The Bulletproof Formula for Solving Any Coding Question
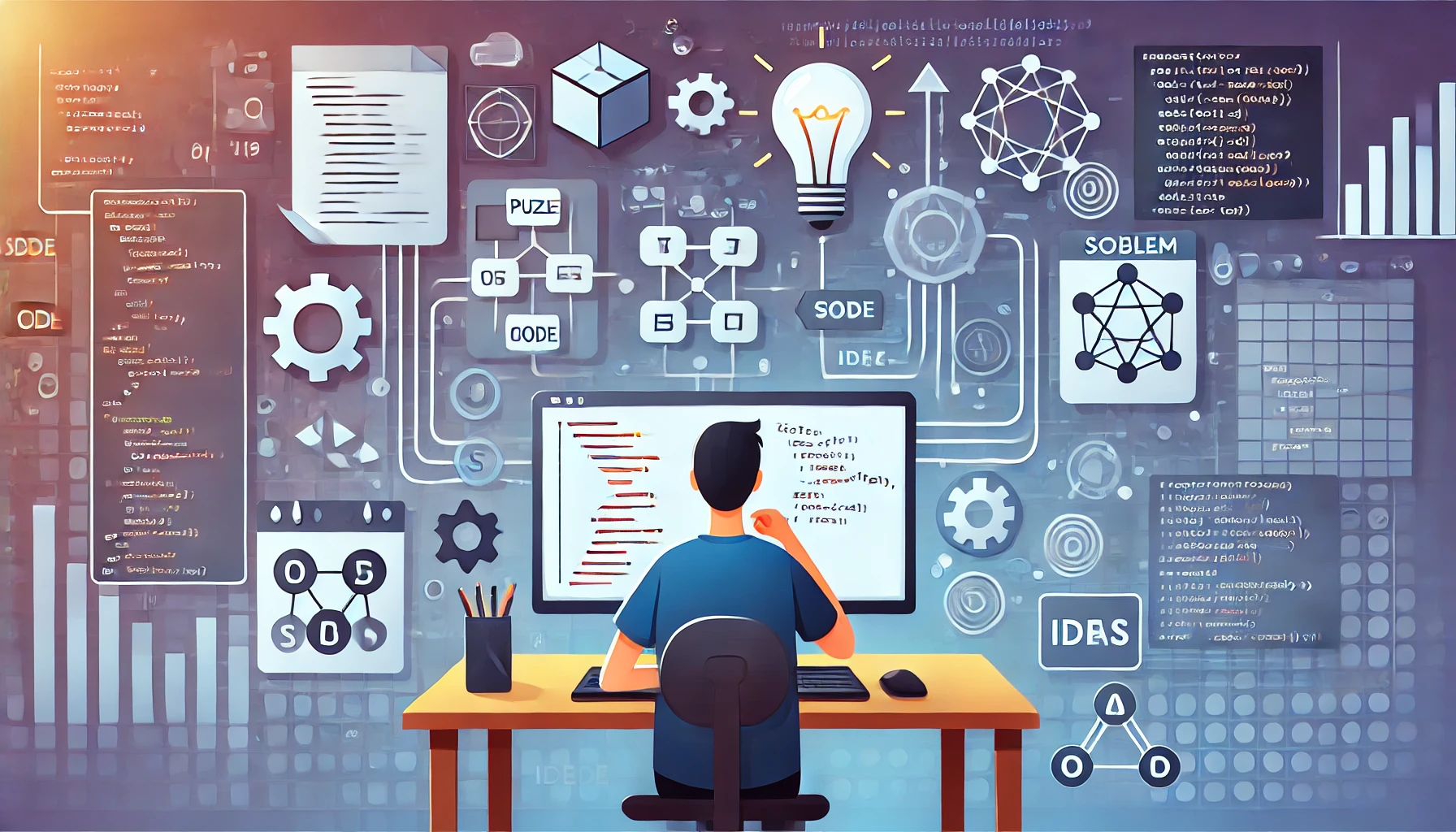
The Bulletproof Formula for Solving Any Coding Question
As aspiring programmers and software engineers, we’ve all been there. You open up a coding question, determined to solve it on your own. You put in time and effort, but eventually hit a wall. Frustrated, you turn to online tutorials or video explanations, only to be left wondering: “How on earth did they come up with that solution? I could never think of that in an interview. You’d have to be a genius!”
If this sounds familiar, you’re not alone. The good news is, there’s a better way. In this post, I’ll share a bulletproof formula for approaching and solving even the toughest coding questions. This method helped me go from feeling stuck and inadequate to confidently tackling complex problems in under 10 minutes. Let’s dive in!
The Problem with Traditional Approaches
When I first started learning to code, I often felt lost when faced with challenging problems. I’d try my best, but frequently ended up resorting to looking up solutions online. While I could usually understand the explanations, I didn’t feel like I was truly becoming a better problem solver. It was like reading Shakespeare – beautiful, but not something I could produce on my own.
The root of the issue was that most online resources and tutorials jump straight to the optimal solution. They present clever algorithms that seem to materialize out of thin air, leaving learners feeling inadequate and unprepared for real-world coding interviews.
What I needed was a clear, step-by-step process for starting from scratch and gradually building up to those elegant solutions. After much trial and error, I developed a three-pillar approach that has revolutionized my problem-solving abilities. Let me introduce you to the Bulletproof Formula.
The Bulletproof Formula: Three Pillars for Success
Pillar 1: Start with Brute Force
The first and most crucial step is to always begin by thinking about a brute force approach. Many students underestimate the power of this technique, assuming it’s not worth the effort to consider a “stupid” slow solution. This couldn’t be further from the truth!
Why Brute Force Matters:
- A slow solution is infinitely better than no solution at all. In fact, I have a friend who passed a coding interview by providing a brute force solution when no other candidates could solve the problem optimally.
- Brute force approaches are often much closer to the optimal solution than you might think. By identifying the bottlenecks in your initial approach, you can often find straightforward optimizations using data structures or techniques you already know.
How to Develop a Brute Force Solution:
- Consider all possible candidates for the solution.
- Try each option systematically until you find the correct one.
- Don’t worry about efficiency at this stage – focus on correctness.
Let’s look at a classic example: the “Two Sum” problem. Given an array of integers and a target sum, you need to find a pair of numbers in the array that add up to the target.
A brute force approach might look like this:
def two_sum_brute_force(nums, target):
for i in range(len(nums)):
for j in range(i+1, len(nums)):
if nums[i] + nums[j] == target:
return [i, j]
return []
This solution checks every possible pair of numbers, which is inefficient but correct. From here, we can identify the bottleneck (checking every pair) and optimize using a hash table for faster lookups.
Pillar 2: Simplify the Problem
When you’re stuck or don’t know where to start, try thinking about an easier version of the problem. This technique helps you build intuition and often leads to insights that solve the original question.
How to Simplify:
- Reduce the number of variables or constraints.
- Consider special cases or smaller input sizes.
- Gradually increase complexity as you gain understanding.
Let’s explore this approach with a more complex example:
Imagine you have a basket of fruits and need to arrange them in a line on a table, ensuring that no two adjacent fruits are the same. How would you approach this?
- Step 1: Start with the simplest case – one type of fruit.
- Step 2: Increase complexity – two types of fruits.
- Step 3: Three types of fruits.
By working through these simplified versions, you build intuition about the problem. You might notice that it’s often optimal to start with the fruit that appears most frequently, as this gives you more options later on.
Pillar 3: Solve Small Examples on Paper
The final pillar involves taking numerous small examples and solving them manually on paper. This hands-on approach helps you:
- Identify patterns and make observations.
- Test and refine your hypotheses.
- Build confidence in your solution before coding.
Continuing with our fruit arrangement problem:
Start with tiny examples, like 1 orange, 1 banana, 1 strawberry. Any arrangement works.
As you expand to larger examples, you’ll start noticing patterns, like starting with the most frequent fruit and alternating between others. This observation can lead to a valid solution for larger problems.
Putting It All Together
The real power of the Bulletproof Formula comes from combining these three pillars. Let’s walk through how you might use them together to solve our fruit arrangement problem:
- Brute Force Approach: Generate all possible permutations and check each one for validity.
- Simplify the Problem: Gradually build up from one type of fruit to multiple types, gathering insights.
- Solve Small Examples: Work through numerous small examples on paper to test your ideas.
Using these methods together can help you discover the optimal solution step by step.
Why This Formula Works
The Bulletproof Formula is effective because:
- It provides a structured approach to problem-solving.
- It builds intuition gradually.
- It mimics the thought process of experienced programmers.
- It’s flexible and can be applied to a wide range of coding problems.
Applying the Formula in Your Coding Journey
To get the most out of this approach:
- Practice regularly.
- Be patient with yourself.
- Embrace “obvious” solutions.
- Combine pillars flexibly.
- Explain your thought process.
- Learn from each problem you solve.
Conclusion
The journey from feeling stuck to confidently tackling complex coding problems is achievable. The Bulletproof Formula gives you the tools to not only solve individual problems but to develop lasting problem-solving skills.
With practice and persistence, even the toughest coding challenges will become manageable. So next time you’re faced with a difficult coding question, take a deep breath, apply the Bulletproof Formula, and watch the problem unravel before your eyes.
Happy coding!