The Benefits of Cross-Disciplinary Learning in Technology
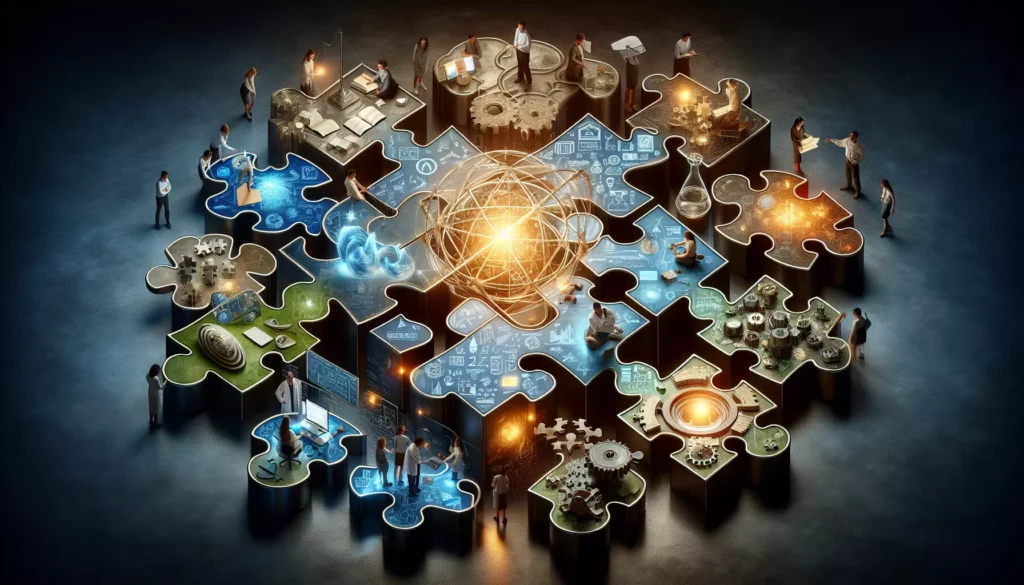
In today’s rapidly evolving technological landscape, the ability to think across disciplines and apply knowledge from various fields has become increasingly valuable. Cross-disciplinary learning in technology not only broadens one’s skill set but also fosters innovation and problem-solving capabilities. This approach is particularly relevant in the context of coding education and programming skills development, which are at the core of platforms like AlgoCademy. In this article, we’ll explore the numerous benefits of cross-disciplinary learning in technology and how it can enhance your journey as a programmer or aspiring tech professional.
Understanding Cross-Disciplinary Learning
Cross-disciplinary learning refers to the practice of combining knowledge and methodologies from different academic disciplines or professional fields. In the context of technology and programming, this could mean integrating concepts from areas such as:
- Computer Science
- Mathematics
- Physics
- Biology
- Psychology
- Design
- Business
- and more
By embracing a cross-disciplinary approach, learners can develop a more holistic understanding of technology and its applications, leading to numerous benefits in their personal and professional development.
Enhanced Problem-Solving Skills
One of the primary advantages of cross-disciplinary learning in technology is the significant improvement in problem-solving skills. When you’re exposed to diverse fields of study, you gain access to a wider array of problem-solving techniques and perspectives. This expanded toolkit allows you to approach coding challenges and technical issues from multiple angles, increasing your chances of finding innovative solutions.
For instance, understanding principles from physics can help you develop more efficient algorithms for simulations or game development. Similarly, knowledge of psychology can enhance your ability to create more intuitive user interfaces and improve user experience in software applications.
Example: Applying Mathematical Concepts to Algorithmic Thinking
Consider the classic problem of finding the shortest path between two points, a common challenge in algorithmic thinking. While this can be solved using purely programming techniques, understanding the mathematical concept of graph theory can lead to more elegant and efficient solutions. Here’s a simple implementation of Dijkstra’s algorithm in Python, which demonstrates this cross-disciplinary approach:
import heapq
def dijkstra(graph, start, end):
distances = {node: float('inf') for node in graph}
distances[start] = 0
pq = [(0, start)]
previous = {node: None for node in graph}
while pq:
current_distance, current_node = heapq.heappop(pq)
if current_node == end:
path = []
while current_node:
path.append(current_node)
current_node = previous[current_node]
return path[::-1], current_distance
if current_distance > distances[current_node]:
continue
for neighbor, weight in graph[current_node].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
previous[neighbor] = current_node
heapq.heappush(pq, (distance, neighbor))
return None, float('inf')
# Example usage
graph = {
'A': {'B': 4, 'C': 2},
'B': {'D': 3, 'E': 1},
'C': {'B': 1, 'D': 5},
'D': {'E': 2},
'E': {}
}
path, distance = dijkstra(graph, 'A', 'E')
print(f"Shortest path: {path}")
print(f"Distance: {distance}")
This example demonstrates how understanding graph theory from mathematics can lead to efficient algorithmic solutions in programming, showcasing the power of cross-disciplinary learning.
Increased Creativity and Innovation
Cross-disciplinary learning fosters creativity by encouraging you to make connections between seemingly unrelated concepts. This ability to draw parallels and apply ideas from one field to another is a cornerstone of innovation in technology. Many groundbreaking technological advancements have come from the intersection of different disciplines.
For example, the field of bioinformatics emerged from the combination of biology and computer science, leading to significant breakthroughs in genomics and personalized medicine. Similarly, the integration of psychology and computer science has given rise to the field of human-computer interaction, revolutionizing the way we design and interact with technology.
Case Study: Machine Learning and Neuroscience
The development of artificial neural networks, a fundamental concept in machine learning, was inspired by the structure and function of biological neural networks in the brain. This cross-disciplinary approach has led to significant advancements in artificial intelligence and deep learning. Here’s a simple example of how this interdisciplinary knowledge can be applied in practice:
import numpy as np
def sigmoid(x):
return 1 / (1 + np.exp(-x))
class NeuralNetwork:
def __init__(self, input_size, hidden_size, output_size):
self.W1 = np.random.randn(input_size, hidden_size)
self.W2 = np.random.randn(hidden_size, output_size)
def forward(self, X):
self.z1 = np.dot(X, self.W1)
self.a1 = sigmoid(self.z1)
self.z2 = np.dot(self.a1, self.W2)
self.a2 = sigmoid(self.z2)
return self.a2
def train(self, X, y, learning_rate, epochs):
for _ in range(epochs):
# Forward propagation
output = self.forward(X)
# Backpropagation
error = y - output
d_output = error * output * (1 - output)
error_hidden = np.dot(d_output, self.W2.T)
d_hidden = error_hidden * self.a1 * (1 - self.a1)
# Update weights
self.W2 += learning_rate * np.dot(self.a1.T, d_output)
self.W1 += learning_rate * np.dot(X.T, d_hidden)
# Example usage
nn = NeuralNetwork(2, 3, 1)
X = np.array([[0, 0], [0, 1], [1, 0], [1, 1]])
y = np.array([[0], [1], [1], [0]])
nn.train(X, y, learning_rate=0.1, epochs=10000)
for input_data in X:
prediction = nn.forward(input_data)
print(f"Input: {input_data}, Prediction: {prediction[0]}")
This example demonstrates how concepts from neuroscience have influenced the development of artificial neural networks, showcasing the power of cross-disciplinary learning in driving technological innovation.
Adaptability and Versatility
In the fast-paced world of technology, adaptability is crucial. Cross-disciplinary learning equips you with a diverse skill set that allows you to quickly adapt to new technologies and changing industry demands. This versatility is particularly valuable in the job market, where employers often seek candidates who can bridge gaps between different domains and bring fresh perspectives to problem-solving.
For instance, a programmer with knowledge of business principles may be better equipped to develop software solutions that align with an organization’s strategic goals. Similarly, a data scientist with an understanding of psychology can create more effective data visualization techniques that resonate with human perception and cognition.
Example: Combining Web Development and Data Science
Consider a scenario where you need to create a web application that not only presents data but also performs complex data analysis. By combining web development skills with data science knowledge, you can create a more comprehensive and powerful solution. Here’s a simple example using Python with Flask for web development and pandas for data analysis:
from flask import Flask, render_template
import pandas as pd
import plotly.express as px
import json
app = Flask(__name__)
@app.route('/')
def index():
# Load and process data
df = pd.read_csv('sales_data.csv')
monthly_sales = df.groupby('month')['sales'].sum().reset_index()
# Create a plot
fig = px.line(monthly_sales, x='month', y='sales', title='Monthly Sales')
graph_json = json.dumps(fig, cls=plotly.utils.PlotlyJSONEncoder)
# Perform some analysis
total_sales = df['sales'].sum()
avg_sales = df['sales'].mean()
best_month = monthly_sales.loc[monthly_sales['sales'].idxmax(), 'month']
return render_template('index.html',
graph_json=graph_json,
total_sales=total_sales,
avg_sales=avg_sales,
best_month=best_month)
if __name__ == '__main__':
app.run(debug=True)
This example demonstrates how combining web development skills (using Flask) with data analysis capabilities (using pandas and plotly) can create a more comprehensive solution, showcasing the benefits of cross-disciplinary learning in technology.
Improved Communication and Collaboration
Cross-disciplinary learning enhances your ability to communicate complex technical concepts to non-technical stakeholders. This skill is invaluable in today’s collaborative work environments, where technologists often need to work closely with professionals from diverse backgrounds such as marketing, finance, and management.
Moreover, understanding multiple disciplines allows you to act as a bridge between different teams, facilitating better collaboration and more efficient project execution. This ability to translate between technical and non-technical languages can significantly boost your career prospects and leadership potential in the tech industry.
Example: Explaining Technical Concepts to Non-Technical Audiences
Imagine you need to explain the concept of encryption to a non-technical audience. By drawing analogies from everyday life and using cross-disciplinary knowledge, you can make the explanation more accessible:
“Encryption is like sending a secret message in a locked box. You (the sender) put your message in the box and lock it with a special key. Then you send the locked box to your friend (the recipient). Only your friend, who has the matching key, can unlock the box and read the message. Anyone who intercepts the box along the way can’t read the message because they don’t have the key.
In the digital world, the ‘box’ is a mathematical algorithm, and the ‘key’ is a very large number. The strength of the encryption depends on how complex the lock (algorithm) is and how long the key (number) is. This is why we often hear about ‘256-bit encryption’ – it refers to the length of the key, with longer keys generally providing stronger security.”
This explanation uses analogies from physical security to explain digital encryption, demonstrating how cross-disciplinary knowledge can aid in communicating complex technical concepts to diverse audiences.
Enhanced Career Opportunities
Cross-disciplinary learning can significantly enhance your career prospects in the technology sector. Many emerging roles in tech require a combination of skills from different disciplines. For example:
- UX/UI designers need to understand both design principles and programming basics.
- Data scientists often combine statistics, programming, and domain-specific knowledge.
- DevOps engineers need to understand both software development and IT operations.
- AI ethicists combine knowledge of artificial intelligence with philosophy and ethics.
By developing a cross-disciplinary skill set, you position yourself for these emerging roles and increase your value in the job market. Additionally, this diverse knowledge base can help you identify unique career paths that align with your interests and strengths.
Case Study: The Rise of FinTech
The financial technology (FinTech) industry is a prime example of how cross-disciplinary skills can lead to new career opportunities. FinTech combines finance, technology, and often regulatory knowledge. Professionals in this field need to understand:
- Financial concepts and regulations
- Software development and cybersecurity
- Data analysis and machine learning
- User experience design
For instance, developing a blockchain-based financial application requires knowledge of cryptography, distributed systems, financial regulations, and often smart contract programming. Here’s a simple example of a smart contract written in Solidity, the programming language used for Ethereum blockchain:
pragma solidity ^0.8.0;
contract SimpleBank {
mapping(address => uint) private balances;
function deposit() public payable {
balances[msg.sender] += msg.value;
}
function withdraw(uint amount) public {
require(balances[msg.sender] >= amount, "Insufficient balance");
balances[msg.sender] -= amount;
payable(msg.sender).transfer(amount);
}
function getBalance() public view returns (uint) {
return balances[msg.sender];
}
}
This example demonstrates how knowledge of programming, finance, and blockchain technology comes together in the FinTech industry, highlighting the career opportunities that arise from cross-disciplinary learning.
Deeper Understanding of Technology’s Impact
Cross-disciplinary learning in technology helps you understand the broader implications and potential impacts of technological advancements on society, economics, and human behavior. This holistic perspective is crucial for developing responsible and ethical technology solutions.
For example, understanding the societal implications of artificial intelligence requires knowledge not just of machine learning algorithms, but also of ethics, psychology, and sociology. Similarly, developing sustainable technology solutions requires an understanding of environmental science alongside computer science.
Example: Ethical Considerations in AI Development
Consider the development of a facial recognition system. While the technical implementation might be straightforward, the ethical implications are complex. Here’s a simple example of how you might incorporate ethical considerations into your code:
import face_recognition
import cv2
import numpy as np
def detect_and_blur_faces(image_path, save_path):
# Load the image
image = face_recognition.load_image_file(image_path)
# Find all face locations in the image
face_locations = face_recognition.face_locations(image)
# Convert the image to a PIL-format image so that we can draw on it
pil_image = Image.fromarray(image)
draw = ImageDraw.Draw(pil_image)
for face_location in face_locations:
top, right, bottom, left = face_location
# Draw a box around the face
draw.rectangle(((left, top), (right, bottom)), outline=(0, 0, 255))
# Blur the face
face_image = image[top:bottom, left:right]
face_image = cv2.GaussianBlur(face_image, (99, 99), 30)
image[top:bottom, left:right] = face_image
# Save the resulting image
cv2.imwrite(save_path, image)
# Usage
detect_and_blur_faces('input_image.jpg', 'output_image.jpg')
In this example, instead of simply identifying faces, the program blurs them to protect privacy. This demonstrates how ethical considerations (in this case, privacy concerns) can be incorporated into technical solutions, showcasing the importance of cross-disciplinary understanding in technology development.
Continuous Learning and Personal Growth
Embracing cross-disciplinary learning fosters a mindset of continuous learning and personal growth. As you explore connections between different fields, you’ll likely discover new areas of interest and opportunities for further development. This approach to learning can help maintain your enthusiasm and motivation in your technology career, preventing burnout and encouraging lifelong learning.
Platforms like AlgoCademy, which focus on coding education and programming skills development, can be excellent resources for this continuous learning journey. They often provide interactive coding tutorials and resources that cover a wide range of topics, allowing learners to explore different areas of technology and discover new interests.
Strategies for Effective Cross-Disciplinary Learning
To make the most of cross-disciplinary learning in technology, consider the following strategies:
- Identify Complementary Fields: Look for disciplines that naturally complement your primary area of focus in technology. For example, if you’re interested in AI, consider studying neuroscience or cognitive psychology.
- Leverage Online Learning Platforms: Use platforms like AlgoCademy, Coursera, or edX to explore courses from various disciplines related to technology.
- Participate in Interdisciplinary Projects: Seek out projects or hackathons that require collaboration between different fields.
- Read Widely: Explore books, articles, and research papers from various disciplines to broaden your knowledge base.
- Attend Diverse Conferences and Workshops: Participate in events that bring together professionals from different backgrounds.
- Practice Applied Learning: Try to apply concepts from one discipline to problems in another. For example, use design thinking principles in software development.
- Engage in Community and Forums: Join online communities or forums where professionals from different backgrounds discuss technology-related topics.
Conclusion
Cross-disciplinary learning in technology offers numerous benefits, from enhanced problem-solving skills and increased creativity to improved career opportunities and a deeper understanding of technology’s impact on society. By embracing this approach to learning, you can develop a more holistic understanding of technology and its applications, positioning yourself for success in an increasingly interconnected and rapidly evolving tech landscape.
Platforms like AlgoCademy play a crucial role in this journey by providing structured learning paths and resources that can help you explore various aspects of technology and develop your cross-disciplinary skills. As you progress in your learning journey, remember that the ability to connect ideas across different fields is not just a valuable skill – it’s a catalyst for innovation and a key driver of technological progress.
Embrace the opportunities for cross-disciplinary learning, stay curious, and continue to explore the fascinating intersections between technology and other fields of knowledge. By doing so, you’ll not only enhance your capabilities as a technologist but also contribute to the broader advancement of technology and its positive impact on the world.