Creating code skeletons is not just about organizing your thoughts – it’s a crucial exercise in algorithmic thinking. Algorithmic thinking is the ability to define clear steps to solve a problem or achieve a goal. This skill is at the heart of computer science and is highly valued in the tech industry.
How Code Skeletons Enhance Algorithmic Thinking
- Problem Decomposition: Breaking down a complex problem into smaller, manageable parts is a key aspect of algorithmic thinking. Code skeletons force you to do this systematically.
- Abstraction: By focusing on the high-level structure of your solution, you’re practicing abstraction – the ability to strip away unnecessary details and focus on the core of the problem.
- Pattern Recognition: As you create more code skeletons, you’ll start to recognize common patterns in problem-solving, which you can apply to new challenges.
- Logical Thinking: Outlining the steps of your algorithm in a skeleton helps develop your ability to think logically and sequentially.
- Efficiency Consideration: Even at the skeleton stage, you’re encouraged to think about the efficiency of your approach, which is a crucial aspect of algorithmic thinking.
Conclusion
Creating a code skeleton before diving into implementation is a powerful technique that can significantly improve your coding process and outcomes. It clarifies your thinking, improves problem-solving skills, saves time, facilitates collaboration, and ultimately leads to better code. Whether you’re a beginner learning to code, a student preparing for technical interviews, or an experienced developer tackling complex projects, the practice of outlining your solution can be a game-changer.
Remember, the goal of a code skeleton is not to have a perfect plan from the start, but to provide a solid foundation and direction for your coding efforts. It’s a flexible tool that evolves as you gain more insight into the problem. By making code skeletons a regular part of your development process, you’ll not only write better code but also strengthen your overall problem-solving and algorithmic thinking skills.
So, the next time you face a coding challenge, resist the urge to jump straight into writing code. Take a step back, grab a pen and paper (or open your favorite digital tool), and start by sketching out your code skeleton. Your future self, knee-deep in implementation, will thank you for the clarity and direction it provides.