The Art of Writing Test Cases on the Whiteboard: A Step-by-Step Guide
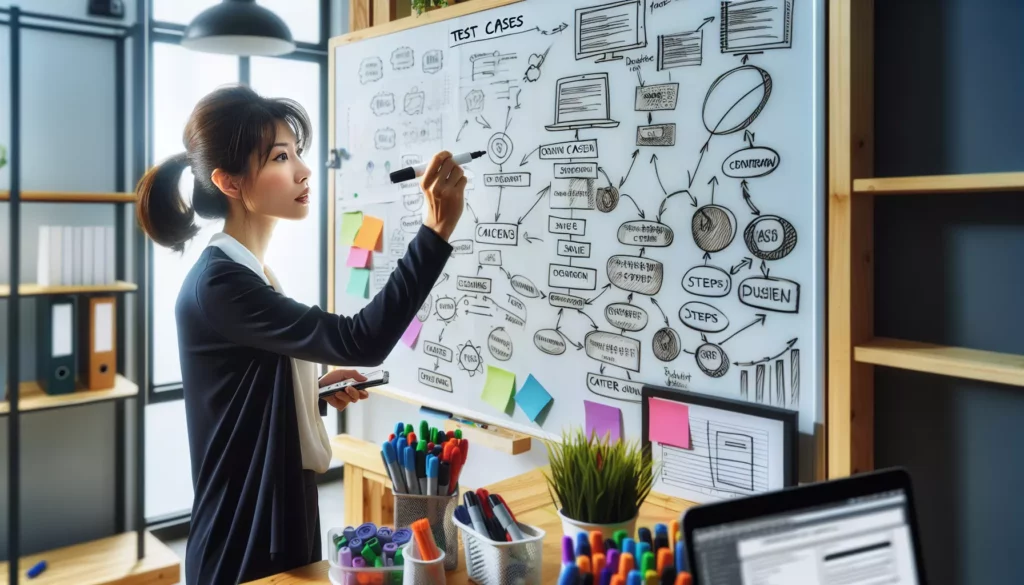
In the world of software development and technical interviews, particularly for positions at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), the ability to write effective test cases on a whiteboard is a crucial skill. This process not only demonstrates your problem-solving abilities but also showcases your attention to detail and your understanding of potential edge cases. In this comprehensive guide, we’ll walk you through the art of writing test cases on a whiteboard, providing you with the tools and techniques to excel in your next technical interview or coding challenge.
Why Writing Test Cases on a Whiteboard Matters
Before we dive into the step-by-step process, let’s understand why this skill is so important:
- Demonstrates thoroughness: By writing comprehensive test cases, you show that you consider various scenarios and don’t just focus on the happy path.
- Reveals thought process: The way you approach writing test cases gives interviewers insight into how you think and solve problems.
- Catches edge cases: Good test cases help identify potential issues that might not be immediately obvious.
- Improves code quality: Thinking about test cases before or while coding can lead to more robust and error-free solutions.
- Simulates real-world scenarios: In actual development work, considering test cases is a crucial part of the software development lifecycle.
Step 1: Understand the Problem
Before you start writing test cases, it’s crucial to have a clear understanding of the problem at hand. This step involves:
- Carefully read or listen to the problem statement: Make sure you grasp all the requirements and constraints.
- Ask clarifying questions: Don’t hesitate to seek clarification on any ambiguous points. This shows engagement and thoroughness.
- Identify the input and output: Clearly define what the function or program should take as input and what it should return as output.
- Note any constraints or special conditions: Pay attention to any limitations on input values, time complexity, or space complexity.
For example, if the problem is to write a function that finds the maximum element in an array, you might ask:
- Can the array contain negative numbers?
- What should the function return if the array is empty?
- Are there any constraints on the size of the array?
- Can the array contain non-integer elements?
Step 2: Start with the Basic Cases
Once you have a clear understanding of the problem, begin by writing down the most basic, straightforward test cases. These should cover the standard scenarios that the function or program is expected to handle correctly.
For our maximum element example, basic test cases might include:
1. Input: [1, 2, 3, 4, 5]
Expected Output: 5
2. Input: [5, 4, 3, 2, 1]
Expected Output: 5
3. Input: [1, 1, 1, 1, 1]
Expected Output: 1
Writing these basic cases serves several purposes:
- It helps you warm up and get into the problem-solving mindset.
- It provides a foundation for more complex cases.
- It ensures that your solution works for the most common scenarios.
Step 3: Consider Edge Cases
Edge cases are scenarios that occur at the extremes of the possible inputs. These are crucial for testing the robustness of your solution. When identifying edge cases, consider:
- Minimum and maximum values
- Empty or null inputs
- Single-element inputs
- Very large inputs
- Inputs that might cause overflow or underflow
For our maximum element example, edge cases might include:
4. Input: []
Expected Output: null (or throw an exception, depending on the requirements)
5. Input: [Integer.MAX_VALUE]
Expected Output: Integer.MAX_VALUE
6. Input: [Integer.MIN_VALUE]
Expected Output: Integer.MIN_VALUE
7. Input: [0]
Expected Output: 0
By including these edge cases, you demonstrate that you’ve thought about potential pitfalls and extreme scenarios that could cause issues in a real-world application.
Step 4: Explore Special Cases
Special cases are those that might be unique to the problem at hand or that test specific aspects of the algorithm or data structure you’re working with. These cases often reveal a deeper understanding of the problem domain.
For the maximum element problem, special cases might include:
8. Input: [-1, -2, -3, -4, -5]
Expected Output: -1
9. Input: [1, 2, 3, 3, 2, 1]
Expected Output: 3
10. Input: [1.5, 2.7, 3.2, 2.8]
Expected Output: 3.2 (if floating-point numbers are allowed)
These special cases test scenarios that might not be immediately obvious but are important for a comprehensive solution.
Step 5: Consider Performance Cases
For many coding problems, especially those asked in technical interviews, performance is a key consideration. Writing test cases that focus on performance can demonstrate your awareness of algorithmic efficiency.
Performance-focused test cases might include:
11. Input: [Large array with 1,000,000 elements]
Expected Output: Correct maximum value
Performance Expectation: Should complete in O(n) time
12. Input: [Array with repeated maximum value]
Expected Output: Correct maximum value
Performance Expectation: Should not require multiple passes through the array
When writing these cases, you don’t necessarily need to provide the full input array. Instead, describe the characteristics of the input and the expected performance.
Step 6: Organize Your Test Cases
As you write your test cases on the whiteboard, organization is key. A clear, well-structured presentation of your test cases not only makes them easier to understand but also demonstrates your ability to communicate effectively.
Here are some tips for organizing your test cases:
- Number your test cases: This makes it easy to refer to specific cases during discussion.
- Group similar cases: Keep basic cases, edge cases, special cases, and performance cases together.
- Use consistent formatting: Present each test case in the same format, typically with input, expected output, and any relevant notes.
- Leave space: If possible, leave some space between groups of test cases. This allows you to add more cases if you think of them later.
- Use clear handwriting: While it might seem obvious, legible handwriting is crucial when working on a whiteboard.
Step 7: Explain Your Thought Process
As you write your test cases, it’s beneficial to explain your thought process out loud. This gives the interviewer insight into your reasoning and problem-solving approach. Some points to cover in your explanation:
- Why you chose each test case
- What aspect of the problem each case is testing
- Any potential issues or edge cases you’re considering
- How your test cases relate to the requirements of the problem
For example, you might say something like:
“I’m including this test case with all negative numbers to ensure that the function correctly handles scenarios where the maximum value is negative. This is important because we didn’t specify that the input would only contain positive numbers.”
Step 8: Be Prepared to Add More Cases
As you work through the problem or discuss your solution with the interviewer, you might realize the need for additional test cases. Always be ready to add more cases to your list. This demonstrates flexibility and the ability to iterate on your solution.
You might say something like:
“Now that we’ve discussed the possibility of the input containing floating-point numbers, I’d like to add a test case to cover this scenario.”
Then, you could add a case like:
13. Input: [1.5, 2.7, 3.2, 2.8, 3.0]
Expected Output: 3.2
Step 9: Use Test Cases to Guide Your Solution
Once you’ve written a comprehensive set of test cases, use them to guide the development of your solution. As you write your code, refer back to your test cases to ensure that your implementation handles all the scenarios you’ve identified.
This approach has several benefits:
- It helps you stay focused on meeting all the requirements.
- It allows you to catch potential issues early in the development process.
- It demonstrates a methodical, test-driven approach to problem-solving.
Step 10: Verify Your Solution Against Test Cases
After implementing your solution, go through each test case and verify that your code produces the expected output. This process, often called “dry running,” is crucial for catching any errors or oversights in your implementation.
As you verify each case, you can mark it on the whiteboard, perhaps with a checkmark or by crossing it out. This visual indication of progress can be helpful for both you and the interviewer.
Common Pitfalls to Avoid
When writing test cases on a whiteboard, be aware of these common pitfalls:
- Focusing only on happy path scenarios: Make sure to include edge cases and potential error conditions.
- Overlooking performance considerations: Don’t forget to think about how your solution will perform with large or complex inputs.
- Ignoring constraints: Always keep in mind any constraints mentioned in the problem statement.
- Writing illegibly: Clear, readable handwriting is crucial when working on a whiteboard.
- Not explaining your thought process: Remember to vocalize your reasoning as you write your test cases.
- Being inflexible: Be open to adding or modifying test cases as you discuss the problem with the interviewer.
Conclusion
Writing effective test cases on a whiteboard is a valuable skill that can set you apart in technical interviews and improve your overall problem-solving abilities. By following this step-by-step guide, you can approach the task methodically and comprehensively.
Remember, the goal is not just to write test cases, but to demonstrate your thought process, attention to detail, and understanding of the problem domain. With practice, you’ll become more adept at quickly identifying important test cases and presenting them clearly and effectively.
As you continue to develop your coding skills, whether through platforms like AlgoCademy or through personal projects, make writing test cases an integral part of your problem-solving process. This habit will not only prepare you for technical interviews but also make you a more thorough and effective programmer in your day-to-day work.
Happy coding, and may your whiteboards always be filled with comprehensive, well-organized test cases!