The Best Git Tutorials for Beginner Developers
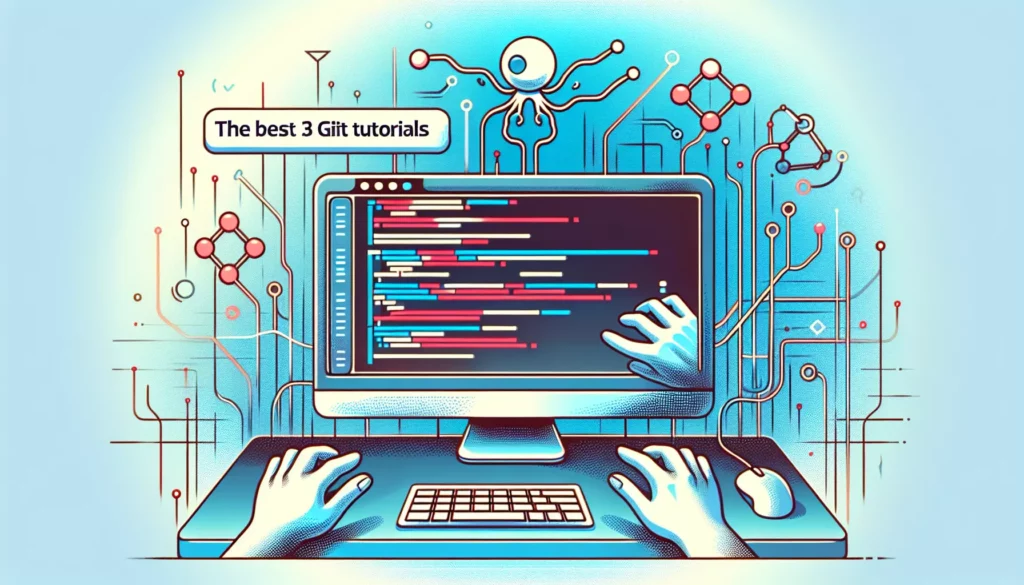
Are you a beginner developer looking to master the art of version control? Look no further! Git is an essential tool in every programmer’s toolkit, and learning it early in your coding journey can set you up for success. In this comprehensive guide, we’ll explore the best Git tutorials available for beginners, helping you navigate the sometimes confusing world of repositories, commits, and pull requests.
Why Learn Git?
Before we dive into the tutorials, let’s quickly recap why Git is so important for developers:
- Version Control: Git allows you to track changes in your code over time.
- Collaboration: It makes working on projects with other developers seamless.
- Backup: Your code is safely stored in remote repositories.
- Experimentation: Branches allow you to try new ideas without affecting the main codebase.
- Industry Standard: Git is used by most professional development teams.
Now that we’ve established the importance of Git, let’s explore the best tutorials to get you started.
1. Git-it (Desktop App)
Difficulty Level: Beginner
Format: Interactive Desktop Application
Cost: Free
Git-it is a desktop app that teaches you Git and GitHub through challenges. It’s an excellent starting point for absolute beginners because it combines theory with practical exercises.
Key Features:
- Step-by-step challenges
- Works offline after initial download
- Available for Windows, Mac, and Linux
- Teaches both Git and GitHub basics
To get started with Git-it, simply download the application from the official GitHub repository and follow the instructions. The app will guide you through setting up Git, creating repositories, making commits, and even contributing to open-source projects.
2. Codecademy’s Learn Git Course
Difficulty Level: Beginner to Intermediate
Format: Interactive Online Course
Cost: Free (Basic), Paid (Pro features)
Codecademy is known for its interactive coding courses, and their Git course is no exception. This tutorial is perfect for visual learners who prefer a hands-on approach.
Course Contents:
- Basic Git Workflow
- How to Backtrack in Git
- Git Branching
- Git Teamwork
The course is structured to take you from a complete beginner to being comfortable with Git’s core concepts. You’ll be writing Git commands directly in your browser, which helps reinforce learning through practice.
Visit the Codecademy Git Course to get started.
3. Git and GitHub for Beginners – Crash Course (YouTube)
Difficulty Level: Beginner
Format: Video Tutorial
Cost: Free
If you prefer video tutorials, this crash course by freeCodeCamp.org on YouTube is an excellent resource. In just over an hour, you’ll get a comprehensive introduction to Git and GitHub.
Video Contents:
- What is Git?
- Installing Git
- Git commands (init, add, commit, push, pull)
- Working with GitHub
- Branching and merging
The video is well-paced and includes practical examples, making it easy to follow along. You can find the video on the freeCodeCamp YouTube channel.
4. Git Handbook by GitHub Guides
Difficulty Level: Beginner to Intermediate
Format: Written Guide
Cost: Free
For those who prefer reading and self-paced learning, the Git Handbook by GitHub Guides is an excellent resource. It’s a comprehensive yet concise guide that covers all the essential Git concepts.
Guide Contents:
- Version Control basics
- Git fundamentals
- GitHub flow
- Advanced Git concepts
The handbook is well-structured and includes diagrams to help visualize Git workflows. It’s a great reference to keep handy as you progress in your Git journey.
Access the Git Handbook on GitHub Guides.
5. Learn Git Branching
Difficulty Level: Beginner to Advanced
Format: Interactive Web Application
Cost: Free
Learn Git Branching is a unique, interactive way to learn Git. It’s a web-based application that visualizes Git commands and their effects on your repository structure.
Key Features:
- Visual representation of Git commands
- Covers basic to advanced Git concepts
- Includes challenging scenarios
- Available in multiple languages
This tutorial is particularly useful for understanding branching and merging, which can be some of the more confusing aspects of Git for beginners. The visual nature of the tutorial makes these concepts much clearer.
Start learning at the Learn Git Branching website.
6. Pro Git Book
Difficulty Level: Beginner to Advanced
Format: E-book
Cost: Free
For those who prefer a more traditional learning approach, the Pro Git book is an excellent resource. Written by Scott Chacon and Ben Straub, this book is available for free online and covers everything from Git basics to advanced topics.
Book Contents:
- Getting Started
- Git Basics
- Git Branching
- Git on the Server
- Distributed Git
- GitHub
- Git Tools
- Customizing Git
The book is continuously updated and is available in multiple formats and languages. It’s an invaluable resource that you can refer back to as you progress in your Git skills.
Read the Pro Git book online or download it for free.
7. Git Immersion
Difficulty Level: Beginner to Intermediate
Format: Guided Tour
Cost: Free
Git Immersion offers a guided tour that walks you through the fundamentals of Git. It’s designed as a series of hands-on labs to give you a practical understanding of Git.
Tutorial Structure:
- 53 progressive labs
- Covers setup to advanced usage
- Includes real-world scenarios
What sets Git Immersion apart is its focus on learning by doing. Each lab includes clear instructions and explanations, ensuring you understand not just how to use Git commands, but why you’re using them.
Begin your immersive Git journey at the Git Immersion website.
Practical Tips for Learning Git
As you work through these tutorials, keep the following tips in mind to maximize your learning:
- Practice Regularly: Git is a skill that improves with consistent use. Try to incorporate Git into your daily coding routine.
- Start Small: Begin with simple projects and gradually increase complexity as you become more comfortable with Git commands.
- Use the Command Line: While GUI tools for Git exist, learning Git through the command line will give you a deeper understanding of how it works.
- Experiment in a Safe Environment: Create test repositories where you can freely experiment with different Git commands without fear of breaking anything important.
- Collaborate with Others: Once you’re comfortable with the basics, try collaborating on a project with friends or contributing to open-source projects to practice Git in a team setting.
- Read Documentation: Get comfortable reading Git’s official documentation. It’s an excellent resource for understanding commands in-depth.
- Visualize Git Workflow: Use tools like GitKraken or SourceTree to visualize your Git workflow, which can help reinforce your understanding of branching and merging.
Common Git Commands for Beginners
As you start your Git journey, here are some essential commands you’ll encounter frequently:
git init # Initialize a new Git repository
git clone <url> # Clone a repository from a remote source
git add <file> # Add a file to the staging area
git commit -m "message" # Commit changes with a descriptive message
git push # Push commits to a remote repository
git pull # Fetch and merge changes from a remote repository
git branch # List, create, or delete branches
git merge <branch> # Merge changes from one branch into the current branch
git status # Check the status of your working directory
git log # View commit history
Remember, these commands are just the tip of the iceberg. As you progress, you’ll learn more advanced Git commands and techniques.
Troubleshooting Common Git Issues
Even experienced developers sometimes run into issues with Git. Here are some common problems you might encounter and how to solve them:
1. Merge Conflicts
Merge conflicts occur when Git can’t automatically resolve differences in code between two commits.
Solution: Open the conflicting files, manually resolve the conflicts, then commit the changes.
git status # Identify conflicting files
# Manually edit files to resolve conflicts
git add <resolved-file>
git commit -m "Resolved merge conflict"
2. Accidentally Committing to the Wrong Branch
Solution: Use git cherry-pick to move the commit to the correct branch.
git checkout <correct-branch>
git cherry-pick <commit-hash>
git checkout <wrong-branch>
git reset --hard HEAD~1
3. Undoing the Last Commit
Solution: Use git reset to undo the last commit.
git reset HEAD~1 # Undo commit but keep changes
# OR
git reset --hard HEAD~1 # Undo commit and discard changes
4. Forgot to Add a File to the Last Commit
Solution: Use git commit –amend to modify the last commit.
git add <forgotten-file>
git commit --amend --no-edit
Git Best Practices
As you become more proficient with Git, consider adopting these best practices:
- Write Clear Commit Messages: Use descriptive, concise messages that explain what changes were made and why.
- Commit Often: Make small, frequent commits rather than large, infrequent ones. This makes it easier to track changes and revert if necessary.
- Use Branches: Create new branches for features or experiments to keep your main branch stable.
- Pull Before You Push: Always pull the latest changes from the remote repository before pushing your own changes to avoid conflicts.
- Use .gitignore: Create a .gitignore file to exclude unnecessary files (like build artifacts or sensitive information) from your repository.
- Review Changes Before Committing: Use git diff to review your changes before staging and committing them.
- Keep Your Repository Clean: Regularly delete merged branches and use git clean to remove untracked files when necessary.
Conclusion
Mastering Git is an essential skill for any developer, and these tutorials provide excellent starting points for beginners. Remember that learning Git is a journey, and proficiency comes with practice and real-world application.
As you progress in your coding journey, you’ll find that Git becomes an indispensable tool in your development workflow. It not only helps you manage your code more effectively but also enables seamless collaboration with other developers.
Don’t be discouraged if you find some concepts challenging at first. Git has a learning curve, but with patience and practice, you’ll soon be navigating repositories, branching, and merging with confidence.
Keep exploring, keep practicing, and most importantly, don’t be afraid to experiment. Git’s ability to track and revert changes means you can always go back if something doesn’t work out. Happy coding, and may your commits always be clear and your merges conflict-free!