The Benefits of Teaching Others What You’ve Learned in Coding
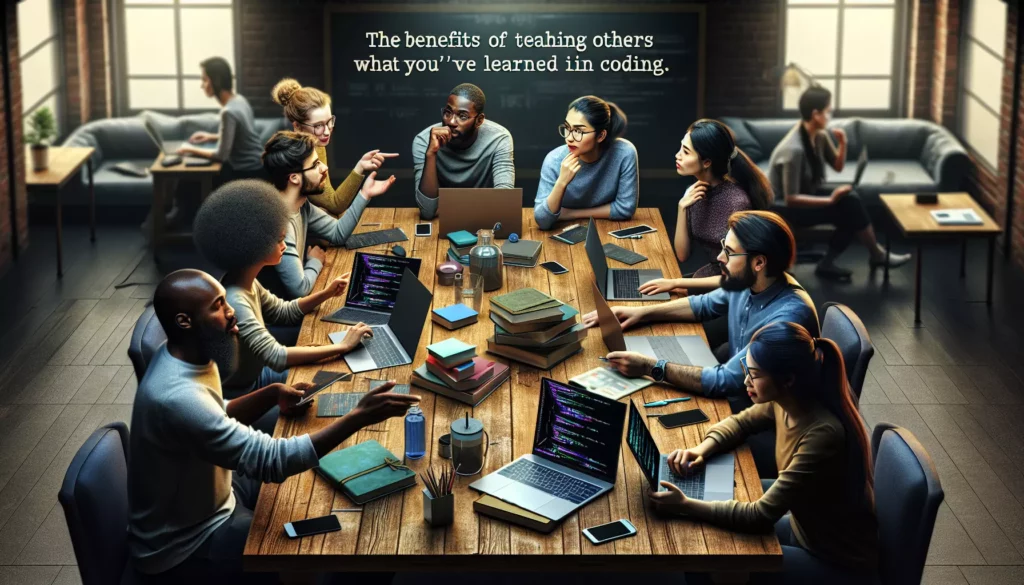
In the ever-evolving world of technology and programming, continuous learning is not just a luxury—it’s a necessity. As you progress in your coding journey, you’ll find that one of the most effective ways to solidify your knowledge and accelerate your growth is by teaching others. This practice, often referred to as the “protégé effect” or “learning by teaching,” has been proven to be a powerful tool for both the teacher and the student. In this article, we’ll explore the numerous benefits of teaching others what you’ve learned in coding, and how it can significantly enhance your own skills and career prospects.
The Power of Knowledge Sharing in Coding
Before we dive into the specific benefits, it’s important to understand why teaching is particularly valuable in the field of coding. Programming is a complex discipline that requires not only technical knowledge but also problem-solving skills, logical thinking, and creativity. When you teach coding concepts to others, you’re not just reciting facts—you’re explaining processes, demonstrating problem-solving techniques, and often coming up with new ways to illustrate complex ideas.
This process of explanation and demonstration forces you to engage with the material on a deeper level, often leading to new insights and a more comprehensive understanding of the subject matter. Now, let’s explore the specific benefits that come from teaching others what you’ve learned in coding.
1. Reinforcing Your Own Understanding
One of the most immediate benefits of teaching coding is the reinforcement of your own knowledge. When you explain a concept to someone else, you need to break it down into its fundamental components and present it in a clear, logical manner. This process requires you to thoroughly understand the topic yourself.
For example, let’s say you’re teaching someone about sorting algorithms. You might start with a simple bubble sort:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
As you explain how this algorithm works, you’ll need to articulate the step-by-step process, discuss its time complexity, and perhaps compare it to other sorting algorithms. This exercise not only reinforces your understanding of bubble sort but also encourages you to think about its practical applications and limitations.
2. Uncovering Knowledge Gaps
Teaching others often reveals gaps in your own knowledge. When a student asks a question you can’t answer or challenges your explanation, it highlights areas where your understanding might be incomplete. These moments are invaluable opportunities for growth.
For instance, while teaching about object-oriented programming, a student might ask about the difference between composition and inheritance. If you find yourself struggling to provide a clear explanation, it’s a sign that you need to deepen your understanding of these concepts.
class Engine:
def start(self):
print("Engine started")
class Car:
def __init__(self):
self.engine = Engine() # Composition
def start(self):
self.engine.start()
class ElectricCar(Car): # Inheritance
def charge(self):
print("Charging...")
This example demonstrates both composition (Car has an Engine) and inheritance (ElectricCar inherits from Car). Explaining the nuances between these two concepts might lead you to explore more advanced OOP principles, ultimately enhancing your own expertise.
3. Improving Communication Skills
Effective communication is crucial in the tech industry, whether you’re explaining your code to team members, presenting ideas to stakeholders, or writing documentation. Teaching coding provides an excellent opportunity to hone these skills.
When you teach, you learn to:
- Explain complex concepts in simple terms
- Adapt your communication style to different learning types
- Use analogies and real-world examples to illustrate abstract ideas
- Respond to questions and provide constructive feedback
These skills are invaluable in a professional setting. For example, being able to clearly explain the benefits and implementation of a new feature to both technical and non-technical team members can significantly impact your effectiveness as a developer.
4. Staying Up-to-Date with Technology
The field of programming is constantly evolving, with new languages, frameworks, and best practices emerging regularly. Teaching others motivates you to stay current with these developments.
For instance, if you’re teaching web development, you’ll need to keep up with the latest updates in popular frameworks like React or Vue.js. This might lead you to explore new features like React Hooks:
import React, { useState, useEffect } from 'react';
function Example() {
const [count, setCount] = useState(0);
useEffect(() => {
document.title = `You clicked ${count} times`;
});
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
By teaching this concept, you’ll not only help your students understand modern React development but also deepen your own understanding of state management and side effects in functional components.
5. Building a Professional Network
Teaching coding can significantly expand your professional network. Whether you’re mentoring junior developers at work, contributing to open-source projects, or creating online tutorials, you’re likely to connect with a diverse group of individuals in the tech community.
These connections can lead to:
- Collaboration opportunities on interesting projects
- Job referrals or recommendations
- Exposure to different perspectives and problem-solving approaches
- A support network for your own learning and career development
For example, you might start by answering questions on Stack Overflow or contributing to documentation for an open-source project. These activities not only help others but also establish your reputation as a knowledgeable and helpful member of the coding community.
6. Enhancing Your Resume and Career Prospects
Teaching experience can be a valuable addition to your resume, setting you apart from other candidates in job applications. It demonstrates not only your technical expertise but also soft skills such as communication, leadership, and the ability to explain complex concepts.
Many tech companies value employees who can mentor junior developers or contribute to internal knowledge sharing. For instance, you might highlight your teaching experience in a job interview by discussing how you’ve led coding workshops or created internal documentation for your team:
// Example of well-documented code you might showcase
/**
* Fetches user data from the API.
* @param {number} userId - The ID of the user to fetch.
* @returns {Promise<Object>} A promise that resolves with the user data.
* @throws {Error} If the API request fails.
*/
async function fetchUserData(userId) {
try {
const response = await fetch(`https://api.example.com/users/${userId}`);
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
const userData = await response.json();
return userData;
} catch (error) {
console.error("Failed to fetch user data:", error);
throw error;
}
}
This example demonstrates not only your coding skills but also your ability to write clear, informative documentation—a skill often honed through teaching.
7. Gaining New Perspectives
Teaching exposes you to diverse viewpoints and problem-solving approaches. Students often ask questions or propose solutions that you might not have considered, leading to new insights and alternative ways of thinking about coding problems.
For example, while teaching algorithms, a student might suggest an unconventional approach to solving a classic problem like finding the longest palindromic substring:
def longest_palindrome(s):
if not s:
return ""
def expand_around_center(left, right):
while left >= 0 and right < len(s) and s[left] == s[right]:
left -= 1
right += 1
return s[left + 1:right]
result = ""
for i in range(len(s)):
# Odd length palindromes
palindrome1 = expand_around_center(i, i)
# Even length palindromes
palindrome2 = expand_around_center(i, i + 1)
result = max(result, palindrome1, palindrome2, key=len)
return result
This approach of expanding around the center might be different from the dynamic programming solution you initially had in mind. Such interactions can broaden your problem-solving toolkit and make you a more versatile programmer.
8. Developing Leadership Skills
Teaching coding inherently involves taking on a leadership role. You’re guiding others, setting the pace of learning, and often making decisions about what to cover and how to present it. These experiences can translate directly into leadership skills valuable in any professional setting.
For instance, you might find yourself leading a study group working on a complex project:
// Project: Building a simple task management system
// Task class
class Task {
constructor(id, description, status = 'pending') {
this.id = id;
this.description = description;
this.status = status;
}
}
// TaskManager class
class TaskManager {
constructor() {
this.tasks = [];
}
addTask(description) {
const id = this.tasks.length + 1;
const newTask = new Task(id, description);
this.tasks.push(newTask);
return newTask;
}
updateTaskStatus(id, newStatus) {
const task = this.tasks.find(t => t.id === id);
if (task) {
task.status = newStatus;
return true;
}
return false;
}
getTasks() {
return this.tasks;
}
}
// Usage
const manager = new TaskManager();
manager.addTask("Complete project proposal");
manager.addTask("Review code");
console.log(manager.getTasks());
manager.updateTaskStatus(1, 'completed');
console.log(manager.getTasks());
In guiding your group through this project, you’ll practice skills like delegating tasks, providing constructive feedback, and ensuring everyone understands the overall structure and goals of the project. These are all valuable leadership skills that can benefit your career in the long run.
9. Boosting Confidence and Job Satisfaction
Successfully teaching others can significantly boost your confidence in your own abilities. As you see your students grasp concepts and grow their skills, you’ll gain a sense of accomplishment and validation of your own expertise.
This increased confidence can have a positive impact on your own work. You might find yourself more willing to take on challenging projects or speak up in team meetings to propose innovative solutions.
Moreover, many developers find teaching to be intrinsically rewarding. The satisfaction of helping others learn and grow can greatly enhance your overall job satisfaction and motivation in your coding career.
10. Cultivating a Growth Mindset
Teaching naturally fosters a growth mindset—the belief that abilities can be developed through dedication and hard work. As you guide others through their learning journey, you’re constantly reminded that skills are not fixed but can be improved with practice and perseverance.
This mindset is crucial in the fast-paced world of technology. It helps you approach new challenges with enthusiasm rather than apprehension. For example, when faced with a new technology stack at work, instead of feeling overwhelmed, you might approach it as an exciting learning opportunity:
// Learning a new framework: Vue.js
// Vue component
Vue.component('task-list', {
data() {
return {
tasks: [
{ id: 1, text: 'Learn Vue.js', done: false },
{ id: 2, text: 'Build a Vue app', done: false }
]
}
},
template: `
<ul>
<li v-for="task in tasks" :key="task.id">
{{ task.text }}
<input type="checkbox" v-model="task.done">
</li>
</ul>
`
})
// Vue instance
new Vue({
el: '#app'
})
By approaching new technologies with a growth mindset, you’re more likely to persist through initial difficulties and achieve mastery.
Conclusion: The Reciprocal Nature of Teaching and Learning in Coding
Teaching what you’ve learned in coding is a powerful way to enhance your own skills while contributing to the growth of others. It reinforces your knowledge, uncovers areas for improvement, hones your communication skills, and keeps you up-to-date with the latest developments in technology. Moreover, it expands your professional network, boosts your career prospects, and cultivates a growth mindset essential for long-term success in the tech industry.
Remember, you don’t need to be an expert to start teaching. Even explaining a basic concept to a peer or writing a blog post about something you’ve recently learned can be a valuable teaching experience. As you progress in your coding journey, consider incorporating teaching into your routine—whether it’s through mentoring, creating online content, or contributing to open-source documentation.
By embracing the role of both learner and teacher, you’ll not only accelerate your own growth but also play a vital part in nurturing the next generation of developers. In the collaborative and rapidly evolving field of coding, the ability to learn from others and pass on your knowledge is not just beneficial—it’s essential for collective progress and innovation.
So, the next time you master a new coding concept or solve a challenging problem, consider taking a moment to share your knowledge. You might be surprised at how much you learn in the process.