The Beginner’s Guide to Data Structures: Building Blocks for Efficient Programming
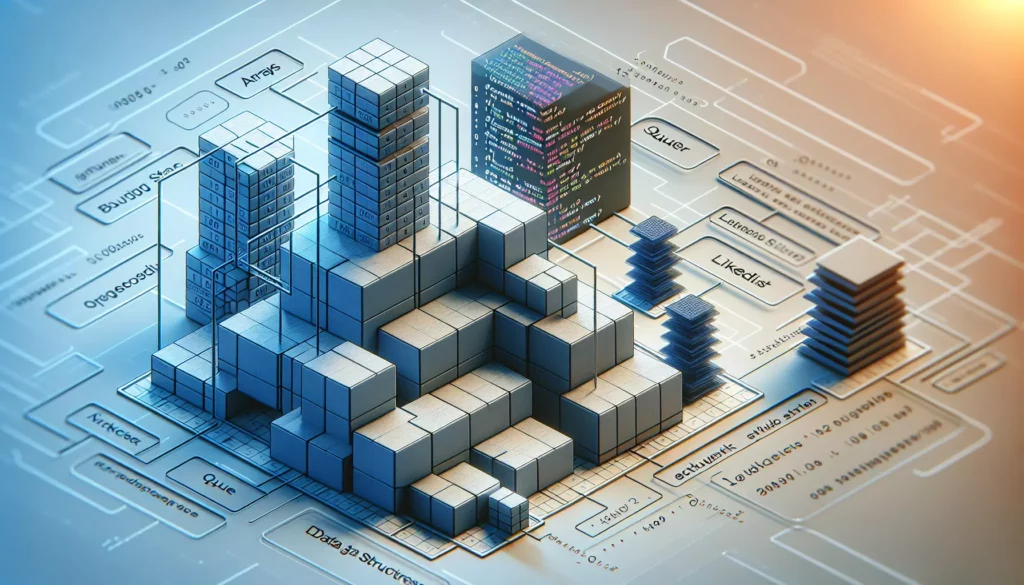
Welcome to the world of data structures! If you’re just starting your journey in computer science or looking to enhance your programming skills, understanding data structures is crucial. They are the fundamental building blocks that allow us to organize and manage data efficiently in our programs. In this comprehensive guide, we’ll explore the basics of data structures, their importance, and how they can help you become a more effective programmer.
Table of Contents
- What Are Data Structures?
- The Importance of Data Structures
- Types of Data Structures
- Arrays
- Linked Lists
- Stacks
- Queues
- Trees
- Graphs
- Hash Tables
- Choosing the Right Data Structure
- Implementing Data Structures
- Practicing with Data Structures
- Conclusion
1. What Are Data Structures?
Data structures are specialized formats for organizing, processing, retrieving, and storing data. They provide a way to manage large amounts of data efficiently for uses such as large databases and internet indexing services. Data structures serve as the basis for abstract data types (ADT) and are essential components in algorithm design and implementation.
Think of data structures as containers that store and organize data in a specific way. Just as you might use different types of containers to store various items in your home, different data structures are suited for different types of data and operations.
2. The Importance of Data Structures
Understanding data structures is crucial for several reasons:
- Efficiency: Proper use of data structures can significantly improve the efficiency of your algorithms, both in terms of time and space complexity.
- Organization: Data structures help in organizing and managing data in a way that makes it easy to access and manipulate.
- Reusability: Once you understand common data structures, you can reuse them in various programming scenarios, saving time and effort.
- Problem-solving: Many complex problems can be solved more easily by choosing the right data structure.
- Interview preparation: Data structures are a common topic in technical interviews, especially for positions at major tech companies.
3. Types of Data Structures
Data structures can be broadly categorized into two types:
- Linear data structures: Elements are arranged in a sequential manner, where each element is connected to its previous and next elements. Examples include arrays, linked lists, stacks, and queues.
- Non-linear data structures: Elements are not organized sequentially. Instead, they are arranged in a hierarchical manner where one element can be connected to several other elements. Examples include trees and graphs.
Let’s dive deeper into some of the most common data structures you’ll encounter as a beginner.
4. Arrays
Arrays are one of the simplest and most widely used data structures. They store elements of the same data type in contiguous memory locations.
Key characteristics of arrays:
- Fixed size (in most programming languages)
- Elements are accessed using an index
- Efficient for random access of elements
- Poor for inserting or deleting elements, especially in the middle of the array
Example of array declaration in Python:
numbers = [1, 2, 3, 4, 5]
print(numbers[2]) # Output: 3
5. Linked Lists
Linked lists consist of nodes, where each node contains a data field and a reference (or link) to the next node in the sequence.
Key characteristics of linked lists:
- Dynamic size
- Efficient for insertion and deletion operations
- Less efficient for random access of elements
- Requires extra memory for storing references
Example of a simple linked list node in Python:
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def append(self, data):
new_node = Node(data)
if not self.head:
self.head = new_node
return
current = self.head
while current.next:
current = current.next
current.next = new_node
6. Stacks
Stacks follow the Last-In-First-Out (LIFO) principle, where the last element added is the first one to be removed.
Key characteristics of stacks:
- Elements can only be added or removed from the top
- Useful for implementing undo/redo functionality, parsing expressions, and managing function calls
- Main operations: push (add) and pop (remove)
Example of a stack implementation in Python:
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
if not self.is_empty():
return self.items.pop()
def is_empty(self):
return len(self.items) == 0
def peek(self):
if not self.is_empty():
return self.items[-1]
def size(self):
return len(self.items)
7. Queues
Queues follow the First-In-First-Out (FIFO) principle, where the first element added is the first one to be removed.
Key characteristics of queues:
- Elements are added at the rear and removed from the front
- Useful for managing tasks in multi-threading, implementing buffers, and breadth-first search algorithms
- Main operations: enqueue (add) and dequeue (remove)
Example of a queue implementation in Python:
from collections import deque
class Queue:
def __init__(self):
self.items = deque()
def enqueue(self, item):
self.items.append(item)
def dequeue(self):
if not self.is_empty():
return self.items.popleft()
def is_empty(self):
return len(self.items) == 0
def size(self):
return len(self.items)
8. Trees
Trees are hierarchical data structures consisting of nodes connected by edges. The topmost node is called the root, and nodes with no children are called leaves.
Key characteristics of trees:
- Non-linear structure
- Efficient for searching and sorting operations
- Used in file systems, organization charts, and decision-making processes
- Common types include binary trees, binary search trees, and balanced trees (e.g., AVL trees, Red-Black trees)
Example of a binary tree node in Python:
class TreeNode:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
class BinaryTree:
def __init__(self):
self.root = None
def insert(self, value):
if not self.root:
self.root = TreeNode(value)
else:
self._insert_recursive(self.root, value)
def _insert_recursive(self, node, value):
if value < node.value:
if node.left is None:
node.left = TreeNode(value)
else:
self._insert_recursive(node.left, value)
else:
if node.right is None:
node.right = TreeNode(value)
else:
self._insert_recursive(node.right, value)
9. Graphs
Graphs consist of vertices (or nodes) connected by edges. They are used to represent networks, relationships, and complex systems.
Key characteristics of graphs:
- Can be directed (edges have a direction) or undirected
- Can be weighted (edges have associated values) or unweighted
- Used in social networks, map applications, and recommendation systems
- Common representations include adjacency lists and adjacency matrices
Example of a simple graph implementation using an adjacency list in Python:
class Graph:
def __init__(self):
self.graph = {}
def add_vertex(self, vertex):
if vertex not in self.graph:
self.graph[vertex] = []
def add_edge(self, vertex1, vertex2):
if vertex1 not in self.graph:
self.add_vertex(vertex1)
if vertex2 not in self.graph:
self.add_vertex(vertex2)
self.graph[vertex1].append(vertex2)
self.graph[vertex2].append(vertex1)
def get_vertices(self):
return list(self.graph.keys())
def get_edges(self):
edges = []
for vertex in self.graph:
for neighbor in self.graph[vertex]:
edges.append((vertex, neighbor))
return edges
10. Hash Tables
Hash tables, also known as hash maps or dictionaries, are data structures that store key-value pairs and provide fast access to values based on their keys.
Key characteristics of hash tables:
- Efficient for insertion, deletion, and lookup operations (average-case O(1) time complexity)
- Uses a hash function to compute an index for each key
- Handles collisions through techniques like chaining or open addressing
- Widely used in database indexing, caches, and symbol tables in compilers
Example of using a hash table (dictionary) in Python:
phone_book = {
"Alice": "123-456-7890",
"Bob": "987-654-3210",
"Charlie": "555-555-5555"
}
print(phone_book["Alice"]) # Output: 123-456-7890
phone_book["David"] = "111-222-3333"
print(phone_book) # Output: {'Alice': '123-456-7890', 'Bob': '987-654-3210', 'Charlie': '555-555-5555', 'David': '111-222-3333'}
11. Choosing the Right Data Structure
Selecting the appropriate data structure for a given problem is crucial for developing efficient algorithms. Consider the following factors when choosing a data structure:
- Type of operations: What are the most frequent operations you need to perform? (e.g., insertion, deletion, search)
- Time complexity: How fast do you need these operations to be?
- Space complexity: How much memory can you afford to use?
- Data size: How much data will you be working with?
- Ease of implementation: How complex is the data structure to implement and maintain?
Here’s a quick reference for common operations and their time complexities for different data structures:
Data Structure | Access | Search | Insertion | Deletion |
---|---|---|---|---|
Array | O(1) | O(n) | O(n) | O(n) |
Linked List | O(n) | O(n) | O(1) | O(1) |
Stack | O(n) | O(n) | O(1) | O(1) |
Queue | O(n) | O(n) | O(1) | O(1) |
Binary Search Tree (balanced) | O(log n) | O(log n) | O(log n) | O(log n) |
Hash Table | N/A | O(1) average | O(1) average | O(1) average |
12. Implementing Data Structures
Implementing data structures from scratch is an excellent way to deepen your understanding of how they work. Here are some tips for implementing data structures:
- Start simple: Begin with basic data structures like arrays and linked lists before moving on to more complex ones.
- Use object-oriented programming: Implement data structures as classes to encapsulate their behavior and properties.
- Write test cases: Create unit tests to verify that your implementation works correctly for various scenarios.
- Consider edge cases: Think about how your data structure should behave with empty inputs, large datasets, or unusual inputs.
- Optimize gradually: First focus on creating a correct implementation, then optimize for performance if necessary.
Here’s an example of implementing a simple binary search tree in Python:
class TreeNode:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
class BinarySearchTree:
def __init__(self):
self.root = None
def insert(self, value):
if not self.root:
self.root = TreeNode(value)
else:
self._insert_recursive(self.root, value)
def _insert_recursive(self, node, value):
if value < node.value:
if node.left is None:
node.left = TreeNode(value)
else:
self._insert_recursive(node.left, value)
else:
if node.right is None:
node.right = TreeNode(value)
else:
self._insert_recursive(node.right, value)
def search(self, value):
return self._search_recursive(self.root, value)
def _search_recursive(self, node, value):
if node is None or node.value == value:
return node
if value < node.value:
return self._search_recursive(node.left, value)
return self._search_recursive(node.right, value)
def inorder_traversal(self):
result = []
self._inorder_recursive(self.root, result)
return result
def _inorder_recursive(self, node, result):
if node:
self._inorder_recursive(node.left, result)
result.append(node.value)
self._inorder_recursive(node.right, result)
# Example usage
bst = BinarySearchTree()
bst.insert(5)
bst.insert(3)
bst.insert(7)
bst.insert(1)
bst.insert(9)
print(bst.inorder_traversal()) # Output: [1, 3, 5, 7, 9]
print(bst.search(7).value) # Output: 7
print(bst.search(4)) # Output: None
13. Practicing with Data Structures
To become proficient with data structures, regular practice is essential. Here are some ways to improve your skills:
- Solve coding problems: Websites like LeetCode, HackerRank, and CodeSignal offer a wide range of problems that involve data structures.
- Implement standard algorithms: Try implementing common algorithms like sorting (e.g., quicksort, mergesort) and graph traversals (e.g., depth-first search, breadth-first search) using different data structures.
- Build projects: Create small projects that utilize various data structures, such as a task manager using a priority queue or a simple database using a hash table.
- Participate in coding competitions: Platforms like Codeforces and TopCoder host regular coding contests that often involve data structure problems.
- Review and analyze existing code: Study open-source projects or standard library implementations to see how data structures are used in real-world applications.
Remember to start with simpler problems and gradually increase the difficulty as you become more comfortable with different data structures.
14. Conclusion
Data structures are fundamental building blocks in computer science and software development. Understanding and effectively using data structures can significantly improve your problem-solving skills and the efficiency of your programs. As a beginner, focus on mastering the basic data structures like arrays, linked lists, stacks, and queues before moving on to more complex ones like trees, graphs, and hash tables.
Keep in mind that choosing the right data structure for a given problem is often more important than micro-optimizations in your code. By practicing regularly and working on diverse problems, you’ll develop an intuition for selecting the most appropriate data structure for different scenarios.
As you continue your journey in programming and computer science, you’ll find that a solid understanding of data structures will serve you well in various aspects of software development, from writing efficient algorithms to designing scalable systems. So, keep learning, practicing, and exploring the fascinating world of data structures!